Struts 2 Query Grid: Overcoming Plugin Limitations
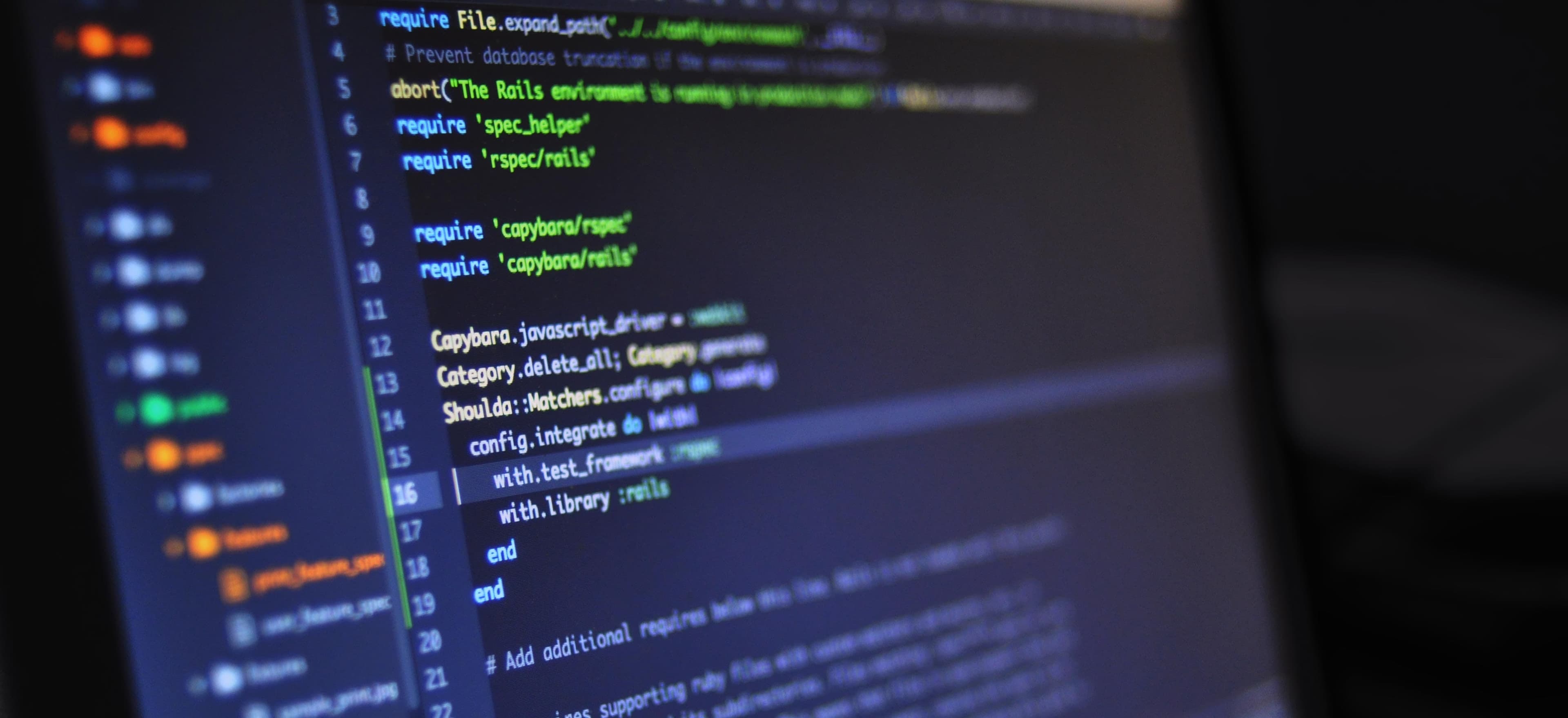
- Published on
Struts 2 Query Grid: Overcoming Plugin Limitations
Struts 2 is a popular framework for creating Java web applications, offering a robust feature set. However, working with various plugins occasionally presents challenges, particularly when handling complex data models. One of these challenges is the Query Grid plugin. This post aims to discuss the limitations of the Struts 2 Query Grid plugin and how to work around them effectively.
What is Struts 2?
Struts 2 is an evolution of the original Struts framework, designed to be simpler and more flexible. Based on the Model-View-Controller (MVC) architecture, it allows developers to create scalable and maintainable applications rapidly. The framework's ability to integrate with various Java libraries and technologies makes it a preferred choice for web applications.
