Struggling with Asciidoctor Integration in Spring MVC?
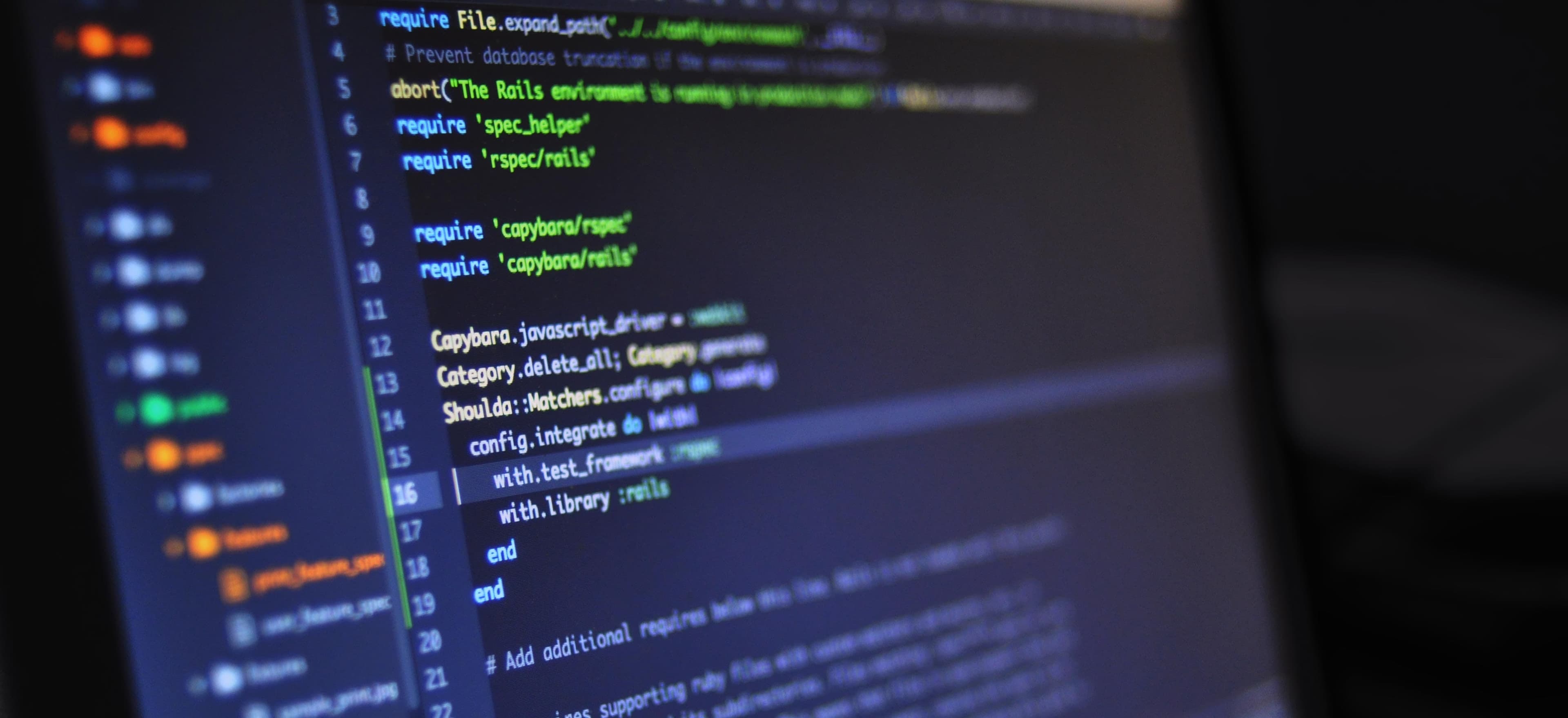
- Published on
Mastering Asciidoctor Integration in Spring MVC
In the landscape of modern software development, documentation is an inseparable aspect of building robust applications. One powerful tool for documentation is Asciidoctor, an open-source text document generation tool for writing and publishing professional documentation. If you are working with Spring MVC, you can integrate Asciidoctor seamlessly for creating stunning technical documents such as API documentation or user guides. This blog post will guide you in successfully integrating Asciidoctor into your Spring MVC project.
What is Asciidoctor?
Asciidoctor transforms AsciiDoc files into various formats, including HTML5, PDF, and more. It enables developers and technical writers to write their documentation in a simple, text-based format, which can then be converted into beautiful, readable output.
