Integrating JavaFX 2 with Spring: Common Pitfalls Explained
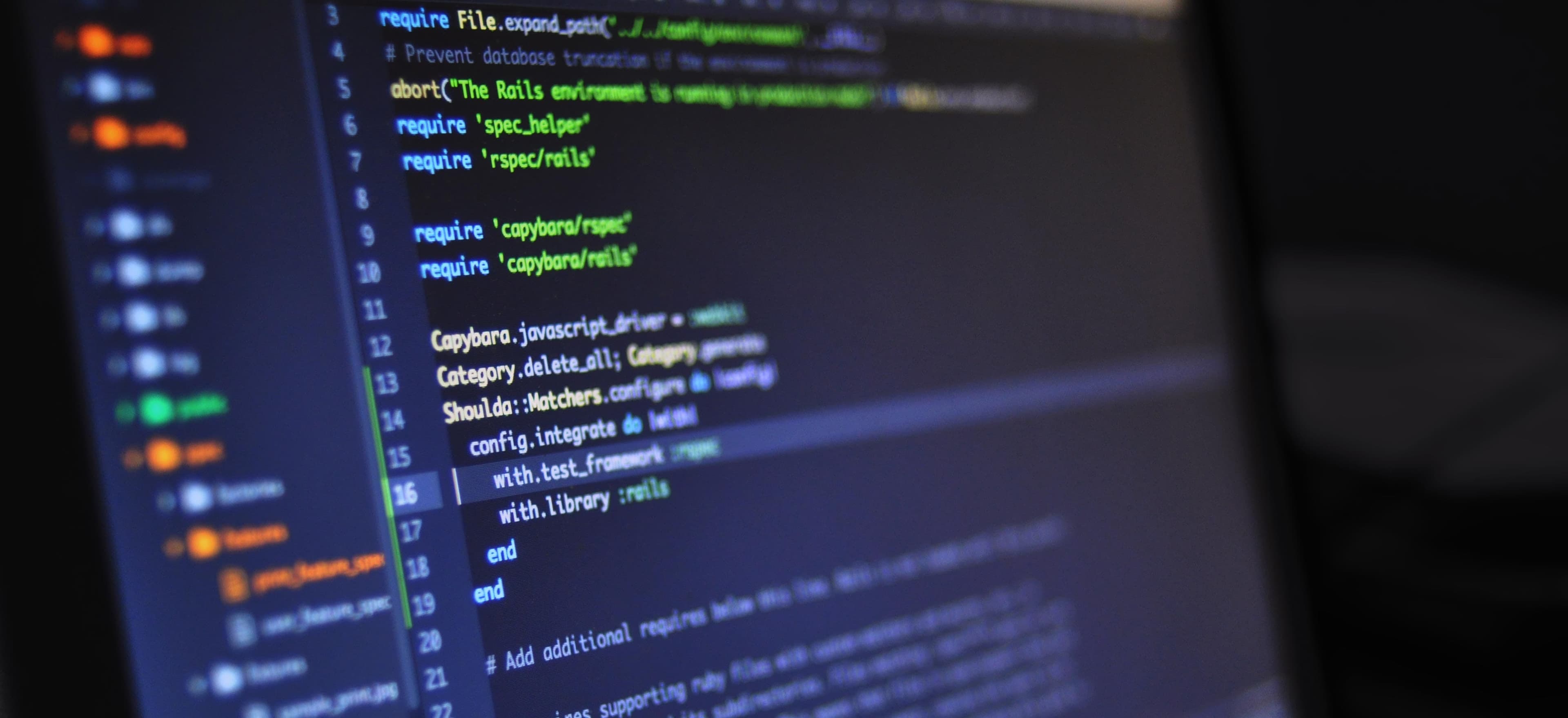
- Published on
Integrating JavaFX 2 with Spring: Common Pitfalls Explained
Integrating JavaFX with the Spring framework can lead to powerful desktop applications that leverage Spring's capabilities. However, there are common pitfalls developers encounter during this integration. In this blog post, we will walk through these challenges and their solutions.
Why Use JavaFX with Spring?
JavaFX is a powerful framework for building rich client applications. When combined with Spring, it allows developers to create applications that are easy to maintain, extend, and test. Spring handles dependency injection and provides a plethora of features, such as transaction management, security, and more.
