Debugging Common AOP Pitfalls in Spring Applications
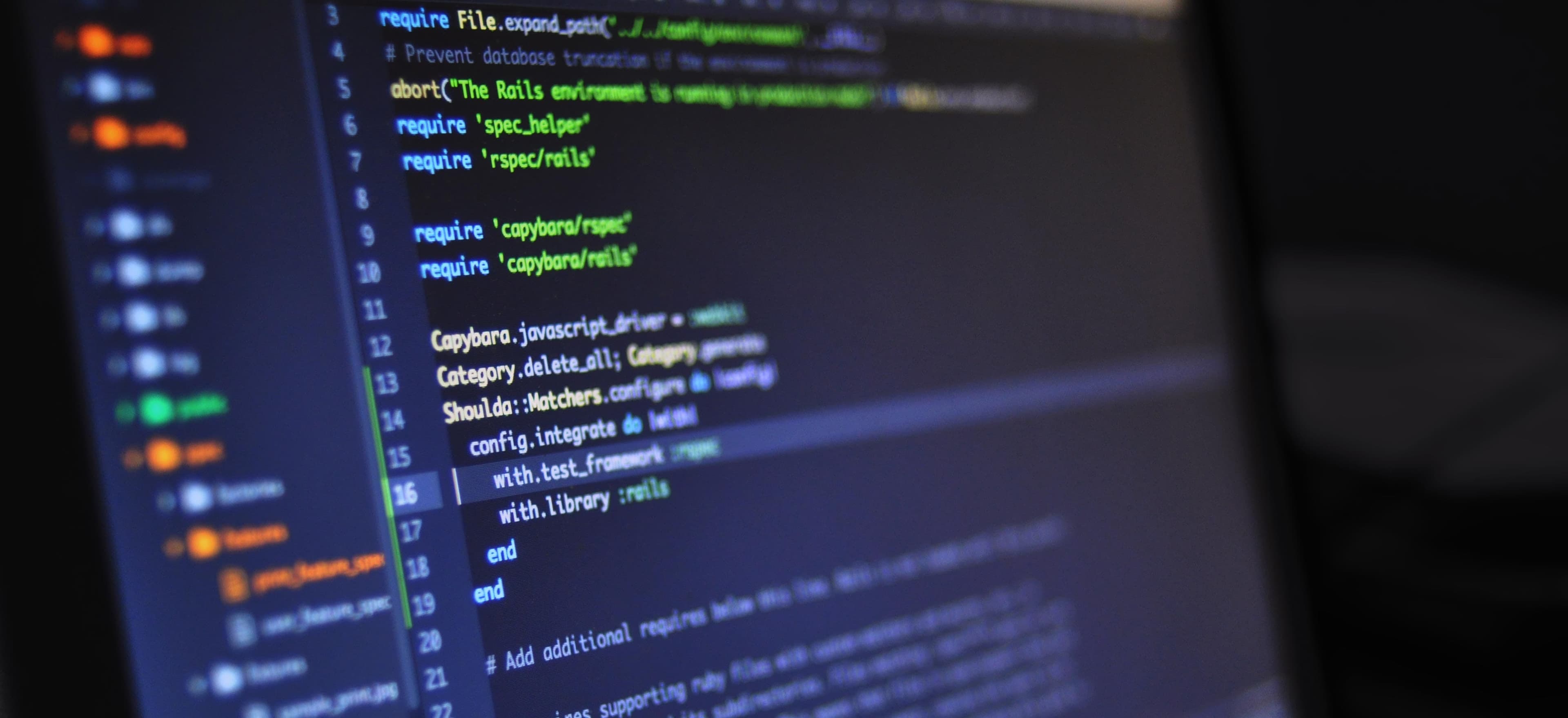
- Published on
Debugging Common AOP Pitfalls in Spring Applications
Aspect-Oriented Programming (AOP) is a powerful paradigm that helps in separating cross-cutting concerns from main business logic in a Spring application. While it comes with significant advantages, AOP can also introduce subtle bugs and issues that can be hard to diagnose. This blog post will delve into some common pitfalls associated with AOP in Spring applications and provide guidance on debugging these issues effectively.
Understanding AOP in Spring
Before we dive into the common pitfalls, let’s first summarize what aspects, join points, and pointcuts are:
-
Aspect: A module that encapsulates a concern that cuts across multiple classes. For example, logging or transaction management.
