Mastering Apache Commons CLI: Common Pitfalls to Avoid
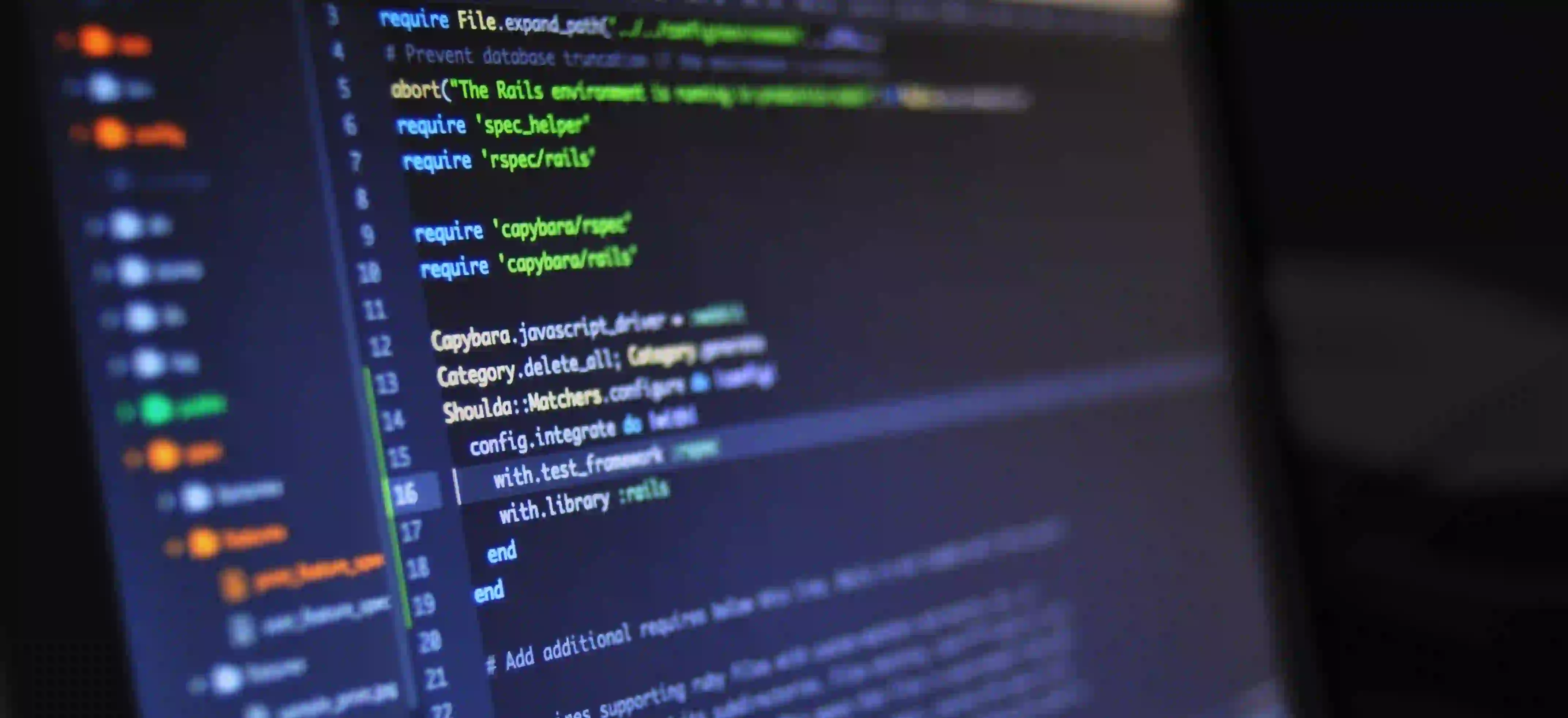
Mastering Apache Commons CLI: Common Pitfalls to Avoid
When it comes to building command-line interfaces (CLIs) in Java applications, the Apache Commons CLI library stands out as a robust and user-friendly tool. However, even experienced developers can run into pitfalls while integrating CLI into their applications. This guide aims to highlight common mistakes developers make when using Apache Commons CLI and how to avoid them, ensuring a smooth command-line experience.
Table of Contents
- Introduction to Apache Commons CLI
- Setting Up Apache Commons CLI
- Common Pitfalls to Avoid
- Misconfigured Options
- Ignoring Help Message
- Failure to Validate Inputs
- Overlooking Exception Handling
- Ignoring Default Values
- Sample Code Implementation
- Conclusion
Getting Started to Apache Commons CLI
Apache Commons CLI is a library that simplifies parsing command-line arguments in Java applications. The library provides a standard mechanism for defining and processing options that users can specify when launching your Java application. You can learn more about Apache Commons CLI on its official website.
Command-line interfaces enhance usability, enabling scripts and applications to run efficiently with various parameters. However, mastering its usage is paramount to avoiding common issues.
