Tackling Java Heap Space: Common Causes and Solutions
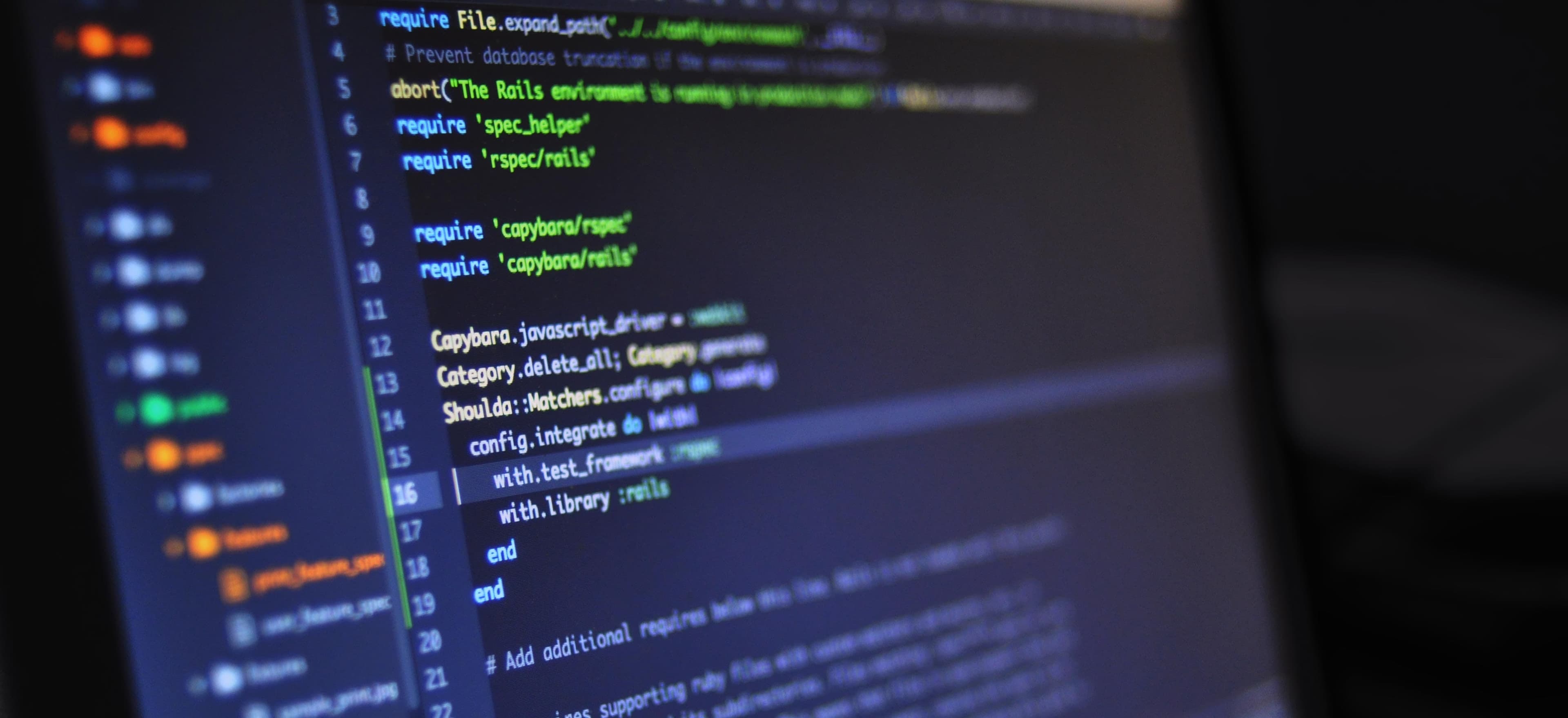
- Published on
Tackling Java Heap Space: Common Causes and Solutions
Java is the cornerstone of many software systems, powering everything from web applications to mobile apps. However, developers often encounter the dreaded OutOfMemoryError: Java heap space
. Understanding and resolving this issue is crucial for maintaining the performance and reliability of Java applications. In this article, we will explore the common causes of Java heap space issues and their solutions, enhancing your knowledge and equipping you with the tools to tackle this pervasive problem.
Understanding Heap Space
Before delving into the causes, it's essential to understand what Java heap space is. The Java Virtual Machine (JVM) uses heap space to allocate memory for all objects and class instances at runtime. This space is dynamically managed; however, if an application tries to use more memory than the heap can provide, an OutOfMemoryError
occurs.
The Structure of Heap Space
Java heap space can be divided into three main areas:
-
Young Generation: It is used to allocate memory for new objects. When the young generation is filled up, a minor garbage collection (GC) is triggered to free memory by removing objects that are no longer in use.
