Common Pitfalls When Using RxJava for Async Operations
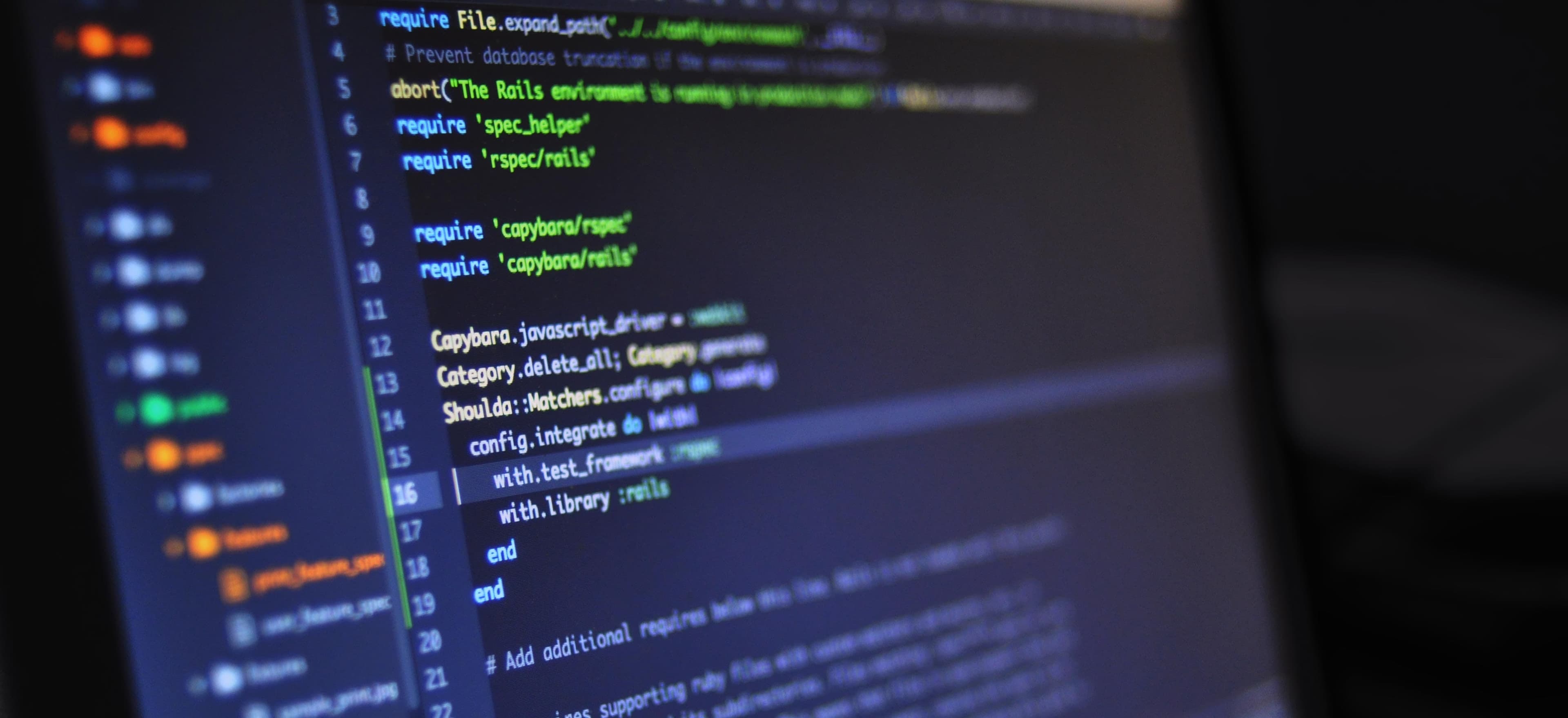
- Published on
Common Pitfalls When Using RxJava for Async Operations
RxJava is a powerful library that provides a great way to manage asynchronous operations in a clean, composable manner. However, with great power comes great responsibility, and it’s easy to fall into a few common pitfalls. Whether you are a seasoned developer or just starting with RxJava, understanding these pitfalls can help you write more efficient and maintainable code.
In this blog post, we'll cover some of the common pitfalls when using RxJava for asynchronous operations, complete with code snippets and explanations.
1. Threading Mismanagement
One of the biggest advantages of RxJava is the ability to manage threads. However, many novice users tend to overlook the importance of specifying the correct thread for subscribing, observing, or performing work.
