Mastering Java Lambda Errors: Common Pitfalls Explained
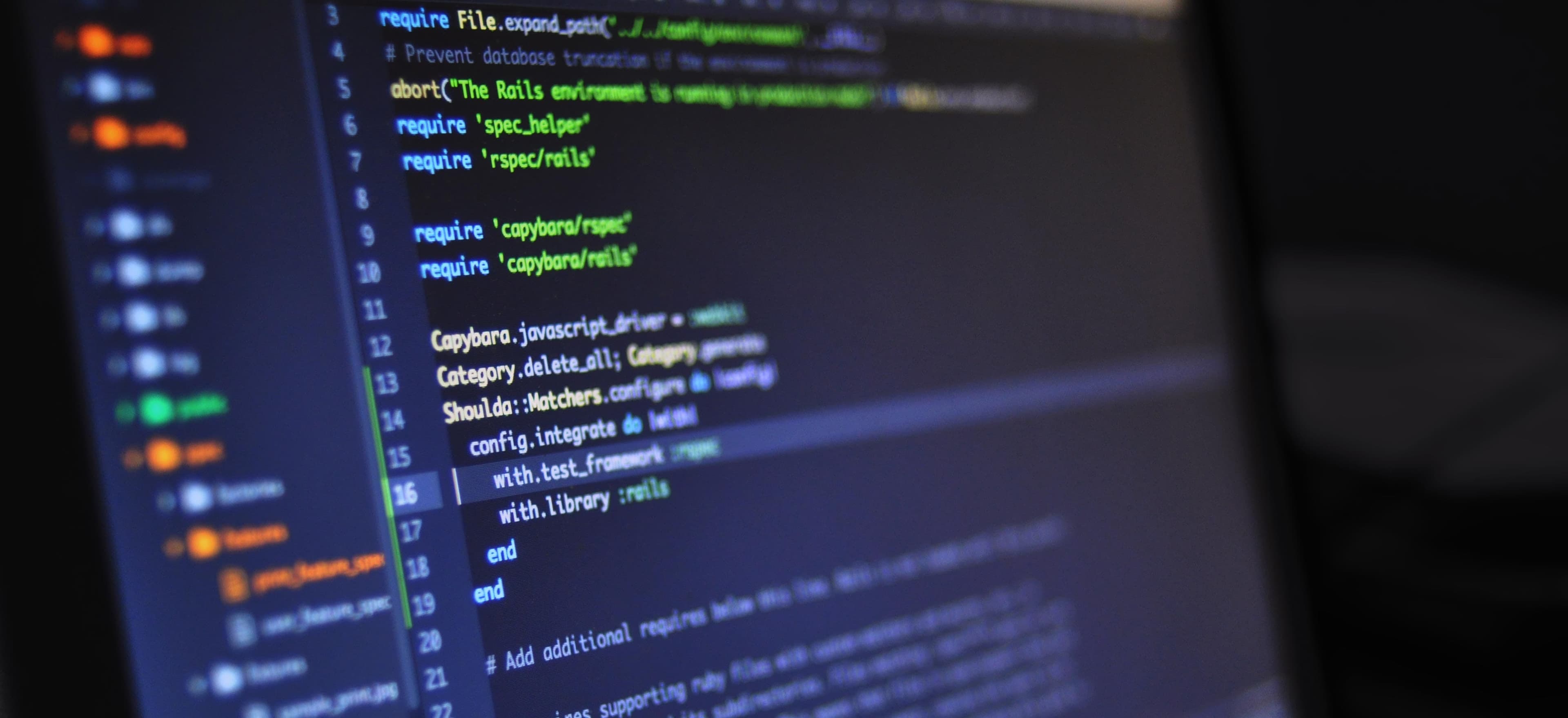
- Published on
Mastering Java Lambda Errors: Common Pitfalls Explained
Java's introduction of lambda expressions has transformed the way developers write code. This powerful feature, added in Java 8, allows for cleaner and more concise code, particularly when you're dealing with functional programming paradigms. However, with great power comes great responsibility - and often, errors. In this blog post, we will explore common pitfalls related to lambda expressions in Java, understand why they occur, and discover simple solutions to rectify them.
What Are Lambda Expressions?
A lambda expression is a block of code that you can pass around as if it were a first-class citizen. They enable you to write clearer and more expressive code, especially when combined with functional interfaces. A functional interface is an interface that contains only one abstract method. Consider the following example for a quick reference:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
}
public class LambdaExample {
public static void main(String[] args) {
// Using a lambda expression to implement the interface
MyFunctionalInterface myLambda = () -> System.out.println("Hello, Lambda!");
myLambda.execute();
}
}
Why Use Lambda Expressions?
- Conciseness: Lambda expressions often reduce boilerplate code, making it easier to read and maintain.
- Improved Readability: Functional styles often make the intent of the code clearer.
