Struggling with Unit Test Scaffolding? Here’s How to Fix It!
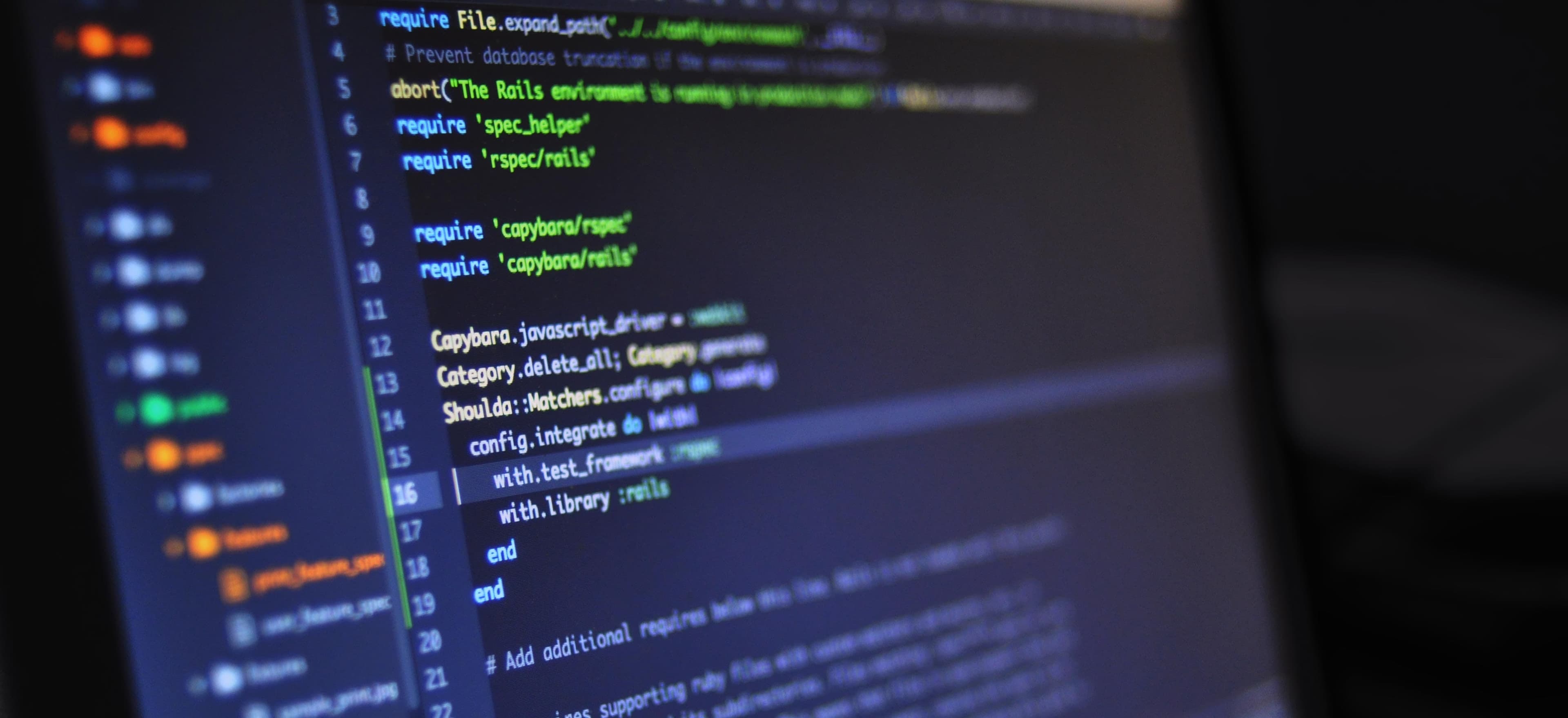
- