Common Query Building Pitfalls in InfluxDBMapper for Java
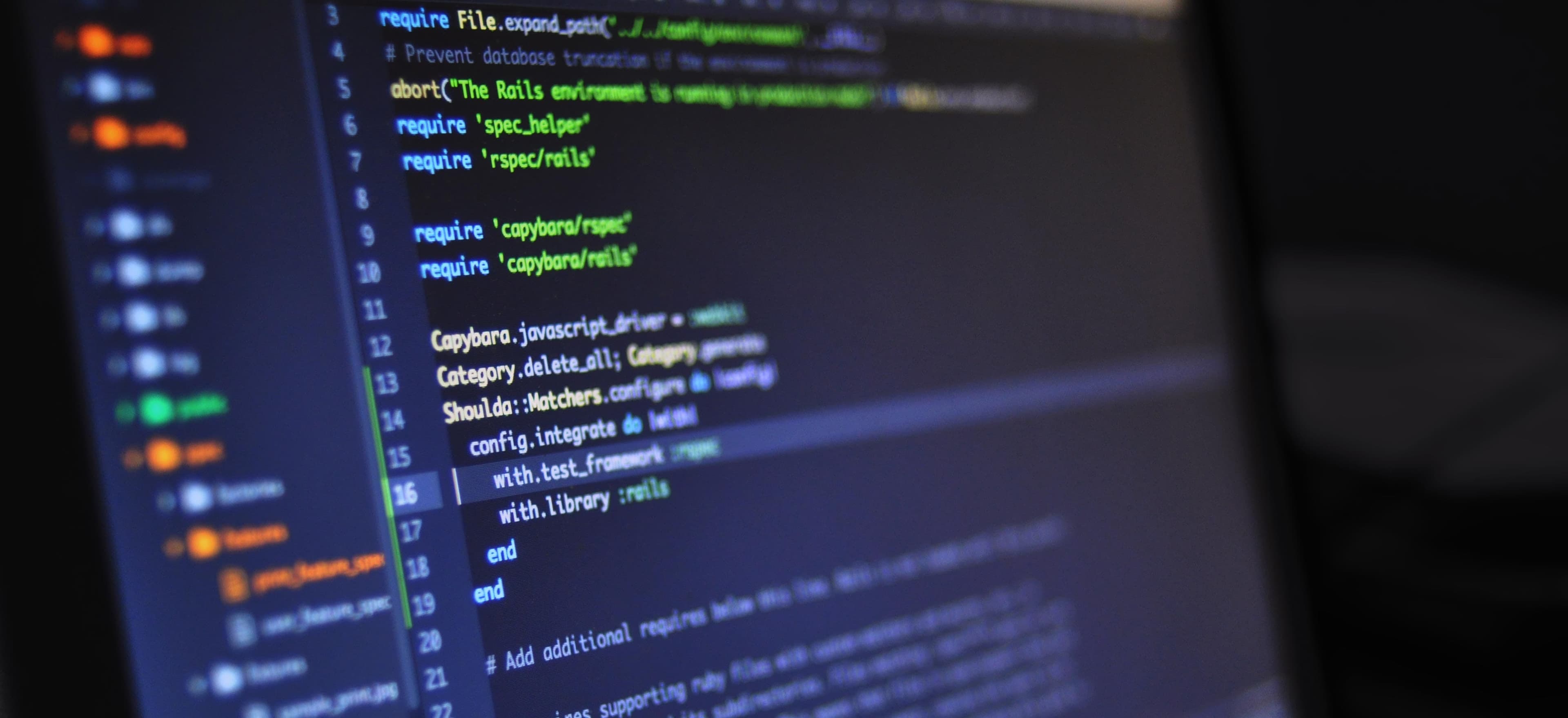
- Published on
Common Query Building Pitfalls in InfluxDBMapper for Java
InfluxDB has become a popular choice for time-series data due to its speed and efficiency. When using InfluxDBMapper in Java, developers can struggle with certain query-building pitfalls. These mistakes can lead to incorrect data retrieval or performance issues. This blog post will discuss common pitfalls in building queries with InfluxDBMapper and provide solutions to ensure your queries are both efficient and accurate.
Understanding InfluxDBMapper
InfluxDBMapper is an ORM-like library that allows Java developers to interact with InfluxDB using Java objects. This abstraction simplifies queries and enhances code readability. However, it is essential to fully understand how it works to avoid common mistakes.
