Overcoming Common Pitfalls in Hystrix Implementation
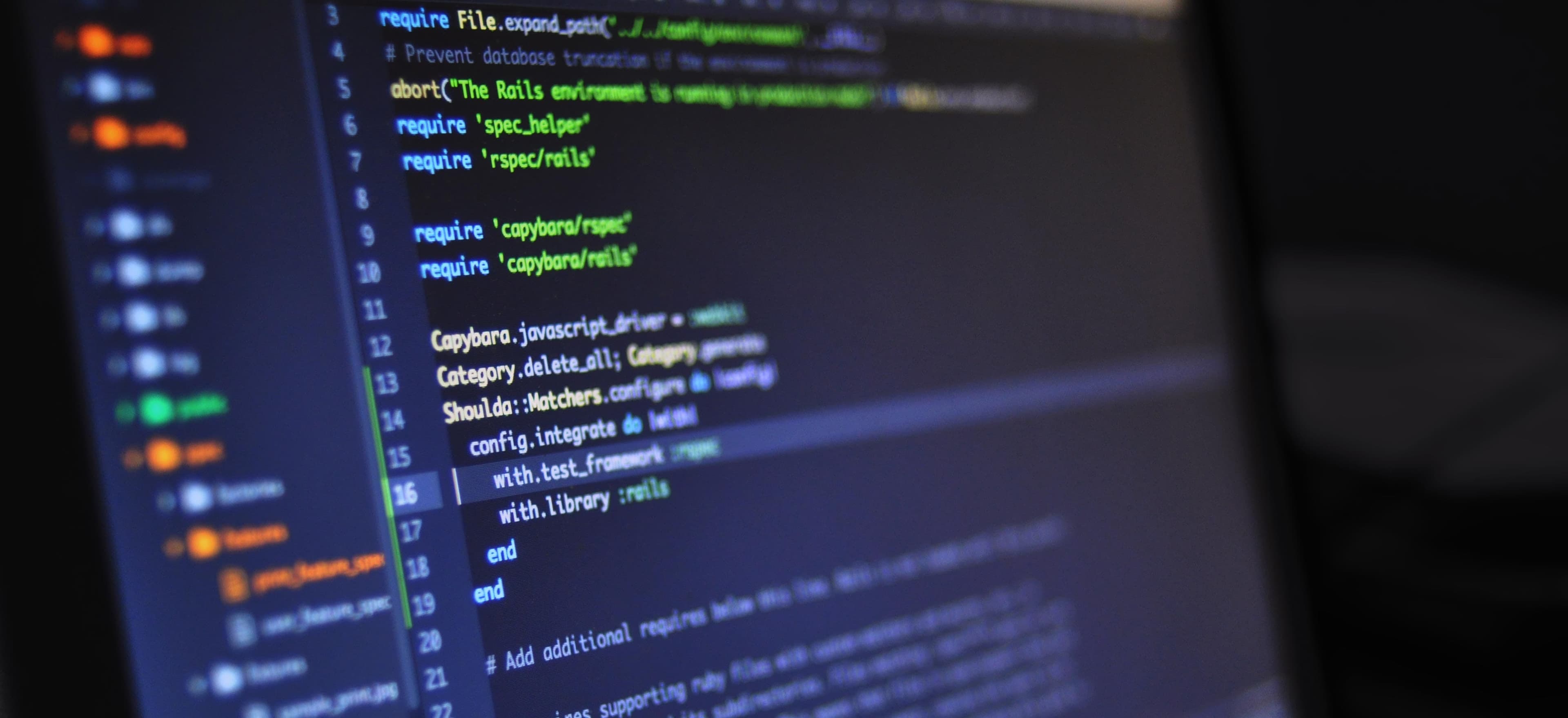
- Published on
Overcoming Common Pitfalls in Hystrix Implementation
In microservices architecture, resilience is paramount. One framework that has gained widespread popularity for building resilient applications is Hystrix. Developed by Netflix, Hystrix creates a barrier around your services, allowing them to fail gracefully. By implementing Hystrix, developers can manage latency and avoid cascading failures in a distributed system. However, despite its robust capabilities, many teams encounter common pitfalls when integrating and utilizing Hystrix. In this blog post, we'll discuss these pitfalls and offer strategies for overcoming them.
What is Hystrix?
Before diving deeper into the pitfalls, let's clarify what Hystrix does. Hystrix is a library that helps control the interactions between services by providing features like:
- Circuit Breaker: Prevents calls to a failing service, allowing the system to recover.
- Fallbacks: Provides an alternative response when the original call fails.
- Timeouts: Ensures that requests that take too long are aborted.
For more information, you can check out the official Hystrix documentation.
