Mastering Complex Expressions: Common Pitfalls in Interpreter Design
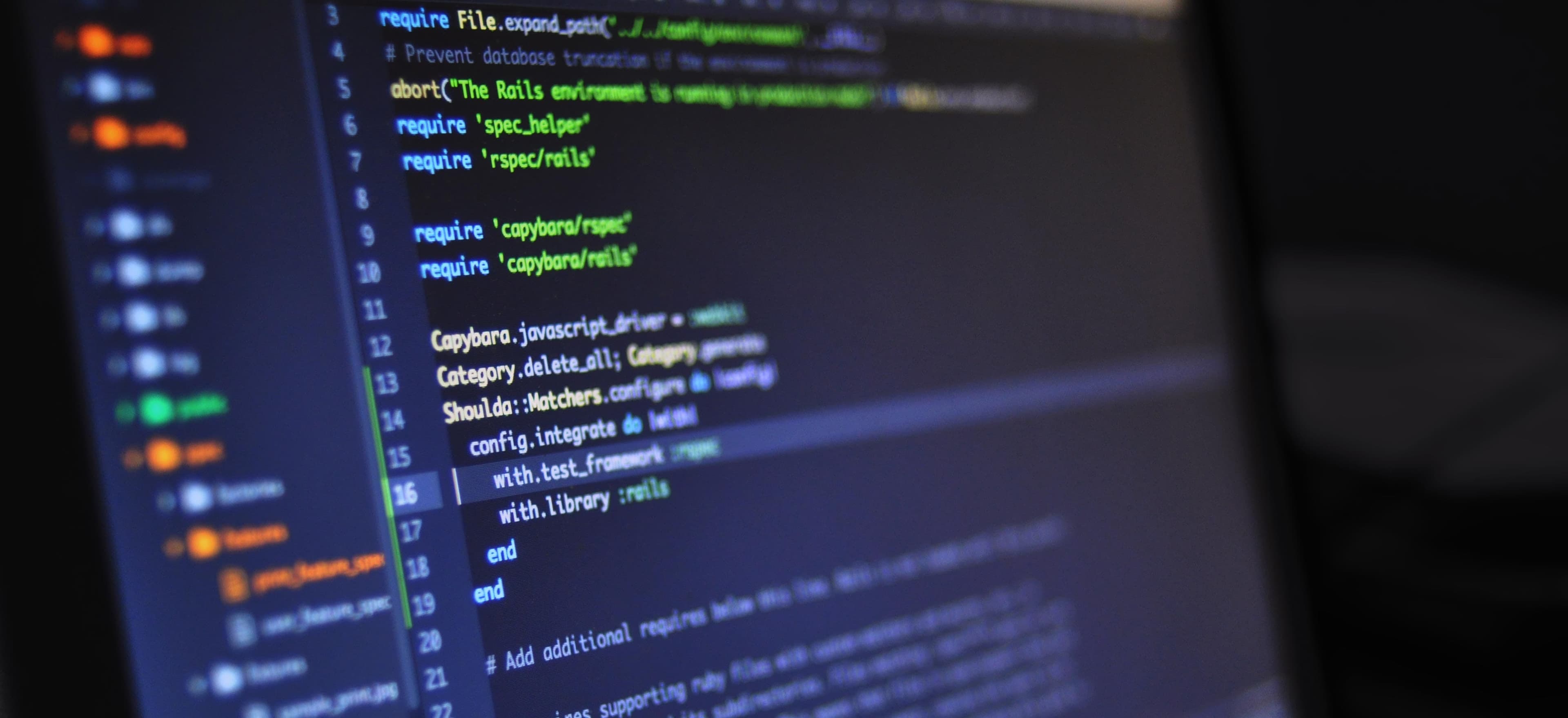
- Published on
Mastering Complex Expressions: Common Pitfalls in Interpreter Design
Interpreters serve as the backbone for executing programming languages, parsing high-level code into machine actions. Crafting an efficient interpreter is a nuanced task layered with intricacies, especially when handling complex expressions. This blog post aims to enlighten you on common pitfalls encountered in interpreter design, while also providing insight into solutions that can enhance your implementation.
What is an Interpreter?
An interpreter translates high-level programming languages into machine language, executing instructions one line at a time. Unlike compilers that translate the entire source code in one go, interpreters break code down and evaluate it dynamically. This offers benefits such as improved execution flexibility and ease of debugging.
