Overcoming Delays in Testing with Hoverfly and Java
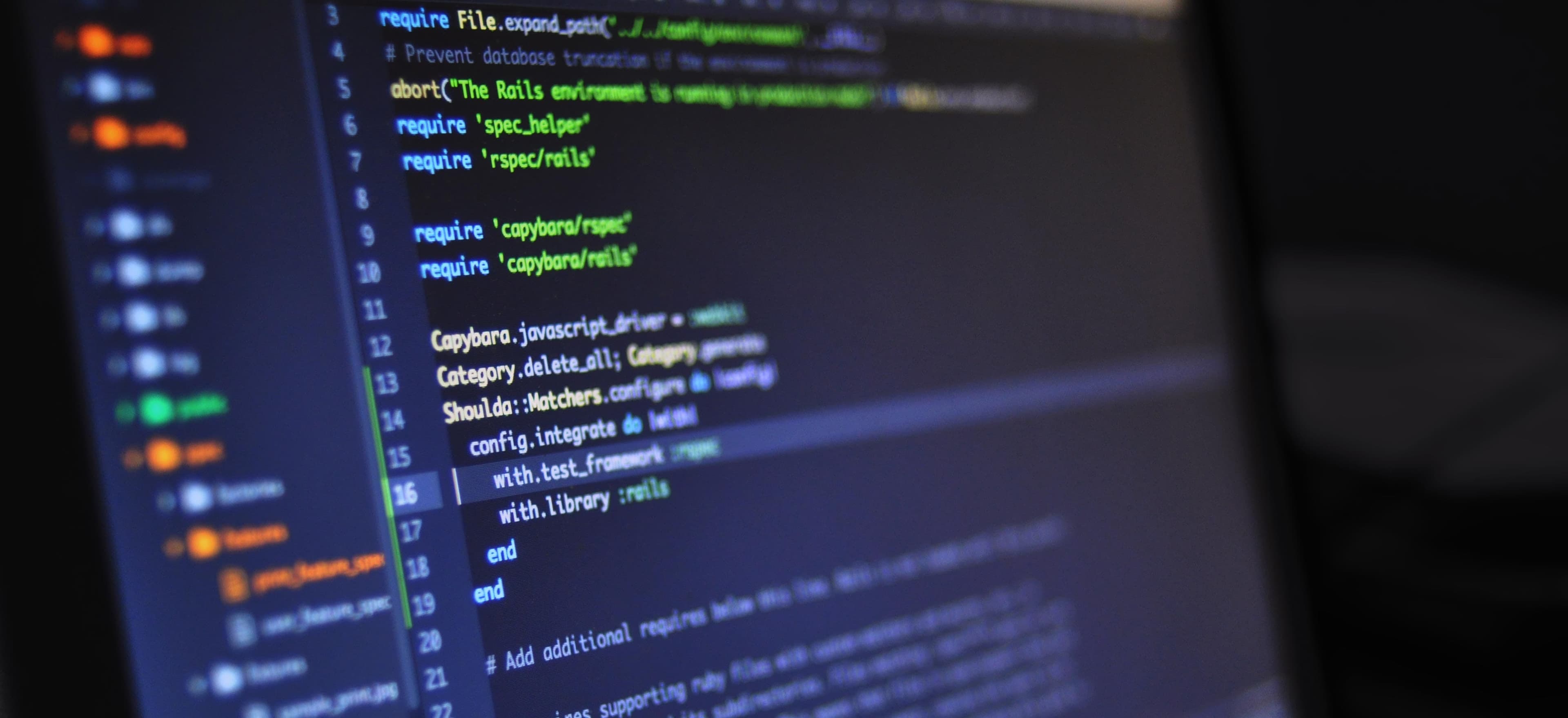
- Published on
Overcoming Delays in Testing with Hoverfly and Java
In today's fast-paced software development world, continuous integration and testing play a crucial role in delivering high-quality applications. Yet, as developers, we often run into delays during testing caused by external dependencies—be it APIs or microservices. This is where Hoverfly comes into play, providing an excellent solution for simulating APIs during testing. In this blog post, we will explore how to overcome delays in testing using Hoverfly and Java, ensuring a streamlined development process.
What is Hoverfly?
Hoverfly is an open-source tool designed for API simulation and service virtualization. It enables developers to create lightweight, performant environments for testing by simulating the behavior of external services. This is highly significant when you're working with applications that depend on various third-party APIs. By using Hoverfly, you can mimic API responses without actually hitting the real endpoints, thus eliminating delays in testing.
Key Benefits of Using Hoverfly
- Speed: By removing the need to wait for external services, testing can be performed much faster.
- Isolation: Tests are run in isolation from real services, allowing for more consistent and reliable outcomes.