Key Features of InfluxDBMapper
- Object-Relational Mapping (ORM): Maps Java objects to InfluxDB measurements.
- Fluent API: Allows for simplified query building using a chainable interface.
- Annotations: Supports annotations to define measurements, fields, and tags.
For more details on how to set up InfluxDBMapper, you can refer to the InfluxDBMapper documentation.
Common Pitfalls in Query Building
1. Incorrect Measurement Naming
One of the first pitfalls is using incorrect measurement names. InfluxDB is case-sensitive, and naming conventions can be unclear. A measurement named "Temperature" is not the same as "temperature."
Solution: Always double-check your measurement names.
Code Example
@Measurement(name = "Temperature")
public class TemperatureReading {
@Field
private double value;
@Tag
private String location;
}
Ensure the measurement name in the annotation matches exactly with the name in the database.
2. Failing to Handle Time Series Data Properly
Another common mistake is mishandling time series data when building queries. This can lead to misinterpretations of data points over time.
Solution: Always be explicit about your time conditions.
Code Example
List<TemperatureReading> readings = influxDBMapper.query(
new Query("SELECT value FROM Temperature WHERE time > now() - 1h")
);
In the example, a query is built to fetch readings from the last hour. Omitting the time condition can lead to retrieving all data points, which could significantly affect performance.
3. Not Using Tags Efficiently
InfluxDB provides tags for indexing, which can speed up query performance. Avoid using only fields for queries that could benefit from tags.
Solution: Utilize tags for frequent filter conditions in your queries.
Code Example
List<TemperatureReading> readings = influxDBMapper.query(
new Query("SELECT value FROM Temperature WHERE location = 'Office'")
);
In this scenario, filtering on the tag 'location' enhances the speed of the query when compared to filtering on a field.
4. Ignoring Time Precision
When storing and querying time series data, InfluxDB allows you to define time precision. However, many developers overlook this and may result in missed data points or unexpected data aggregation.
Solution: Specify the time precision explicitly when inserting data.
Code Example
TemperatureReading reading = new TemperatureReading();
reading.setValue(22.5);
reading.setLocation("Office");
influxDBMapper.save(reading, TimeUnit.SECONDS);
By specifying TimeUnit.SECONDS
, you ensure that the time stored in InfluxDB has the correct precision. Failure to specify accuracy can lead to unintentional data loss.
5. Aggregating Data Incorrectly
Many developers misunderstand how to aggregate their data, which can lead to inaccurate conclusions from their queries.
Solution: Approach aggregation with caution and verify your queries.
Code Example
List<AggregateResult> results = influxDBMapper.query(
new Query("SELECT MEAN(value) FROM Temperature GROUP BY location")
);
By using MEAN(value)
, you are aggregating and grouping the results by 'location' effectively. Misunderstanding aggregation can skew your data analysis.
6. Overlooking the Performance Impact of Wildcards
Using wildcards in your queries can lead to performance issues, especially in large datasets.
Solution: Avoid using SELECT * or partial wildcards if possible.
Code Example
List<TemperatureReading> readings = influxDBMapper.query(
new Query("SELECT value FROM Temperature WHERE location = 'Office'")
);
This practice ensures you only query necessary fields, optimizing performance and speed.
7. Lack of Error Handling
Developers often forget to implement error handling while querying InfluxDB. This step is essential for ensuring that issues do not crash the application.
Solution: Always implement try-catch blocks around your query execution.
Code Example
try {
List<TemperatureReading> readings = influxDBMapper.query(
new Query("SELECT value FROM Temperature WHERE time > now() - 1h")
);
} catch (InfluxDBException e) {
System.err.println("Error querying InfluxDB: " + e.getMessage());
}
By incorporating error handling, you can gracefully manage any potential issues during your queries.
My Closing Thoughts on the Matter
Building queries with InfluxDBMapper can be streamlined and effective once you understand common pitfalls. By paying careful attention to measurement naming, time handling, and how you use tags, you can improve both accuracy and performance. Remember to implement best practices, such as precise time specifications and sound error management.
By avoiding these mistakes, your experience with InfluxDB in Java can be significantly more productive, allowing you to focus on deriving insights from your time-series data.
For further reading and examples, consider checking out the official InfluxDB documentation and connect with the Open Source Community to learn from other developers' experiences.
Your journey with InfluxDBMapper is just beginning. Keep exploring, learning, and improving your code. Happy coding!
Checkout our other articles