Cutting AWS Costs: Going Off-Heap for Better Latency
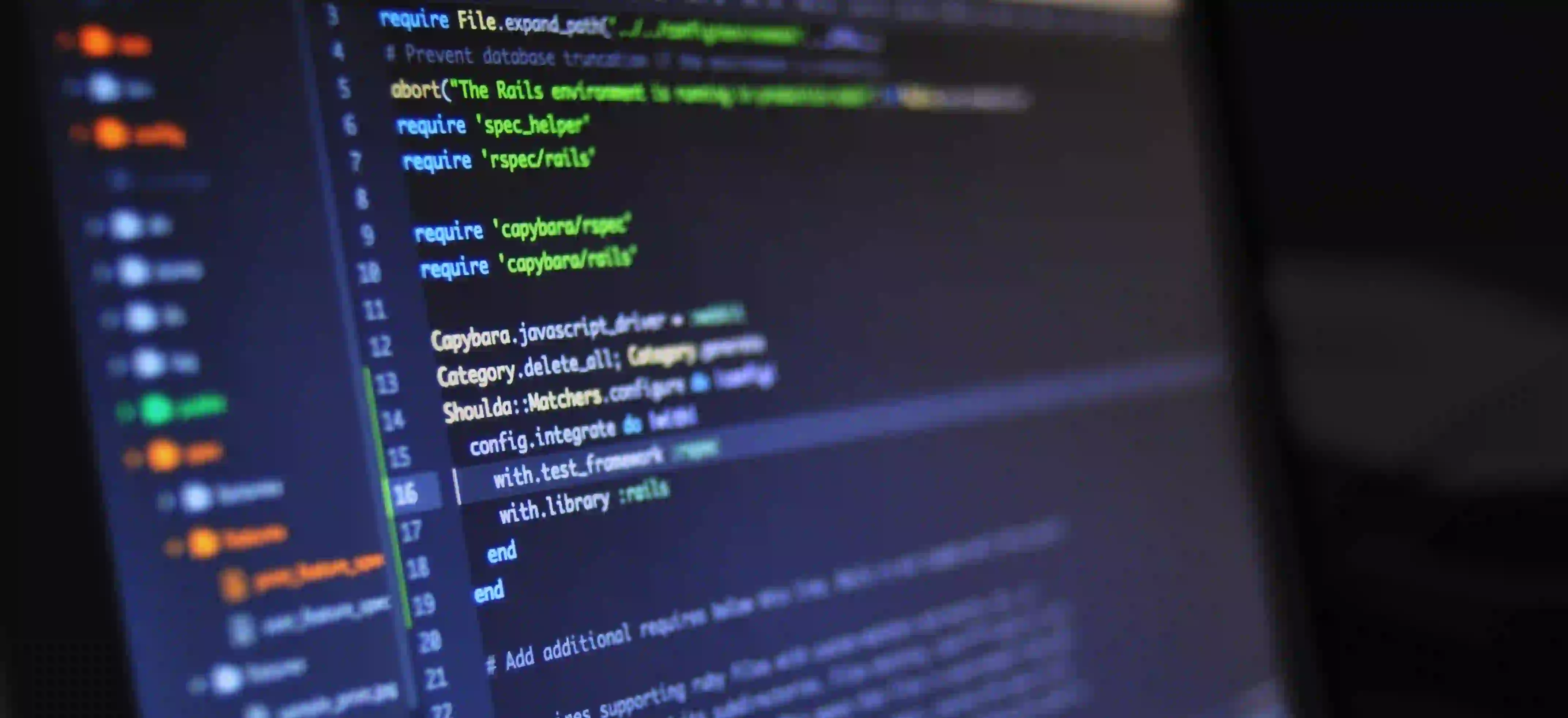
Cutting AWS Costs: Going Off-Heap for Better Latency
In the ever-evolving world of cloud computing, Amazon Web Services (AWS) dominates as a powerful platform. However, with great power comes great expense. Many businesses are constantly searching for ways to minimize costs while maximizing performance. One effective strategy to achieve these goals is to shift from on-heap to off-heap memory. This blog post will delve into what going off-heap actually means, its advantages concerning cost-saving and efficiency, and how to implement it with Java.
Understanding On-Heap vs. Off-Heap Memory
Heap Memory is the area of memory used for dynamic memory allocation in Java. Memory is allocated from the heap using the new
keyword, and this memory is managed by the Java Garbage Collector (GC). The primary benefits of heap memory include ease of allocation and automatic garbage collection, which can make development faster:
String myString = new String("Hello, World!");
However, all good things come with downsides. As applications scale, the overhead of garbage collection can lead to increased latency and inconsistent performance. Moreover, heap memory is subject to the limits of the Java Virtual Machine (JVM), which can further complicate scaling.
