Designing Intuitive UI: JavaFX Challenges in Sci-Fi Games
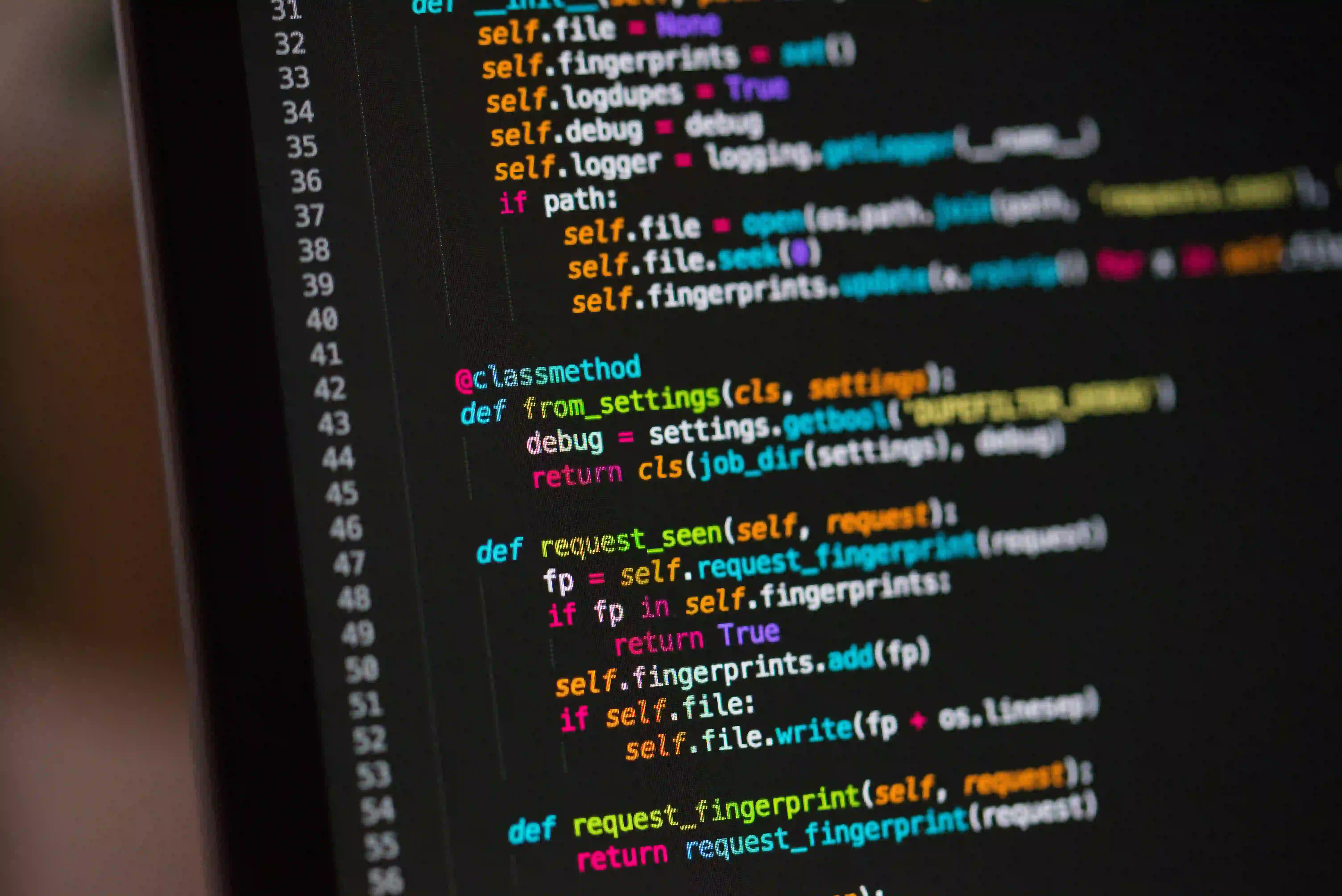
Designing Intuitive UI: JavaFX Challenges in Sci-Fi Games
Creating an engaging user interface (UI) for sci-fi games is a daunting task, especially when utilizing JavaFX. While JavaFX provides powerful tools for building compelling UIs, many challenges arise due to the complexity and uniqueness demanded by the sci-fi genre. In this blog post, we will explore the intricacies of building intuitive UIs for sci-fi games using JavaFX, discuss common challenges, and provide practical code snippets to guide you through the process.
Understanding the Basics of JavaFX
Before diving into the challenges, let's briefly cover JavaFX itself. JavaFX is a set of graphics and media packages that allows developers to design rich internet applications (RIAs) that can run on a variety of platforms. It provides a lightweight UI toolkit with a focus on features like properties, bindings, and events.
JavaFX makes creating sophisticated and modern UIs easier compared to its predecessors. The following is a basic setup of a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SciFiGameUI extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 600, 400);
primaryStage.setTitle("Sci-Fi Game UI");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This code snippet creates a simple JavaFX application. The start
method sets up the main UI elements, defining a StackPane
as the root layout. The Scene
contains the UI elements and is displayed in a Stage
, which acts as the primary window.
