Overcoming Common TestContainer Issues in Spring Boot
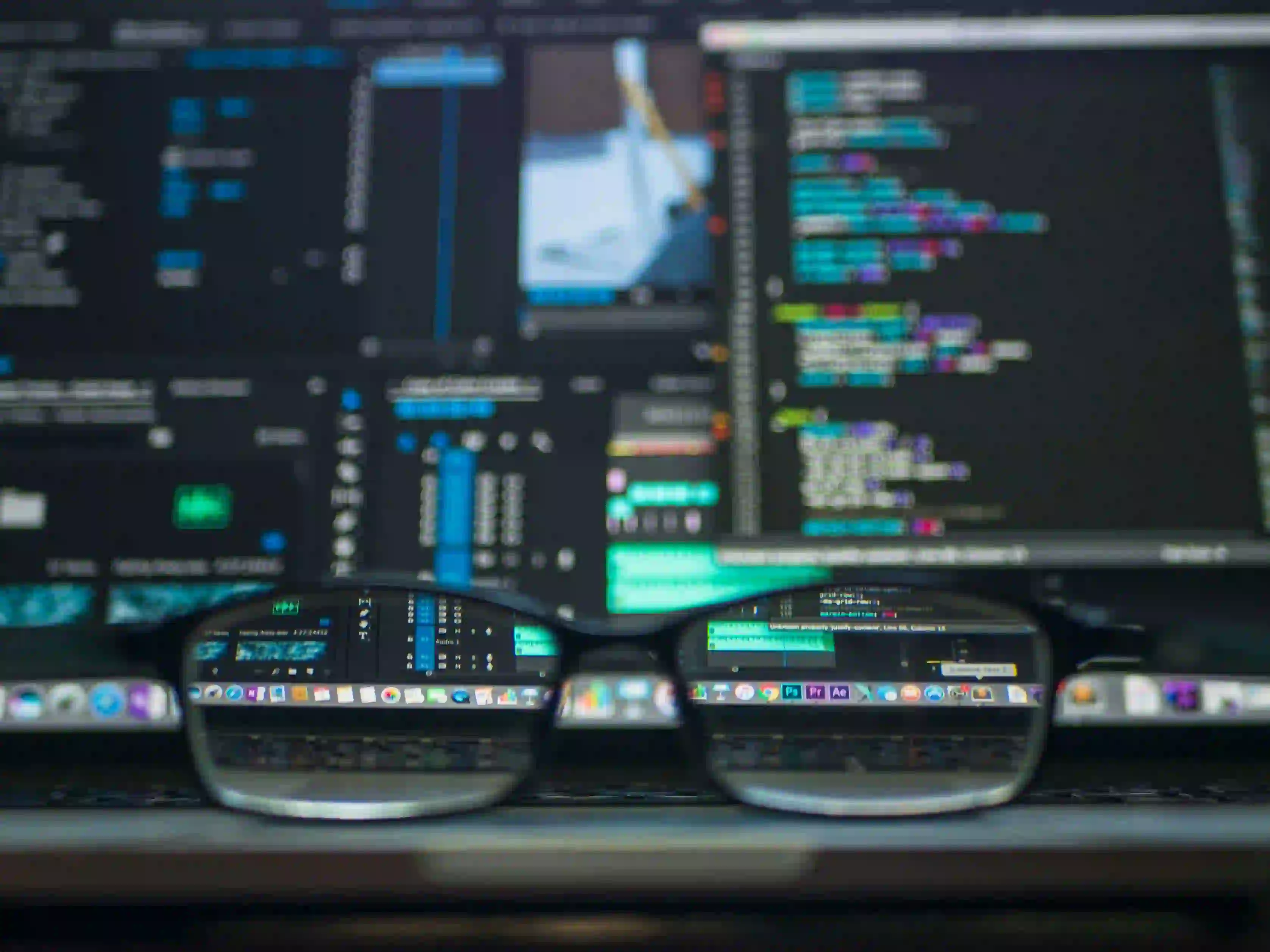
Overcoming Common TestContainer Issues in Spring Boot
Testing is an integral part of software development, ensuring that applications function correctly and meet user requirements. Spring Boot has transformed Java development with its simplicity, reliability, and support for various testing frameworks. When testing with containerized environments, TestContainers—a Java library that supports JUnit tests based on Docker containers—can be a significant asset. However, developers often encounter issues when setting up and using TestContainers within a Spring Boot application. This post discusses these common issues and how to resolve them effectively.
What is TestContainers?
TestContainers provides lightweight, disposable instances of common databases, Selenium web browsers, or anything that can run in a Docker container. It allows us to create integration tests that closely resemble a production environment, leading to more reliable test results.
Here's an example of how you might leverage TestContainers with a PostgreSQL database in a Spring Boot application:
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
import org.testcontainers.containers.PostgreSQLContainer;
@SpringBootTest
public class ApplicationTests {
// Defining the PostgreSQL container
static PostgreSQLContainer<?> postgresContainer = new PostgreSQLContainer<>("postgres:latest")
.withDatabaseName("testdb")
.withUsername("test")
.withPassword("test");
static {
postgresContainer.start();
}
@Test
void testDatabaseConnection() {
// Your test logic here
assertTrue(postgresContainer.isRunning());
}
}
In this example, a new PostgreSQL container is created, started, and tested against. This approach allows developers to focus on writing tests rather than the complexities of maintaining a local database environment.
