Common Pitfalls in Implementing Prototype Design Pattern
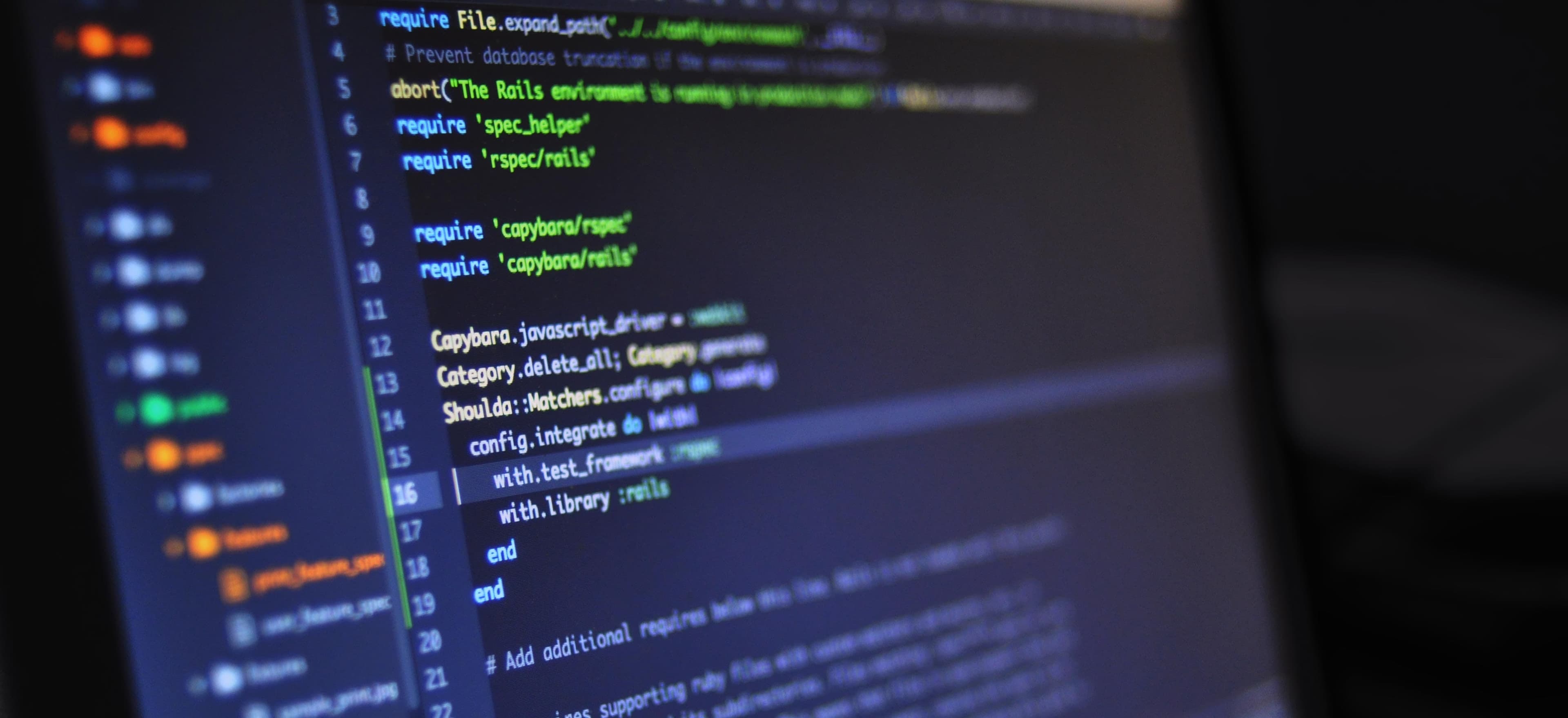
- Published on
Common Pitfalls in Implementing the Prototype Design Pattern
In the realm of software design patterns, the Prototype design pattern is a powerful tool that allows for the creation of new objects by copying existing ones. This pattern is most useful when the cost of creating an object is higher than copying an existing object. However, despite its advantages, developers often encounter several pitfalls when implementing this pattern. In this blog post, we will explore the Prototype design pattern, its benefits, and the common pitfalls you should avoid to ensure a smooth implementation.
What is the Prototype Design Pattern?
