Maximizing Performance: Tackling Off-Heap Memory Issues in ChronicleMap
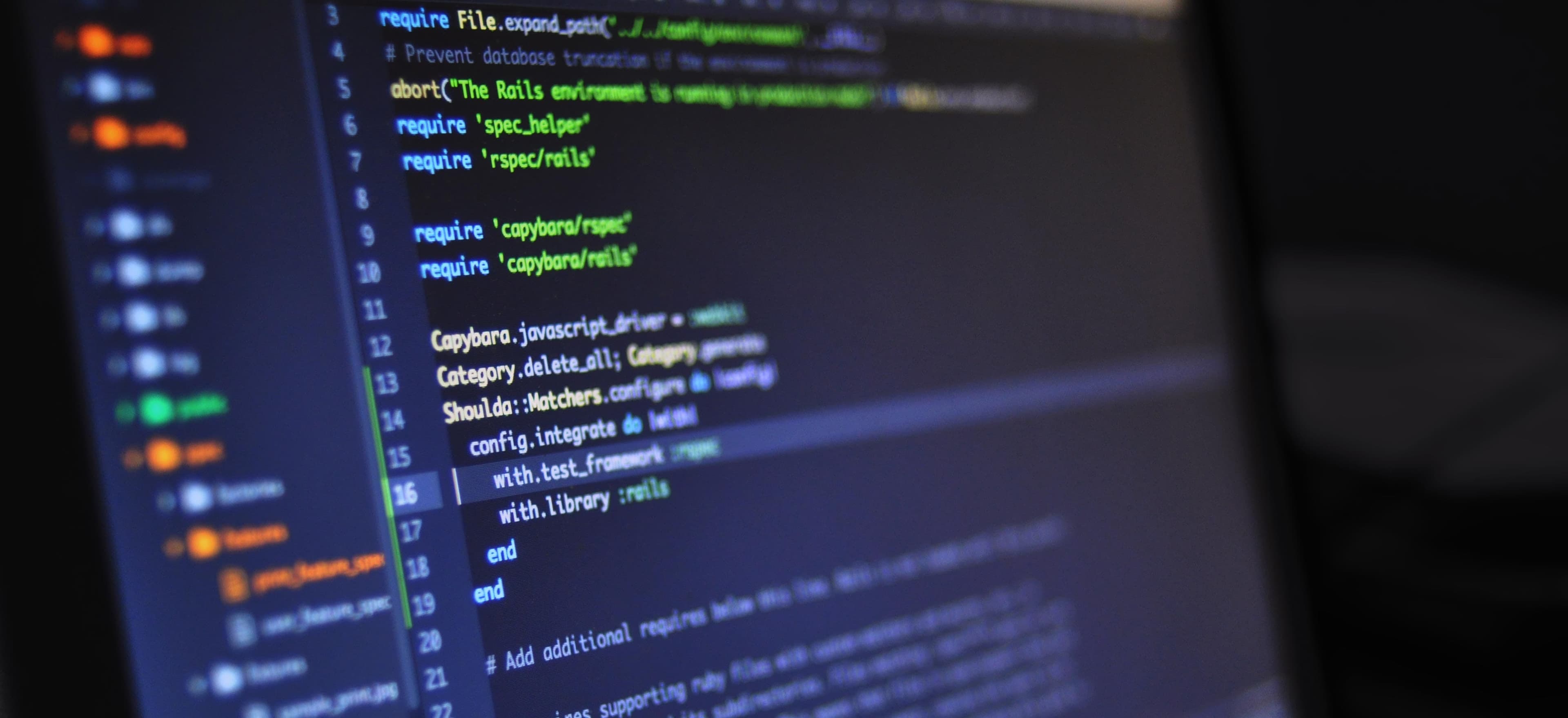
- Published on
Maximizing Performance: Tackling Off-Heap Memory Issues in ChronicleMap
In the realm of performance-critical applications, memory management often becomes the linchpin of overall program efficiency. Among various strategies for optimized memory usage, off-heap memory management stands out as an essential technique, especially in Java applications. One compelling solution in this space is ChronicleMap, a high-performance, off-heap key-value store. However, like any powerful tool, it comes with its own set of challenges, especially when it comes to memory management. In this post, we dive deep into maximizing performance by tackling off-heap memory issues in ChronicleMap.
Understanding Off-Heap Memory
Before we delve into ChronicleMap, let’s clarify what we mean by off-heap memory. In Java, object allocation occurs within the heap, which is garbage-collected memory. Off-heap memory, on the other hand, allows the storage of large objects outside the Java heap space. This technique reduces garbage collection pauses, lowers heap pressure, and provides higher performance for large datasets, which is critical for applications that require instant read and write operations.
