Common Challenges in Defining Jakarta EE Specifications
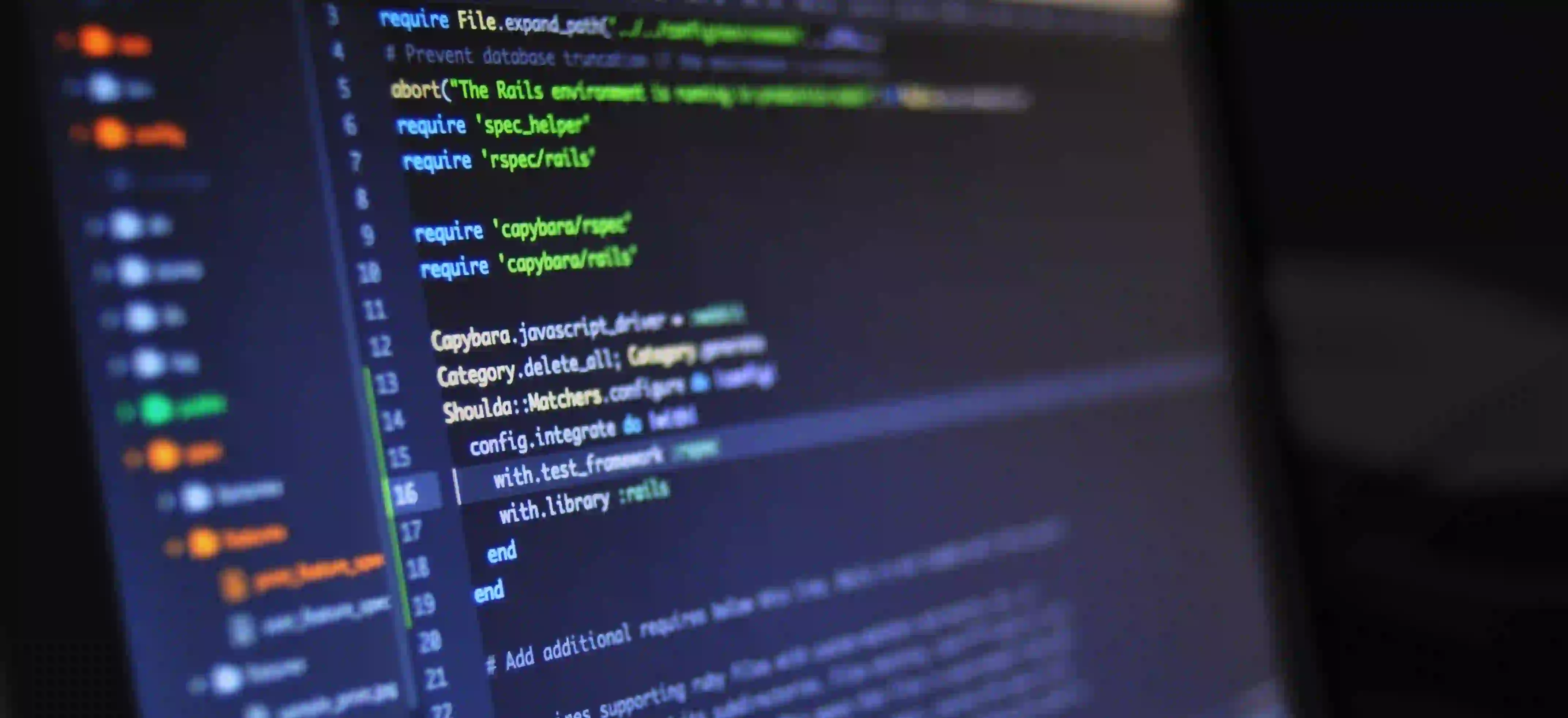
Common Challenges in Defining Jakarta EE Specifications
Jakarta EE, formerly known as Java EE, is a set of specifications that extend the Java SE with specifications for enterprise features such as distributed computing and web services. Defining these specifications is no small feat, as it involves navigating a landscape rich with complexity, demands, and expectations from developers, businesses, and the open-source community. In this blog post, we will explore some common challenges faced when defining Jakarta EE specifications.
What is Jakarta EE?
