Unraveling Complexities: Merging Maps in Java Concisely
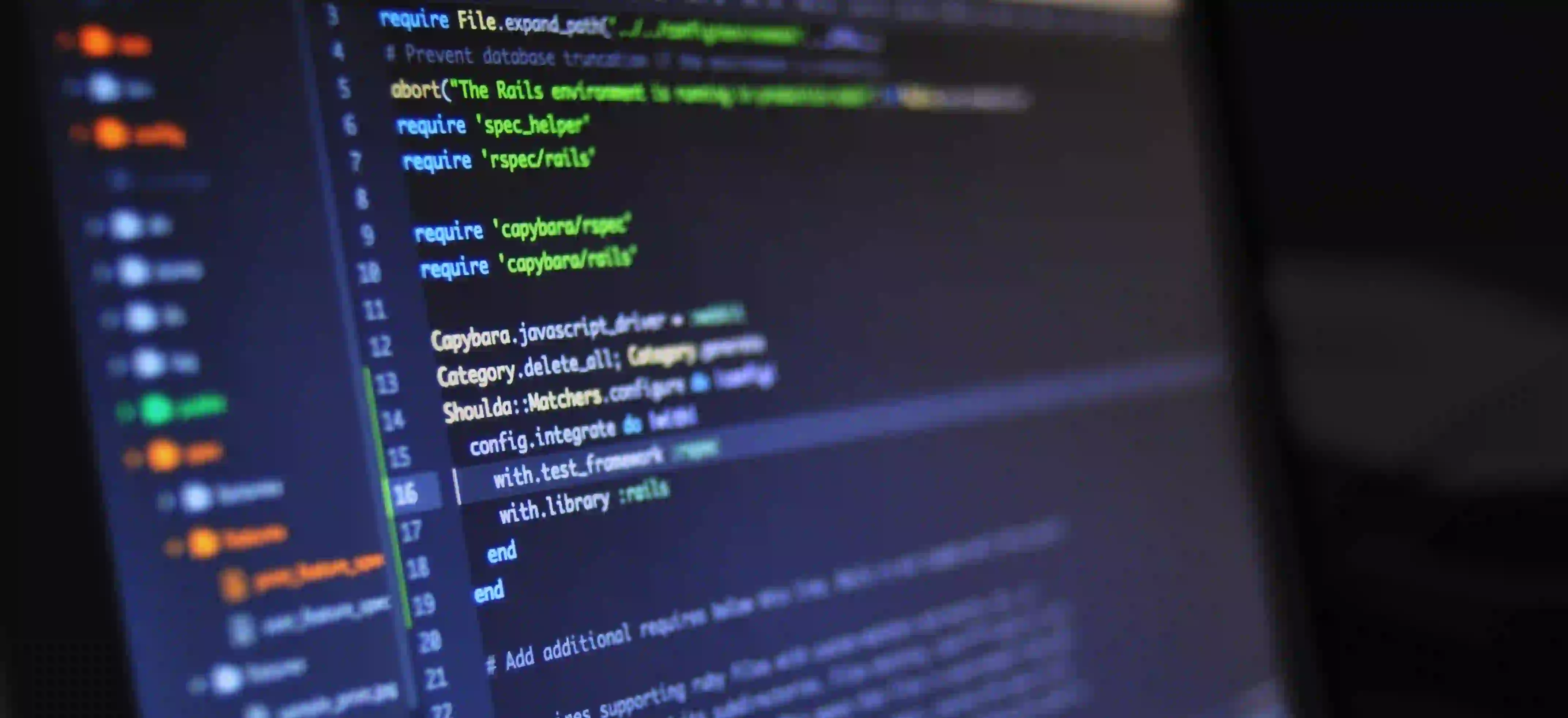
Unraveling Complexities: Merging Maps in Java Concisely
Java, as one of the most popular programming languages, has witnessed vast advancements over the years. One such advancement is the sophisticated handling of collections, particularly Maps. Merging Maps is a common operation that arises often, whether you’re summarizing data or consolidating configurations. In this blog post, we’ll explore how to merge maps concisely in Java, examine the various methods available, and provide you with practical examples to illuminate the subject.
Understanding Maps in Java
Before diving into the merging techniques, let's take a moment to understand what Maps are. A Map in Java is an object that maps keys to values. It cannot contain duplicate keys, and each key can map to at most one value.
Why Use Maps?
Maps are advantageous for several reasons:
- Fast Lookup: You can retrieve values quickly using keys.
- Flexible Structure: They can store any type of key or value.
- Customizability: You can implement interfaces to create complex map structures.
Now, let's get into the meat of the topic!
