Why Input Validation is Crucial for App Security
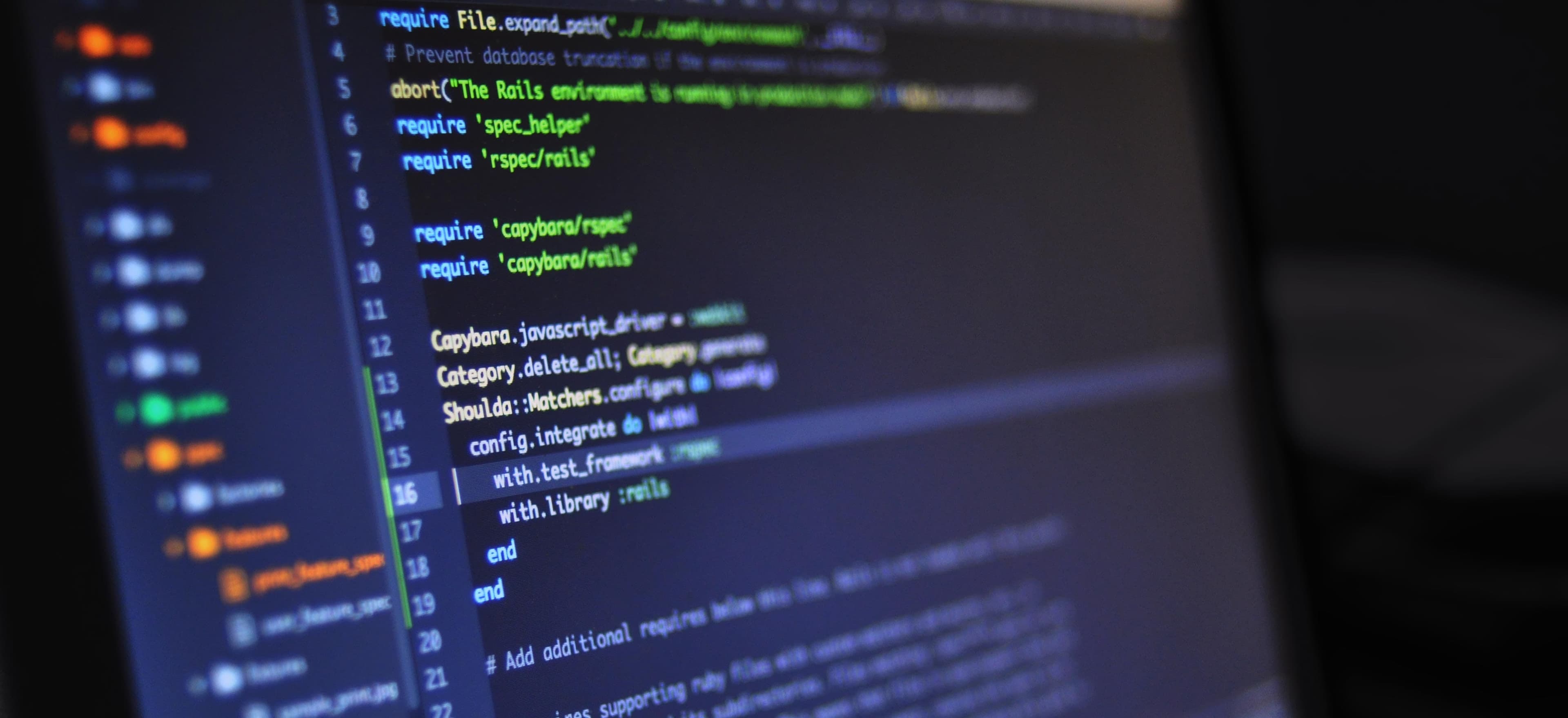
- Published on
Why Input Validation is Crucial for App Security
Input validation is a fundamental security practice in software development, particularly when it comes to building applications that handle user data. Not only does it ensure that the data being processed is appropriate and expected, but it also plays an integral role in protecting applications from various types of attacks, such as SQL injection and Cross-Site Scripting (XSS). In this blog post, we will discuss the importance of input validation, explore common validation techniques in Java, and provide code examples to illustrate the practical implications of robust input validation.
Understanding the Importance of Input Validation
In the digital age, applications are constantly under threat. A significant number of security breaches arise from the lack of proper input validation. Attackers often exploit this gap, injecting harmful data that can corrupt databases, steal sensitive information, or even take over entire systems.
Here are a few reasons why input validation is crucial for application security:
-
Data Integrity: Validating user input ensures that your application is only processing data that meets specific criteria. This helps maintain the integrity of your data and prevents erroneous or malicious entries from corrupting your database.
-
Minimizing Attack Surface: By implementing strict input validation, you reduce the number of potential vulnerabilities that attackers can exploit. Fewer vulnerabilities mean a more secure application.
