Mastering Navigation Drawers: Common Pitfalls in Android Apps
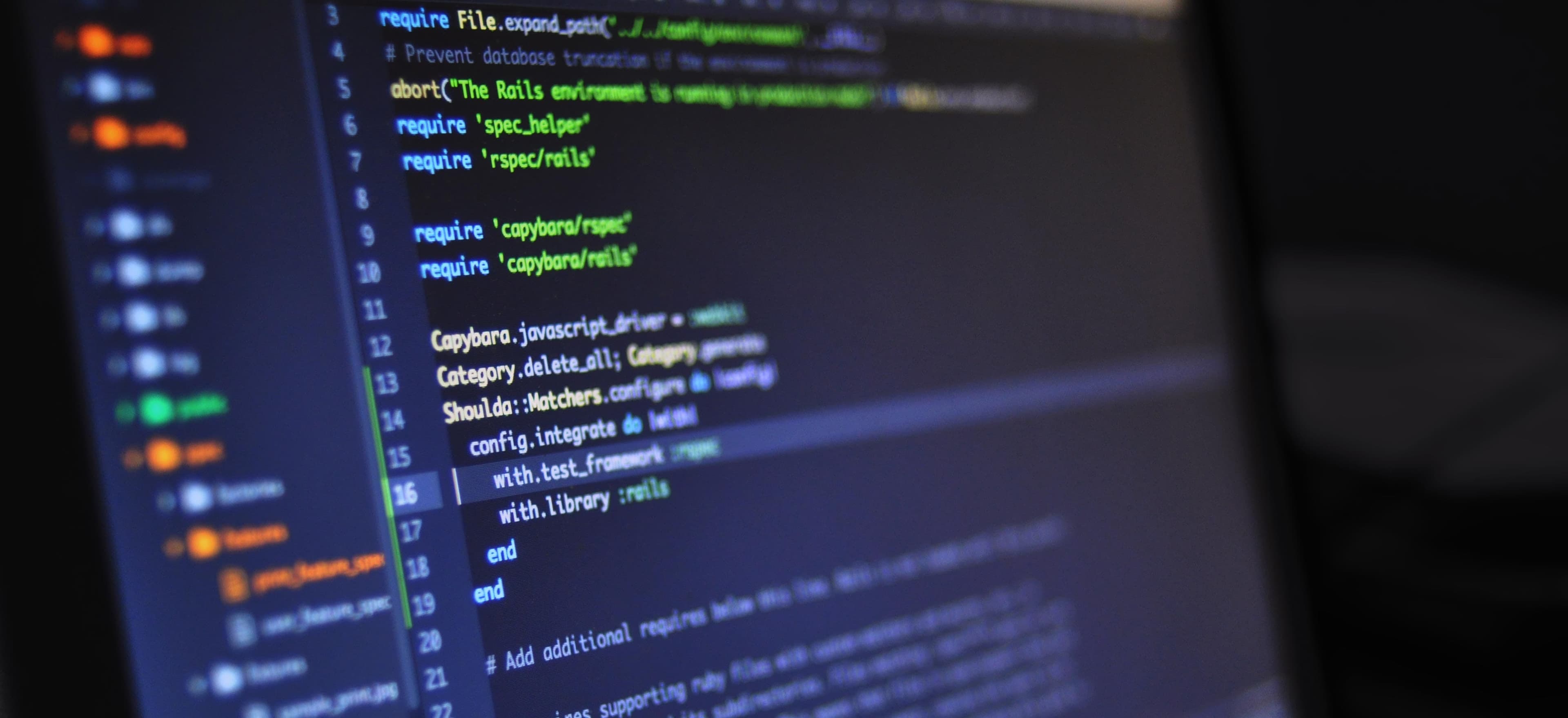
- Published on
Mastering Navigation Drawers: Common Pitfalls in Android Apps
Navigation drawers are a crucial component of Android app design, allowing users to access various sections of an app smoothly. However, mastering navigation drawers comes with its own set of challenges. In this blog post, we'll delve into common pitfalls developers encounter when implementing navigation drawers in Android apps and how to avoid them.
Table of Contents
- What is a Navigation Drawer?
- Common Pitfalls in Navigation Drawers
- Overcomplicated Navigation
- Poor Integration with Fragments
- Ignoring Accessibility
- Lack of Testing on Different Devices
- Inconsistent UI Design
- Best Practices for Navigation Drawers
- Example Implementation
- Conclusion
What is a Navigation Drawer?
A navigation drawer is a sliding panel that allows users to navigate through different sections of an application. Typically, the drawer can be pulled from the left edge, revealing options like different activities, profiles, settings, and more. The benefits of using a navigation drawer include improved organization, enhanced user experience, and a clean interface.