Common Pitfalls in Hystrix Implementation
1. Lack of Configuration
One common issue when implementing Hystrix is a lack of appropriate configuration. By default, Hystrix comes with its own settings, which may not suit your application's needs.
Solution
Customize the Hystrix settings to match your specific use case. The following parameters are commonly configured:
- Timeout: The maximum time to wait for a response.
- Circuit Breaker Thresholds: Define how many failures are allowed before the circuit breaker kicks in.
Here is an example of setting these configurations:
import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand;
public class ExampleService {
@HystrixCommand(
fallbackMethod = "fallbackMethod",
commandKey = "exampleCommand",
commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "3000"),
@HystrixProperty(name = "circuitBreaker.requestVolumeThreshold", value = "5"),
@HystrixProperty(name = "circuitBreaker.sleepWindowInMilliseconds", value = "5000"),
@HystrixProperty(name = "circuitBreaker.errorThresholdPercentage", value = "50")
}
)
public String riskyOperation() {
// Simulated risky operation that may fail
return "Success!";
}
public String fallbackMethod() {
return "Fallback response!";
}
}
In this example, we configure Hystrix to have a timeout of 3 seconds, a request volume threshold of 5, and a circuit breaker that activates when 50% of requests fail. Adjust these settings based on your system requirements to prevent unnecessary service outages.
2. Ignoring Fallbacks
Another common mistake is overlooking the importance of fallback methods. Without adequate fallback strategies, your service becomes less resilient.
Solution
Always provide a fallback method for every Hystrix command. Fallback methods should return relevant results or an appropriate error message, ensuring that the client still receives a response even if the original operation fails.
Example:
public String fallbackMethod() {
return "Service is currently down. Please try again later.";
}
By providing a friendly error response, you maintain a good user experience even in failure situations.
3. Overusing the Circuit Breaker
While the circuit breaker is an essential feature, overusing it can lead to decreased throughput for your application. Too many services trying to communicate with one another can cause multiple circuit breakers to trigger, leading to what is known as a "cascading failure."
Solution
Be judicious in your use of circuit breakers. They should only be used where there is a genuine risk of failure. Evaluate service dependencies and ensure that you have implemented circuit breakers only for the most critical calls.
4. Not Using Thread Isolation
Hystrix allows you to choose between thread isolation and semaphore isolation. Not utilizing thread isolation when necessary can significantly affect your application performance.
Solution
Thread isolation creates a separate thread pool for your Hystrix commands, preventing them from blocking the main thread. Use the following configuration for thread isolation:
@HystrixCommand(
threadPoolKey = "myThreadPool",
threadPoolProperties = {
@HystrixProperty(name = "coreSize", value = "10"),
@HystrixProperty(name = "maxQueueSize", value = "5")
}
)
In this example, the command has its own thread pool with a core size of 10 and a max queue size of 5. Adjust these properties based on your system's needs to reduce latency and improve performance.
5. Neglecting Monitoring and Metrics
One of the most significant mistakes is neglecting to monitor Hystrix metrics. Failing to monitor can lead to unexpected system behavior and hinder your ability to analyze failures.
Solution
Utilize Hystrix's dashboard or integrate with monitoring tools like Spring Cloud Netflix to keep track of metrics and visualize the health of your services. Here’s how you can set up Hystrix Dashboard:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-hystrix-dashboard</artifactId>
</dependency>
With the dashboard in place, you can visualize metrics such as response time, error rates, and circuit breaker states, providing invaluable insights into system performance.
6. Inadequate Testing of Hystrix Commands
Many teams fail to adequately test Hystrix commands, especially their fallback methods. This oversight can lead to further issues when the application is live and experiencing failures.
Solution
Implement thorough unit and integration tests for Hystrix commands, particularly focusing on testing fallback methods under various failure conditions.
Example of a test case:
@Test
public void testFallbackMethod() {
ExampleService service = new ExampleService();
String response = service.riskyOperation(); // simulate failure
assertEquals("Fallback response!", service.fallbackMethod());
}
By ensuring your fallback methods work as expected, you can maintain a high level of resilience in your application.
The Last Word
Implementing Hystrix can significantly enhance your microservices' resilience, but it’s crucial to avoid common pitfalls. Customizing configurations, ensuring adequate fallbacks, being cautious about circuit breaker usage, utilizing thread isolation, actively monitoring metrics, and testing thoroughly are vital aspects of a successful Hystrix implementation.
To deepen your understanding of Hystrix and resilience patterns, consider reading through additional resources such as Microservices Patterns by Chris Richardson.
By following these best practices, your distributed system will be better positioned to handle failures gracefully, providing a seamless experience to your users. Happy coding!