Common MongoDB Configuration Issues with Spring Data
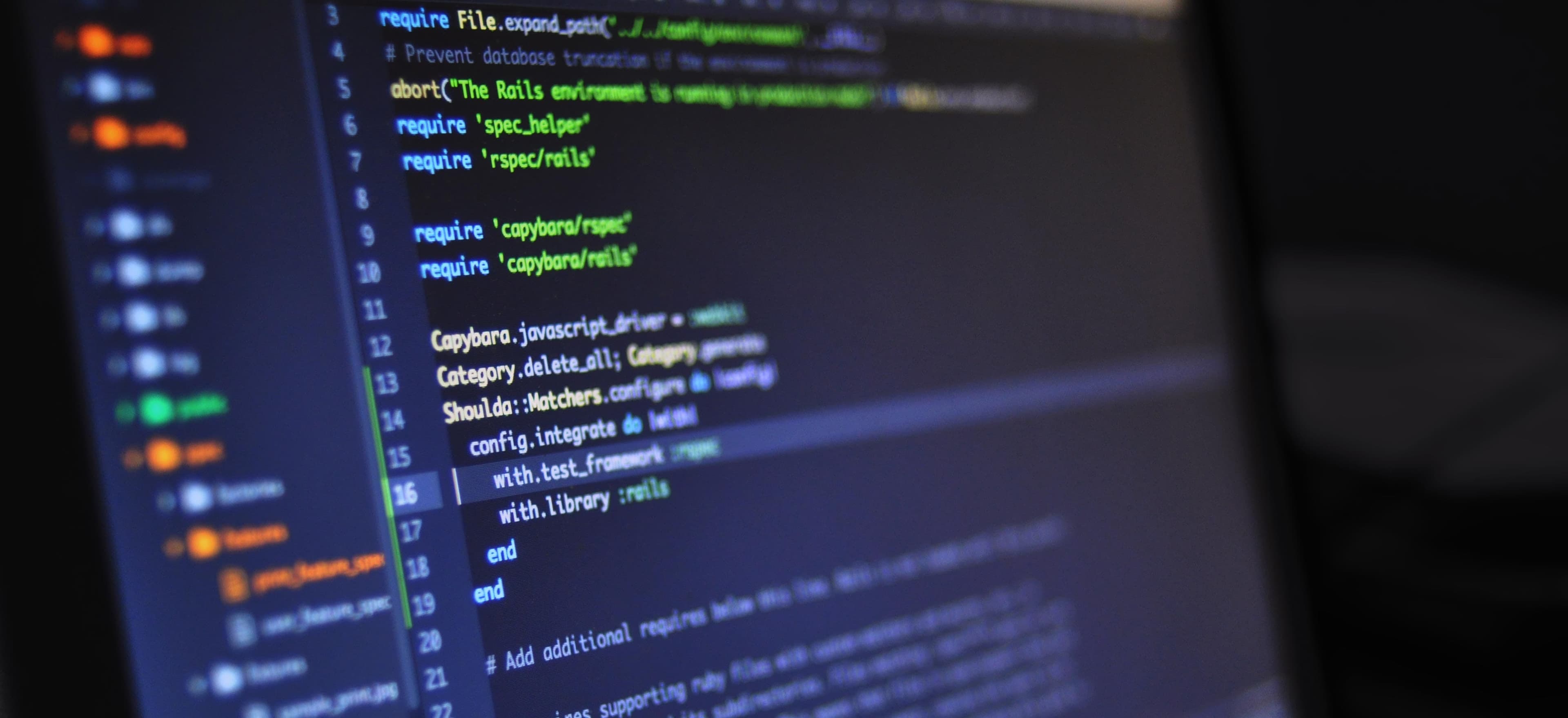
- Published on
Common MongoDB Configuration Issues with Spring Data
Spring Data MongoDB has revolutionized the way developers interact with MongoDB databases using Java. While it simplifies operations, issues may arise during configuration or usage, especially for those new to the framework or MongoDB itself. In this post, we will explore some common configuration challenges, highlight best practices, and examine code snippets to illustrate solutions.
Overview of Spring Data and MongoDB
Spring Data MongoDB provides a simplified way to implement data access layers, making it easier to work with MongoDB in a Spring application. It abstracts the low-level details while still providing powerful features like repository support, custom queries, and mapping entities to documents.