Basic Map Merge Functionality
With Java 8 and later versions, the Map
interface introduced a few convenient methods that simplify merging significantly. Two commonly used methods are putAll()
and merge()
.
Using putAll()
The putAll()
method copies all mappings from one map to another. If the keys already exist in the target map, their values are overwritten with the values from the source map.
Here’s a simple code snippet to illustrate:
import java.util.HashMap;
import java.util.Map;
public class MapMergeExample {
public static void main(String[] args) {
Map<String, Integer> map1 = new HashMap<>();
map1.put("One", 1);
map1.put("Two", 2);
Map<String, Integer> map2 = new HashMap<>();
map2.put("Two", 3);
map2.put("Three", 3);
map1.putAll(map2);
// Output: {One=1, Two=3, Three=3}
System.out.println(map1);
}
}
Commentary:
- In this example, we created two maps and merged
map2
intomap1
usingputAll()
. - Notice that the key "Two" has been overwritten with the value from
map2
, which is3
.
Why Use putAll()
?
- It's straightforward and effective for simply combining maps.
- It is ideal when you don't need to perform any operations on duplicate keys.
Merging with merge()
The merge()
method allows for more complex merging. It takes a key, a value, and a remapping function. If the key already exists, you can define how the values should be merged.
Here's an example:
import java.util.HashMap;
import java.util.Map;
public class MapMergeExample {
public static void main(String[] args) {
Map<String, Integer> map1 = new HashMap<>();
map1.put("One", 1);
map1.put("Two", 2);
Map<String, Integer> map2 = new HashMap<>();
map2.put("Two", 3);
map2.put("Three", 3);
map2.forEach((key, value) ->
map1.merge(key, value, Integer::sum)
);
// Output: {One=1, Two=5, Three=3}
System.out.println(map1);
}
}
Commentary:
- In this example, we're adding here the value of "Two" from both maps using
Integer::sum
. - The
merge()
method is powerful, allowing us to specify how to combine existing values.
Why Use merge()
?
- It provides greater flexibility.
- Enables operations on conflicting key values according to custom business logic.
Merging Maps of Different Types
Let’s say you have a map of strings to lists of integers and you want to merge them. You can apply similar logic using merge()
. This showcases the versatility of Java Maps effectively.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ComplexMapMerge {
public static void main(String[] args) {
Map<String, List<Integer>> map1 = new HashMap<>();
map1.put("A", new ArrayList<>(List.of(1, 2)));
map1.put("B", new ArrayList<>(List.of(3)));
Map<String, List<Integer>> map2 = new HashMap<>();
map2.put("A", new ArrayList<>(List.of(4, 5)));
map2.put("C", new ArrayList<>(List.of(6)));
map2.forEach((key, value) ->
map1.merge(key, value, (v1, v2) -> {
v1.addAll(v2);
return v1;
})
);
// Output: {A=[1, 2, 4, 5], B=[3], C=[6]}
System.out.println(map1);
}
}
Commentary:
- In this example, we merged lists of integers mapped to strings.
- The merging function combines existing lists correctly by adding new elements into the old list.
Considerations When Merging Maps
Performance Implications
Merging large maps can have performance implications. The time complexity is generally O(n), where n is the number of keys in the source map being merged.
Handling Null Values
Null values can cause your merging operations to behave unexpectedly. Always consider how you want to handle nulls to prevent errors.
Using Streams for Merging
With streams available in Java 8 or newer, you can conveniently merge maps in a more functional style:
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class StreamMergeExample {
public static void main(String[] args) {
Map<String, Integer> map1 = new HashMap<>();
map1.put("A", 1);
map1.put("B", 2);
Map<String, Integer> map2 = new HashMap<>();
map2.put("B", 3);
map2.put("C", 4);
Map<String, Integer> mergedMap = Stream.concat(map1.entrySet().stream(), map2.entrySet().stream())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
Integer::sum
));
// Output: {A=1, B=5, C=4}
System.out.println(mergedMap);
}
}
Commentary:
- This snippet illustrates how to merge two maps using Java Streams.
- The
merge
operation is expressed cleanly, adhering to functional programming paradigms.
The Last Word
Merging maps in Java, while seemingly straightforward, can be nuanced depending on the requirements and conditions of your data. Understanding methods such as putAll()
and merge()
, alongside the functional power of Streams, offers versatility to any Java developer.
In summary, whether you need the simplicity of putAll()
or the geared manipulation powered by merge()
, understanding how to navigate these operations can greatly enhance your development capabilities. Utilize these methods to manage collections effectively, clear unnecessary complexities, and render your code robust.
For further reading, you can refer to the Java Collections Framework and Java Streams for more advanced operations and concepts.
Happy Coding!