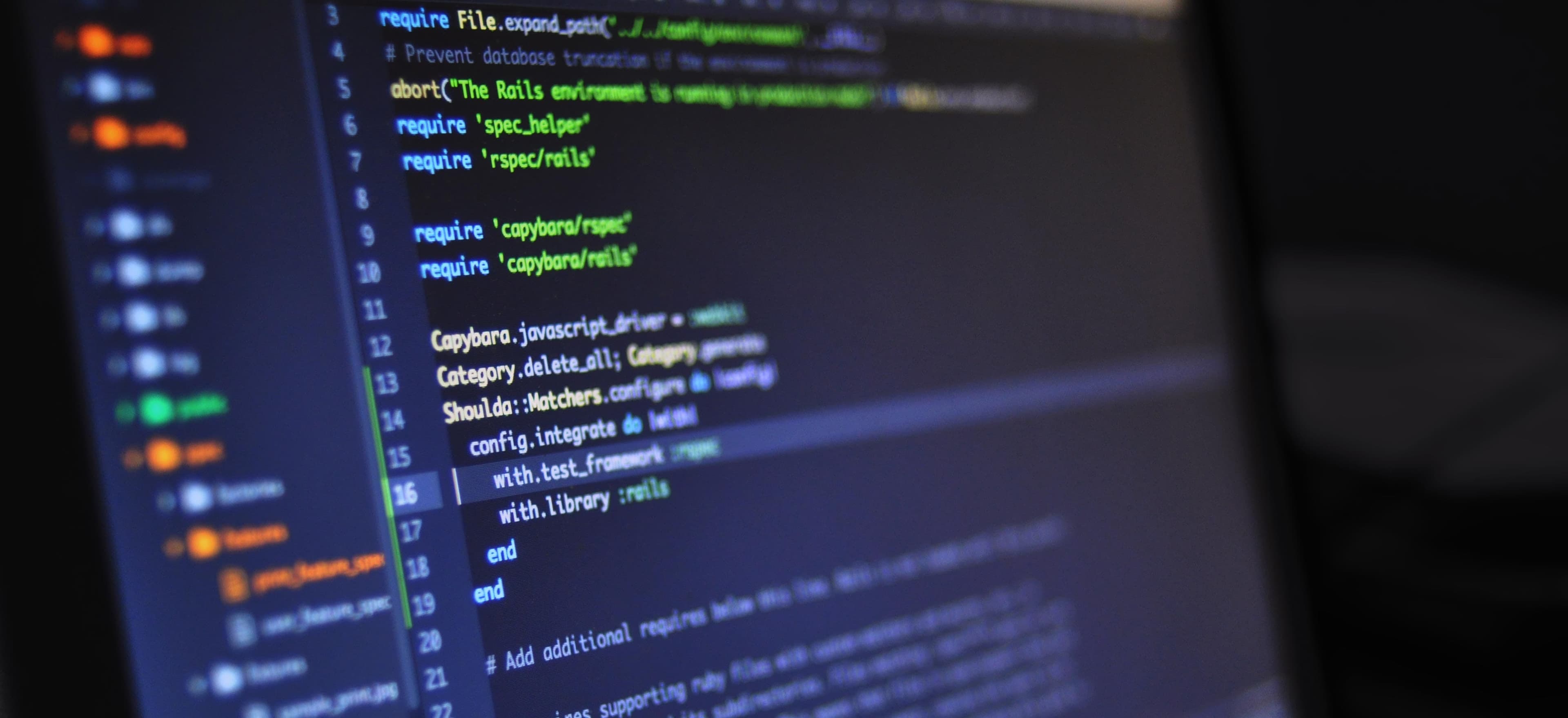
- Published on
Top 5 Common Spring Security Misconfigurations
Spring Security is a powerful and flexible authentication and access control framework for Java applications. While it's a robust tool, the configurational choices made during implementation can either strengthen your application's security posture or expose it to vulnerabilities. In this blog post, we will explore the top five common Spring Security misconfigurations, backed by code snippets, and provide actionable solutions to mitigate these issues.
