Common Pitfalls in Testing Client-Side RESTful Services
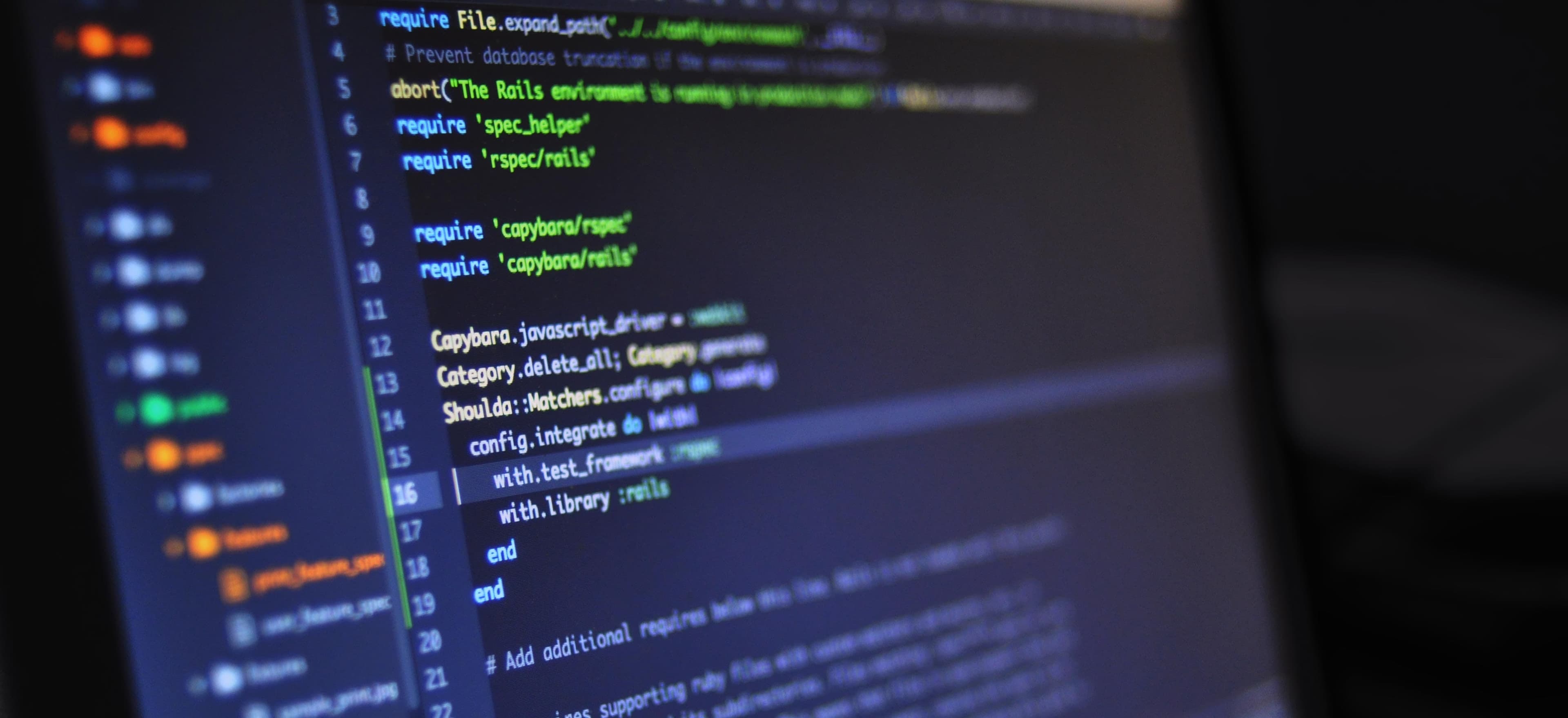
- Published on
Common Pitfalls in Testing Client-Side RESTful Services
The demand for robust, efficient web applications is higher than ever, and RESTful services play a crucial role in modern client-server architectures. Testing these services effectively can lead to a seamless user experience. Yet, many developers encounter several common pitfalls when it comes to that testing process. In this post, we will explore these challenges and provide actionable solutions to help you navigate them.
Understanding RESTful Services
Before we delve into the pitfalls, it's important to have a solid understanding of what REST (Representational State Transfer) services encapsulate. They enable communication between clients and servers using standardized HTTP methods such as GET, POST, PUT, DELETE, etc. When designing and testing RESTful APIs, the following principles must be upheld:
- Stateless operations: Each request from client to server must contain all the necessary information.
- Resource-based architecture: Every resource should be accessible via a unique URI.
- Use of standard HTTP methods: Each method should perform a specific action related to the resource.
