Why Data Encoding is Crucial for App Security
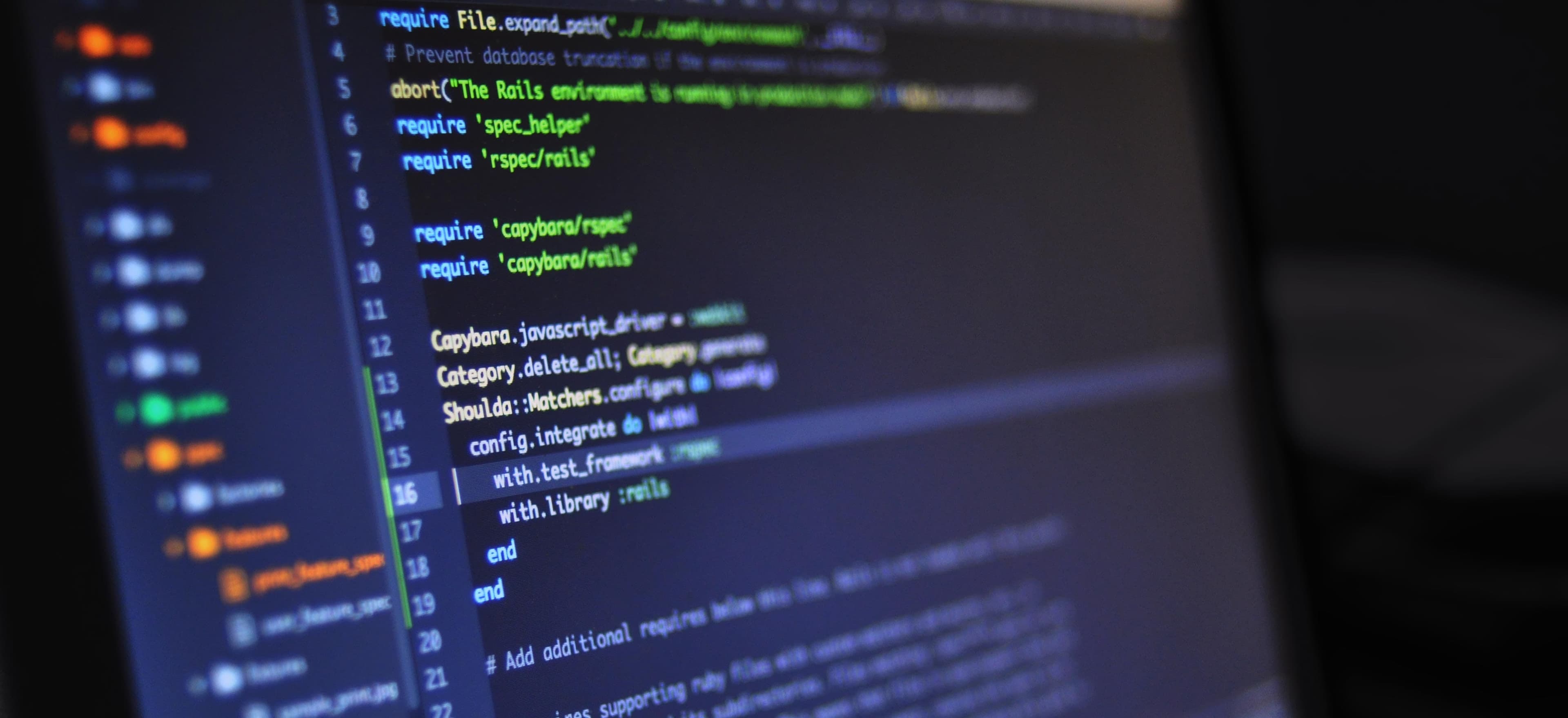
- Published on
Why Data Encoding is Crucial for App Security
In today's digital landscape, securing applications against potential threats is paramount. Data encoding is one of the key techniques that aid this endeavor. It involves transforming data into a different format using a scheme that is publicly available. Encoding is not only essential for data integrity and confidentiality but also plays a pivotal role in protecting applications from various vulnerabilities.
In this blog post, we will delve into the importance of data encoding in application security, discuss various encoding techniques, and highlight the best practices for implementing these techniques in your Java applications.
Understanding Data Encoding
Data encoding refers to the process of converting data from one form to another. While it may seem like a straightforward task, encoding serves multiple purposes:
- Data Integrity: It ensures that data remains intact during transmission.
