Mastering Recursion: Optimize Your Fibonacci with DP
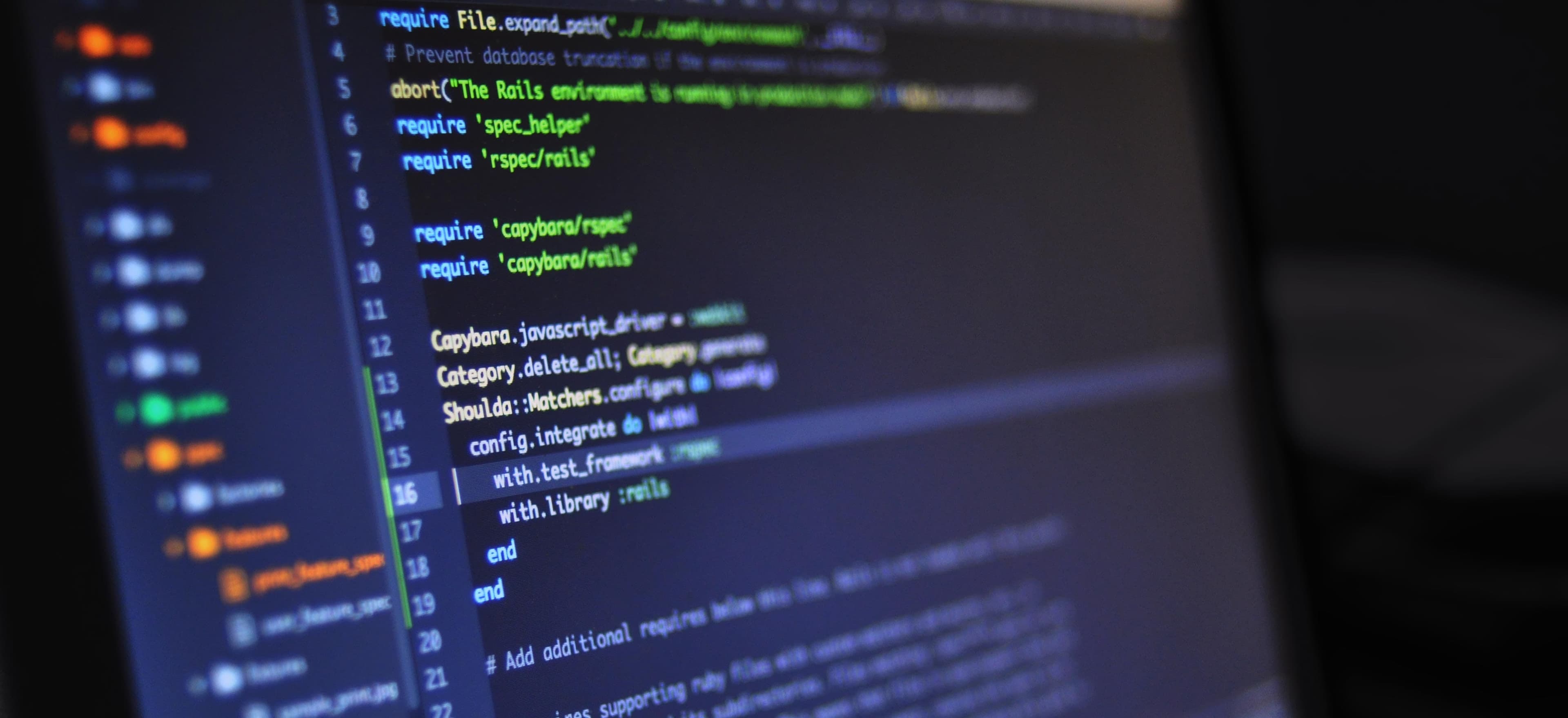
- Published on
Mastering Recursion: Optimize Your Fibonacci with Dynamic Programming
Recursion is one of the most fundamental aspects of programming, often leading to elegant solutions. However, its efficiency can be a concern in scenarios like calculating Fibonacci numbers. This blog post will delve into the world of recursion, focusing on how we can optimize the basic Fibonacci recursive algorithm using Dynamic Programming (DP).
Understanding the Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, typically starting with 0 and 1. The sequence looks like this:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...
