Mastering Property-Based Testing: Elevate Your Code Quality
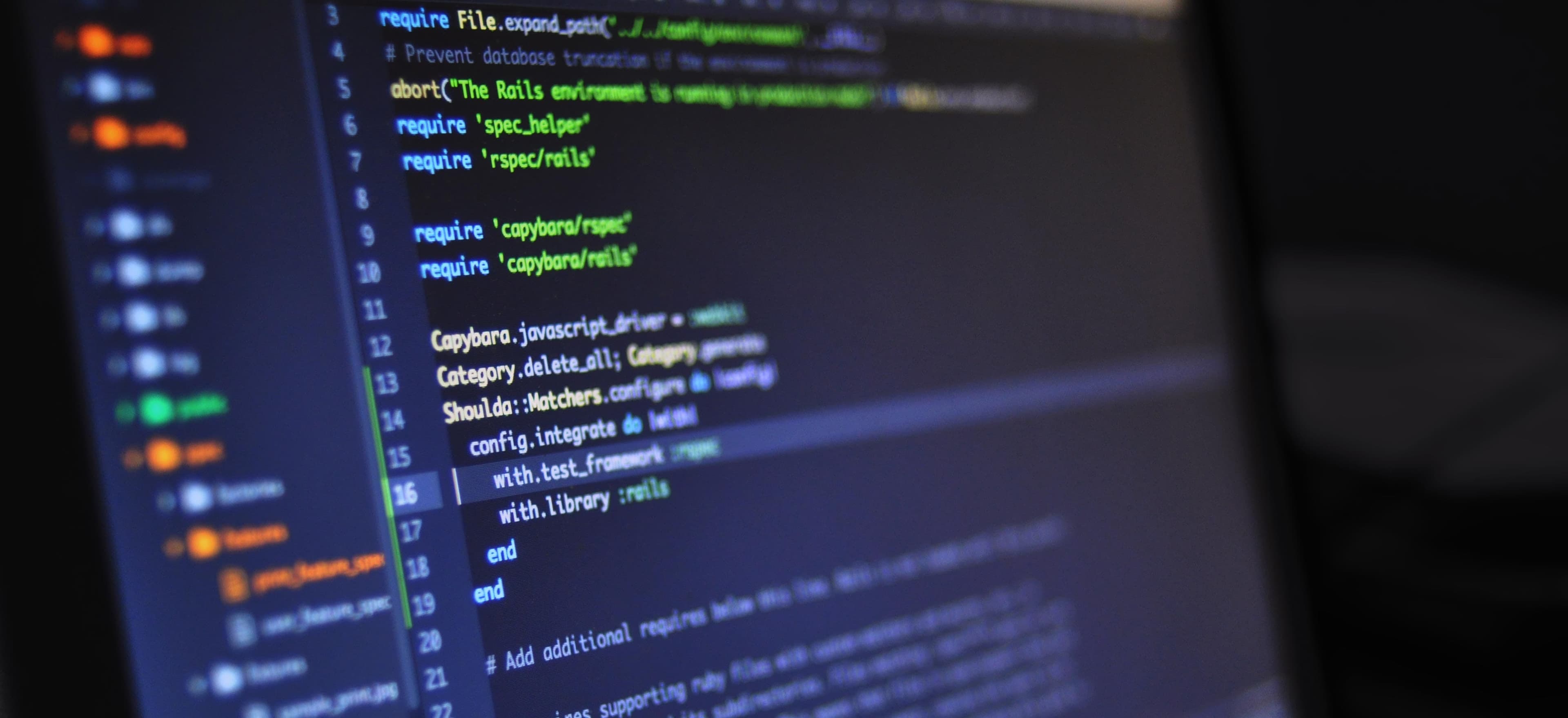
- Published on
Mastering Property-Based Testing: Elevate Your Code Quality
In the realm of software development, ensuring code quality is paramount. Traditional testing methodologies, while effective, often fall short in covering the vast combinations of input states that a program can encounter. This is where property-based testing (PBT) emerges as a powerful ally. In this blog post, we will explore what property-based testing is, how it can elevate your code quality, and how to implement it effectively in Java.
What is Property-Based Testing?
Property-based testing is a testing methodology where properties (invariants) of the code are defined, and tests are automatically generated to verify that these properties hold true across a wide range of input data. Unlike classical examples, which are predefined sets of inputs to test against expected outcomes, PBT focuses on ensuring that the stated properties or behaviors of the system are maintained regardless of the input.
