Mastering Layout Managers: Common Pitfalls in Android Dev
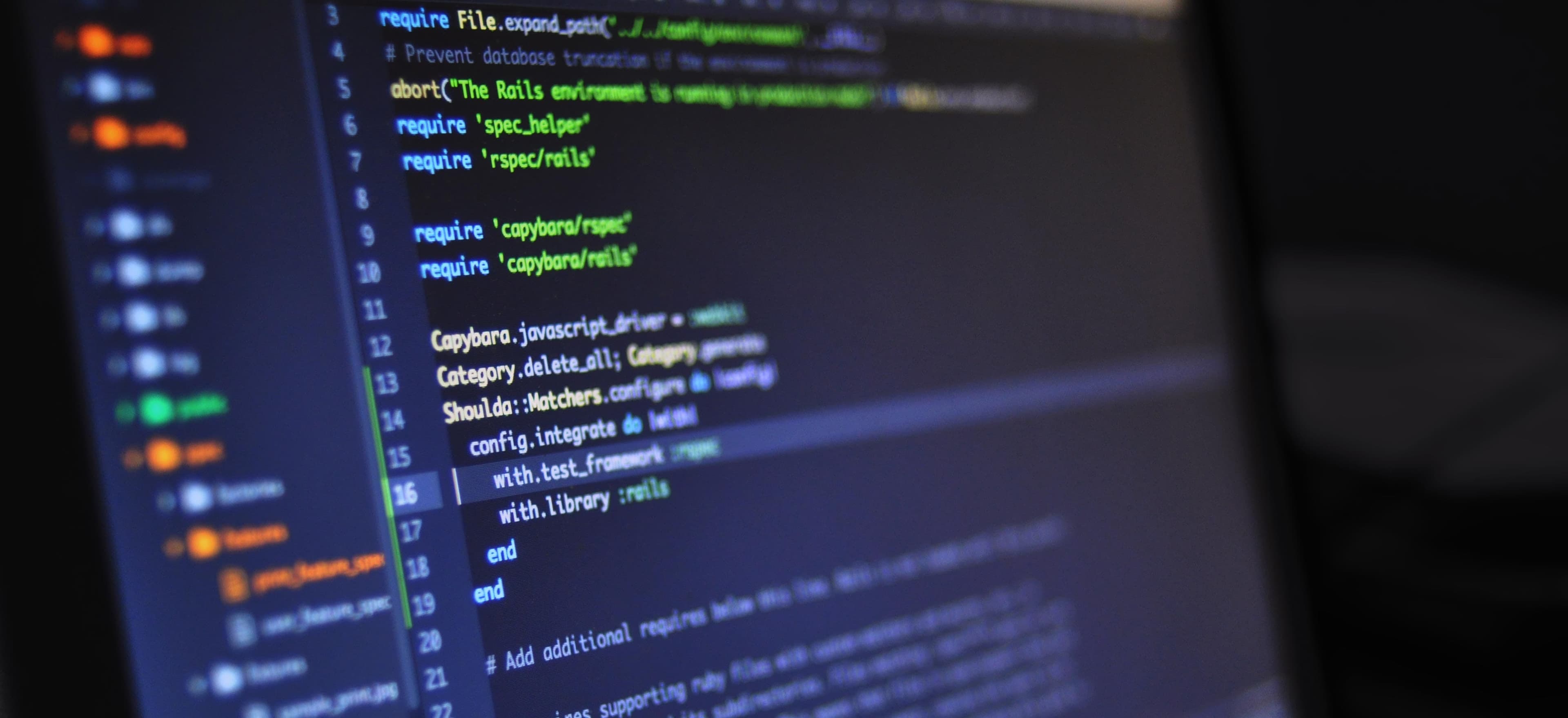
- Published on
Mastering Layout Managers: Common Pitfalls in Android Development
When developing Android applications, proper layout management is crucial for creating a seamless user experience. Layout managers control how your UI components are organized in the available screen space. Yet, many developers encounter common pitfalls when working with them. In this post, we'll explore these pitfalls in detail, review best practices, and highlight effective solutions.