Key Pitfalls in Integrating JavaFX with Spring
1. Misunderstanding Thread Management
JavaFX works on a single thread known as the JavaFX Application Thread, while Spring may run processes on different threads. Here’s why understanding this is essential: UI updates should always occur on the JavaFX Application Thread, and failure to do so can lead to runtime exceptions or the application becoming unresponsive.
Example: Updating UI from a Background Thread
import javafx.application.Platform;
public void updateUIFromThread() {
// Background task
new Thread(() -> {
// Perform some long-running task
String result = longRunningTask();
// Update UI on the JavaFX Application Thread
Platform.runLater(() -> {
label.setText(result);
});
}).start();
}
In this code, Platform.runLater ensures that UI updates can safely occur on the JavaFX Application Thread, preventing any concurrency issues. For more information on JavaFX threading, refer to the JavaFX concurrency documentation.
2. Failing to Manage Application Context Lifecycle
Spring manages the lifecycle of its beans, while JavaFX does not. If your application context is not correctly managed, it can lead to resource leaks or non-functional components.
Solution: Use ApplicationContextAware Interface
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import javafx.application.Application;
public class MyApplication extends Application implements ApplicationContextAware {
private static ApplicationContext context;
@Override
public void setApplicationContext(ApplicationContext applicationContext) {
context = applicationContext;
}
public static void main(String[] args) {
Application.launch(MyApplication.class, args);
}
}
In this example, we implement ApplicationContextAware to hold a reference to the Spring application context. This reference can then be used to retrieve Spring-managed beans throughout the application.
3. Ignoring Property Injection
Developers often forgo using JavaFX properties, leading to issues in property binding, which is a key feature of JavaFX. By utilizing properties, you gain access to reactive programming benefits.
Example: Using SimpleStringProperty
import javafx.beans.property.SimpleStringProperty;
public class Person {
private SimpleStringProperty name = new SimpleStringProperty(this, "name", "");
public String getName() {
return name.get();
}
public void setName(String value) {
name.set(value);
}
}
By using SimpleStringProperty
, your UI can automatically reflect changes made to the underlying data model, leading to a more dynamic user experience.
4. Not Creating a Spring-Managed JavaFX Application
Difficulties can arise if the JavaFX application is not Spring-aware. JavaFX initialization can often proceed without Spring managing it, leading to improper instantiation of beans and other components.
Example: Creating a Spring-Managed JavaFX Application
import javafx.application.Application;
import javafx.stage.Stage;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApp extends Application {
public static void main(String[] args) {
SpringApplication.run(MainApp.class, args);
launch(args);
}
@Override
public void start(Stage stage) {
// Inject your controllers or services here
// Use Spring's context if needed
}
}
Here, SpringApplication.run initializes the Spring context before launching the JavaFX application, allowing you to seamlessly use Spring's bean management features.
5. Not Utilizing FXML Controllers
Another common pitfall is not effectively using FXML controllers with Spring. This can limit the modularity and testability of your JavaFX application.
Example: Using Spring for FXML Controllers
import javafx.fxml.FXML;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyController {
@FXML
private Label label;
@Autowired
private MyService myService;
public void initialize() {
label.setText(myService.getGreeting());
}
}
In this code, we use the @Component
annotation to let Spring manage the controller. The @Autowired
annotation injects MyService
, enabling a clean separation of concerns.
6. Inefficient Resource Loading
JavaFX applications often require resources like images and stylesheets, which should be loaded efficiently. Failing to do so can lead to slow startup times or unresponsive UI.
Example: Efficient Resource Loading
import javafx.scene.image.Image;
public class ResourceLoader {
public static Image getImage(String path) {
return new Image(ResourceLoader.class.getResourceAsStream(path));
}
}
By centralizing resource loading, you can optimize performance further while keeping your codebase maintainable. Always ensure that resources are referenced correctly relative to your package structure.
Closing the Chapter
Integrating JavaFX with Spring opens the door to creating robust, rich client applications. However, it's vital to approach this integration mindfully, being aware of common pitfalls such as thread management, lifecycle issues, and efficient resource handling.
Key Takeaways:
- Always perform UI updates on the JavaFX Application Thread.
- Manage the Spring application context correctly to avoid lifecycle issues.
- Utilize properties in your models for efficient binding.
- Make your JavaFX application Spring-aware for better integration.
- Use FXML controllers in conjunction with Spring for cleaner architecture.
- Efficiently load resources to boost performance.
For more detailed information, explore the official JavaFX Documentation. Happy coding!