Battling Out-of-Memory: How to Prevent Process Kill Dilemmas
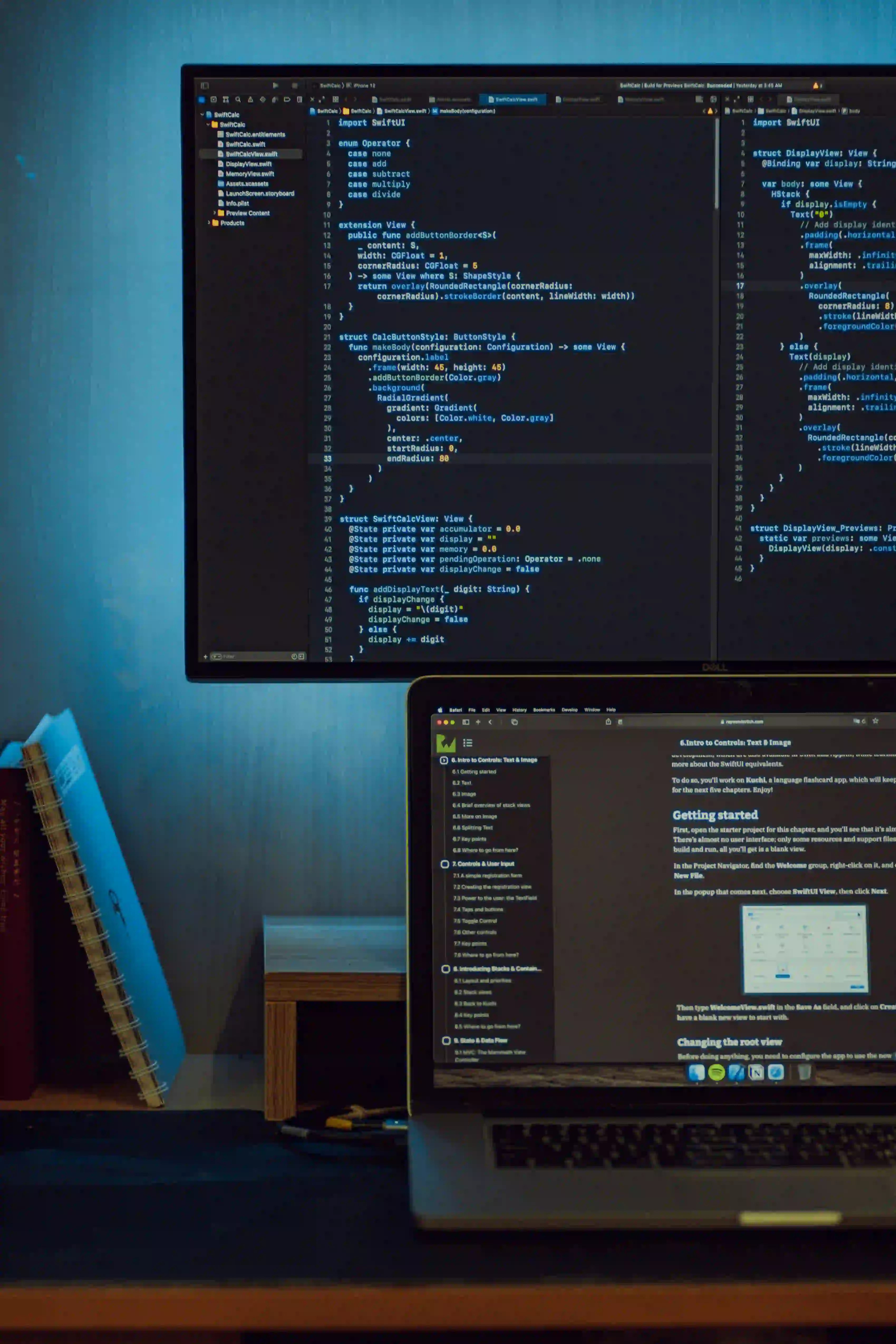
Battling Out-of-Memory: How to Prevent Process Kill Dilemmas in Java
In the world of Java development, running into out-of-memory errors can feel like a fatal blow to your application. These errors manifest when the Java Virtual Machine (JVM) fails to allocate an object because it has run out of memory, often leading to the termination of the process. In this blog post, we will explore the causes, prevention mechanisms, and best practices for handling out-of-memory situations in your Java applications. Let’s dive in!
Understanding Out-of-Memory Errors
Before we jump into solutions, it’s crucial to understand what out-of-memory errors are and how they occur. Java applications run on the JVM, which manages memory allocation and deallocation. There are primarily two types of out-of-memory errors you might encounter:
- Java Heap Space: This error occurs when the program tries to allocate an object in the heap, but there's no space available.