Common Pitfalls in Interpreter Design
1. Ambiguity in Grammar
One of the first challenges an interpreter can face is ambiguity in the grammar of expressions. Ambiguity arises when the syntax is unclear, leading to multiple interpretations of a single expression.
Example
Consider the expression 3 + 4 * 5
. Should it evaluate as (3 + 4) * 5
or 3 + (4 * 5)
? The lack of clarity can frustrate both the interpreter and the end-user.
Solution
To avoid such pitfalls, establish a clear precedence and associativity for operators. Here’s a simplified version of implementing operator precedence in Java:
public class ExpressionEvaluator {
// Define operator precedence
private static final Map<String, Integer> precedence = new HashMap<>();
static {
precedence.put("+", 1);
precedence.put("-", 1);
precedence.put("*", 2);
precedence.put("/", 2);
}
public int evaluate(String expression) {
// Implementation redirecting to parse and evaluate
return parseExpression(expression);
}
// ... other necessary methods
}
Here, using a HashMap ensures that the operator precedence is stored and can be easily referenced. This allows the interpreter to prioritize operations correctly.
2. Lack of Error Handling
Robustness is key when handling user input. Poor error handling can lead the interpreter to unexpected behaviors or crashes.
Example
Imagine parsing the expression 5 + * 3
. A well-designed interpreter should catch this early rather than attempting to evaluate an erroneous expression.
Solution
Implement thorough error-checking mechanisms. Here is an example of handling error detection in a lexing phase:
public void lex(String source) {
for (char c : source.toCharArray()) {
if (!Character.isDigit(c) && "+-*/".indexOf(c) == -1) {
throw new SyntaxError("Unexpected character: " + c);
}
}
}
By proactively checking for valid characters, the interpreter can provide timely feedback, making it user-friendly.
3. Inefficient Evaluation of Nested Expressions
Complex nested expressions can lead to scalability issues. A naive approach to evaluating nested structures often results in redundant calculations or redundant data structures.
Solution
Utilize a stack-based approach, a common technique in both parsing and evaluating expressions. Here’s a basic implementation:
public int evaluateExpression(String expr) {
Stack<Integer> values = new Stack<>();
Stack<Character> ops = new Stack<>();
for (String token : expr.split(" ")) {
if (isNumeric(token)) {
values.push(Integer.parseInt(token));
} else {
while (!ops.empty() && precedence.get(token) <= precedence.get(ops.peek().toString())) {
values.push(applyOp(ops.pop(), values.pop(), values.pop()));
}
ops.push(token.charAt(0));
}
}
while (!ops.empty()) {
values.push(applyOp(ops.pop(), values.pop(), values.pop()));
}
return values.pop();
}
private boolean isNumeric(String str) {
return str.matches("\\d+");
}
private int applyOp(char op, int b, int a) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
}
return 0;
}
This implementation effectively breaks down the evaluation process and uses stacks for both values and operators, resulting in optimized performance and avoidable redundancy.
4. State Management
Managing state in an interpreter is crucial, especially when dealing with variable assignments and scope.
Example
A common error is failing to recognize different scopes for variables, leading to shadowing or unintentional reuse of variable names in different contexts.
Solution
Implement a symbol table to manage variable states across different scopes.
public class SymbolTable {
private Map<String, Integer> variables = new HashMap<>();
public void set(String name, int value) {
variables.put(name, value);
}
public int get(String name) {
if (!variables.containsKey(name)) {
throw new RuntimeException("Variable not defined: " + name);
}
return variables.get(name);
}
}
By utilizing a symbol table, you can maintain clear visibility over variable states, enhancing both performance and reliability.
To Wrap Things Up
Designing an interpreter capable of effectively managing complex expressions presents a myriad of challenges. By being mindful of grammar ambiguity, ensuring robust error handling, efficiently evaluating expressions, and maintaining careful state management, developers can craft interpreters that are not just functional but also resilient against common pitfalls.
For more detailed guidance on interpreter design, you may find the following resources helpful:
By adhering to these principles and leveraging a proper understanding of core design challenges, you position yourself better in mastering interpreter design, ensuring that your code can tackle the wild complexity inherent in programming languages.
Feel free to reach out with comments or questions below. Happy coding!
Checkout our other articles