Common Pitfalls When Integrating Spring with Apache Hadoop
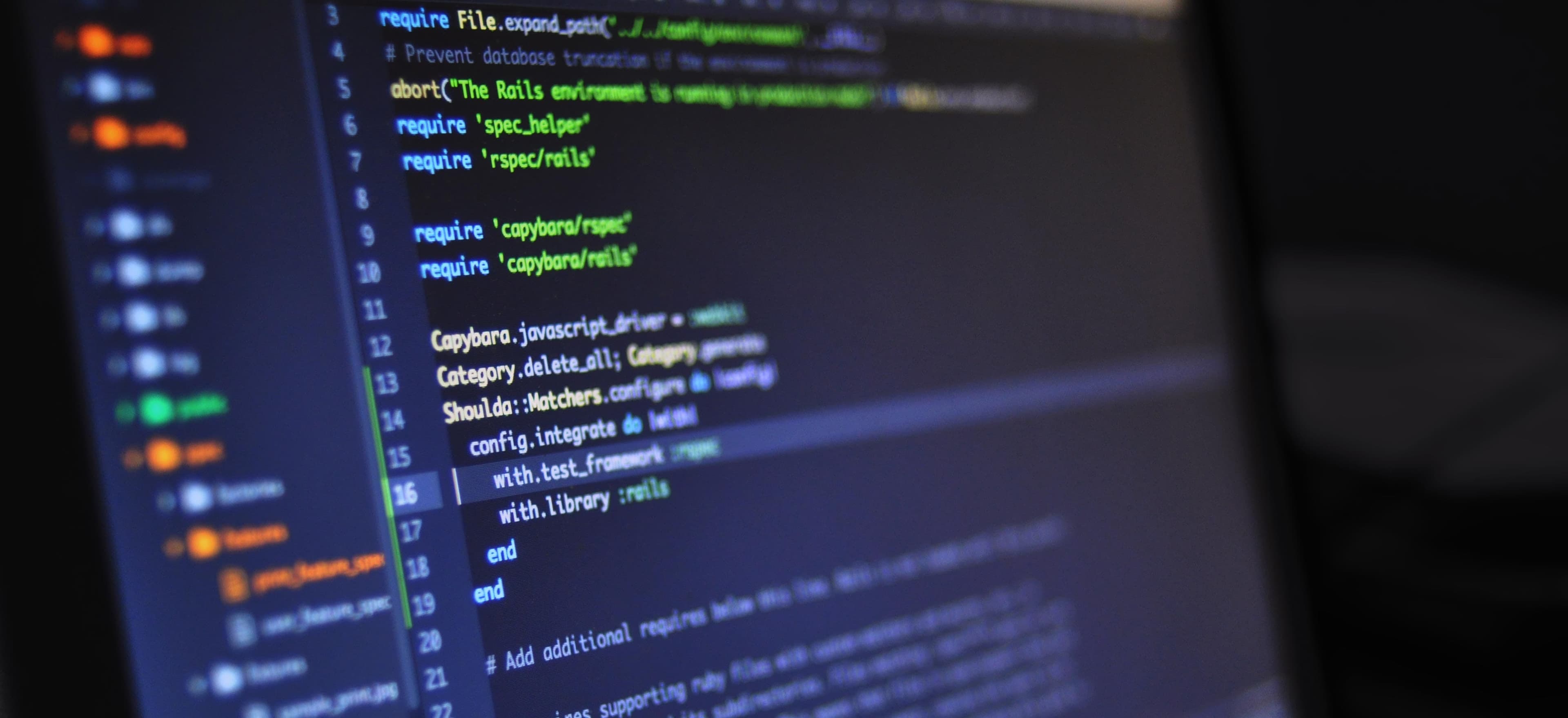
- Published on
Common Pitfalls When Integrating Spring with Apache Hadoop
Integrating Spring Framework with Apache Hadoop is a common task for developers dealing with big data applications in Java. Both frameworks have their strengths, and marrying them can lead to powerful data processing capabilities. However, this integration can introduce several pitfalls that developers need to be aware of.
Understanding these challenges can significantly streamline your development process and elevate the quality of your applications. In this post, we will discuss common pitfalls, elaborate on code examples, and provide guidelines on how to overcome these obstacles effectively.
1. Incorrect Dependency Management
One of the first hurdles developers face is dependency management. Both Spring and Hadoop have their versions, and mismatches can lead to runtime issues or compile-time errors.
Why It Matters
When your application utilizes multiple libraries, it's crucial that compatible versions are used. If a newer version of Spring is used that relies on updated libraries yet is paired with an older version of Hadoop, conflicts may arise.
