Mastering Type Checking: Replace instanceof with Visitor in Java 8
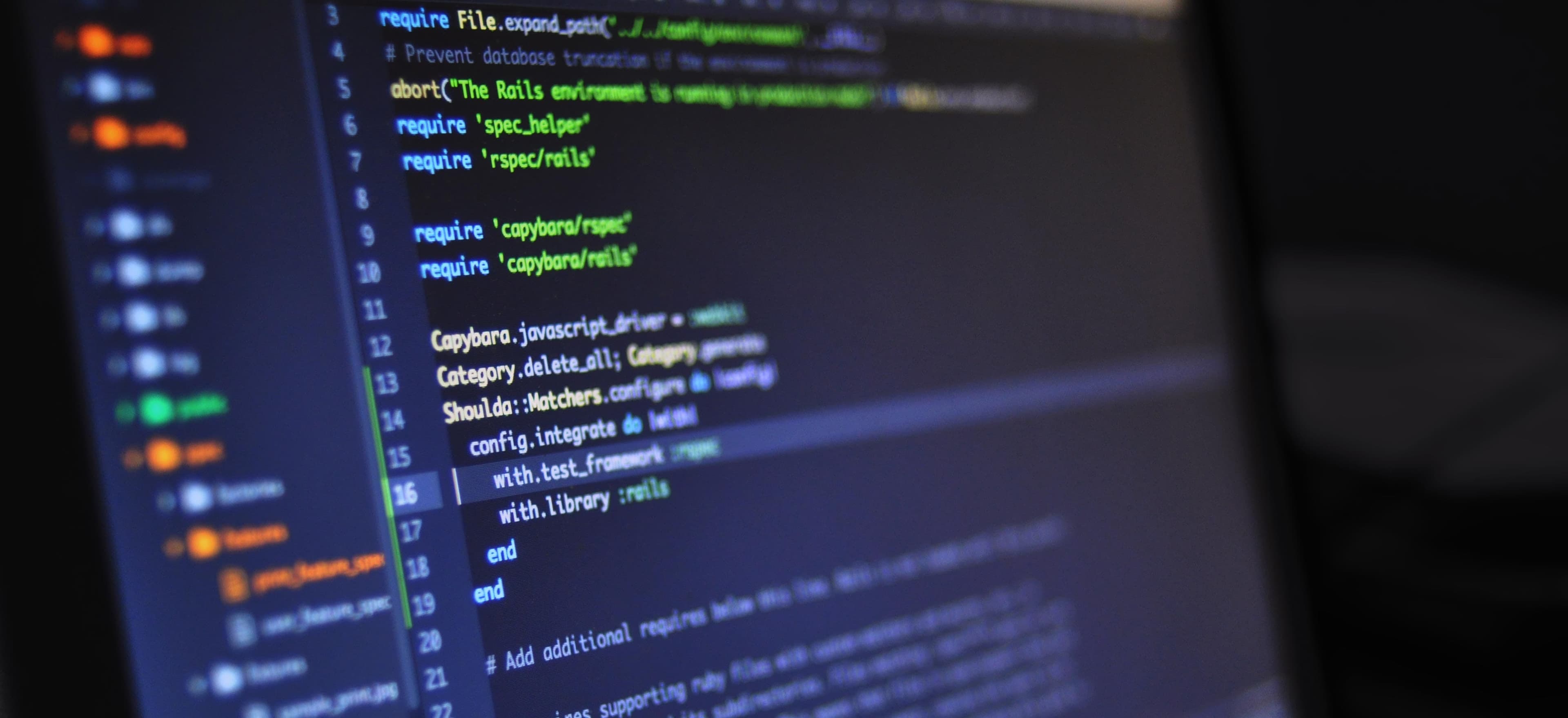
- Published on
Mastering Type Checking: Replace instanceof with Visitor in Java 8
In Java, type checking is a critical aspect of ensuring code reliability and maintainability. While instanceof
is a commonly used operator for determining an object's runtime type, it poses certain challenges, particularly as code bases grow in size and complexity. In this blog post, we will explore how to replace instanceof
with the Visitor pattern in Java 8, demonstrating both the benefits and the implementation details of this design pattern.