Problem Illustration
Often, developers may unintentionally block the main thread by performing long operations directly.
Here's a simple example:
Observable.fromCallable(() -> {
// Some long-running task
Thread.sleep(5000);
return "Result";
})
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(result -> System.out.println(result),
throwable -> throwable.printStackTrace());
Why This Matters
In the above snippet, we correctly used subscribeOn(Schedulers.io())
to indicate that the task should run on an IO thread. However, the observeOn(AndroidSchedulers.mainThread())
is used correctly for sending results back to the UI thread. Always pair your threading choices wisely to avoid blocking any essential threads, especially the UI thread in Android.
2. Improper Error Handling
Failing to correctly manage errors in reactive programming can lead to unhandled exceptions, which may crash your application silently.
Problem Illustration
Consider the following example:
Observable.just("Hello World")
.map(string -> {
// Intentionally cause an error for demonstration
return Integer.parseInt(string);
})
.subscribe(System.out::println);
Why This Matters
In the example above, once the error is thrown, the observable chain is terminated and any downstream subscribers will not receive any data. To handle this properly, adding an error handler is crucial.
A Better Approach
Here's how to handle errors effectively:
Observable.just("Hello World")
.map(string -> Integer.parseInt(string))
.subscribe(System.out::println, throwable -> {
System.err.println("Error occurred: " + throwable.getMessage());
});
With the error handling mechanism in place, even if an error occurs, your application can respond gracefully.
3. Creating Memory Leaks with Subscriptions
One of the pitfalls developers face is not properly managing subscriptions, which can lead to memory leaks. In RxJava, any observable that you subscribe to will hold a strong reference to its observer, and failing to manage that can cause the application to retain resources unnecessarily.
Problem Illustration
Failing to dispose of a subscription can lead to a memory leak.
CompositeDisposable disposables = new CompositeDisposable();
disposables.add(
Observable.interval(1, TimeUnit.SECONDS)
.subscribe(seconds -> System.out.println("Tick: " + seconds))
);
Better Management
You should always dispose of your subscriptions, especially in the onStop or onDestroy methods.
@Override
protected void onDestroy() {
super.onDestroy();
disposables.clear(); // Clear all subscriptions to prevent memory leaks
}
Using CompositeDisposable
helps keep track of multiple subscriptions and provides an easy way to dispose of them when the object is no longer needed.
4. Using Blocking Calls in Observables
It may appear tempting to use blocking calls within your observable chains. However, this can lead to a deadlock situation or block critical threads.
Problem Illustration
Beware of calling blocking methods directly in observables:
Observable.create(emitter -> {
// Perform a blocking I/O task
emitter.onNext(performBlockingIO());
emitter.onComplete();
})
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(System.out::println);
Why This Matters
The performBlockingIO()
method can block the IO thread, which negates the entire benefit of using RxJava for asynchronous operations. Blocking operations will lead to degraded performance and application freezes.
A Better Approach
Instead, handle these operations within the worker thread:
Observable.fromCallable(() -> performBlockingIO())
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(System.out::println, throwable -> System.out.println("Error: " + throwable));
5. Over-Subscribing
Sometimes, developers inadvertently subscribe multiple times to an observable. This can lead to unexpected behaviors and resource consumption.
Problem Illustration
Observable<String> observable = Observable.create(emitter -> {
emitter.onNext("First emission");
emitter.onComplete();
});
observable.subscribe(System.out::println);
observable.subscribe(System.out::println); // This is a new subscription, leading to duplicate emissions
Better Management
Instead, consider using share()
to control the number of subscriptions:
Observable<String> sharedObservable = observable.share();
sharedObservable.subscribe(System.out::println);
sharedObservable.subscribe(System.out::println);
By using share()
, you can safely share the emissions among multiple subscribers without duplicating the work.
Final Considerations
RxJava offers tremendous capabilities for managing asynchronous operations, but it also brings various complexities. By being aware of common pitfalls, developers can leverage the library in a way that enhances application performance and maintainability.
Further Reading
- RxJava Documentation: Great reference for understanding RxJava’s API.
- Error Handling in RxJava: A deep dive into error handling techniques in RxJava.
By following best practices and being aware of these common mistakes, you can build robust reactive applications with RxJava, ensuring your asynchronous code is not just functional but also efficient. Happy coding!