Handling Message Duplication in SQS with Spring Boot
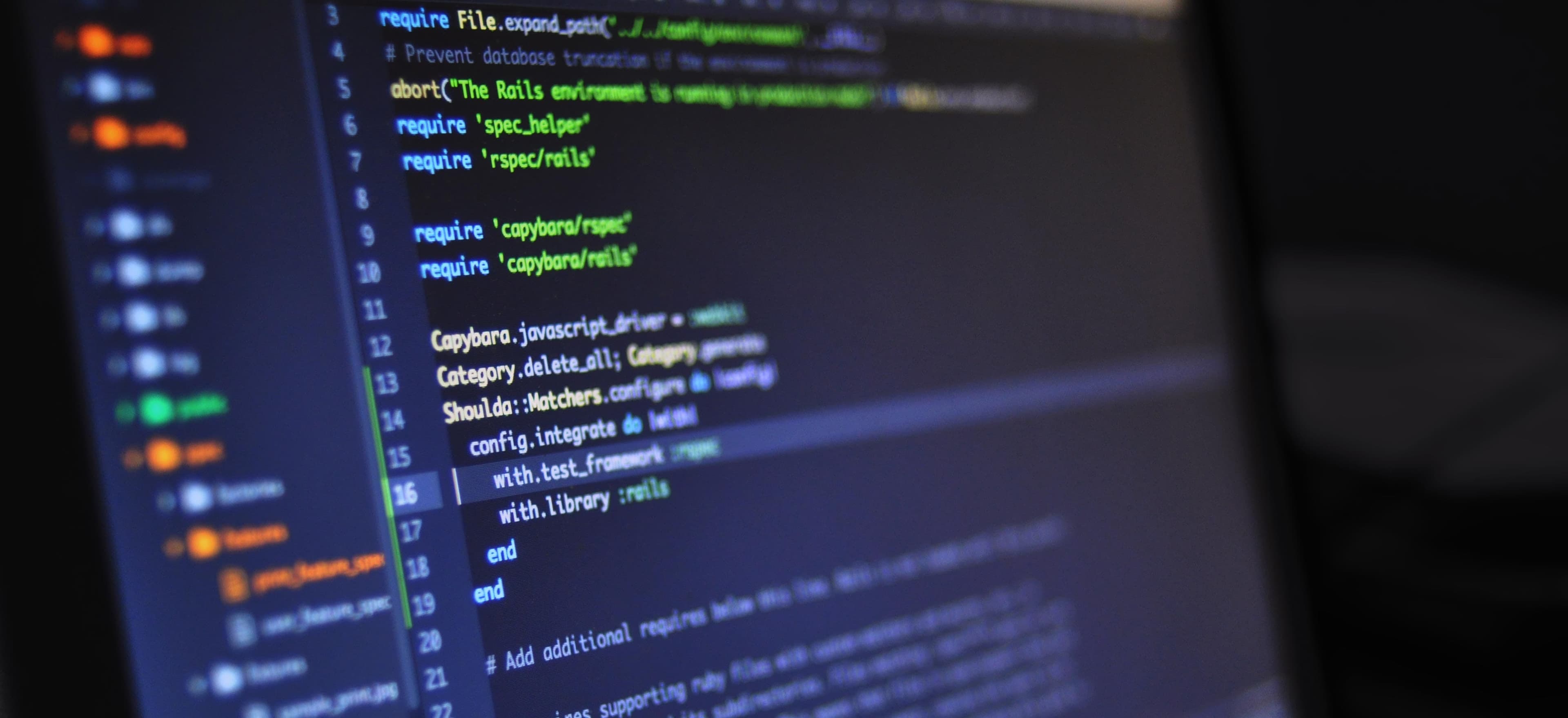
- Published on
Handling Message Duplication in SQS with Spring Boot
Amazon Simple Queue Service (SQS) provides a scalable and fully managed message queuing service that allows you to decouple and distribute microservices in a cloud environment. However, one of the challenges developers face when using SQS is message duplication. In a distributed system, it's common for messages to be delivered more than once, leading to potential inconsistencies and unintended side effects. In this blog post, we will explore effective strategies to handle message duplication in SQS using Spring Boot.
Understanding Message Duplication
Before we dive into the implementation details, it’s important to grasp why message duplication occurs in SQS. Some common reasons for duplicates include:
- Network Interruptions: If the network disconnects during the processing of a message, SQS assumes that the message was not processed and may deliver it again.
- Consumer Failures: If an application crashes after receiving a message but before successfully processing it, SQS may redeliver it.
