Avoiding NullPointerExceptions with Guava Preconditions
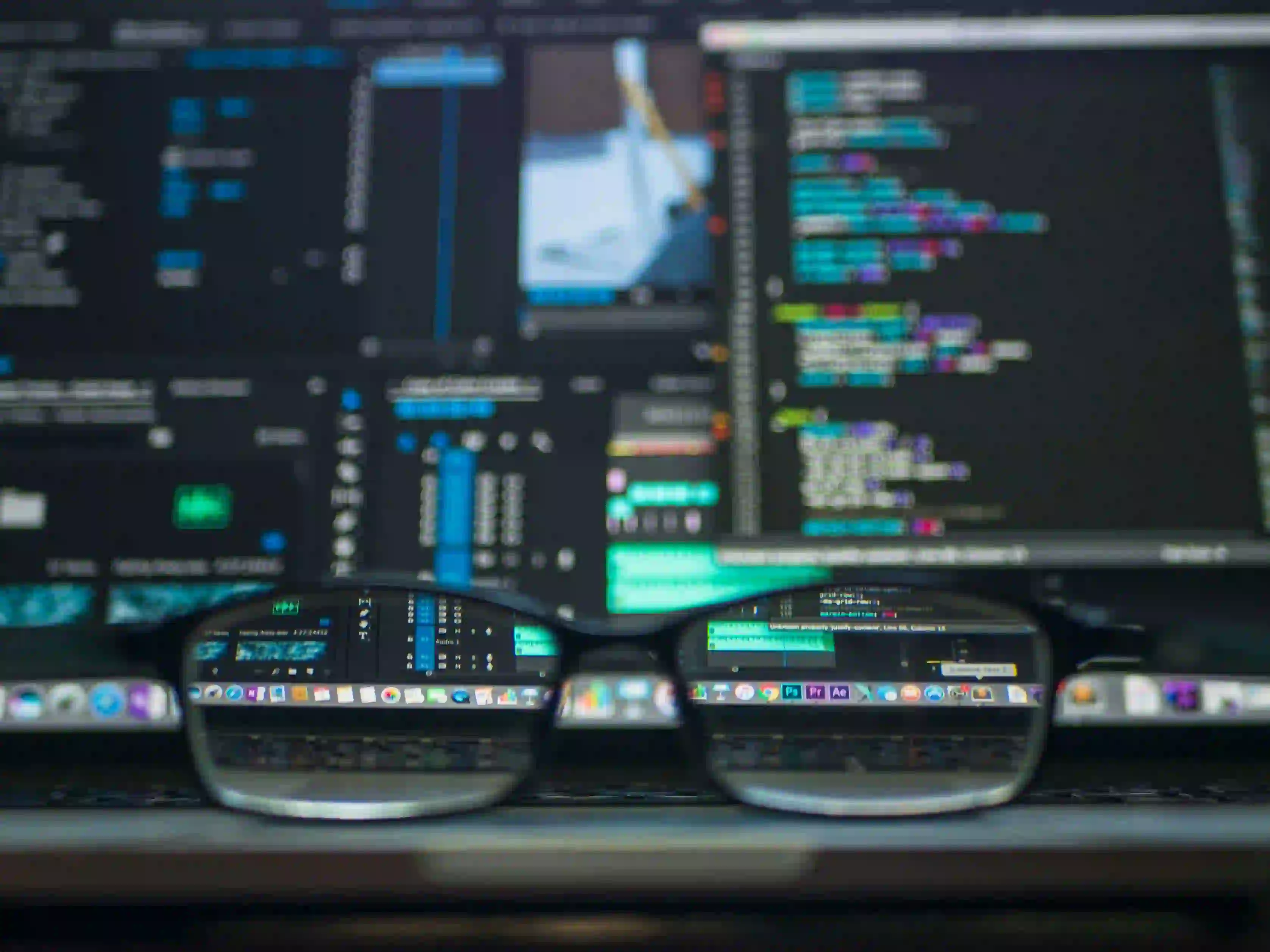
Avoiding NullPointerExceptions with Guava Preconditions
NullPointerExceptions (NPEs) pose one of the most common threats in Java programming, often leading to unpredictable behavior and hard-to-trace bugs. While NullPointerExceptions can be avoided through diligent coding practices, Java’s optional handling of nulls sometimes leaves room for error. In this blog post, we will explore how to effectively mitigate NPEs using Google Guava's Preconditions
class, enhancing your code's robustness and reliability.
What is Google Guava?