Example Code
Here’s how you can manage dependencies using Maven:
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>3.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
</dependency>
Recommendation
Always check for compatibility using the Spring framework compatibility matrix when you are integrating it with Hadoop. Familiarize yourself with the release notes of both frameworks to avoid incompatibilities.
2. Misconfiguration of Spring Context
Another common pitfall is misconfiguring the Spring application context. While Spring makes it easy to manage object lifecycles and configurations, incorrect settings can lead to performance degradation or app crashes.
Why It Matters
Hadoop applications often require substantial resources and specific configurations. Not setting up the Spring context properly can lead to issues where Hadoop may not utilize the desired configurations.
Example Code
Ensure the proper context is configured like this:
<context:component-scan base-package="com.example.hadoop"/>
<bean id="hadoopConfig" class="org.apache.hadoop.conf.Configuration">
<property name="fs.defaultFS" value="hdfs://localhost:9000"/>
</bean>
<bean id="hadoopJob" class="org.apache.hadoop.mapreduce.Job">
<property name="configuration" ref="hadoopConfig"/>
</bean>
Recommendation
Make sure to test the configuration in a controlled environment before deploying it to production. Tools like Spring Boot can help streamline this process further.
3. Inefficient Data Serialization
When working with Hadoop, data serialization/deserialization can significantly affect performance. New developers often overlook the importance of efficient serialization mechanisms when integrating with Spring.
Why It Matters
Hadoop runs best with specific serialization formats like Avro or Protobuf, which outperform Java serialization. Utilizing Java's default serialization can result in increased memory use and slower serializing speed.
Example Code
Use Avro for serialization like this:
import org.apache.avro.specific.SpecificRecordBase;
public class MyAvroRecord extends SpecificRecordBase {
// Field and methods defined here for Avro serialization
}
Recommendation
Always opt for better serialization methods. For more information on Avro serialization, read the Apache Avro documentation.
4. Ignoring Fault Tolerance
Hadoop inherently provides fault-tolerance mechanisms. However, when using Spring, developers might inadvertently disable these features.
Why It Matters
Fault tolerance ensures that your application remains resilient even in the face of failures. Not leveraging these built-in capabilities can lead to data loss or prolonged downtime.
Example Code
You can explicitly set up retry mechanisms in Spring:
@Bean
public RetryTemplate retryTemplate() {
RetryTemplate retryTemplate = new RetryTemplate();
FixedBackOffPolicy policy = new FixedBackOffPolicy();
policy.setBackOffPeriod(5000); // 5 seconds
retryTemplate.setBackOffPolicy(policy);
return retryTemplate;
}
Recommendation
Use Spring's @Retryable
annotation or RetryTemplate
to safeguard critical operations. Always analyze the error handling strategies while designing your application.
5. Underestimating Resource Management
Hadoop is resource-intensive, and many developers overlook the resource management settings in Spring applications. Poor management may lead to resource leaks, high memory usage, or even application crashes.
Why It Matters
Hadoop clusters need optimal resource allocation to function efficiently. Failing to manage resources can result in degraded performance, especially under heavy loads.
Example Code
To manage resources effectively, use this configuration:
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="org.h2.Driver"/>
<property name="url" value="jdbc:h2:mem:testdb"/>
<property name="username" value="sa"/>
<property name="password" value=""/>
</bean>
Recommendation
Always monitor your application's resource usage using tools like Apache Ambari or Spring Boot Actuator.
6. Lack of Unit Testing
The integration logic between Spring and Hadoop can get convoluted, and many developers neglect unit testing those integrations. Not writing tests can result in hidden bugs that only surface during production.
Why It Matters
Comprehensive tests help to catch mistakes early in the development process, ensuring that the code meets the required performance and functionality before hitting production.
Example Code
Writing a simple unit test might look like this:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {AppConfig.class})
public class HadoopIntegrationTest {
@Autowired
private Job hadoopJob;
@Test
public void testJobExecution() {
// Setup and execute the Hadoop job
assertTrue(hadoopJob.isComplete());
}
}
Recommendation
Leverage testing frameworks like JUnit or Mockito to ensure you have adequate coverage for your integration code.
To Wrap Things Up
Integrating Spring with Apache Hadoop can be an incredibly powerful step in your Java application development, but it is fraught with potential pitfalls. From dependency management to fault tolerance, understanding the common challenges can save you from long-term headaches.
By following the recommendations and learning from the examples provided, you can create robust, scalable applications that leverage the strengths of both Spring and Hadoop effectively. If you would like to dive deeper into these frameworks, consider exploring the official Spring documentation and the Apache Hadoop documentation. Keep these points in mind, and happy coding!