1. Default Security Configurations
One of the most common misconfigurations is neglecting the default security settings provided by Spring Security. When you create a new Spring Boot application, it typically comes with some default security that may not suit your application’s needs.
Why It Matters
Using default settings might expose your application to unnecessary risks, such as weak password policies or excessive permissions for users.
What to Do
Customize the security settings to fit your application's requirements. Here’s a simple example of how to configure a basic authentication mechanism with custom user details:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
// Use in-memory authentication for demonstration purposes
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}admin").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll() // Public access to home
.anyRequest().authenticated() // All other requests require authentication
.and()
.formLogin().permitAll() // Allow all users to login
.and()
.logout().permitAll(); // Allow all users to logout
}
}
Using {noop}
tells Spring Security not to encode the password, which is acceptable for demonstration but should be replaced with a password encoder for production.
2. Ignoring HTTPS
Another prevalent misconfiguration is neglecting to enforce HTTPS across your application. This is often overlooked, especially in development environments.
Why It Matters
Transport Layer Security (TLS) provides a safe channel to prevent attackers from intercepting data (like passwords). Without HTTPS, user credentials and sensitive data can be transmitted over the network unencrypted.
What to Do
Enforce HTTPS by configuring Spring Security to redirect HTTP traffic to HTTPS. You can configure this in the HttpSecurity
object:
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.anyRequest()
.requiresSecure();
// Your existing security config goes here
}
This configuration ensures that all requests are served over HTTPS, enhancing the security of transmitted data.
3. CSRF Protection Disabled
Cross-Site Request Forgery (CSRF) is a type of attack where an unauthorized command is transmitted from a user that the web application trusts. Another frequent misconfiguration is disabling CSRF protection.
Why It Matters
Ignoring CSRF protection can lead to unauthorized actions performed on behalf of users without their consent, such as fund transfers or profile changes.
What to Do
Enable CSRF protection unless you have a valid reason to disable it. Here is how you can explicitly ensure that CSRF protection is enabled:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.and()
.authorizeRequests()
.anyRequest().authenticated();
}
The above configuration explicitly includes CSRF protection in your application. If you're using non-browser clients, make sure to implement CSRF tokens correctly.
4. Handling Passwords Insecurely
Frequently, applications mishandle user passwords, either by storing them in plaintext or employing weak hashing algorithms.
Why It Matters
Storing passwords insecurely can lead to severe consequences if an attacker gains access to your database. A compromised password could be used for unauthorized access to users' accounts.
What to Do
Always hash passwords using a strong algorithm. Spring Security provides support for BCrypt, which is a robust hashing algorithm. Here’s how to implement it:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
// Hashing a password before storing it
public String encodePassword(String rawPassword) {
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
return passwordEncoder.encode(rawPassword);
}
When authenticating users, compare the raw password with the hashed value:
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(encodePassword("password")).roles("USER")
.and()
.withUser("admin").password(encodePassword("admin")).roles("ADMIN");
}
This approach effectively secures passwords and prevents unauthorized access.
5. Inadequate User Role Management
Failing to manage user roles and authorities properly can lead to privilege escalation attacks. This mistake often arises from just assigning broad access roles like ROLE_USER
without further specificity.
Why It Matters
Granting excessive permissions can allow regular users to execute administrative functions or access sensitive resources.
What to Do
Use finer-grained control over user roles and permissions. For example:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
In this example, only users with the ADMIN
role can access /admin/**
, while the USER
role is required for /user/**
.
The Bottom Line
Spring Security is powerful, but with great power comes great responsibility. Misconfigurations can lead to vulnerabilities that compromise the integrity of your application. By ensuring that you avoid these common pitfalls, you can create a more secure environment for your users.
For further reading on Spring Security best practices, you can refer to the Spring Security Documentation. Investing time in understanding and configuring security applications is essential for protecting sensitive data and maintaining user trust. Remember, security is not a one-time setup, but an ongoing practice that requires continuous evaluation and updates.
By regularly revisiting your security configurations, staying informed on the latest vulnerabilities, and adapting to emerging threats, you can significantly enhance the security posture of your Spring applications.
Checkout our other articles