Google Guava is an open-source, core libraries project that offers various utilities for Java programmers. It provides collections, caches, primitives support, concurrency libraries, common annotations, string processing, I/O, and more.
By using Guava’s Preconditions
class, checking conditions becomes both succinct and clear. The syntax is simple, and it elevates your code’s readability, explicitly handling null checks in a maintainable manner.
Why Use Preconditions?
Using preconditions helps to:
- Clearly define expectations for method arguments.
- Fail fast by throwing informative exceptions.
- Simplify control flow and reduce nesting.
- Improve code quality by stating conditions upfront.
Setting Up Guava
Before we dive into the details of Preconditions
, you need to integrate Guava into your project. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version> <!-- Make sure to check for the latest version -->
</dependency>
For Gradle, include this line in your build.gradle
file:
implementation 'com.google.guava:guava:31.0.1-jre' // Check for updates before using
Using Preconditions
The Preconditions
class offers several static methods that ensure the provided arguments meet expected conditions. Let's look at some commonly used methods.
Example 1: Validating Arguments
Suppose you have a method that calculates the area of a rectangle, which should not accept null dimensions.
import com.google.common.base.Preconditions;
public class Rectangle {
private final double length;
private final double width;
public Rectangle(Double length, Double width) {
this.length = Preconditions.checkNotNull(length, "Length must not be null");
this.width = Preconditions.checkNotNull(width, "Width must not be null");
}
public double getArea() {
return length * width;
}
}
Why Use checkNotNull?
The checkNotNull
method checks whether the argument is null. If it is, it throws a NullPointerException
with the provided message. This technique allows you to fail fast—immediately identifying the source of the error right at the method entry, thus simplifying debugging.
Example 2: Checking State Conditions
Imagine a method that expects both length and width to be positive.
public Rectangle(Double length, Double width) {
this.length = Preconditions.checkNotNull(length, "Length must not be null");
this.width = Preconditions.checkNotNull(width, "Width must not be null");
Preconditions.checkArgument(length > 0, "Length must be positive");
Preconditions.checkArgument(width > 0, "Width must be positive");
}
Here, we use checkArgument
to enforce that the values are positive. If either check fails, a more informative exception is thrown, allowing developers to resolve the issue quickly.
Example 3: Chaining Preconditions
You can also chain precondition checks, which can be particularly useful in more complex methods where multiple conditions must be validated.
public void setDimensions(Double length, Double width) {
Preconditions.checkNotNull(length, "Length must not be null");
Preconditions.checkArgument(length > 0, "Length must be positive");
Preconditions.checkNotNull(width, "Width must not be null");
Preconditions.checkArgument(width > 0, "Width must be positive");
this.length = length;
this.width = width;
}
Chaining checks provides clarity. Each Precondition
effortlessly communicates the intent behind the validation, ultimately enhancing code readability.
Practical Use Case: Understanding NullPointerExceptions
Consider a hypothetical application where you accept user inputs for rectangle dimensions. Without proper null checks, if a user inputs a null value, the application throws an NPE, potentially crashing the entire system.
By incorporating the precautions we've just discussed, you are proactively preventing this issue.
Example 4: Integration in an Application
Suppose you are creating an application that calculates the area based on user input.
import java.util.Scanner;
public class RectangleApp {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter length of rectangle: ");
Double length = scanner.nextDouble();
System.out.println("Enter width of rectangle: ");
Double width = scanner.nextDouble();
try {
Rectangle rectangle = new Rectangle(length, width);
System.out.println("Area: " + rectangle.getArea());
} catch (NullPointerException | IllegalArgumentException e) {
System.err.println("Error: " + e.getMessage());
}
scanner.close();
}
}
Why This is Important
By including robust argument checks, you ensure that only valid inputs are processed. The catch block informs the user of invalid input, improving the user experience while safeguarding the program from crashing unexpectedly.
My Closing Thoughts on the Matter
NullPointerExceptions can derail even the most well-structured Java applications. However, by employing Guava’s Preconditions
, we can significantly reduce the chances of encountering these exceptions. You set clear expectations for method parameters, leading to improved code readability and fewer runtime errors.
Whether you are developing a simple utility or a complex application, the Preconditions
class should become a part of your standard coding practices. So next time you write a method, take a moment to consider how you can utilize Guava to protect against null values and ensure proper argument checks.
For further exploration of Guava’s capabilities, refer to the official documentation here.
By following these practices, not only do you enhance code quality, but you also foster a culture of robustness and reliability in your software development practices. Happy coding!