Understanding Query Grid in Struts 2
The Query Grid plugin is designed to simplify data display and manipulation within Struts 2 applications. It provides a way to present tabular data, making pagination, sorting, and filtering easier. This plugin is particularly useful for displaying large datasets in a user-friendly manner.
Key Features of Query Grid Plugin
- Data Binding: Easily bind your data model with the grid.
- Pagination: Automatically paginates data to enhance display.
- Sorting and Filtering: Users can sort and filter their views effortlessly.
However, as with many plugins, the Query Grid has some limitations that developers should be aware of.
Common Limitations of Struts 2 Query Grid Plugin
1. Limited Customization Options
While the Query Grid provides a straightforward way to display data, its default styles and functionalities can be somewhat restrictive. Customizing the appearance or behavior often requires digging deep into the plugin's internals.
Solution: Utilize CSS styles and JavaScript to enhance the grid's functionality and appearance. Here's an example of adding custom CSS:
/* Custom Grid Style */
.grid-header {
background-color: #4CAF50;
color: white;
}
.grid-row:hover {
background-color: #f1f1f1;
}
2. Complex Data Structures
The Query Grid plugin works best with simple data structures. When dealing with complex nested objects or relationships, the integration can become cumbersome.
Solution: Flatten your data structure before passing it to the grid. Use a data transfer object (DTO) to encapsulate your data into a simpler format.
public class UserDTO {
private Long id;
private String username;
private String email;
// Getters and Setters
}
In this case, using DTOs allows the Query Grid plugin to display data without the complexity of the underlying data models.
3. Sorting and Filtering Limitations
While sorting and filtering capabilities are built into the Query Grid plugin, they can sometimes function incorrectly, especially with large datasets or specific data types.
Solution: Implement custom sorting or filtering logic in your backend. By processing data on the server-side, you can ensure accurate results irrespective of the grid limitations.
public List<User> getUsers(String sortField, String sortOrder) {
// Sort Users based on parameters
// Pseudo code - replace with actual implementation
return userDao.sortUsers(sortField, sortOrder);
}
Implementing a Custom Query Grid
Let's walk through an example of creating a simple Query Grid to display user data while actively overcoming these limitations.
Step 1: Set Up Your Struts 2 Application
Ensure you have a basic Struts 2 application set up. If you're starting from scratch, check out the official Struts 2 documentation for instructions.
Step 2: Create Your Action Class
Define an action class that will interact with your data source and provide data to the grid.
import com.opensymphony.xwork2.ActionSupport;
import java.util.List;
public class UserAction extends ActionSupport {
private List<UserDTO> userList;
// Service that retrieves users
private UserService userService;
public String execute() {
userList = userService.getAllUsers();
return SUCCESS;
}
public List<UserDTO> getUserList() {
return userList;
}
}
Here, we are using the UserService
to fetch all users and populate our DTO list.
Step 3: Configure Struts XML
In the struts.xml
, configure the action and result mapping for the Query Grid.
<action name="userAction" class="com.example.UserAction">
<result name="success">/userGrid.jsp</result>
</action>
Step 4: Create the JSP Page
Create userGrid.jsp
to display the Query Grid.
<%@ taglib prefix="s" uri="/struts-tags" %>
<html>
<head>
<title>User Grid</title>
<link rel="stylesheet" type="text/css" href="styles/grid.css"/>
</head>
<body>
<table>
<thead>
<tr>
<th>Username</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<s:iterator value="userList" var="user">
<tr>
<td><s:property value="#user.username"/></td>
<td><s:property value="#user.email"/></td>
</tr>
</s:iterator>
</tbody>
</table>
</body>
</html>
Step 5: Implement Custom Functions
Compile additional functions for sorting and filtering as needed.
public String sortUsers() {
// Custom sorting logic here
return SUCCESS;
}
The Closing Argument
Navigating the Struts 2 Query Grid plugin may pose challenges, particularly concerning customization, data complexity, and sorting capabilities. However, by adopting methods such as utilizing DTOs, applying custom styles, and implementing server-side logic, you can significantly improve functionality and user experience.
For more advanced strategies, explore topics like Java Persistence API (JPA) for data handling or JavaScript frameworks for better UI management.
Embrace the power of Struts 2 while learning from its limitations; with these strategies, you can effectively manage your data presentation layer and build sophisticated applications.
Happy coding!