However, configuring Spring Data with MongoDB isn’t always straightforward. Below, we will discuss common issues and solutions.
Common Configuration Issues
1. MongoDB Not Found or Connection Refusal
One of the most frequent problems developers face is the inability to connect to the MongoDB server. This may happen due to incorrect URI configuration.
Solution: Check the MongoDB URI in your Spring application configuration file (application.properties or application.yml).
Code Snippet
spring.data.mongodb.uri=mongodb://localhost:27017/mydatabase
Why: Ensure that the URI points to the right IP address and port. The default port for MongoDB is 27017. Make sure MongoDB is running on your machine or the specified server.
2. Spring Boot Autoconfiguration Issues
Using Spring Boot simplifies configuration, but sometimes, autoconfiguration might not work as intended. This often results in exceptions like No MongoDbFactory bean found
.
Solution: Ensure that you have the correct dependencies in your pom.xml
file.
Code Snippet
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
Why: This starter dependency includes MongoDB configuration and necessary libraries, ensuring Spring Boot can handle MongoDB-related operations seamlessly.
3. Missing or Incorrect Database Name Configuration
Failing to specify the database name can cause issues where the application might connect to a default or incorrect database.
Solution: Explicitly specify your database name in the application configuration.
Code Snippet
spring.data.mongodb.database=mydatabase
Why: Defining the database name prevents ambiguity and directs your application to interact with the intended MongoDB database.
4. Security Authentication Issues
If your MongoDB requires authentication, failing to provide the correct credentials often results in connection failures.
Solution: Update your MongoDB URI to include the user and password.
Code Snippet
spring.data.mongodb.uri=mongodb://username:password@localhost:27017/mydatabase
Why: Properly setting credentials in your connection string ensures that your application has the necessary permissions to access the database.
5. Using Non-Default Port
If your MongoDB is running on a non-default port, neglecting this detail will lead to connection errors.
Solution: Specify the custom port in your URI.
Code Snippet
spring.data.mongodb.uri=mongodb://localhost:27018/mydatabase
Why: Adjusting the URI to include the correct port allows your application to connect successfully, as MongoDB listens for connections on the specified port.
6. Mapping Issues with Custom Classes
Sometimes, you may experience mapping issues when trying to persist or retrieve objects with custom class structures.
Solution: Utilize annotations like @Document
, @Id
, and others properly.
Code Snippet
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "users")
public class User {
@Id
private String id;
private String name;
// getters and setters
}
Why: Proper annotations ensure that your Java objects are recognized as MongoDB documents, allowing for seamless data persistence and retrieval.
7. Repository Not Found Exception
If you try to autowire a repository and get a RepositoryNotFoundException
, it usually means that Spring could not find the repository interface.
Solution: Ensure repository interfaces extend the appropriate Spring Data interfaces.
Code Snippet
import org.springframework.data.mongodb.repository.MongoRepository;
public interface UserRepository extends MongoRepository<User, String> {
User findByName(String name);
}
Why: By extending MongoRepository
, your repository gains pre-defined methods for common operations, significantly reducing boilerplate code.
8. Document Versioning Conflicts
Concurrent modifications can lead to issues if you don't handle document versioning.
Solution: Use the @Version
annotation.
Code Snippet
import org.springframework.data.annotation.Version;
@Document(collection = "users")
public class User {
@Id
private String id;
@Version
private Long version;
// other fields
}
Why: Adding the @Version
field ensures that concurrent modifications are handled correctly, preventing lost updates.
9. Query Performance Issues
MongoDB can return slow queries if the appropriate indexing is not set up.
Solution: Create indexes on fields you often query against.
Code Snippet
@Indexed
private String name;
Why: Indexes significantly improve query performance, especially for large datasets. It's important to analyze query plans for optimization.
Additional Resources
Bringing It All Together
Configuring Spring Data MongoDB can sometimes be daunting, but being aware of common issues can significantly ease the process. Ensure your configurations are correct, dependencies are included, and that you properly handle data mapping and repositories. As you get familiar with the nuances of Spring Data MongoDB, catching these common pitfalls will become second nature.
By following best practices outlined in this blog, you can harness the full power of Spring Data MongoDB while avoiding common configuration obstacles. Happy coding!