Setting Up Apache Commons CLI
Before delving into pitfalls, let's get started with the setup process. Ensure you include the Commons CLI library in your project's dependencies. If you are using Maven, the following dependency should be added to your pom.xml
:
<dependency>
<groupId>commons-cli</groupId>
<artifactId>commons-cli</artifactId>
<version>1.4</version>
</dependency>
Once set up, you can begin implementing command-line options in your application.
Common Pitfalls to Avoid
1. Misconfigured Options
One of the most common mistakes is misconfiguring the command-line options. Developers might forget to specify whether an option is required or not.
Example:
import org.apache.commons.cli.*;
public class CommandLineExample {
public static void main(String[] args) {
Options options = new Options();
options.addOption("f", "file", true, "Input file path");
options.addOption("o", "output", true, "Output file path");
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
} catch (ParseException e) {
System.out.println("Failed to parse command line arguments. Error: " + e.getMessage());
}
}
}
Why is this important? Failing to configure options properly may lead to unexpected behaviors. If an option is crucial for your application, you should always define it as required.
2. Ignoring Help Messages
Often, developers overlook the importance of providing a help message for users. A well-crafted help message gives users clarity on how to use your application.
How to Implement Help Message:
public static void main(String[] args) {
Options options = new Options();
options.addOption("h", "help", false, "Show help message");
// Other options...
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
if (cmd.hasOption("h")) {
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("CommandLineExample", options);
return;
}
} catch (ParseException e) {
// Handle exception
}
}
Why is this important? Users appreciate guidance. Help messages can significantly reduce user friction and misunderstandings related to your application.
3. Failure to Validate Inputs
Another frequent oversight is failing to validate the user inputs effectively. Accepting inputs without sanitation can lead to runtime errors or undesired behavior.
Incorporation of Validation:
if (cmd.hasOption("f")) {
String filePath = cmd.getOptionValue("f");
if (!isValidFilePath(filePath)) {
throw new IllegalArgumentException("Invalid file path provided.");
}
}
Why is this important? Validating inputs before processing ensures that your application behaves reliably, providing users with immediate feedback if something is amiss.
4. Overlooking Exception Handling
Exception handling is critical when parsing command-line arguments. Developers sometimes neglect to manage exceptions appropriately, which can lead to crashes or unmanageable error states.
Example of Exception Handling:
try {
CommandLine cmd = parser.parse(options, args);
} catch (ParseException e) {
System.err.println("Error: " + e.getMessage());
System.err.println("Use -h or --help for usage information.");
System.exit(1);
}
Why is this important? Graceful error handling is essential. It helps users understand what went wrong and provides options to rectify their input easily.
5. Ignoring Default Values
Setting default values for options is a practical approach many developers often overlook. It allows your application to run smoothly even if certain parameters are not specified by the user.
How to Implement Default Values:
options.addOption(Option.builder("v")
.longOpt("verbose")
.hasArg()
.desc("Set verbosity level")
.build());
// Setting the default value
String verbosity = cmd.getOptionValue("v", "1"); // Default is 1
Why is this important? Default values enhance usability and reduce frustration for users. It ensures that your application can operate effectively without requiring exhaustive input.
Sample Code Implementation
Below is a complete Java example that incorporates all the tips mentioned above:
import org.apache.commons.cli.*;
public class CommandLineApp {
public static void main(String[] args) {
Options options = new Options();
// Adding options
options.addOption("f", "file", true, "Input file path (required)");
options.addOption("o", "output", true, "Output file path");
options.addOption("v", "verbose", true, "Set verbosity level (default = 1)");
options.addOption("h", "help", false, "Display help");
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
// Help option
if (cmd.hasOption("h")) {
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("CommandLineApp", options);
return;
}
// Validate inputs
if (cmd.hasOption("f")) {
String filePath = cmd.getOptionValue("f");
if (!isValidFilePath(filePath)) {
throw new IllegalArgumentException("Invalid file path provided.");
}
}
// Get verbosity (with default)
String verbosity = cmd.getOptionValue("v", "1");
System.out.println("Verbosity level: " + verbosity);
// Additional application logic...
} catch (ParseException e) {
System.err.println("Error: " + e.getMessage());
System.err.println("Use -h or --help for usage information.");
System.exit(1);
} catch (IllegalArgumentException e) {
System.err.println("Error: " + e.getMessage());
}
}
private static boolean isValidFilePath(String filePath) {
// Implement a basic file path validation logic
return filePath != null && !filePath.isEmpty();
}
}
In the code snippet above, we encapsulate all the discussed pitfalls, creating an effective command-line application with Apache Commons CLI.
My Closing Thoughts on the Matter
Mastering Apache Commons CLI can significantly enhance the usability of your Java applications, but avoiding common pitfalls is essential for a polished product. By ensuring proper configuration, including help messages, validating inputs, incorporating good exception handling, and leveraging default values, you can create a user-friendly command-line interface.
As always, when embarking on application development, reference the official Apache Commons CLI documentation for the most detailed and accurate information. Happy coding!