For more comprehensive details about navigation components, visit the official Android documentation.
Common Pitfalls in Navigation Drawers
1. Overcomplicated Navigation
Explanation: One of the first pitfalls developers encounter is creating an overly complicated navigation structure. Users can quickly feel overwhelmed by too many options or nested screens.
Solution: Keep your navigation structure simple. Limit the main items displayed in the drawer to 5 - 7 key sections. Use submenus or secondary navigation components for additional options.
Example: Consider this navigation drawer structure:
<!-- navigation_header.xml -->
<android.widget.ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@drawable/header_image" />
<androidx.drawerlayout.widget.DrawerLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- Main Application Layout -->
</RelativeLayout>
<navigation:NavigationView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
app:headerLayout="@layout/navigation_header"
app:menu="@menu/drawer_menu" />
</androidx.drawerlayout.widget.DrawerLayout>
This example establishes a basic structure for a navigation drawer. Ensure that your menu is not cluttered.
2. Poor Integration with Fragments
Explanation: Navigation drawers often integrate with fragments to change content dynamically without recreating the activity. Many developers fail to manage the fragment lifecycle properly, causing UI issues or crashes.
Solution: Always replace your fragments correctly when a menu item is selected. Use the FragmentManager
to manage fragment transactions safely.
Example: Here’s a code snippet to demonstrate fragment transactions:
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
Fragment selectedFragment = null;
switch (item.getItemId()) {
case R.id.nav_home:
selectedFragment = new HomeFragment();
break;
case R.id.nav_profile:
selectedFragment = new ProfileFragment();
break;
// Include other cases as needed
}
if (selectedFragment != null) {
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, selectedFragment);
transaction.commit();
}
drawerLayout.closeDrawer(GravityCompat.START);
return true;
}
By using FragmentTransaction
, seamless transitions occur when changing fragments.
3. Ignoring Accessibility
Explanation: Accessibility is often an afterthought in app design. Ignoring it can alienate users with disabilities.
Solution: Ensure your navigation drawer and its items are accessible. Use the appropriate attributes to describe UI elements for screen readers.
Example:
<androidx.drawerlayout.widget.DrawerLayout
...
android:contentDescription="@string/navigation_drawer">
Using contentDescription
enhances accessibility, ensuring that screen readers can describe the elements effectively.
4. Lack of Testing on Different Devices
Explanation: Many developers test their navigation drawers only on a few devices, which can lead to usability issues on other screen sizes or orientations.
Solution: Always test your UI on various devices and orientations to ensure usability. Use Android's emulators or test services to assess performance across different configurations.
5. Inconsistent UI Design
Explanation: Inconsistent design between the navigation drawer and the rest of the application can confuse users.
Solution: Adhere to Material Design principles to maintain consistency. Use similar font styles, colors, and padding throughout the app.
Best Practices for Navigation Drawers
- Use Icons Wisely: Icons can convey meaning and enhance user experience. Make sure they are recognizable and align with the text.
- Highlight the Current Item: Use selected states to inform the user about their location within the app.
- Animation and Feedback: Utilize subtle animations when opening and closing the drawer, and provide feedback for item selections.
- Stay Updated: Always check for the latest best practices from the Android Developers Blog and Material Design guidelines.
Example Implementation
To tie everything together, here’s a full implementation of a navigation drawer with a simplified menu:
public class MainActivity extends AppCompatActivity {
DrawerLayout drawerLayout;
NavigationView navigationView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
drawerLayout = findViewById(R.id.drawer_layout);
navigationView = findViewById(R.id.nav_view);
navigationView.setNavigationItemSelectedListener(this::onNavigationItemSelected);
}
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
Fragment selectedFragment = null;
switch (item.getItemId()) {
case R.id.nav_home:
selectedFragment = new HomeFragment();
break;
case R.id.nav_about:
selectedFragment = new AboutFragment();
break;
}
if (selectedFragment != null) {
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, selectedFragment);
transaction.commit();
}
drawerLayout.closeDrawer(GravityCompat.START);
return true;
}
}
In this code, we set up a simple navigation drawer that handles fragment transactions effectively.
The Last Word
Navigating the intricacies of navigation drawers in Android development is essential for creating user-friendly applications. By avoiding common pitfalls and adhering to best practices, you can ensure your navigation drawers not only function effectively but also enhance the overall user experience.
Feel free to explore additional resources such as Google's Material Design Documentation to continue your learning about navigation drawers. Happy coding!
This blog post aims to provide insightful discussions interspersed with practical code examples that demonstrate effective navigation drawer implementation in Android apps. For further discussions or questions, feel free to comment below!
Checkout our other articles