- Support for Functional Programming: Java begins to embrace functional programming, aligning with languages like Scala and Python.
While lambda expressions come with these advantages, they also introduce complex error scenarios. Let's delve into some common pitfalls and how to handle them effectively.
Common Pitfalls and Their Solutions
1. Type Inference Issues
Java's type inference may sometimes lead to confusion when using lambda expressions, especially on method parameters.
import java.util.Arrays;
import java.util.List;
public class TypeInferenceExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using a lambda expression without explicit type definition
names.forEach(name -> {
System.out.println(name.toUpperCase());
});
}
}
Why it Matters
In more complex code, failing to explicitly define parameter types can lead to errors that are hard to debug. This is especially true when dealing with collections that contain elements of different types.
Solution
Always ensure you correctly define the types of the parameters if unsure about type inference. This is particularly true in generically typed methods or when overloading methods:
names.forEach((String name) -> System.out.println(name.toUpperCase()));
2. this
Reference in Lambda Expressions
One common mistake developers make is the usage of this
within a lambda expression. Unlike anonymous classes, lambda expressions inherit this
from their enclosing context.
public class OuterClass {
private String instanceVariable = "Outer";
public void execute() {
Runnable runnable = () -> {
System.out.println(instanceVariable); // Correctly accesses OuterClass's instance variable
};
runnable.run();
}
public static void main(String[] args) {
new OuterClass().execute();
}
}
Why it Matters
If you intend to refer to the lambda's own instance variable, you will inadvertently access the instance variable of the containing class.
Solution
If you need to differentiate between the enclosing instance and the instance of the lambda, consider using a different name or using an explicit reference to the outer class.
public void execute() {
OuterClass outer = this; // Explicit reference
Runnable runnable = () -> {
System.out.println(outer.instanceVariable);
};
runnable.run();
}
3. Checked Exceptions
Another significant pitfall arises from dealing with checked exceptions. Unlike traditional methods, lambda expressions cannot throw checked exceptions unless they are declared.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class CheckedExceptionExample {
public static void main(String[] args) {
Runnable runnable = () -> {
try {
Files.readAllBytes(Paths.get("nonexistentfile.txt"));
} catch (IOException e) {
e.printStackTrace();
}
};
runnable.run();
}
}
Why it Matters
Failing to handle checked exceptions properly can lead to runtime exceptions if you forget to include the try-catch block.
Solution
Always manage checked exceptions within the lambda body or use custom functional interfaces that allow checked exceptions.
@FunctionalInterface
public interface CheckedRunnable {
void run() throws Exception;
static void run(CheckedRunnable runnable) {
try {
runnable.run();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
// Usage
CheckedRunnable myRunnable = () -> Files.readAllBytes(Paths.get("nonexistentfile.txt"));
CheckedRunnable.run(myRunnable);
4. Non-Static Context Issues
When you declare lambda expressions in static contexts, they may not have access to instance variables unless they are effectively final or are accessed via the enclosing class reference.
public class StaticContextExample {
private String instanceVariable = "Access Me!";
public static void main(String[] args) {
StaticContextExample example = new StaticContextExample();
Runnable runnable = () -> System.out.println(example.instanceVariable); // This is OK, as example is effectively final
runnable.run();
}
}
Why it Matters
If you try to reference non-static variables from a static context without proper qualification, you'll receive compilation errors.
Solution
Maintain good coding practices by accessing instance variables through an instance reference, or keep your non-static and static methods and fields separate.
5. Parallel Stream Pitfalls
When using parallel streams with lambda expressions, you might face thread-safety issues. Each thread might operate on shared mutable data, leading to unpredictable results.
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Potential data corruption if modifying a shared list in parallel
numbers.parallelStream().forEach(n -> {
// This might lead to unexpected behavior
// sharedList.add(n);
});
}
}
Why it Matters
Using non-thread-safe collections or mutable shared states in a parallel stream can lead to concurrent modification exceptions or incorrect data states.
Solution
When working with parallel streams, prefer using thread-safe collections like ConcurrentHashMap
, or better yet, avoid mutable shared state.
import java.util.concurrent.CopyOnWriteArrayList;
List<Integer> sharedList = new CopyOnWriteArrayList<>();
numbers.parallelStream().forEach(n -> sharedList.add(n));
A Final Look
Java’s lambda expressions offer powerful functionalities that can enhance code readability and maintainability. However, being aware of their common pitfalls helps avoid frustrating errors that can hinder development. By keeping the intricacies of the lambda syntax and functional interfaces in mind, you can leverage Java's capabilities to write cleaner, more effective code.
Should you want to read more about lambda expressions and functional programming in Java, check out the Oracle Java Documentation or dive into Baeldung's Lambda in Java for detailed tutorials.
By mastering lambda expressions and their potential errors, you'll help your team and your projects thrive.
Happy coding! For any questions or further clarifications on specific lambda issues or Java programming in general, feel free to leave a comment.