Jakarta EE is a collection of APIs and a set of standards that help developers build enterprise applications. It covers various aspects of application development such as data persistence, transaction management, messaging, and web services. Jakarta EE is governed by the Jakarta EE Working Group, a collective of industry leaders and contributors. The transition from Java EE to Jakarta EE marked a significant moment in which the APIs were transitioned to the Eclipse Foundation.
Key Challenges in Defining Jakarta EE Specifications
1. Fragmentation of Requirements
One of the primary challenges faced during the definition of Jakarta EE specifications is the fragmentation of requirements. Different stakeholders have diverse needs and expectations:
- Developers often push for better user experience.
- Businesses prioritize performance and security.
- Middleware vendors focus on interoperability and compatibility.
The challenge lies in balancing these conflicting priorities while maintaining a coherent standard. The Jakarta EE Working Group must cultivate open communication and facilitate discussions among all stakeholders.
2. Backward Compatibility
Backward compatibility is crucial for any specification. It ensures that applications built on earlier versions of Jakarta EE can seamlessly transition to newer versions without needing major rewrites. The challenge here is ensuring that new features and improvements do not break existing functionality.
For instance, when introducing a new API or updating an existing one, it must be compatible with legacy systems. If not, companies may shy away from adopting the latest version, hindering progress and innovation.
Example: Building a Simple CRUD Application
Here’s a simple example demonstrating a straightforward CRUD (Create, Read, Update, Delete) application using Jakarta EE. This serves to illustrate the importance of backward compatibility.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
// Default constructor for JPA
public Employee() {}
public Employee(String name) {
this.name = name;
}
// Getters and Setters
public Long getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, we define an Employee
entity with fields to store employee data alongside JPA annotations for persistence. Tools that rely on JPA for ORM will continue to function, illustrating the significance of keeping existing structures intact.
3. Handling Changes in Technology
The technological landscape is continually evolving, and Jakarta EE specifications must keep pace with advancements. Technologies such as microservices, containerization (like Docker), and cloud-native development are changing the way applications are built and deployed.
The challenge is not only to incorporate new paradigms into the existing specifications but also to ensure that legacy applications can migrate towards modern standards without severe implications. Ensuring that Jakarta EE stays relevant while maintaining a degree of continuity can be daunting.
For example, a specification may need to define standards for REST APIs in a microservices architecture, diverging from traditional monolithic approaches. This can create confusion or reluctance among teams who are more accustomed to older models.
4. Governance and Collaboration
Governance in the development of Jakarta EE is executed through open-source collaboration. While this is a significant advantage, it also presents challenges in achieving consensus. Multiple organizations (like Red Hat, IBM, and Payara) may have different strategies, increasing the potential for misalignment.
The challenge is to manage this collaborative effort effectively while ensuring a clear vision for Jakarta EE's future. Encouraging open dialogues and fostering a culture of transparency can mitigate such challenges.
5. Documentation and Community Support
Good documentation is essential for any specification, as it serves as a reference for developers. However, creating comprehensive and accessible documentation is often overlooked during the specification process.
Moreover, community support is also fundamental. Without a robust community backing, even the best-defined specifications may not gain traction. Utilizing platforms such as GitHub for community contributions and feedback can significantly improve documentation quality.
Example: Setting Up a RESTful Endpoint
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
@Path("/employee")
public class EmployeeResource {
@GET
@Produces("application/json")
public String getEmployee() {
// Mock employee data for demonstration
return "{\"id\": 1, \"name\": \"John Doe\"}";
}
}
This simple REST endpoint retrieves an employee's information and returns it in JSON format. Proper documentation of this endpoint in the Jakarta EE specification can facilitate wider usage among developers.
Final Considerations
Defining Jakarta EE specifications comes with its share of challenges, including managing fragmentation of requirements, ensuring backward compatibility, adapting to technological advancements, governance challenges, and maintaining robust documentation.
Despite these hurdles, the efforts of the Jakarta EE Working Group have led to sustained progress in providing a robust enterprise application framework. Through open collaboration and a commitment to continuous improvement, Jakarta EE remains poised to adapt to the ever-evolving landscape of enterprise computing.
For more detailed information on Jakarta EE, you can visit Jakarta EE Official Documentation and dive into specifics of the API and specifications.
Further Reading
- Enterprise Java Development
- Building Microservices with Jakarta EE
In the ever-changing world of technology, optimizing Jakarta EE definitions can help developers embrace modern practices while preserving legacy benefits. Happy coding!