-
User Trust: Users are more likely to trust an application that demonstrates a commitment to security through practices like input validation. This trust can positively impact user engagement and retention.
-
Compliance: Many industries have strict regulatory standards regarding data protection (e.g., GDPR, HIPAA). Input validation is often a requirement for compliance, helping organizations avoid legal repercussions.
Common Input Validation Techniques
When validating inputs in Java applications, it's important to adopt best practices to ensure security and robustness. Below are common techniques you can use:
1. Whitelisting vs. Blacklisting
Whitelisting involves defining a set of acceptable input values (e.g., allowing only specific characters or formats), while blacklisting identifies which inputs to reject. Whitelisting is generally the preferred approach for input validation.
Example of Whitelisting:
import java.util.regex.*;
public class InputValidator {
public static boolean isValidUsername(String username) {
// Username must be alphanumeric and 5-15 characters
String regex = "^[a-zA-Z0-9]{5,15}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(username);
return matcher.matches();
}
}
In this example, the isValidUsername
method uses a regex pattern to ensure the username contains only alphanumeric characters and is between 5 and 15 characters in length. By enforcing specific criteria, we eliminate most inputs that could lead to malicious behavior, such as SQL injection.
2. Length Checking
Limiting the length of input is another important validation technique. By only allowing inputs within a specific range, you can thwart certain attacks that exploit buffer overflow vulnerabilities.
Example of Length Checking:
public class InputValidator {
public static boolean isValidPassword(String password) {
// Password must be between 8 and 20 characters
if (password.length() >= 8 && password.length() <= 20) {
return true;
}
return false;
}
}
In this case, the password is validated based purely on its length, ensuring that users create strong passwords while also preventing excessively long inputs that could lead to security risks.
3. Type Validation
It's also essential to validate data types to ensure that the input matches the expected type. For instance, ensuring that numeric fields only accept numeric values.
Example of Numeric Validation:
public class InputValidator {
public static boolean isValidAge(String age) {
try {
int numericAge = Integer.parseInt(age);
return numericAge > 0 && numericAge <= 120; // Valid ages from 1 to 120
} catch (NumberFormatException e) {
return false; // Input was not a valid integer
}
}
}
In this example, we employ a try-catch block to handle non-numeric input gracefully, ensuring that only valid integers are processed. This technique also protects against type-related vulnerabilities.
4. Exception Handling
Handling exceptions properly is a crucial part of input validation. Whenever user inputs are processed, wrapping code in try-catch blocks can help mitigate risks and display user-friendly error messages.
Example of Exception Handling with Validation:
public class InputValidator {
public static boolean isValidEmail(String email) {
// Simple regex for validating email format
String regex = "^[A-Za-z0-9+_.-]+@(.+)$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(email);
if (!matcher.matches()) {
throw new IllegalArgumentException("Invalid email format");
}
return true;
}
}
In this case, if the email input does not match the required format, an exception is thrown. This not only helps in sanitizing input but also gives developers a clear understanding of what went wrong.
Implementing Input Validation in Java Applications
To effectively integrate input validation into a Java application, consider the following strategies:
Modular Validation Methods
Creating separate validation methods for different types of inputs adheres to the DRY (Don't Repeat Yourself) principle. This also makes the code more maintainable and easier to test.
Use of Frameworks
Java frameworks like Hibernate Validator and Apache Commons Validator can facilitate input validation significantly. These libraries provide built-in annotations and utilities that simplify the validation process. You can explore more here.
Regularly Update Validation Rules
As regulations and security threats evolve, so should your validation rules. Regularly review your application to ensure input validation remains robust and effective against new attack vectors.
Additional Best Practices
-
Keep User Feedback Clear: Provide clear feedback to users when their input fails validation. This enhances their experience and encourages them to enter correct data.
-
Test for Edge Cases: Always test your validation logic against various inputs, including edge cases, to ensure that your application does not unintentionally permit malicious data.
-
Limit Input Sources: Avoid using untrusted external sources for input. If you must, apply strict validation to any input stemming from those sources.
The Last Word
Input validation is not just a technical necessity; it's a fundamental practice in securing applications against various threats. By implementing robust input validation strategies, you can maintain data integrity, minimize security vulnerabilities, and foster user trust.
The repercussions of ignoring input validation can be catastrophic. From data breaches to compromised user experiences, the cost of overlooking this crucial aspect of application security can be monumental. Make input validation a priority in your development lifecycle.
For further reading on application security, consider checking out the OWASP Top Ten, which outlines the most critical web application security risks.
By putting strong input validation practices in place, you not only enhance security but also pave the way for creating a reliable and resilient application. Let's keep our applications safe, one validated input at a time.