Why Use Asciidoctor?
- Simplicity: AsciiDoc is much easier to write compared to Markdown or other documentation formats.
- Flexibility: It supports complex layouts and structures, making it ideal for technical documentation.
- Tooling: Asciidoctor provides a rich set of tools that can be easily integrated into build systems.
Setting Up Spring MVC
Before diving into Asciidoctor integration, let’s set up a simple Spring MVC project if you don’t have one already. You can do this via Spring Initializr:
- Visit Spring Initializr.
- Choose your preferred project metadata.
- Add
Spring Web
in dependencies. - Click on
Generate
, which will download a ZIP file containing your project setup.
Once you have your project, you can structure it with the necessary MVC components.
Basic Spring MVC Components
Here's a quick overview of how your main components may look:
1. Application.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Why: This class serves as the entry point for your Spring application. The @SpringBootApplication
annotation enables component scanning and auto-configuration.
2. HomeController.java
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HomeController {
@GetMapping("/")
public String home() {
return "index"; // returns index.html
}
}
Why: This controller handles the home route and directs to the index.html
view.
2. Adding Asciidoctor Dependency
Next, let’s add Asciidoctor into your project. You can achieve this by including the Asciidoctor Maven plugin. Open your pom.xml
and add the following dependency:
<dependency>
<groupId>org.asciidoctor</groupId>
<artifactId>asciidoctor-spring5</artifactId>
<version>2.3.1</version>
</dependency>
Why: This dependency provides integration between Asciidoctor and Spring MVC, allowing us to render AsciiDoc files as HTML pages.
3. Configuring the Build Tool
In order to use the Asciidoctor plugin effectively, ensure that your Maven build is configured properly. Here’s how to add the Asciidoctor plugin to your pom.xml
.
<build>
<plugins>
<plugin>
<groupId>org.asciidoctor</groupId>
<artifactId>asciidoctor-maven-plugin</artifactId>
<version>2.3.1</version>
<configuration>
<outputDir>${project.build.directory}/asciidoc</outputDir>
<strip-header>true</strip-header>
</configuration>
</plugin>
</plugins>
</build>
Why: This configuration specifies where the output files will be generated and ensures that unnecessary headers in the HTML output are stripped for clarity.
4. Writing AsciiDoc Files
Now that everything is set up, you can start writing your documentation. Create a new directory src/main/asciidoc
and add a file named documentation.adoc
:
= My Documentation
:toc: macro
== Introduction
This is a sample documentation generated using Asciidoctor and Spring MVC.
== Features
* Easy to read
* Simple syntax
* Multiple output formats
Why: This is the core content of your documentation, written in a clear, structured format that will be converted to HTML.
5. Rendering Documentation in Spring MVC
To make your newly created Asciidoc content accessible via your Spring MVC application, you’ll need to create a view controller. Here’s how to add a new controller:
DocumentationController.java
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class DocumentationController {
@GetMapping("/docs")
public String documentation() {
return "documentation"; // links to documentation.html
}
}
Why: This controller maps a /docs
route to serve your documentation.
6. Accessing Your Documentation
Now, with everything set up, you can run your Spring Boot application and access your documentation by navigating to http://localhost:8080/docs
.
7. Building and Viewing Documentation
To build the Asciidoctor documentation into HTML, run the Maven build command:
mvn clean install
This will generate your HTML files in target/asciidoc
. You can find documentation.html
in this directory, and you can open it in a web browser to see your beautiful documentation.
Additional Features
While the integration outlined above is quite powerful, Asciidoctor offers additional features worth exploring:
- Custom Styles: You can create custom CSS styles to enhance the presentation of your documentation.
- PDF Output: Asciidoctor can be configured to generate PDF files alongside HTML outputs.
- Built-in Extensions: Take advantage of the various built-in extensions to enhance the functionality of your documentation.
For more detailed features, check the official Asciidoctor User Manual.
Wrapping Up
Integrating Asciidoctor with Spring MVC allows you to create and serve documentation effortlessly. With the simplicity and flexibility that Asciidoctor offers, your project documentation can be both beautiful and informative.
As we have discussed, with just a few steps, writing documentation that keeps pace with your code becomes not only feasible but enjoyable. Start leveraging Asciidoctor in your projects today, and empower your software with the documentation it deserves!
Checkout our other articles