Off-Heap Memory, on the other hand, refers to memory allocated outside of the JVM's heap. This kind of memory offers a few significant advantages:
- Reduced Latency: By controlling memory management and avoiding the overhead of GC, applications can typically respond faster.
- Performance Scaling: Off-heap memory is not constrained by the JVM's limits, allowing for better resource utilization.
- Cost Efficiency: Reducing the number of allocated JVM instances can directly decrease your AWS costs.
Why Choose Off-Heap?
1. Improved Latency
Latency is a critical metric for many applications, particularly those that require real-time processing. Off-heap memory can significantly reduce pause times caused by garbage collection. Instead of waiting for the GC to run, applications can access off-heap memory directly. This direct memory access allows for faster data retrieval and manipulation.
2. Better Control
Using off-heap memory gives developers finer control over memory management. You can decide when to allocate and deallocate memory, allowing for optimization based on your application's needs.
3. Cost Cutting
AWS charges based on the resources you consume. Operating with a smaller heap can lead to reduced costs, especially when scaling applications. By using off-heap memory, you can lower your JVM requirements, subsequently decreasing costs associated with EC2 instances, memory utilization, and overall infrastructure expenses.
Implementing Off-Heap Memory in Java
To effectively utilize off-heap memory in Java, you can leverage the Java Direct ByteBuffer API. This allows you to allocate memory outside of the JVM heap and enables you to perform read/write operations on this memory with extreme efficiency.
Example Code
Below is a simple example of using DirectByteBuffer in Java:
import java.nio.ByteBuffer;
public class OffHeapExample {
public static void main(String[] args) {
// Allocate off-heap memory
int bufferSize = 1024; // 1 KB buffer
ByteBuffer buffer = ByteBuffer.allocateDirect(bufferSize);
// Write data to off-heap memory
for (int i = 0; i < bufferSize; i++) {
buffer.put((byte) i);
}
// Read data from off-heap memory
buffer.flip(); // Switch from writing to reading
while (buffer.hasRemaining()) {
System.out.println(buffer.get());
}
}
}
Commentary
-
allocateDirect(int capacity): This method allocates a buffer with a specified capacity directly in the off-heap memory.
-
put(byte) and get(): These methods allow writing to and reading from the allocated off-heap memory.
-
flip(): This method prepares the buffer for reading by resetting the position and limiting it to the current position.
Choosing off-heap over on-heap is particularly beneficial for applications handling large data sets, such as caching datasets or processing high-frequency trading information.
Libraries for Off-Heap Management
While the native Java DirectByteBuffer is a good start, several libraries can help make off-heap management even easier and more efficient. Some of the most notable ones include:
- Apache Ignite: A distributed in-memory computing platform that supports off-heap memory management. Apache Ignite Off-Heap
- MapDB: This offers a concurrent Java-based database engine with off-heap memory management capabilities. MapDB Documentation
- Chronicle: A high-performance library designed for message passing and off-heap memory. Chronicle Documentation
Utilizing these libraries can significantly simplify the implementation and management of off-heap memory.
Performance Considerations
Although off-heap memory presents several advantages, it's essential to be aware of potential downsides:
- Complexity: Managing memory manually can result in bugs if not done carefully. This requires rigorous testing and diligence.
- No Automatic Cleanup: You've got to ensure that resources are freed explicitly to avoid memory leaks.
Use Cases for Off-Heap Memory
Determining when to use off-heap memory is crucial. Here are some scenarios where off-heap memory shines:
- In-Memory Caching: Systems like Redis or Memcached operating in-memory might benefit significantly from off-heap memory for faster access.
- High-Throughput Applications: Applications that require low-latency data access, such as trading platforms or online gaming.
- Large Batch Processing: Systems needing to manage large data after extensive processing without overhead from the garbage collector.
Wrapping Up
Cutting AWS costs while maintaining performance can feel like a daunting task. However, shifting towards off-heap memory management can effectively alleviate some of the burdens associated with memory allocation. With reduced latency, improved control, and potential for significant cost savings, going off-heap is an excellent choice for many Java applications.
Make sure to perform thorough testing and consider using libraries to simplify your off-heap management. With the right strategies and a keen understanding of your application's needs, you can leverage off-heap memory to make AWS both economical and efficient.
If you're ready to dive deeper into off-heap memory management, start exploring Apache Ignite or MapDB today. Your AWS costs will thank you.