Basic Recursive Implementation
Let's start with the basic implementation of the Fibonacci sequence using recursion:
public class Fibonacci {
public static int fib(int n) {
if (n <= 1) return n; // Base case: return n if n is 0 or 1
return fib(n - 1) + fib(n - 2); // Recursive case
}
public static void main(String[] args) {
int n = 10; // Change this value for different Fibonacci numbers
System.out.println("Fibonacci of " + n + " is " + fib(n));
}
}
Why Recursion is Inefficient
While this implementation is straightforward and easy to understand, it's highly inefficient for larger values of n
. The problem stems from the fact that it recalculates Fibonacci numbers multiple times. For instance, to compute fib(5)
, it will call fib(4)
and fib(3)
, and so on, resulting in an exponential time complexity of O(2^n). As n
increases, the number of function calls grows, making it impractical for larger n
.
Dynamic Programming Explained
Dynamic Programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful when the same subproblems recur repeatedly.
The Two Approaches in Dynamic Programming
-
Top-Down Approach (Memoization) - This involves storing results of expensive function calls and re-using them when the same inputs occur again.
-
Bottom-Up Approach (Tabulation) - This builds a table in a bottom-up manner and uses previously computed values.
In this tutorial, we will implement both approaches for calculating Fibonacci numbers.
Top-Down Approach: Memoization
Here’s how to optimize the Fibonacci recursive solution using memoization:
import java.util.HashMap;
public class Fibonacci {
private static HashMap<Integer, Integer> memo = new HashMap<>();
public static int fib(int n) {
if (n <= 1) return n; // Base case
if (memo.containsKey(n)) return memo.get(n); // Return cached value
int result = fib(n - 1) + fib(n - 2); // Calculate Fibonacci value
memo.put(n, result); // Store in cache
return result;
}
public static void main(String[] args) {
int n = 10;
System.out.println("Fibonacci of " + n + " is " + fib(n));
}
}
Why Use Memoization?
By storing the results of previous computations, we avoid redundant calculations. If fib(5)
has been computed, any subsequent call to fib(5)
will return the cached value instantly. This reduces the time complexity from O(2^n) to O(n).
Bottom-Up Approach: Tabulation
An alternative to memoization is the bottom-up approach, which constructs a table iteratively. Here’s how it looks:
public class Fibonacci {
public static int fib(int n) {
if (n <= 1) return n; // Base case
int[] fibTable = new int[n + 1]; // Initialize table
fibTable[0] = 0;
fibTable[1] = 1;
for (int i = 2; i <= n; i++) {
fibTable[i] = fibTable[i - 1] + fibTable[i - 2]; // Fill table
}
return fibTable[n]; // Return the desired Fibonacci number
}
public static void main(String[] args) {
int n = 10;
System.out.println("Fibonacci of " + n + " is " + fib(n));
}
}
Advantages of Tabulation
The bottom-up approach avoids the overhead of recursive calls and greatens performance. With a time complexity of O(n) and space complexity of O(n) due to the array, the implementation is concise and efficient.
Space Optimization
Although the previous implementations utilize O(n) space, we can reduce it to O(1) by only keeping track of the last two Fibonacci numbers. Here’s a more optimized version:
public class Fibonacci {
public static int fib(int n) {
if (n <= 1) return n; // Base case
int prev1 = 0, prev2 = 1; // Store the last two Fibonacci numbers
for (int i = 2; i <= n; i++) {
int current = prev1 + prev2; // Current Fibonacci number
prev1 = prev2; // Update previous numbers
prev2 = current;
}
return prev2; // Return the last Fibonacci number
}
public static void main(String[] args) {
int n = 10;
System.out.println("Fibonacci of " + n + " is " + fib(n));
}
}
In this version, we only keep the last two Fibonacci numbers, leading to constant space complexity O(1) and maintaining the O(n) time complexity.
My Closing Thoughts on the Matter
Recursion is a powerful tool in programming, but it can be inefficient when not used wisely. By adopting dynamic programming techniques, particularly memoization and tabulation, you can optimize recursive solutions dramatically. The techniques learned in this post not only apply to the Fibonacci sequence but can also be extended to solve many other complex problems efficiently.
If you're interested in furthering your understanding of algorithms and data structures, don't forget to check out GeeksforGeeks or LeetCode for comprehensive resources and challenges.
By mastering recursion and dynamic programming, you're empowering yourself to tackle a wider range of programming problems with confidence. Happy coding!