Key Benefits of Property-Based Testing
- Broad Test Coverage: PBT can generate a multitude of test cases, catching edge cases that might not have been considered.
- Facilitates Better Design: Writing properties encourages developers to think critically about the behavior of their software.
- Documentation: Properties serve as documentation for expected behavior, making the codebase easier to understand.
- Efficiency in Debugging: When a test fails, PBT often provides a nearer-to-failing input, facilitating quicker resolution.
Getting Started with Property-Based Testing in Java
To implement property-based testing in Java, we can utilize frameworks like JUnit-QuickCheck or jqwik. In this blog, we will focus on JUnit-QuickCheck
, which integrates seamlessly with JUnit 5, one of the most popular testing frameworks in the Java ecosystem.
Setting Up JUnit-QuickCheck
First, ensure that you have the necessary dependencies set up in your Maven pom.xml
:
<dependency>
<groupId>com.pholser</groupId>
<artifactId>junit-quickcheck-core</artifactId>
<version>0.9.0</version>
<scope>test</scope>
</dependency>
Writing Your First Property-Based Test
Let’s dive into a simple example. Suppose we have a utility class that provides basic arithmetic operations. We want to ensure that our addition method is associative. The associative property states that for any three integers a, b, and c:
(a + b) + c == a + (b + c)
Here’s how we can set up a property-based test for this scenario:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import com.pholser.junit.quickcheck.From;
import com.pholser.junit.quickcheck.Property;
import static org.junit.jupiter.api.Assertions.assertEquals;
@ExtendWith(JUnitQuickcheck.class)
public class ArithmeticTest {
@Property
public void additionIsAssociative(@From(Integer.class) int a,
@From(Integer.class) int b,
@From(Integer.class) int c) {
int left = (a + b) + c;
int right = a + (b + c);
assertEquals(left, right);
}
}
Code Breakdown
- @ExtendWith(JUnitQuickcheck.class): This integrates JUnit-QuickCheck with JUnit 5.
- @Property: This annotation indicates that the following method is a property test.
- @From(Integer.class): This generates random integers as input for the test method.
In this test, the framework generates numerous combinations of integers a, b, and c to verify the associative nature of addition. If any combination fails the assertion, it produces a detailed report of the failure.
Advanced Property-Based Testing Concepts
While basic properties are powerful in their own right, let’s explore some advanced concepts that can further enhance your property-based testing experience.
Custom Generators
Sometimes, you need inputs that follow specific criteria. You can define custom generators for your tests. Here’s an example of a generator that produces positive integers:
import com.pholser.junit.quickcheck.Generator;
public class PositiveIntegerGenerator extends Generator<Integer> {
public PositiveIntegerGenerator() {
super(Integer.class);
}
@Override
public Integer next() {
return Math.abs(nextInt());
}
}
You can then use this generator in your property testing:
@Property
public void positiveAddition(@From(PositiveIntegerGenerator.class) int a,
@From(PositiveIntegerGenerator.class) int b) {
assertEquals(a + b, b + a); // Commutative property
}
Combining Properties
Multiple properties can often be tested together. For example, if you have an implementation of multiplication as well as addition, you might verify that multiplication distributes over addition:
@Property
public void multiplicationDistributesOverAddition(int a, int b, int c) {
assertEquals(a * (b + c), (a * b) + (a * c));
}
Integration with CI/CD Pipelines
Integrating property-based tests into your Continuous Integration/Continuous Deployment (CI/CD) pipelines is straightforward. Ensure your build server runs all tests, including your property-based tests, to maintain high code quality standards.
Handling Shrinking
When a test fails, QuickCheck automatically tries to find the smallest input that triggers the failure, known as "shrinking." This process is crucial for debugging. It allows you to reduce complex bugs to simpler cases, making diagnosing issues much more manageable.
In Conclusion, Here is What Matters: Elevating Code Quality with Property-Based Testing
Property-based testing can transform your approach to ensuring code quality. By verifying that properties hold true for a wide range of inputs, you reduce the risk of errors sneaking into your code base. The integration of frameworks like JUnit-QuickCheck
simplifies the process, allowing both novice and seasoned developers to benefit.
As software systems grow increasingly complex, embracing advanced testing methodologies, such as PBT, can lead to more robust and maintainable code.
For further exploration of Java testing strategies, you might find the following resources helpful:
- Effective Unit Testing - A comprehensive guide on unit testing techniques.
- Introduction to Test-Driven Development (TDD) - Insights from Martin Fowler on TDD practices.
Elevate your code quality, improve your testing strategy, and consider integrating property-based tests into your workflow today!