Common Pitfalls When Using InfluxDB Mapper in Java
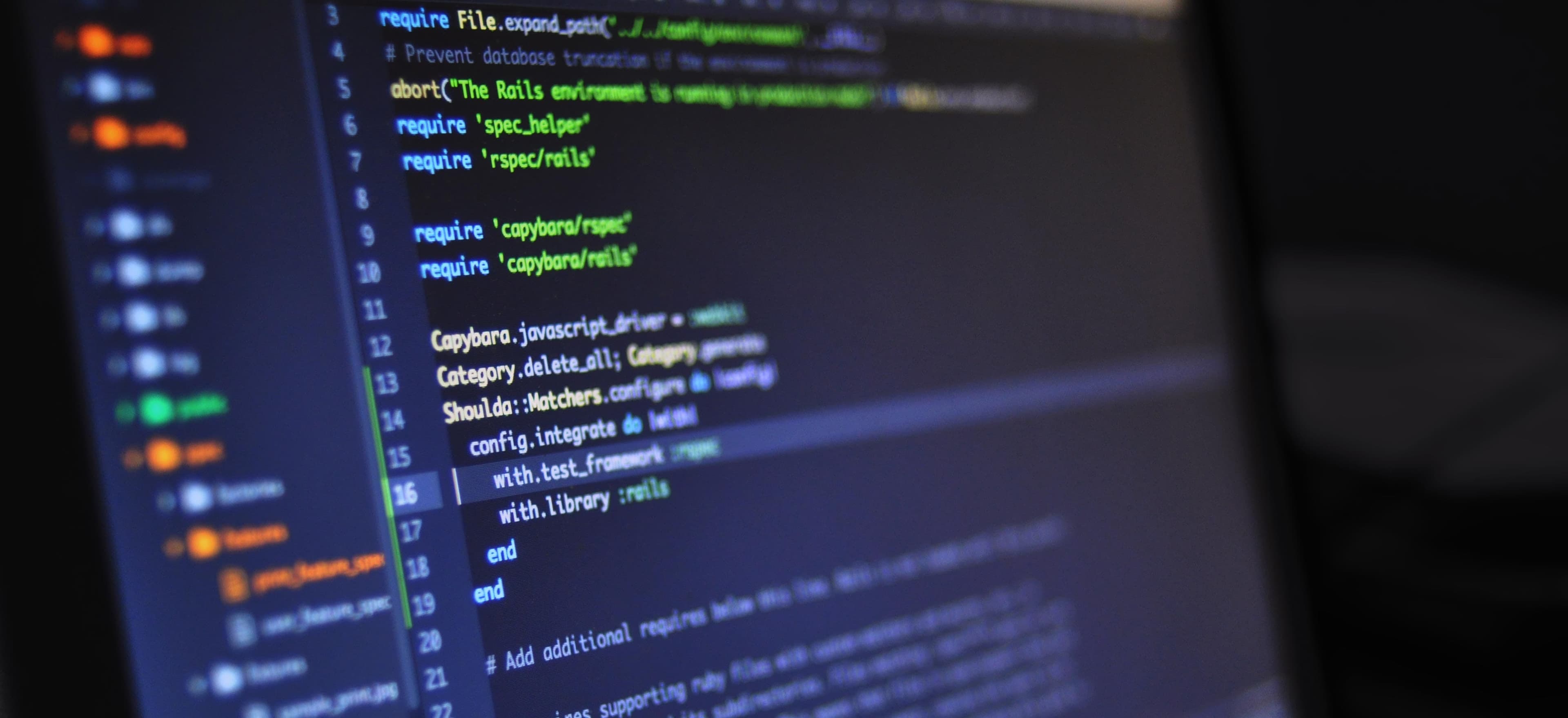
- Published on
Common Pitfalls When Using InfluxDB Mapper in Java
InfluxDB is a powerful time-series database designed for high availability and high performance. When using it in Java, the InfluxDB Mapper offers a more object-oriented approach, allowing developers to work more naturally with their data. However, this convenience comes with its own set of challenges. Below, we’ll explore common pitfalls when utilizing the InfluxDB Mapper in Java and how to avoid them.
Understanding InfluxDB Mapper
The InfluxDB Mapper bridges the gap between the Java objects and the time-series data in InfluxDB. It allows developers to map their Java classes to database entries, simplifying the interaction with the database.
However, pitfalls often arise from misunderstandings of how the Mapper functions. Let’s dive into some of the most common issues you might face.
Pitfall 1: Schema Mismatch
One of the biggest mistakes occurs when the Java object does not align with the InfluxDB schema.
