Common Pitfalls When Using Java for REST Clients
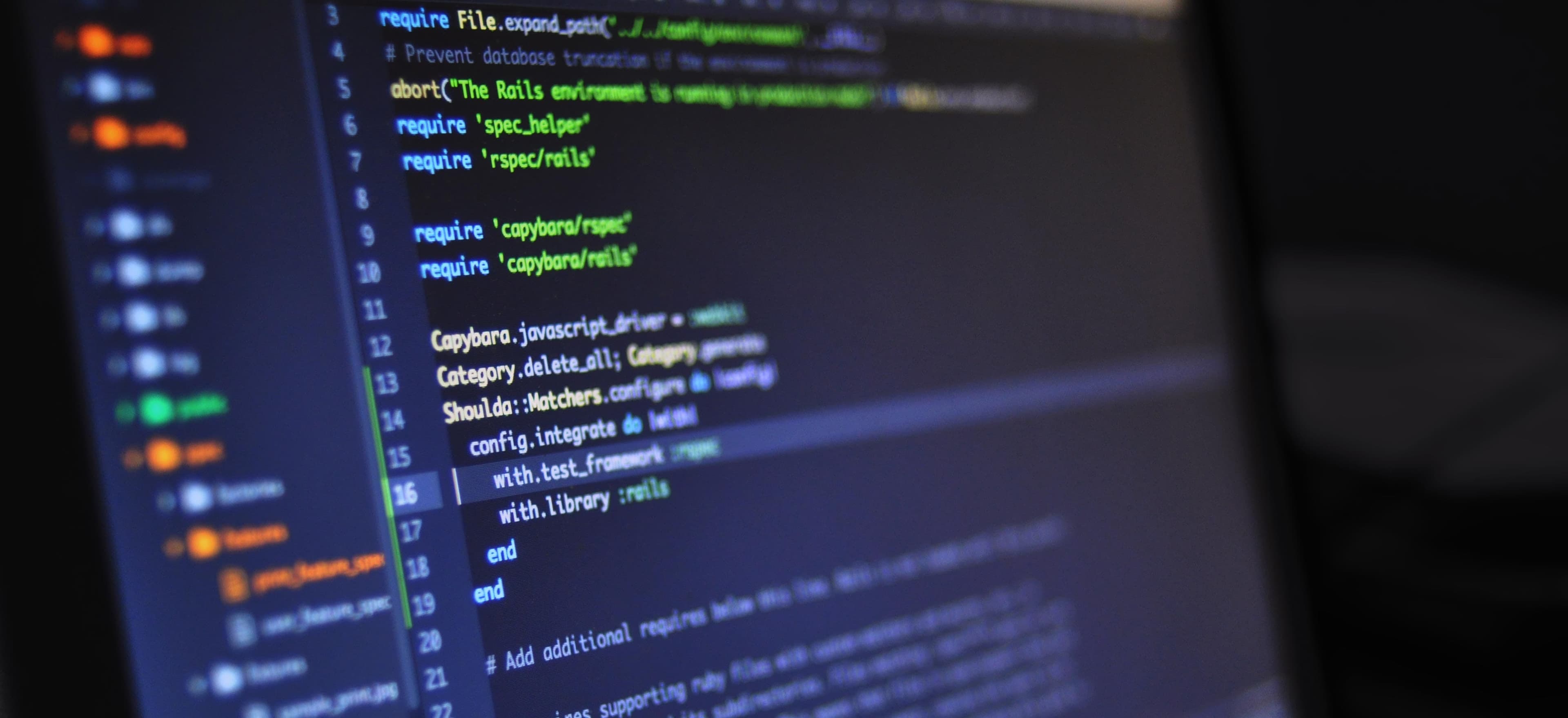
- Published on
Common Pitfalls When Using Java for REST Clients
Java has become one of the most widely used programming languages for creating RESTful web services due to its robust ecosystem and rich library support. However, when it comes to using Java for REST clients, developers often trip over common pitfalls that can lead to performance issues, security vulnerabilities, and buggy applications.
In this blog post, we will explore some of the most prevalent pitfalls in building REST clients using Java, while providing practical advice and code examples to help you avoid these issues. Whether you're a seasoned Java developer or a newcomer, this article aims to enhance your skills and make your REST client development smoother.
