Solving Common Issues in Spring REST API Swagger Integration
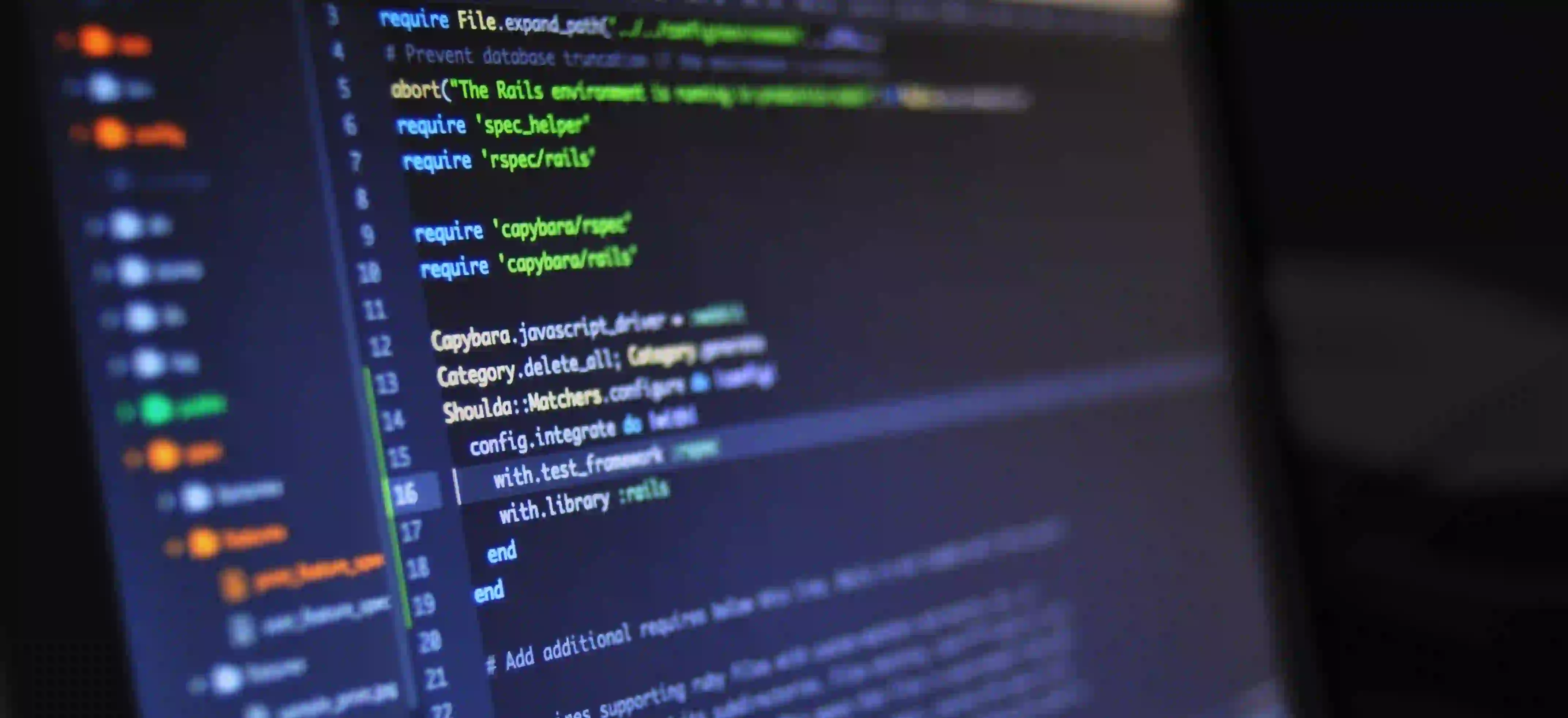
Solving Common Issues in Spring REST API Swagger Integration
Integrating Swagger with your Spring REST API can significantly enhance your development process. It provides a dynamic API documentation interface that helps developers and consumers understand the API's structure. However, there can be several issues that arise during the integration. This blog post will cover common problems you may encounter when integrating Swagger with a Spring REST API, along with solutions and best practices.
What is Swagger?
Before diving into the issues, let’s briefly clarify what Swagger is. Swagger, now part of the OpenAPI Specification, is a framework for documenting and testing RESTful APIs. It allows developers to describe the functionalities of their APIs in a machine-readable format.
Why Use Swagger?