Understanding Layout Managers
In Android, layout managers are responsible for arranging UI components (Views) within their parent containers (Layouts). Each layout manager is designed for specific use cases:
- LinearLayout: Arranges its children either vertically or horizontally.
- RelativeLayout: Positions children in relation to each other or the parent.
- ConstraintLayout: Offers fine-grained control over positioning and is efficient in optimizing the UI.
- FrameLayout: Stacks all children on top of each other.
- GridLayout: Organizes children in a grid format.
Choosing the appropriate layout manager is essential for optimal performance and usability. However, many developers encounter pitfalls that can hinder their development process. Let’s dive into common issues and how to avoid them.
Common Pitfalls
1. Overusing Nested Layouts
One of the frequent pitfalls is excessive nesting of layout managers, which not only complicates the layout hierarchy but can also severely affect performance.
Why is it a problem?
Nesting layouts increases the view hierarchy depth, resulting in longer rendering times and decreased overall application performance. To illustrate, consider this nested structure:
<LinearLayout>
<LinearLayout>
<TextView />
<ImageView />
</LinearLayout>
<Button />
</LinearLayout>
Solution: Utilize ConstraintLayout
to flatten the hierarchy. For example:
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/myTextView"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
<ImageView
android:id="@+id/myImageView"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintTop_toBottomOf="@id/myTextView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toTopOf="@id/myButton"/>
<Button
android:id="@+id/myButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
This layout structure improves performance by reducing the number of nested views.
2. Ignoring Layout Parameters
Developers often forget to define important layout parameters, which can lead to an unpredictable UI.
Why is it critical?
Ignoring these parameters may result in unexpected behavior due to default settings being applied. For instance, failing to set layout_width
to wrap_content
or match_parent
leads to poorly sized views.
Example:
<TextView
android:layout_width="200dp" // May not be the best size
android:layout_height="match_parent" />
Solution: Always specify layout parameters based on your design requirements. An optimal approach to the above would be:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:padding="16dp"/>
This ensures the TextView is appropriately sized based on its content.
3. Not Testing on Different Screen Sizes
Failing to test your layouts on various screen sizes can lead to usability issues.
Why is it a concern?
Screens come in different sizes and resolutions, and a layout that works well on one device may be completely broken on another.
Solution: Utilize Android’s built-in tools such as the layout inspector and emulator with various configurations to ensure that your UI holds up across different scenarios.
Additionally, consider using resource qualifiers such as layout-sw600dp
for tablets. This enables you to provide specific layouts optimized for larger screens.
4. Misusing Weight in LinearLayout
Many developers misuse the layout_weight
attribute, leading to uneven or unintended spacing.
Why does this happen?
Weight is used to distribute space between views in a LinearLayout, but miscalculating weights can disrupt the intended layout.
Example:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
Solution: Ensure the sum of weights is deliberate. If you want one TextView
to take twice the space of another, clarify that in your attributes. Additionally, consider other layout options where weight isn’t needed, like using ConstraintLayout
.
5. Ignoring Accessibility Features
Accessibility is often overlooked while creating layouts.
Why is it important?
Not considering accessibility can alienate users with disabilities, making the application cumbersome or impossible to use.
Solution: Always provide content descriptions for UI elements and ensure that your layout is navigable with assistive technologies like screen readers. An example of adding accessibility features:
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:contentDescription="@string/button_description"/>
Bringing It All Together
Mastering layout managers is essential for any Android developer. Awareness of common pitfalls can help streamline the development process and enhance user experience. By adopting best practices—such as minimizing nesting, using layout parameters wisely, conducting thorough testing across devices, managing weights effectively in layouts, and ensuring accessibility—you can build robust and user-friendly applications.
Further Reading
For more insights into Android layouts, consider the following resources:
- Android Developer Documentation on Layouts
- Building a Responsive UI with ConstraintLayout
- Android Accessibility Guidelines
By following these guidelines, developers can avoid pitfalls and create exceptional user interfaces that perform well across various devices. Happy coding!