- Data Representation: Different systems require different formats for data representation.
- Security: Encoding helps prevent unauthorized access by obscuring the original data.
Why Is Encoding Important for Security?
Data encoding plays a critical role in application security domains, including:
-
Input Validation: Many attacks exploit unvalidated input. Proper encoding can prevent code injection vulnerabilities, such as SQL injection, cross-site scripting (XSS), and command injection.
-
Data Exposure: Sensitive data, if unencoded, can be easily accessed or manipulated by unauthorized users. Encoding helps mask that data, adding a layer of protection.
-
Data Interchange: When transmitting data between different systems (e.g., between a client and a server), encoding ensures that the data remains consistent and unchanged.
Common Encoding Techniques
1. Base64 Encoding
Base64 encoding is a widely-used technique that transforms binary data into a text-based representation. It’s predominantly utilized for transmitting data over media designed to handle text.
Example of Base64 Encoding in Java
Here's how you can apply Base64 encoding in Java:
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
String originalInput = "Hello World";
// Encode the string to Base64
String encodedString = Base64.getEncoder().encodeToString(originalInput.getBytes());
System.out.println("Encoded String: " + encodedString);
// Decode back to the original string
byte[] decodedBytes = Base64.getDecoder().decode(encodedString);
String decodedString = new String(decodedBytes);
System.out.println("Decoded String: " + decodedString);
}
}
In the example above, we first encode the input string "Hello World" to Base64, then decode it back to its original format. This approach is frequently applied in web applications for data transmission.
2. URL Encoding
URL encoding is a scheme used to encode characters in URLs. It converts unsafe ASCII characters into a format that can be transmitted over the internet.
Example of URL Encoding in Java
Here’s a quick way to perform URL encoding in Java:
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class UrlEncodingExample {
public static void main(String[] args) {
try {
String originalUrl = "https://www.example.com/search?query=hello world";
String encodedUrl = URLEncoder.encode(originalUrl, "UTF-8");
System.out.println("Encoded URL: " + encodedUrl);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
By using URLEncoder.encode
, we safely encode spaces and special characters in the URL, ensuring that they are transmitted correctly. This is especially vital when dealing with user-generated content.
3. HTML Encoding
HTML encoding is crucial when displaying user input on web pages. It prevents XSS attacks by encoding special HTML characters.
Example of HTML Encoding in Java
Here’s how to perform HTML encoding in Java:
import org.apache.commons.text.StringEscapeUtils;
public class HtmlEncodingExample {
public static void main(String[] args) {
String unsafeString = "<script>alert('XSS Attack');</script>";
String safeString = StringEscapeUtils.escapeHtml4(unsafeString);
System.out.println("Original: " + unsafeString);
System.out.println("Encoded: " + safeString);
}
}
By using StringEscapeUtils
, we encode dangerous characters like <
, >
, and &
, ensuring that the input does not get executed as script code. This is a fundamental technique for preventing XSS attacks.
Best Practices for Data Encoding
To ensure robust app security with data encoding, consider the following best practices:
-
Always Encode User Input: Never trust user input. Always encode it before processing or displaying it in your application.
-
Utilize Built-in Libraries: Rely on established libraries (like
java.net.URLEncoder
or Apache Commons Text) for encoding. Custom implementations might overlook critical nuances. -
Use the Right Encoding for the Context: Know which encoding is suitable for your specific use case. For instance, use URL encoding for data in URLs, and HTML encoding for web content.
-
Regularly Update Libraries: Keep your libraries and dependencies updated to ensure you have the latest security patches and improvements.
-
Educate Your Team: Ensure that your development team understands the importance of encoding in web security. Conduct regular training sessions if necessary.
The Closing Argument
Data encoding is an indispensable aspect of application security. By transforming data into a secure format before transmission or processing, you significantly mitigate the risks associated with potential vulnerabilities such as SQL injection or XSS.
Incorporating various encoding techniques, from Base64 to URL and HTML encoding, provides an extra layer of defense that can determine the success of your application's security strategy. By following best practices and staying informed about modern security standards, you can build robust applications that stand the test of time.
For further reading on secure coding practices, check out OWASP's Secure Coding Practices and Java Security. Together, these resources can enhance your understanding and implementation of security in application development.
Embrace data encoding, and safeguard your applications against security threats effectively.