Streamlining Your Database: Common Pitfalls to Avoid
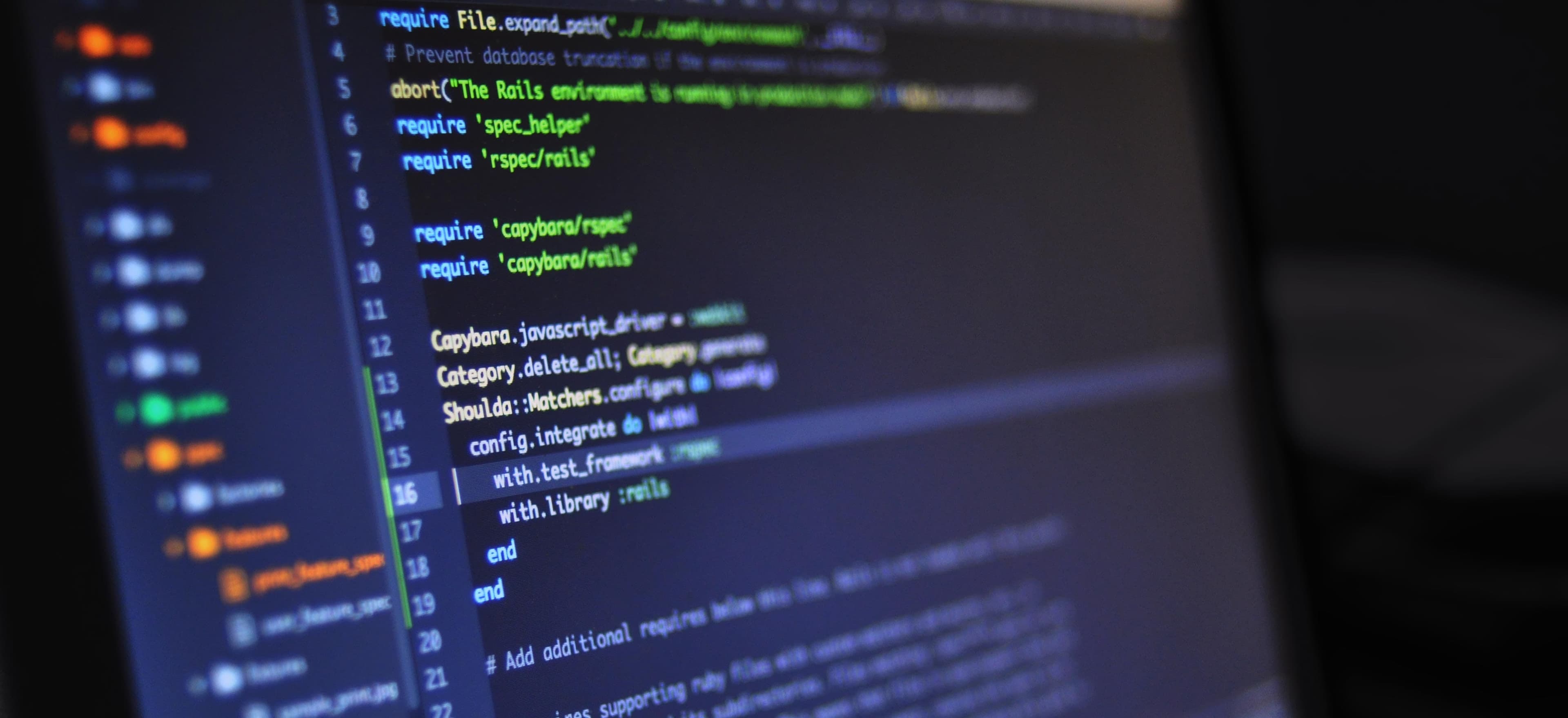
- Published on
Streamlining Your Database: Common Pitfalls to Avoid
In the world of web development, databases serve as the backbone of practically every application. They store, manage, and retrieve data efficiently, which is crucial for optimal performance and user experience. However, poorly managed databases can lead to performance issues, data corruption, and scalability challenges. In this blog post, we will explore common pitfalls that developers encounter when managing databases and how to avoid them.
Understanding Database Design
Before diving into specific pitfalls, it’s essential to recognize that efficient database design is the foundation of a robust application. A database should be designed with scalability, speed, and maintainability in mind. For a deep dive into database design principles, consider reading The Database Normalization Explained to grasp the importance of normalization.
1. Inadequate Normalization
Normalization is the process of organizing data to reduce redundancy. Many developers ignore this essential practice, leading to bloated database schemas. The first normal form (1NF) requires that your tables contain atomic values, while further normalization forms (2NF, 3NF, etc.) help eliminate transitive dependencies.
-- A poorly designed table example
CREATE TABLE Orders (
order_id INT,
customer_name VARCHAR(255),
product_name VARCHAR(255),
product_quantity INT
);
-- Instead, normalize to reduce redundancy.
