Mastering Java 8 Lambdas: Common Pitfalls to Avoid
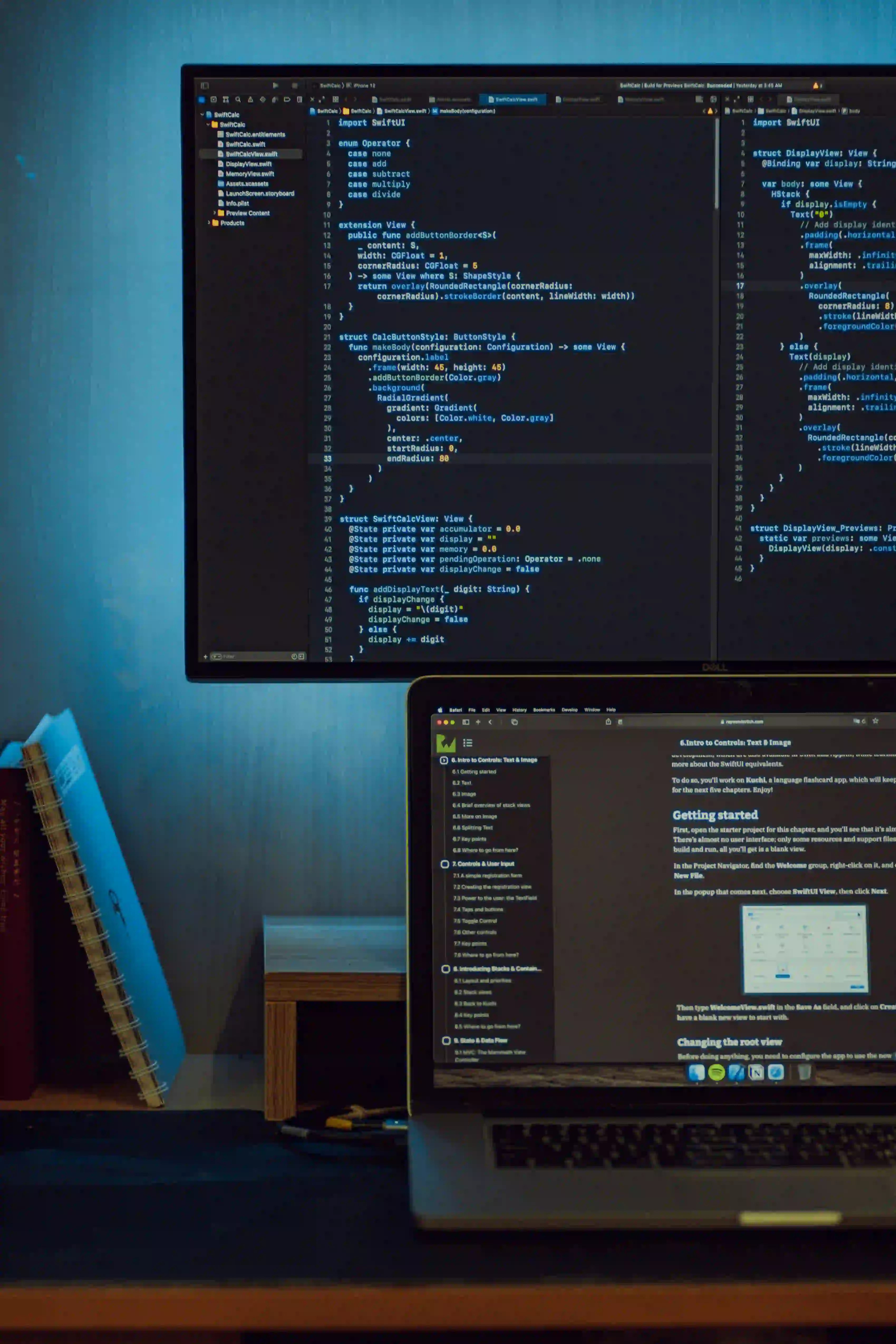
Mastering Java 8 Lambdas: Common Pitfalls to Avoid
Java 8 introduced a revolutionary way to write code by incorporating lambda expressions. These powerful constructs enable developers to express instances of single-method interfaces (functional interfaces) in a concise way. However, along with these conveniences come common pitfalls that can hinder productivity or lead to confusing code. In this blog post, we will explore the fundamentals of Java 8 lambdas, identify prevalent pitfalls, and provide actionable tips for writing clear and maintainable lambda expressions.