-
Join Point: A specific point in the execution of the program, like a method execution or an exception being thrown.
-
Pointcut: An expression that selects the join points where advice should be applied.
Example of AOP Setup
Here's a simple example of AOP configuration using Spring:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBeforeMethod() {
System.out.println("A method is about to be called");
}
}
In this example, we create an aspect (LoggingAspect
) that logs a message before any method in the service package executes. The @Before
annotation indicates that this advice should run before the method execution specified by the pointcut expression.
Common AOP Pitfalls
1. Aspects Not Being Applied
One of the most common issues developers face is that the aspects do not seem to be applied. This can be traced back to several causes:
-
Component Scanning Issues: Ensure that your aspect classes are within the scanned packages. If you are using Spring Boot, your main application class should be in a parent package with
@SpringBootApplication
. -
Proxy-based AOP: Spring AOP is proxy-based. If the target class is not a Spring bean, the aspect won’t work. Ensure the classes are annotated with
@Component
,@Service
, or similar annotations.
Solution:
Check your Spring configuration to ensure that you are scanning the right components.
@SpringBootApplication(scanBasePackages = "com.example")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2. Advices Not Executed in Self-Invocations
AOP does not apply advice on self-invocations. When a method in a Spring-managed bean calls another method of the same bean, the AOP proxy does not come into play. This leads to situations where you expect your advice to run, but it does not.
Solution:
To work around this, use an internal service to handle such calls or refactor your code.
@Service
public class MyService {
@Autowired
private MyService self; // Injecting self reference to enable AOP
public void firstMethod() {
// Do something
self.secondMethod(); // This call will trigger aspect
}
public void secondMethod() {
// Do something else
}
}
3. Parameter Binding Issues
If your aspect is designed to work with specific parameters, there might be cases where those parameters are not bound correctly. This is particularly common when using wildcards in pointcut expressions.
Solution:
Ensure that your pointcut definitions are specific enough to match the desired methods and their parameters.
@Before("execution(* com.example.service.UserService.saveUser(..)) && args(user)")
public void logSaveUser(JoinPoint joinPoint, User user) {
System.out.println("Saving user: " + user.getName());
}
4. Issues with Advice Order
When multiple advices apply to the same join points, the order in which they execute is important. If not configured correctly, it can cause unexpected results.
Solution:
In Spring, you can set precedence using the @Order
annotation. Lower values indicate higher priority.
@Aspect
@Order(1)
public class SecondaryAspect {
// ...
}
@Aspect
@Order(2)
public class PrimaryAspect {
// ...
}
5. Aspect Configuration in Proxied Beans
Sometimes, a bean defined in another context might not be proxied correctly. Beans defined outside the Spring context generally do not have AOP applied.
Solution:
Make sure all your beans are created and managed by the Spring container. Utilize annotations responsibly.
6. Performance Overhead
While AOP provides modularity, it can introduce a small overhead due to additional proxying. It is crucial to monitor for performance issues when many aspects are applied, especially in critical parts of the application.
Solution:
Profile the application to identify performance bottlenecks. Use conditional AOP to enable/disable aspects based on configuration.
Debugging AOP Issues
When debugging AOP issues in a Spring application, keep these practices in mind:
-
Enable AOP Proxy Debugging: Set the logging level for AOP-related packages to
DEBUG
to see detailed logging about what advice is applied and when.logging.level.org.springframework.aop=DEBUG
-
Use Spring AOP Tools: Leverage tools like Spring's AspectJ weaving if you need compile-time or load-time weaving for more complex scenarios.
-
Unit Testing: Create unit tests to verify that your aspects are applied correctly. Utilize mocking frameworks (like Mockito) to simulate calls and check if the aspects behave as expected.
A Final Look
Aspect-oriented programming is a powerful asset in the hands of developers working with Spring applications. Yet, it comes with its own set of challenges. Understanding these common pitfalls and their solutions can save you from a lot of head-scratching down the line.
To delve deeper into enhancing your understanding of Spring AOP, consider exploring the official Spring documentation for comprehensive insights or additional AOP tutorials to boost your practical skills.
By grasping how to navigate these common pitfalls, you can enhance the robustness and maintainability of your Spring applications. Happy coding!