CREATE TABLE Customers (
customer_id INT PRIMARY KEY,
customer_name VARCHAR(255)
);
CREATE TABLE Products (
product_id INT PRIMARY KEY,
product_name VARCHAR(255)
);
CREATE TABLE Orders (
order_id INT PRIMARY KEY,
customer_id INT,
product_id INT,
product_quantity INT,
FOREIGN KEY (customer_id) REFERENCES Customers(customer_id),
FOREIGN KEY (product_id) REFERENCES Products(product_id)
);
In the example above, the normalization process has led to separate tables for customers and products, eliminating redundancy and improving data integrity. This structure prevents anomalies during data insertion or deletion.
2. Rigid Schemas
One of the biggest mistakes developers make is creating rigid database schemas that don't adapt to changing requirements. Your application will inevitably evolve, and your database should accommodate growth.
To mitigate this pitfall:
- Use flexible data types when possible, such as JSON or XML, to store varying types of data within a single column.
- Leverage NoSQL databases if your data structure is continually changing. For instance, MongoDB is a popular option that supports document-oriented storage.
3. Ignoring Indexing
Indexes are critical for performance optimization. Without proper indexing, queries may take significantly longer, especially as the amount of data grows. Developers often neglect to create indexes or over-index, leading to longer write times.
-- Example of adding an index
CREATE INDEX idx_product_name ON Products(product_name);
In this code snippet, we create an index on the product_name
column in the Products table. This enhances search performance, allowing faster retrieval of data.
4. Failing to Monitor Performance
Many developers skip performance monitoring until issues become apparent. This approach can lead to inefficient queries and unresponsive applications. Utilize tools such as Grafana or New Relic to monitor your database and catch performance issues early.
Key metrics to track include:
- Query execution times
- Database uptime
- Disk usage
5. Unoptimized Queries
Writing optimized SQL queries is a non-negotiable proficiency for any developer. Common mistakes include:
- Using
SELECT *
instead of specifying the required columns. - Failing to consider the execution plan, which shows how SQL queries will be executed.
-- Instead of this:
SELECT * FROM Orders WHERE product_quantity > 10;
-- Use this:
SELECT order_id, customer_id FROM Orders WHERE product_quantity > 10;
By limiting data retrieval to only the necessary columns, performance improves, and resource consumption decreases.
6. Neglecting Backups
Backups are your insurance policy against data loss. Some developers maintain sporadic backup practices, leading to disastrous consequences when data corruption happens.
Implement a robust backup strategy, such as:
- Full backups weekly
- Incremental backups daily
- Test restoring backups consistently
Cloud solutions like AWS S3 can be a reliable option for storing backups.
7. Ignoring Transaction Management
When working with multiple queries, failing to use transactions can lead to inconsistent database states. A transaction ensures that a sequence of operations either completes entirely or not at all.
BEGIN;
INSERT INTO Orders (order_id, customer_id, product_id, product_quantity) VALUES (1, 1, 1, 10);
UPDATE Products SET product_quantity = product_quantity - 10 WHERE product_id = 1;
COMMIT;
In this example, if anything goes wrong during the insertion or update, the changes will not be committed, preventing inconsistent states and ensuring data integrity.
8. Hard-Coding Database Credentials
Hard-coding sensitive information, like database credentials, can expose your application to vulnerabilities. Instead, utilize environment variables to store credentials securely.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
public Connection connect() {
try {
String url = System.getenv("DB_URL");
String user = System.getenv("DB_USER");
String password = System.getenv("DB_PASSWORD");
return DriverManager.getConnection(url, user, password);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
}
By using environment variables, you safeguard sensitive information from unauthorized access.
9. Not Utilizing Database Caching
Caching can dramatically enhance performance by reducing load times. Implement solutions like Redis or Memcached to cache frequently accessed data.
Use caching for:
- User sessions
- Frequently read data
- Database query results
// Sample caching implementation with Redis
import redis.clients.jedis.Jedis;
public class CacheExample {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
jedis.set("user:1000", "John Doe");
String user = jedis.get("user:1000");
System.out.println("User: " + user);
}
}
In this example, Redis is used to cache user information, dramatically reducing response times for frequently requested data.
Bringing It All Together
Streamlining your database is essential for optimizing performance, ensuring data integrity, and enhancing user experience. By avoiding common pitfalls such as inadequate normalization, rigid schemas, unoptimized queries, and neglecting backups, developers can create efficient, robust databases.
Regular performance monitoring, effective transaction management, and the proper use of caching and indexing will lead to a more streamlined database. For further insights into database optimization techniques, check out Database Optimization Techniques.
Incorporating these practices into your development workflow will pave the way for a more resilient and high-performing database architecture. Happy coding!