Common Issues Faced with TestContainers
While TestContainers is a powerful tool, users often encounter several common challenges. Let's explore these issues and their solutions to make your integration testing smoother.
1. Docker Daemon Not Running
Issue: The most common issue arises when the Docker daemon is not running. Without Docker, TestContainers cannot create or manage containers.
Solution: Ensure that Docker is installed and running on your machine. You can verify this by running the docker ps
command in your terminal. If it returns an error, start the Docker service.
2. Insufficient Resources for Docker
Issue: Another common problem occurs when Docker does not have enough allocated resources (CPU, Memory) to run the required containers.
Solution: Adjust the resource settings in the Docker desktop application or the Docker daemon configuration. For local development, it's recommended to allocate sufficient RAM and CPU, especially when working with multiple containers.
3. Dependency Conflicts
Issue: Conflicts between TestContainers and other libraries can arise, often leading to NoClassDefFoundError
or similar issues.
Solution: Always ensure your dependencies are compatible. It’s advisable to check the TestContainers documentation for the required versions of libraries like JUnit or Spring Boot. Use the following Maven dependency as an example:
<dependency>
<groupId>org.testcontainers</groupId>
<artifactId>testcontainers</artifactId>
<version>1.17.1</version><!-- Update to the latest version -->
<scope>test</scope>
</dependency>
4. Using TestContainers with Maven Profiles
Issue: Developers may encounter issues when trying to run tests with different setups or environments managed by Maven profiles.
Solution: Use the @ActiveProfiles
annotation to specify which profiles to activate during testing. Here's an example:
import org.springframework.test.context.ActiveProfiles;
@ActiveProfiles("test")
@SpringBootTest
public class ApplicationTests {
// Your tests here
}
This helps manage your application properties, ensuring that connection strings or test configurations are loaded correctly.
5. Configuring Container Port Mappings
Issue: Misconfigured port mappings can lead to connection issues, particularly if you're attempting to connect to a containerized database.
Solution: Use TestContainers' getMappedPort()
method to obtain the port that should be used for the connection. Here’s a snippet that demonstrates this:
int mappedPort = postgresContainer.getMappedPort(PostgreSQLContainer.POSTGRESQL_PORT);
String jdbcUrl = "jdbc:postgresql://localhost:" + mappedPort + "/testdb";
System.out.println(jdbcUrl); // Use this for your database connection in tests
6. Cleanup of Containers After Tests
Issue: Sometimes, testers forget to clean up the used containers, leading to resource leaks and slow performance over time.
Solution: TestContainers will automatically handle the cleanup of containers after the tests are complete. However, if you're managing containers manually or overriding this behavior, make sure to call stop()
or close()
methods in your test cleanup methods.
7. Networking Issues
Issue: When running tests that depend on external services (like REST APIs), networking issues can occur, especially on CI/CD environments.
Solution: Ensure that you are using hostnames that the containers can resolve. You can access localhost services from the container using the special DNS name host.docker.internal
. Here’s how you can access an API in a container:
String apiUrl = "http://host.docker.internal:8080/api/resource";
8. Logging in TestContainers
Issue: Developers often find it challenging to debug container logs during tests.
Solution: TestContainers provides an easy way to access logs using the getLogs()
method. Here's an example:
String containerLogs = postgresContainer.getLogs();
System.out.println(containerLogs); // Print logs for debugging
Best Practices for Using TestContainers
Here are some best practices to keep in mind when using TestContainers in your Spring Boot applications:
-
Use a Base Image: Whenever possible, use a specific version of an image instead of
latest
to avoid unexpected issues when the image updates. -
Reuse Containers: Use
@DynamicPropertySource
to customize properties based on the container state, reducing the overhead of starting containers frequently. -
Keep Tests Isolated: Each test should run independently from others. This avoids the risk of tests interfering with each other.
-
Parallel Testing: Run tests in parallel when feasible, but ensure containers can handle it and consider the implications on resource consumption.
-
Version Control: Keep track of TestContainers and Docker versions to ensure compatibility. Update regularly to take advantage of new features and bug fixes.
A Final Look
TestContainers is a powerful library for integration testing in Spring Boot applications, but common issues can derail your progress. This guide has provided comprehensive insights into dealing with some of the most frequent challenges developers face, along with best practices for leveraging TestContainers effectively.
By harnessing the power of TestContainers, your testing process can become smoother and more reliable, giving you the confidence needed to deploy robust applications in a production environment. For more resources, tutorial videos, and examples, do explore the TestContainers documentation or check out additional Spring Boot resources.
By proactively addressing potential issues, you can ensure a seamless integration testing experience in your Spring Boot applications.
Feel free to comment below if you have encountered any issues with TestContainers and how you managed to resolve them, or share your tips and tricks for seamless integration testing!