Understanding instanceof
The instanceof
operator is used to test whether an object is an instance of a specific class or interface. Its syntax can be as simple as:
if (obj instanceof MyClass) {
// process obj as MyClass
}
While this is straightforward, over-reliance on instanceof
can lead to code that is hard to maintain and extend. Any additional class that must be handled requires modifying existing code, which can introduce bugs and violate the Open/Closed Principle.
Drawbacks of instanceof
- Code Smell: Frequent use of
instanceof
can indicate poor design. - Maintenance Bugs: Every time a new class is introduced, you must modify existing evaluators.
- Mixing Concerns: Business logic starts to intermingle with type-checking logic.
To overcome these limitations, the Visitor pattern provides an elegant solution.
The Visitor Pattern Overview
The Visitor pattern allows you to define new operations on objects without changing the classes of the elements on which it operates. It's particularly useful when you need to perform operations on a structure of objects with different types.
Components of the Visitor Pattern
- Visitor Interface: Defines visit methods that correspond to each type of element.
- Element Interface: Defines an
accept
method to accept a visitor. - Concrete Elements: Classes that implement the Element interface.
- Concrete Visitors: Classes that implement the Visitor interface.
Example Implementation
Let’s see how we can implement the Visitor pattern in Java 8 to avoid using instanceof
.
Step 1: Define the Element and Visitor Interfaces
// Visitor interface
interface Visitor {
void visit(ElementA elementA);
void visit(ElementB elementB);
}
// Element interface
interface Element {
void accept(Visitor visitor);
}
Step 2: Create Concrete Elements
class ElementA implements Element {
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
public void operationA() {
System.out.println("Operation A");
}
}
class ElementB implements Element {
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
public void operationB() {
System.out.println("Operation B");
}
}
In this code, ElementA
and ElementB
implement the accept
method, which takes a Visitor
as an argument. This allows the Visitor
to "visit" the specific elements.
Step 3: Create Concrete Visitors
class ConcreteVisitor implements Visitor {
@Override
public void visit(ElementA elementA) {
elementA.operationA();
System.out.println("Visited Element A");
}
@Override
public void visit(ElementB elementB) {
elementB.operationB();
System.out.println("Visited Element B");
}
}
The ConcreteVisitor
implements the Visitor
interface, providing specific behavior for ElementA
and ElementB
.
Step 4: Using the Visitor
public class VisitorPatternDemo {
public static void main(String[] args) {
Element elementA = new ElementA();
Element elementB = new ElementB();
Visitor visitor = new ConcreteVisitor();
elementA.accept(visitor);
elementB.accept(visitor);
}
}
Why Use the Visitor Pattern?
- Separation of Concerns: Business logic for processing different elements is separated from the elements themselves.
- Easier Maintenance: Adding new elements or operations requires minimal changes to existing code.
- Flexibility: You can easily add operations without altering the element classes.
Real-World Scenarios for Visitor Pattern
Here are a few situations where the Visitor pattern shines:
- Complicated Object Structures: If you are working with a complex hierarchy of objects where many operations need to be performed on them.
- Multiple Operations: When you need to perform various operations on the same set of objects but want to keep the object structure unchanged.
- Reduce Conditional Logic: It greatly reduces the use of conditional statements (like
instanceof
) spread across multiple classes.
Alternatives to the Visitor Pattern
While the Visitor pattern is powerful, it’s important to remember that it's not the only option. Here are some alternative approaches:
- Double Dispatch: This is inherent to the Visitor pattern and could be implemented using interfaces or abstract classes.
- Dynamic Dispatch: Leveraging polymorphism to handle behavior, but this could lead to heavier maintenance.
- Functional Programming: Java 8 introduced lambdas, allowing for more compact code handling types and behaviors dynamically.
My Closing Thoughts on the Matter
The Visitor pattern offers a robust alternative to frequent use of instanceof
in Java 8, promoting cleaner and more maintainable code. Through clearly defined structures of visits, operations can be easily added, yielding less error-prone and more scalable code.
For more insights on design patterns in Java, check out the following resources:
By internalizing these design principles, you'll elevate your coding practices, from mundane to exemplary. Happy coding!
Checkout our other articles