- Automatic Documentation: Automatically generates visual documentation for your API.
- Interactive Testing: Allows developers to interact with API endpoints from a web interface.
- Client-Side Generation: Facilitates automatic generation of client libraries in various programming languages.
Setting Up Swagger in a Spring REST API
To begin, you need to add the necessary dependencies in your pom.xml
if you are using Maven.
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
Basic Configuration
Next, you can configure Swagger in your Spring Boot application:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example"))
.paths(PathSelectors.any())
.build();
}
}
In this configuration:
- The
@Configuration
annotation indicates that this is a Spring configuration class. - The
@EnableSwagger2
annotation activates Swagger in your Spring application. - The
Docket
bean configures how to scan and document your APIs by specifying the base package.
Common Issues and Solutions
1. Swagger UI Not Available
Issue: You might find that Swagger UI does not render properly when navigating to http://localhost:8080/v2/api-docs
.
Solution: Check the following:
- Ensure that the correct Swagger dependencies are included in your
pom.xml
. - Make sure that your API endpoints are annotated with
@RestController
or@Controller
. - Ensure that your application is running without any errors.
2. 404 Error on Swagger UI endpoint
Issue: When trying to access http://localhost:8080/swagger-ui/
, you get a 404 error.
Solution: It is possible that the UI path has changed. With SpringFox 3.0, you can now access Swagger UI using:
http://localhost:8080/swagger-ui/index.html
3. API Endpoints Not Displayed
Issue: Your API endpoints are not appearing in the Swagger UI.
Solution:
- Check your controller annotations. Make sure they are annotated with
@RequestMapping
,@GetMapping
,@PostMapping
, etc. - Confirm that there are no security configurations blocking access to the API endpoints. If you are using Spring Security, you may need to allow public access to Swagger's endpoints.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/v2/api-docs", "/swagger-ui/**").permitAll()
.anyRequest().authenticated();
}
4. Model Properties Not Displaying Correctly
Issue: The model properties are not displaying as expected in the Swagger UI.
Solution:
- Ensure your model classes are properly annotated with Swagger annotations, such as
@ApiModel
and@ApiModelProperty
.
Example:
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@ApiModel(description = "Details about the user")
public class User {
@ApiModelProperty(notes = "The unique ID of the user")
private Long id;
@ApiModelProperty(notes = "The name of the user")
private String name;
// Getters and setters
}
5. Versioning APIs
Issue: Handling multiple versions of your APIs can be tricky with Swagger.
Solution:
Create a configuration that captures different versions. You can set up multiple Docket beans. Here’s an example of how to configure it:
@Bean
public Docket apiV1() {
return new Docket(DocumentationType.SWAGGER_2)
.groupName("v1")
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.v1"))
.paths(PathSelectors.any())
.build();
}
@Bean
public Docket apiV2() {
return new Docket(DocumentationType.SWAGGER_2)
.groupName("v2")
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.v2"))
.paths(PathSelectors.any())
.build();
}
By defining additional Docket beans, each version's endpoints can be displayed separately in the Swagger UI, allowing clear differentiation.
6. Customizing Swagger Documentation
Issue: The default Swagger documentation might not meet your requirements.
Solution: Use annotations to customize the documentation. You can set a description, terms of service, and contact information directly in your Docket configuration.
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build()
.apiInfo(new ApiInfo("Your API Title",
"API Description",
"1.0",
"Terms of service URL",
new Contact("Your Name", "www.yourwebsite.com", "yourmail@example.com"),
"License",
"License URL",
Collections.emptyList()));
Best Practices for Integrating Swagger
- Keep Dependencies Up to Date: Regularly update Swagger dependencies to leverage new features and keep the documentation secure.
- Follow REST Principles: Maintain RESTful practices in your API design to make documentation meaningful.
- Use Meaningful Descriptions: Provide clear descriptions for each endpoint and data model. This enhances the documentation's usability.
- Version Your API: This will help consumers adapt to breaking changes over time.
A Final Look
Swagger is a powerful tool that can enhance your Spring REST API development process. While integrating it into your application can lead to a few common issues, most can be resolved with straightforward configurations or annotations.
By implementing the solutions outlined above, you can leverage Swagger to its full potential, providing a comprehensive documentation landscape that benefits both developers and users alike.
For further reading, check out the official Swagger documentation. If you have any questions or issues not covered in this post, feel free to leave a comment below!
Happy coding!