Understanding these principles is fundamental to ensuring that your client-side interactions with RESTful services are effective.
Common Pitfalls in Testing RESTful Services
1. Ignoring Negative Test Cases
Many developers focus exclusively on positive test cases, assuming that if the happy path works, the service is functioning correctly. This is a significant oversight. Negative test cases are crucial as they evaluate how well your application handles unexpected inputs.
Example:
Suppose you are testing a login service. You might use the following valid credentials in your positive tests and expect success:
public void testValidLogin() {
// Assuming loginSuccess() communicates with the REST API
assertTrue(loginSuccess("user", "pass123")); // Expect true
}
However, consider the negative case where invalid credentials are supplied:
public void testInvalidLogin() {
assertFalse(loginSuccess("user", "wrongPass")); // Expect false
}
Why: By ignoring negative cases, you might overlook critical vulnerabilities that could be exploited or usability issues that could frustrate users.
2. Not Using Proper Assertions
Another common pitfall is failing to use meaningful assertions in your tests. Confirming an HTTP response status code is not enough. You should check not only for correct status codes but also the response body and headers.
Example:
Response response = client.target("http://api.example.com/resource/1")
.request()
.get();
assertEquals(200, response.getStatus()); // Check if the status is success
assertNotNull(response.readEntity(String.class)); // Ensure the body is not null
Why: This ensures that the data returned is valid and meets your expectations. Lack of comprehensive assertions may result in missing crucial bugs.
3. Testing in Isolation
While unit testing is essential for individual components, testing client-side RESTful services in complete isolation can lead to complacency. Integration testing should also be a priority to capture issues that may arise when components interact.
Example:
@Test
public void testFullServiceInteraction() {
// Simulate a full interaction between the client and REST API
// Assuming API returns user details
Response response = client.target("http://api.example.com/user/1")
.request()
.get();
User user = response.readEntity(User.class);
assertEquals("John", user.getName()); // Check integrated result
}
Why: This provides more confidence that the system works as a whole, rather than just as individual pieces.
4. Stepping Over Rate Limiting and Timeouts
Another issue often faced in testing RESTful services is neglecting to account for rate limits or timeouts defined by the server. When tests fail due to these constraints, developers may overlook underlying issues.
Example:
@Test(expected = TimeoutException.class)
public void testServiceTimeout() {
client.property("javax.ws.rs.client.connectTimeout", 1000);
Response response = client.target("http://api.example.com/slow-service")
.request()
.get();
}
Why: Implementing proper timeout checks helps to ensure that your application can gracefully handle possible delays in service responses.
5. Overusing Mocking
Mocking is a powerful technique in testing; however, over-reliance on it can create an unrealistic testing environment. Mocked responses may not adequately reflect real-world scenarios, potentially masking vulnerabilities.
Example:
when(apiClient.getUser(anyInt())).thenReturn(mockUser); // Mocked response
Why: Utilize mocks judiciously, ensuring at least some tests involve actual API calls to simulate realistic behavior and edge cases more effectively.
6. Lack of Thorough Documentation
Comprehensive documentation of your testing strategy and results is often overlooked. Without clear, understandable documentation, other developers may misinterpret how the tests work, leading to gaps in knowledge when changes are needed.
Why: Proper documentation allows for consistency during team collaboration, as well as offering a point of reference for debugging and enhancing tests in the future.
Best Practices for Testing Client-Side RESTful Services
To sidestep these pitfalls, consider the following best practices:
-
Adopt a Comprehensive Testing Strategy: Cover positive, negative, and boundary cases for a thorough examination of your services.
-
Utilize Meaningful Assertions: Go beyond simple status checks and assert relevant response data.
-
Incorporate Integration Testing: Test interactions between client and RESTful services to ensure they work harmoniously.
-
Mind Rate Limiting and Timeouts: Factor these conditions into your tests conclusively.
-
Balance Mocking with Real Calls: Use mocks when appropriate, but maintain real interactions in at least some test cases.
-
Document Your Findings: Keep track of your tests and their outcomes for consistent communication among team members.
Closing Remarks
Testing client-side RESTful services requires attention to detail and a structured approach. By being aware of and addressing the common pitfalls discussed in this article, you can elevate your testing processes, significantly improving your applications' resiliency and overall user experience.
In summary, remember that effective testing encompasses comprehensive cases, meaningful assertions, real-world interactions, and thorough documentation. By embracing these principles, you can navigate the challenges of RESTful service testing with confidence.
For further reading on RESTful API testing strategies, check out this guide on Baeldung, and explore the nuances of mocking in tests by Martin Fowler.
Happy testing!