An Overview of ChronicleMap
ChronicleMap is designed to utilize off-heap memory efficiently. It is also built for high concurrency and can handle large datasets without the performance bottlenecks like traditional on-heap stores can experience. With ChronicleMap, you can store data with minimal overhead, which is particularly suited for use cases involving caching, data streaming, and real-time analytics.
Key Features of ChronicleMap:
- Off-heap storage: Uses off-heap memory to minimize garbage collection impact.
- High throughput: Optimized for multiple threads, allowing concurrent read and write operations.
- Persistence: Can serialize data for durability, making it persistent across application restarts.
- Custom Serialization: Supports custom serialization strategies for fine-tuning performance.
Setting Up ChronicleMap
To get started, we first need to add ChronicleMap to our project. You can include it as a dependency in your Maven or Gradle build file.
Maven Dependency
<dependency>
<groupId>net.openhft</groupId>
<artifactId>chronicle-map</artifactId>
<version>latest_version_here</version>
</dependency>
Gradle Dependency
implementation 'net.openhft:chronicle-map:latest_version_here'
Replace latest_version_here
with the latest stable version available.
Creating a ChronicleMap Instance
Once the dependency is included, we can create a simple ChronicleMap instance:
import net.openhft.chronicle.map.ChronicleMap;
ChronicleMap<Integer, String> map = ChronicleMap
.of(Integer.class, String.class) // Specify the key and value types
.name("MyMap") // Set a name for identification
.entries(10000) // Define expected maximum entries
.create(); // Create the map instance
Explanation:
- Key and Value Types: We define the types of keys and values, which helps in reducing cast times during data retrieval.
- Name: Giving a name helps in identifying the map during debugging.
- Entries: Pre-defining the number of entries helps optimize memory allocation, preventing unnecessary overhead.
Handling Off-Heap Memory Issues
While ChronicleMap offers numerous advantages, there are a handful of off-heap memory issues that you might encounter:
1. Memory Leakage
Memory leaks occur when allocated off-heap memory isn’t correctly deallocated. ChronicleMap should be able to manage this automatically, but mid-execution changes can lead to leaks if not handled carefully.
Tip: Always monitor your application using tools like VisualVM to inspect memory usage.
2. Serialization Overhead
Off-heap memory requires serialization of data. If your serialization methods are not optimal, you could face performance bottlenecks.
Here’s an efficient serialization alternative using Java Serialization:
import java.io.Serializable;
class UserData implements Serializable {
private String name;
public UserData(String name) {
this.name = name;
}
// Getters and setters...
}
Why: Implementing Serializable
helps ChronicleMap to seamlessly manage data serialization and deserialization, making the process more efficient.
3. Reading from Off-Heap Memory
Accessing off-heap memory can be slower than accessing in-heap memory. Cache frequently accessed data in the heap to minimize access times.
String value = map.get(1); // Access the map
if (value == null) {
// Handle absence of value
}
4. JVM Options for Off-Heap Management
Adjust JVM settings to optimize off-heap memory usage.
Example JVM Options:
-XX:MaxDirectMemorySize=2g
-Djava.nio.MaxDirectMemorySize=2g
This ensures that your application can utilize sufficient off-heap memory for performance.
Use Cases for ChronicleMap
Caching with ChronicleMap
One prevalent use case of ChronicleMap is caching frequently accessed data. By maintaining a cache off-heap, we can achieve significant performance improvements.
// Simple caching mechanism
UserData user = map.get(userId);
if (user == null) {
user = fetchFromDatabase(userId);
map.put(userId, user);
}
// Utilize user data
System.out.println(user.getName());
Session Management
Another compelling use case could be managing user sessions in a web application. ChronicleMap can store session information in a fast, off-heap memory structure.
Real-time Analytics
ChronicleMap serves excellently in real-time analytics environments where large volumes of data need to be processed rapidly. Systems that analyze streams of data can utilize it to store and access real-time metrics without any delays.
The Last Word
In summary, ChronicleMap’s off-heap memory management offers a powerful approach to handling large datasets efficiently while providing high-performance capabilities. However, it’s essential to be vigilant about off-heap memory issues such as memory leaks, serialization overhead, and proper JVM tunings to maximize performance.
By understanding the intricacies of memory management and applying best practices, you can unlock the full potential of ChronicleMap for your Java applications. Always remember that performance tuning is an iterative process; regularly profile your application and adjust strategies accordingly.
For an in-depth look into ChronicleMap documentation, visit ChronicleMap Documentation.
By applying these strategies and considerations, you can leverage ChronicleMap to stay ahead in the performance-critical landscape of modern applications. Happy coding!
Checkout our other articles