Understanding Lambda Expressions
At its core, a lambda expression is a concise way to represent an anonymous function. The syntax is straightforward:
(parameters) -> expression
Or for multiple statements:
(parameters) -> {
statement1;
statement2;
// more statements
}
Why Use Lambdas?
Lambdas improve code readability and reduce boilerplate by allowing inline implementation of functional interfaces. This leads to cleaner and more expressive code, especially in operations like sorting or filtering collections.
Here's an example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
// Using lambda to sort names
Collections.sort(names, (a, b) -> a.compareTo(b));
Common Pitfalls to Avoid
1. Misunderstanding Scope and Variable Shadowing
One of the common mistakes developers make is misunderstanding how scoping works with lambda expressions. When a lambda captures a variable from an enclosing scope, it must be effectively final. This means that you cannot reassign the variable after its creation.
Example:
String greeting = "Hello";
Runnable r = () -> System.out.println(greeting);
// This will compile but lead to an error if trying to reassign 'greeting' afterward
greeting = "Hi";
Tip: Use final or effectively final variables within lambdas to avoid unexpected behaviors.
2. Using this
Keyword Incorrectly
The this
keyword within a lambda refers to the enclosing instance, which might be confusing if you're coming from other programming paradigms.
Example:
class Outer {
private String name = "Outer";
void show() {
Runnable r = () -> System.out.println(this.name); // Refers to Outer class
r.run();
}
}
To access the lambda's enclosing context, use the enclosing class's scope explicitly when needed:
class Outer {
private String name = "Outer";
void show() {
Runnable r = () -> System.out.println(Outer.this.name); // Explicitly Outer
r.run();
}
}
3. Overusing Lambdas Instead of Method References
While lambdas can reduce boilerplate, it's crucial not to overuse them to the point of compromising readability. In some cases, simple method references can achieve what you need with higher clarity.
Example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
// Lambda syntax
names.forEach(name -> System.out.println(name));
// Method reference
names.forEach(System.out::println);
Tip: Always prefer method references when you can use them. They are less verbose and clearer, making code easier to understand.
4. Forgetting to Handle Exceptions
Lambdas inherently cannot throw checked exceptions unless they are declared in the functional interface's method signature. This can lead to runtime exceptions if not handled properly.
Example:
import java.util.Arrays;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> items = Arrays.asList("item1", "item2");
// This will fail if we don't handle exceptions
items.forEach(item -> {
try {
throwException(item);
} catch (Exception e) {
e.printStackTrace(); // Proper exception handling
}
});
}
public static void throwException(String item) throws Exception {
if ("item1".equals(item)) {
throw new Exception("Error occurred!");
}
}
}
Tip: Always consider exception handling within your lambda expressions to prevent unforeseen runtime errors.
5. Overcomplicating Lambda Expressions
While lambdas are meant to simplify your code, it is easy to fall into the trap of overcomplicating them. If a lambda is illustrating a complex operation, consider creating a method instead.
Example:
// Overly complex lambda
Comparator<String> comp = (a, b) -> {
if (a.length() == b.length()) return 0;
return a.length() > b.length() ? 1 : -1;
};
// Simpler via method
Comparator<String> comp = Comparator.comparingInt(String::length);
Tip: As a general rule, if you have multiple lines of code, consider extracting the logic into a separate method for clarity.
A Final Look
Java 8 lambdas are a powerful feature that can enhance code readability and maintainability. However, they come with their own set of pitfalls. By understanding variable scoping, the correct use of the this
keyword, the balance between lambdas and method references, proper exception handling, and avoiding unnecessary complexity, you can write better Java code.
For further reading on lambdas and functional programming in Java, you can check out the Java 8 in Action book or the official Java documentation.
By mastering these concepts and avoiding these common pitfalls, you can leverage Java 8 lambdas to improve your programming capabilities and write cleaner, more maintainable code. Happy coding!