-
Old Generation (Tenured Generation): Long-lived objects are eventually promoted to the old generation. When this area becomes full, a major GC is executed, which can be more time-consuming than minor GCs.
-
Permanent Generation (MetaSpace): This area holds metadata such as method definitions and class data. Note that with Java 8 and later, the permanent generation was replaced with Metaspace, which is allocated in native memory.
Understanding this structure helps us identify where issues may arise and how to address them effectively.
Common Causes of Java Heap Space Issues
-
Memory Leaks
- What They Are: A memory leak occurs when an application fails to release memory that is no longer needed, leading to increased usage over time until the application runs out of memory.
- Why It Happens: Objects that are no longer referenced might still be reachable due to lingering references, preventing them from being garbage collected.
Solution
Use profiling tools such as VisualVM or Eclipse Memory Analyzer to identify and eliminate memory leaks. For example:
// Example of a memory leak in a static collection public class MemoryLeakExample { private static List<Object> leakContainer = new ArrayList<>(); public static void createMemoryLeak() { leakContainer.add(new Object()); // This object will never be removed. } }
The above code snippet keeps adding objects to a static list, which remains in memory indefinitely. Once identified, you can use proper approaches to manage memory effectively.
-
Insufficient Heap Size
- What It Is: The default maximum heap size allocated might not be sufficient, especially for applications that require more resources, leading to memory errors.
- Why It Happens: The default value varies based on JVM and system specifications.
Solution
You can increase the heap size using the
-Xms
(initial heap size) and-Xmx
(maximum heap size) parameters when starting your Java application. Example:java -Xms512m -Xmx4g -jar yourapp.jar
This command sets the initial heap size to 512MB and the maximum size to 4GB. Adjust these values based on the application's requirements and testing.
-
Large Object Allocation
- What It Is: Allocating large objects can fill the heap quickly, especially if they are not collected promptly.
- Why It Happens: If your application handles large data sets or file streams inefficiently, it can quickly consume memory.
Solution
Use efficient data handling practices, such as streaming or batching processing when dealing with large datasets. Here’s an example of a more memory-efficient approach to handling a file:
// Improved file processing using streams try (Stream<String> lines = Files.lines(Paths.get("largefile.txt"))) { lines.forEach(System.out::println); } catch (IOException e) { e.printStackTrace(); }
In this example, we are using Java’s stream API to process a large file line by line, which helps to minimize memory usage compared to loading the entire file into memory.
-
Unbounded Caching
- What It Is: When storing results in memory (caching) without size limitations, you can fill up the heap space.
- Why It Happens: Without policies on cache size, old entries may never be evicted from memory.
Solution
Implement an eviction policy or maximum cache size using libraries like Guava or Caffeine. An example using Guava:
Cache<String, String> cache = CacheBuilder.newBuilder() .maximumSize(100) .expireAfterWrite(10, TimeUnit.MINUTES) .build(); cache.put("key", "value"); // Correct usage with size limit
This code snippet creates a cache that allows a maximum of 100 entries and evicts entries that haven’t been accessed in the last 10 minutes.
-
Excessive Multi-Threading
- What It Is: Creating too many threads that hold onto memory can lead to increased consumption of heap space.
- Why It Happens: Each thread has its stack and can increase memory demand significantly.
Solution
Use thread pools from the Executor framework rather than creating new threads directly. This approach helps control memory use more effectively.
ExecutorService executor = Executors.newFixedThreadPool(10); // Limit the number of threads executor.submit(() -> { // Task code here }); executor.shutdown();
In this example, we create a thread pool with a fixed number of threads, preventing excessive memory consumption through unbounded thread creation.
Monitoring and Maintenance
Monitoring Java heap space is a proactive measure that can save you from headaches down the line. There are various tools available for this, including:
- JVisualVM: A monitoring, troubleshooting, and profiling tool visualizing JMX beans.
- JConsole: An integral part of the JDK, available for monitoring heap space usage.
- Heap Dumps: Taking a heap dump when an
OutOfMemoryError
occurs allows you to analyze memory usage and pinpoint leaks.
Final Considerations
Tackling Java heap space issues requires a mix of detection, prevention, and solution implementations. By understanding the common causes, applying best practices in coding, and investing time in monitoring tools, you can maintain an efficient and effective Java application. As Java continues evolving, staying abreast of memory management tools and techniques will empower you as a developer to write robust applications.
For further reading on memory management in Java, consider visiting Oracle's Official Documentation on garbage collection tuning.
By proactively addressing potential pitfalls and gaining insight into your application's memory behavior, you'll reduce the frequency of OutOfMemoryError
occurrences and provide a seamless experience for your users.