- At-Least-Once Delivery: SQS uses an at-least-once delivery policy, meaning that messages may be delivered multiple times.
Understanding these mechanisms helps in designing a robust duplicate message handling system.
Strategies for Handling Message Duplication
1. Idempotency
One of the simplest and most effective ways to handle message duplication is to ensure your message processing logic is idempotent. This means that no matter how many times your processing logic is executed for a given message, the outcome will remain the same.
For example, consider a banking application where a message instructs to transfer an amount from one account to another. If this operation is executed multiple times due to duplication, it might lead to multiple deductions from the sender's account. To avoid this, you can implement a unique transaction ID in the message payload.
public class TransferRequest {
private String transactionId;
private String fromAccount;
private String toAccount;
private BigDecimal amount;
// Getters and Setters
}
In your service where you handle this request, you can maintain a record of processed transaction IDs:
@Service
public class TransferService {
private Set<String> processedTransactions = ConcurrentHashMap.newKeySet();
public void processTransfer(TransferRequest request) {
if (processedTransactions.contains(request.getTransactionId())) {
// Log duplicate message
return;
}
// Process the transfer
// Update accounts, etc.
// Mark this transaction as processed
processedTransactions.add(request.getTransactionId());
}
}
This approach ensures that even if the same message is processed multiple times, it only has an effect on your system once.
2. Deduplication Strategies
Another crucial approach is to implement a deduplication strategy. While the idempotency design can significantly mitigate the effects of duplicate messaging, having a centralized deduplication mechanism is vital.
AWS SQS supports a deduplication feature for FIFO queues using a MessageDeduplicationId
. This ID should be unique for every unique message but the same for duplicates. While the following example is specifically for FIFO queues, you can implement similar logic for standard queues, albeit manually.
Here is a sample implementation of a Spring Boot service that uses a FIFO queue with deduplication:
@Bean
public Queue queue() {
return QueueBuilder.durable("MyQueue.fifo")
.withArgument("FifoQueue", true)
.withArgument("ContentBasedDeduplication", true)
.build();
}
In your message listener:
@SqsListener("MyQueue.fifo")
public void processMessage(String message) {
// Deserialize message
// Call deduplication logic
}
Utilizing AWS's native deduplication capabilities minimizes the overhead on your application while providing built-in safeguards.
3. Tracking Processed Messages with a Database
If the application cannot fully guarantee idempotency, a practical solution is to persist processed message IDs in a centralized database. This can be particularly useful for long-running processes.
You can store processed messages in a database table with the following schema:
CREATE TABLE processed_messages (
id SERIAL PRIMARY KEY,
transaction_id VARCHAR(255) UNIQUE,
processed_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
The service would then check this table before processing any new messages.
For example:
@Repository
public interface ProcessedMessageRepository extends JpaRepository<ProcessedMessage, Long> {
Optional<ProcessedMessage> findByTransactionId(String transactionId);
}
@Service
public class EnhancedTransferService {
@Autowired
private ProcessedMessageRepository repository;
public void processTransfer(TransferRequest request) {
// Check if this transaction has already been processed
if (repository.findByTransactionId(request.getTransactionId()).isPresent()) {
// Log duplicate message
return;
}
// Process the transfer here...
// Save to the database to mark as processed
ProcessedMessage processedMessage = new ProcessedMessage();
processedMessage.setTransactionId(request.getTransactionId());
repository.save(processedMessage);
}
}
In this setup, you have an additional layer of safety by verifying the database before processing each message.
Monitoring for Duplicates
Monitoring your SQS queues is an essential practice not only for identifying duplicate messages but also for evaluating the overall health of your systems. Utilize AWS CloudWatch to set alarms and track metrics like NumberOfMessagesSent
, NumberOfMessagesReceived
, and ApproximateNumberOfMessagesVisible
. This can help you diagnose issues proactively.
Additional Considerations
- Configure Visibility Timeout: Ensure your visibility timeout is long enough for your consumers to process messages without the risk of duplication.
- Leverage Dead Letter Queues (DLQ): In case your consumer fails after processing duplicates, configure DLQs to capture these failed messages for later inspection.
- Error Handling: Implement resilient error handling to manage any issues arising during message processing.
A Final Look
Handling message duplication in SQS in a Spring Boot application can be achieved through several strategies such as idempotency, leveraging AWS features like deduplication, and maintaining a database of processed messages. By implementing these strategies, you can significantly mitigate the risks associated with message duplication, ensuring that your application remains robust and reliable.
For further reading, you might find these resources useful:
By following the guidelines outlined in this post, you will be better equipped to manage message duplication effectively and enhance your application's reliability.