Example of Schema Mismatch
Suppose you have a Java class like this:
import org.influxdb.annotation.Column;
import org.influxdb.annotation.Measurement;
@Measurement(name = "weather")
public class Weather {
@Column(name = "time")
private Long timestamp;
@Column(name = "temperature")
private Double temp;
// getters and setters
}
If you are pushing data to a measurement that does not exist, you will likely get errors. Ensure that the measurement name and column names in your annotations match exactly with your InfluxDB schema.
Why This Matters
Mismatches can lead to runtime exceptions. Always verify field names and types in your Java class against the InfluxDB measurement schema. Using tools like InfluxDB Studio can help you visualize your database schema.
Pitfall 2: Time Format Issues
InfluxDB relies heavily on timestamps. If timestamps are not correctly managed, it can result in data inconsistency.
Managing Timestamps
When you define the timestamp in your Java class, use UNIX timestamps:
@Column(name = "time")
private Long timestamp;
// Inserting data
this.timestamp = System.currentTimeMillis(); // milliseconds since epoch
Why This Matters
Using the correct time format helps InfluxDB efficiently organize data in chronological order. If your timestamp is in an incorrect format, your queries will suffer, leading to inaccurate results.
Pitfall 3: Overloading Measurements
Developers sometimes try to store too much information in a single measurement. While InfluxDB can handle a significant amount of data, overloading measurements can lead to performance issues.
Optimizing Measurements
Instead of combining unrelated metrics into one measurement, separate them into carefully defined measurements. For example, consider separating temperature and humidity into distinct measurements.
@Measurement(name = "temperature")
public class Temperature {
@Column(name = "time")
private Long timestamp;
@Column(name = "value")
private Double value;
}
@Measurement(name = "humidity")
public class Humidity {
@Column(name = "time")
private Long timestamp;
@Column(name = "value")
private Double value;
}
Why This Matters
Separation ensures a more organized database schema, leading to more efficient queries. Each measurement will have defined tags and fields that can be indexed appropriately.
Pitfall 4: Ignoring Tags and Fields
Tags and fields play a crucial role in how InfluxDB handles data. Developers sometimes ignore this distinction.
Defining Tags and Fields
Tags are indexed, which means they are stored in a more efficient way for queries. Fields are not indexed and are more suited for actual measurement values.
@Measurement(name = "sensor_data")
public class SensorData {
@Column(name = "time")
private Long timestamp;
@Column(name = "temperature")
private Double temperature;
@Column(name = "unit")
private String unit; // example of a tag
}
Why This Matters
Using tags for frequently queried attributes (like unit types) can improve query performance. Do not treat all attributes the same; leverage the unique characteristics of fields and tags.
Pitfall 5: Not Configuring InfluxDB Connection Properly
A poor connection setup can lead to unexpected errors.
Configuring Connection
Ensure you initialize the InfluxDB client properly with appropriate authentication and configurations.
InfluxDB influxDB = InfluxDBFactory.connect("http://localhost:8086", "username", "password");
influxDB.setDatabase("your_db_name");
Why This Matters
A faulty connection can hinder data writes and reads. Properly managing your connection settings can save you a lot of debugging time.
Pitfall 6: Ignoring Error Handling
InfluxDB interactions can fail for numerous reasons, from connection issues to schema mismatches.
Implementing Error Handling
Always wrap database interactions in appropriate try-catch blocks:
try {
influxDB.write(Point.measurement("sensor_data")
.time(System.currentTimeMillis(), TimeUnit.MILLISECONDS)
.addField("temperature", temperature)
.addField("humidity", humidity)
.build());
} catch (InfluxDBException e) {
// Handle error appropriately
e.printStackTrace();
}
Why This Matters
Ignoring error handling can cause silent failures, leading to corrupted or loss of data. A robust error handling strategy improves the reliability of your application.
Pitfall 7: Query Performance Issues
InfluxDB is optimized for performance, but inefficient queries can slow it down.
Optimizing Queries
When querying data, always limit the range and use timestamps effectively:
Query query = new Query("SELECT * FROM sensor_data WHERE time >= now() - 1h", "your_db_name");
QueryResult result = influxDB.query(query);
Why This Matters
Efficient queries return results more quickly, improving user experience and reducing server load. Learn more about InfluxDB query optimization for best practices.
The Bottom Line
Using the InfluxDB Mapper in Java can significantly streamline your interactions with time-series data. However, understanding common pitfalls and implementing best practices can save developers from unnecessary headaches. Maintain a clear schema, handle timestamps correctly, utilize tags and fields judiciously, and ensure your connection and queries are robust.
By being aware of these pitfalls, you can take full advantage of what InfluxDB has to offer without compromising your application's performance or integrity. For a deeper dive into InfluxDB features or to see sample applications, check out InfluxData's official documentation.
With these insights and practices, you're now better equipped to use InfluxDB Mapper effectively in your Java projects. Happy coding!