The Prototype design pattern falls under the category of creational design patterns. It offers a way to create a new object by copying an existing object (the prototype), rather than creating a new instance from scratch.
Key Components of the Prototype Pattern
- Prototype: An interface declaring the clonable operation.
- ConcretePrototype: The class that implements the Prototype interface and defines cloning logic.
- Client: The class that uses the prototype to create new objects based on existing ones.
Benefits of Using the Prototype Pattern
- Performance: Cloning an object can be more efficient than creating a new instance, especially for complex objects.
- Decoupling: It reduces dependencies by not requiring client code to know about specific classes.
- Dynamic Configuration: The prototype can be updated at runtime, enabling dynamic changes to configurations.
Common Pitfalls
While the Prototype design pattern has its merits, developers might face several challenges during implementation. Let's dive into some of the most common pitfalls and how to avoid them.
1. Ignoring Deep vs. Shallow Copying
One of the most significant pitfalls is neglecting the difference between shallow and deep copies. A shallow copy duplicates the object's structure but not the objects referenced within it. Conversely, a deep copy duplicates not only the object itself but all its nested objects.
Example
class Address {
String street;
String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public Address deepClone() {
return new Address(this.street, this.city);
}
}
class Person implements Cloneable {
String name;
Address address;
public Person(String name, Address address) {
this.name = name;
this.address = address;
}
@Override
protected Object clone() throws CloneNotSupportedException {
Person cloned = (Person) super.clone();
cloned.address = address.deepClone(); // Deep copy
return cloned;
}
}
Why This Matters
If you only implement a shallow copy in a scenario that requires a deep copy, changes made to the cloned object's nested objects will reflect in the original object, leading to surprising and hard-to-debug behavior.
2. Not Implementing the Cloneable Interface Properly
In Java, the Cloneable
interface must be implemented for an object to be cloned. Failing to do so often results in CloneNotSupportedException
.
public class Car implements Cloneable {
private String model;
public Car(String model) {
this.model = model;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
Why This Matters
Without properly implementing the Cloneable
interface, cloning will not work, forcing you to wrestle with exceptions in your code.
3. Failing to Override Clone Method in All Subclasses
When a superclass implements cloning, subclasses must also override the clone
method. Failing to provide specific cloning logic in subclasses may lead to incomplete object states.
class Bike extends Vehicle {
private String type;
public Bike(String type) {
this.type = type;
}
// Mistake: If we don't override clone, we receive a shallow clone of Vehicle without handling Bike-specific fields.
}
Why This Matters
Not overriding the clone
method in subclasses can lead to inherited state inconsistencies. Each subclass should know how to handle its specifics when cloning.
4. Violating the Principle of Encapsulation
When cloning objects, if you directly expose the internals (like member variables), you're breaking encapsulation principles. It's vital to ensure that internal state remains protected during cloning.
class Employee implements Cloneable {
private String name;
private int age;
// Getter methods
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
Why This Matters
Maintaining encapsulation ensures that the cloned object adheres to the same rules and logic dictated by your class design.
5. Forgetting to Handle Circular References
If an object contains circular references, cloning can lead to infinite recursion or stack overflow errors. Safeguarding against this during the cloning operation is crucial.
class Node {
Node next;
protected Object clone() throws CloneNotSupportedException {
Node clonedNode = (Node) super.clone();
clonedNode.next = this.next != null ? (Node) this.next.clone() : null; // Safely clone next node
return clonedNode;
}
}
Why This Matters
Circular references can cause severe runtime issues and must be handled appropriately to maintain code stability.
In Conclusion, Here is What Matters
The Prototype design pattern is a valuable addition to any developer's toolkit, offering unique advantages in terms of performance, flexibility, and ease of object creation. However, as we've outlined in this article, there are several common pitfalls that developers encounter during implementation. Key takeaways include understanding the nuances of shallow vs. deep copies, properly implementing the Cloneable
interface, appropriate handling of subclasses, maintaining encapsulation, and safeguarding against circular references.
By keeping these potential issues in mind, you can successfully leverage the Prototype pattern to improve your Java applications while avoiding common mistakes that could lead to complicated bugs.
For further reading on design patterns, you might want to check out Design Patterns: Elements of Reusable Object-Oriented Software by Gamma et al., which serves as a great foundational text for understanding and implementing various design patterns effectively.
Happy coding!
Checkout our other articles