- Cost-Effective: Reduces the need for extensive test environments, leading to savings in server costs.
- Reproducibility: Easily replicate test scenarios that can be hard to reproduce when working with real APIs.
Setting Up Hoverfly
To use Hoverfly effectively with Java, you first need to install it. Here's a quick guide:
-
Download Hoverfly: You can download the application from Hoverfly's official GitHub repository.
-
Run Hoverfly: Once you have downloaded Hoverfly, you can run it via command-line interface (CLI) using:
hoverfly -webserver
This launches the Hoverfly web server, ready to intercept requests.
-
Add Hoverfly to Your Java Project: If you're using Maven, add the dependency in your
pom.xml
:<dependency> <groupId>io.specto.hoverfly</groupId> <artifactId>hoverfly-java</artifactId> <version>0.14.0</version> <scope>test</scope> </dependency>
Using Hoverfly with Java
Now that we have Hoverfly set up, let’s get into how to use it in our Java tests. The steps involve starting the Hoverfly server, specifying the requests and responses, and then writing tests to verify the behavior.
Basic Example of Using Hoverfly
Here's an example illustrating how to use Hoverfly in your Java tests:
import io.specto.hoverfly.junit.HoverflyRule;
import org.junit.Rule;
import org.junit.Test;
import org.springframework.web.client.RestTemplate;
import static io.specto.hoverfly.junit.core.HoverflyConfig.sampleSource;
import static io.specto.hoverfly.junit.core.HoverflyConfig.proxyAll;
import static io.specto.hoverfly.junit.core.SimulationSource.*;
public class ApiServiceTest {
@Rule
public HoverflyRule hoverfly = HoverflyRule.inCaptureOrSimulationMode("simulations.json");
private final RestTemplate restTemplate = new RestTemplate();
@Test
public void testApiWithHoverfly() {
String url = "http://example.com/api/resource";
String response = restTemplate.getForObject(url, String.class);
// Assert the response is what we expect
assertEquals("Mocked response", response);
}
}
Code Explanation
- Dependencies: We include Hoverfly as a JUnit rule, allowing us to define which simulation to use—here,
simulations.json
. - RestTemplate: This is the Spring template for making HTTP calls. In our test, we will call a mocked API.
- Test Method: It fetches a mocked response from the URL defined in Hoverfly, allowing us to validate against it.
- Assertions: We can assert that the response matches our expectations.
Creating Simulation Files
To effectively simulate API interactions, you need to create simulation files. Here's an example of what simulations.json
can look like:
{
"data": {
"pairs": [
{
"request": {
"method": "GET",
"path": "/api/resource"
},
"response": {
"status": 200,
"body": "Mocked response",
"headers": {
"Content-Type": "application/json"
}
}
}
]
}
}
Why Use Simulation Files?
- Flexibility: Easily switch between different scenarios in your tests.
- Version Control: Keep track of API behavior over time.
- Collaborative Development: Share simulation files with your team for consistent testing.
Expanding Test Scenarios with Hoverfly
The example shown earlier provides a basic overview. However, you can use Hoverfly to simulate more complex interactions, including errors or delays. This is essential when you want to test how your application behaves under different conditions without relying on real APIs.
Simulating API Errors
To simulate an HTTP error, add an entry to your simulations.json
:
{
"data": {
"pairs": [
{
...
},
{
"request": {
"method": "GET",
"path": "/api/error"
},
"response": {
"status": 500,
"body": "Internal Server Error",
"headers": {
"Content-Type": "application/json"
}
}
}
]
}
}
Then you can conduct tests to confirm your application handles errors gracefully.
Handling Response Delays
If you want to simulate delays in responses:
{
"data": {
"pairs": [
{
...
},
{
"request": {
"method": "GET",
"path": "/api/delayed"
},
"response": {
"status": 200,
"body": "Delayed response",
"delay": {
"duration": 2000,
"timeUnit": "MILLISECONDS"
}
}
}
]
}
}
By introducing delays, you can effectively test the resilience of your application's logic.
The Last Word: The Value of Hoverfly in Testing
As we have explored, utilizing Hoverfly with Java can significantly improve the speed and reliability of your testing practices. By escaping the confines of real external dependencies, developers can embrace a more efficient approach to software testing.
Using Hoverfly not only saves time but also enhances your team’s ability to introduce code changes swiftly, making your development cycle robust and responsive to change.
For more complex scenarios and deeper insights on testing, dive into the official Hoverfly documentation and discover advanced functionalities that suit your project's needs.
With Hoverfly’s capabilities, the days of sitting idle while waiting on API calls are over. Embrace this tool, and elevate your testing process – ensuring your applications are as dependable as they are innovative.