- GC Overhead Limit Exceeded: This indicates that the garbage collector is spending too much time trying to free up memory but is only recovering a minimal amount.
Common Causes of Out-of-Memory Errors
Out-of-memory issues can arise due to various scenarios. Here are a few common culprits:
- Memory Leaks: When objects are no longer in use but still referenced, they consume memory unnecessarily.
- Large Data Processing: Handling large datasets can consume more heap space than allocated, overwhelming the system.
- High-Concurrency Applications: In multi-threaded applications, many threads can create a spike in memory usage.
How to Prevent Out-of-Memory Errors
1. Optimize Memory Usage
Optimizing memory usage is essential for preventing out-of-memory errors. This begins with analyzing your code and identifying objects that can be freed or cleaned up early.
Here’s a simple code snippet demonstrating how to utilize weak references:
import java.lang.ref.WeakReference;
class LargeObject {
// Represents a large object
// ...
}
// Weak references do not prevent their referents from being made available for garbage collection
WeakReference<LargeObject> weakRef = new WeakReference<>(new LargeObject());
if (weakRef.get() != null) {
// Use the object
} else {
// The object has been collected; recreate or handle null case
}
Why Use Weak References?
Using weak references can help manage memory consumption by allowing the garbage collector (GC) to reclaim memory when application memory becomes constrained. This is particularly useful with cache implementations or similar scenarios.
2. Increase Heap Size
If your application continuously runs out of memory under normal circumstances, consider increasing the heap size of the JVM. You can specify the heap size using the -Xms
(initial size) and -Xmx
(maximum size) parameters.
java -Xms512m -Xmx2g -jar YourApp.jar
Why Adjust Heap Size?
By adjusting the heap size, you provide your application with more memory to work with. However, this is only a stopgap measure and should not be a substitute for proper memory management.
3. Use Profilers
Profilers are invaluable tools for identifying memory usage patterns and locating memory leaks in your applications. Tools like VisualVM or YourKit allow you to monitor the memory behavior of your Java applications in real-time.
How to Profile Memory Usage
Here's how to use VisualVM:
- Download and install VisualVM, which comes with the JDK.
- Launch it and connect it to your running Java application.
- Navigate to the 'Memory' tab and observe the allocation patterns.
4. Implement Caching Wisely
Caching is a common strategy to improve performance, but excessive caching can lead to increased memory usage. It’s essential to strike a balance.
import java.util.LinkedHashMap;
import java.util.Map;
class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
}
Explanation of the Code
In this Example, an LRU (Least Recently Used) Cache is implemented. By automatically removing the least recently accessed entries in the cache, you maintain controlled memory usage.
5. Handle Exceptions Gracefully
Ensure your application can handle OutOfMemoryError
gracefully. Consider wrapping your critical memory-using code with try-catch blocks to cleanly handle resource management.
try {
// Code that could throw OutOfMemoryError
} catch (OutOfMemoryError e) {
System.err.println("Memory limit exceeded! Cleansing resources.");
// Perform cleanup or fallback
}
Monitoring and Diagnosing Memory Issues
Continuous monitoring of your Java application is crucial in preemptively preventing out-of-memory errors. Tools like Java Mission Control can assist in this endeavor, offering insights into memory usage, GC activity, and application performance.
JVM GC Logs
You can enable garbage collection logs to get insights into memory usage. This can be done using the following command-line arguments:
-XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log
Use these logs to analyze GC pauses and identify trends in memory usage over time.
6. Choose the Right Data Structures
Understanding and choosing the right data structures can dramatically affect memory usage. For example, using ArrayList
may be less memory-efficient if you frequently add or remove elements compared to LinkedList
.
Also, consider if some data structures can be replaced with more memory-efficient alternatives.
To Wrap Things Up
Out-of-memory errors can pose significant challenges in Java development, but with careful planning, resource management, and monitoring, you can prevent such dilemmas. From optimizing memory usage and increasing heap size to leveraging profilers and implementing caching wisely, each technique offers a viable solution.
Always remember, the goal is to maintain a healthy balance between application performance and efficient memory usage. Developing with an edge of foresight can provide your Java applications with the robustness they need to thrive in today's competitive landscape.
For more in-depth reading, check out Java Performance Tuning and Effective Java.
Implement these strategies to keep your Java applications running smoothly and avoid the dreaded out-of-memory errors!
By following this comprehensive strategy, you can ensure that your Java applications remain stable, efficient, and scalable. Happy coding!