1. Poor Exception Handling
The Problem
REST APIs can fail for a multitude of reasons, including network issues, server errors, or unexpected API responses. Developers often neglect to implement adequate exception handling, resulting in clients that crash without providing clear feedback.
The Solution
Utilizing Java's exception handling mechanism elegantly allows for better management of potential issues. Here's an example of how to handle exceptions in a REST client gracefully.
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class RestClient {
public String callApi(String apiUrl) {
HttpURLConnection connection = null;
try {
URL url = new URL(apiUrl);
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// Process the response here
return "Success!";
} else {
// Handle non-200 responses gracefully
throw new RuntimeException("Failed: HTTP error code : " + responseCode);
}
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException("Unable to connect to the REST API.");
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}
Commentary
In this snippet, we use try-catch blocks to handle IOException
. We also manage the HTTP response code properly. This not only prevents our application from crashing but also gives developers a more descriptive error message to work with.
2. Ignoring HTTP Status Codes
The Problem
Sometimes developers focus too much on the data being returned and completely ignore the HTTP status codes. This oversight can lead to inconsistencies in how requests are executed and lead to attempting to handle bad data.
The Solution
Always check the HTTP status code before proceeding to process the response. For example:
if (responseCode == HttpURLConnection.HTTP_OK) {
// Process the response
} else if (responseCode == HttpURLConnection.HTTP_NOT_FOUND) {
throw new RuntimeException("Resource not found.");
} else {
throw new RuntimeException("Unexpected response: " + responseCode);
}
Commentary
This careful handling of HTTP status codes will provide a more robust communication mechanism with the REST API. By understanding the meaning of each status code, you can tailor your logic in the client application accordingly.
3. Not Using Asynchronous Calls
The Problem
Blocking operations in network calls can slow down your application significantly. REST APIs can sometimes respond slowly, and blocking waiting for a response can lead to poor user experience.
The Solution
Using asynchronous calls can help improve performance. Java offers several options for asynchronous programming, including CompletableFuture
and the ExecutorService
. Here's an example using CompletableFuture
:
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.concurrent.CompletableFuture;
public class AsyncRestClient {
public CompletableFuture<String> callApiAsync(String apiUrl) {
return CompletableFuture.supplyAsync(() -> {
try {
URL url = new URL(apiUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
// Handle the response
return responseCode == HttpURLConnection.HTTP_OK ? "Success!" : "Failed";
} catch (Exception e) {
throw new RuntimeException("Unable to connect to the REST API.");
}
});
}
}
Commentary
This method allows other parts of your application to continue running while waiting for the API response. It’s a great way to ensure your application remains responsive.
4. Hardcoding URLs
The Problem
Hardcoding URLs in your code can lead to a range of problems, including difficulty in managing environment configurations, versioning, and security risks.
The Solution
Utilize configuration files or environment variables. For instance, use a properties file to manage your API endpoints:
# config.properties
api.url=https://api.example.com
You can then load this configuration:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class ConfigLoader {
private Properties properties = new Properties();
public ConfigLoader() throws IOException {
try (FileInputStream input = new FileInputStream("config.properties")) {
properties.load(input);
}
}
public String getApiUrl() {
return properties.getProperty("api.url");
}
}
Commentary
By externalizing URLs, you can easily update them without changing the codebase. This is a common best practice that also improves code readability and maintainability.
5. Not Configuring Timeouts
The Problem
Network calls can hang indefinitely, especially if the remote service is slow or unresponsive. Without proper timeouts, such situations lead to poor application performance.
The Solution
Configure connection and read timeouts on your HTTP connection. Here’s how you can do it:
connection.setConnectTimeout(5000); // Set connection timeout to 5 seconds
connection.setReadTimeout(5000); // Set read timeout to 5 seconds
Commentary
Setting timeouts ensures your application does not wait endlessly for a response. This is crucial for maintaining responsiveness and reliability.
A Final Look
Building REST clients in Java can be a rewarding yet complex endeavor. By being aware of these common pitfalls—such as poor exception handling, ignoring HTTP status codes, blocking calls, hardcoded URLs, and not configuring timeouts—you can enhance the reliability and performance of your RESTful implementations.
For more detailed guidelines on building REST APIs in Java, feel free to check out the Spring Documentation on REST or explore Java Networking Basics.
By applying these best practices in your development workflow, you can create Java REST clients that are robust, efficient, and user-friendly. Happy coding!
Checkout our other articles