Challenges of Designing Intuitive UIs in Sci-Fi Games
Designing a user interface for a sci-fi game is different from traditional game genres due to unique thematic elements, advanced functionalities, and player expectations. Here are some critical challenges you might face:
1. Thematic Consistency
Challenge: In sci-fi games, maintaining a consistent thematic style across the UI is crucial. Color schemes, font choices, and graphical elements must reinforce the science fiction experience.
Solution: Employ a style guide that dictates the design elements. Use JavaFX’s CSS capabilities to create distinctive styles.
/* styles.css */
.root {
-fx-background-color: #000000; /* Deep space black */
}
.button {
-fx-background-color: #1e90ff; /* Dodger blue for futuristic look */
-fx-text-fill: white;
}
By leveraging CSS, you can elegantly define a UI theme that immerses players into the sci-fi world. Applying these styles within JavaFX can enhance the visual coherence across all components.
2. Complex Layouts
Challenge: Sci-fi games often feature dynamic and detailed environments requiring complex layouts. Managing elements effectively to respond to different screen resolutions and orientations can be tricky.
Solution: Utilize layout panes provided by JavaFX, such as GridPane
, VBox
, and HBox
. Here’s a layout snippet utilizing GridPane
:
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
// Inside your start method
GridPane grid = new GridPane();
Button btnStart = new Button("Start Game");
Button btnSettings = new Button("Settings");
Button btnExit = new Button("Exit");
grid.add(btnStart, 0, 0);
grid.add(btnSettings, 1, 0);
grid.add(btnExit, 2, 0);
root.getChildren().add(grid);
This example organizes buttons in a grid, providing a clean interface. Such layouts can be adjusted easily for various resolutions, ensuring intuitive navigation.
3. Information Overload
Challenge: Sci-fi games often require displaying a significant amount of information to players, from dashboards showing stats to maps and objectives. Too much information can overwhelm users.
Solution: Focus on a minimalistic design approach. Use visual hierarchies to differentiate between essential and auxiliary information. Implement tooltips for additional information without cluttering the UI.
Here’s how to add a tooltip to a button:
btnSettings.setTooltip(new Tooltip("Adjust game settings"));
This approach keeps the UI clean while still providing players with the information they need on demand.
4. Interactive Elements
Challenge: Sci-fi games frequently involve multiple interactive elements such as inventory systems, skill trees, and upgrade menus. Ensuring that these elements are user-friendly is vital.
Solution: JavaFX supports event handling that can be employed to create responsive interfaces. Here’s an example of adding event listeners:
btnStart.setOnAction(e -> {
// Logic to start the game
System.out.println("Game Started");
});
btnExit.setOnAction(e -> {
// Logic to exit the game
System.out.println("Game Exited");
});
Through event handling, gameplay can seamlessly connect to UI actions, providing players a smooth experience.
5. Responsive Design
Challenge: Players may be using devices with different screen sizes and resolutions. Designing an adaptable UI can be problematic.
Solution: JavaFX’s binding capabilities help create responsive UIs. You can bind component properties to adapt dynamically. For example, binding button size to the scene dimensions:
btnStart.setPrefWidth(scene.widthProperty().divide(3).doubleValue());
This code snippet ensures that the button adjusts its width based on the scene size, providing a flexible UI across different devices.
Incorporating Sci-Fi Elements
Use of Animations
Animations can add depth to your game UI. JavaFX supports rich animations that can enhance user experience. Here’s how to implement fade-in effect on a button:
import javafx.animation.FadeTransition;
import javafx.util.Duration;
// Add this after button creation
FadeTransition fade = new FadeTransition(Duration.seconds(1), btnStart);
fade.setFromValue(0);
fade.setToValue(1);
fade.play();
This code snippet gradually increases the button’s opacity, making the UI feel more vibrant. Dynamic animations can attract players' attention effectively.
Key Takeaways
Designing an intuitive user interface for sci-fi games using JavaFX presents a unique set of challenges. However, by understanding these challenges and employing best practices such as maintaining thematic consistency, managing complex layouts, focusing on user interaction, enabling responsive design, and incorporating animations, developers can craft an engaging and aesthetically pleasing UI.
As you embark on your journey to design your game UI, remember to iterate and seek player feedback. Your UI is a pivotal element of the player's experience, and ensuring that it enhances gameplay will lead to a more immersive and enjoyable experience.
For more in-depth tutorials on JavaFX, check out Official JavaFX Documentation, or explore community-driven projects on platforms like GitHub for real-world applications you can draw inspiration from.
With these insights, you’re one step closer to creating an unforgettable sci-fi gaming experience!
Additional Resources
- JavaFX CSS Reference Guide
- JavaFX Animation Documentation
- JavaFX Layout Panes Overview
Feel free to leave your thoughts or questions about creating UI in sci-fi games using JavaFX!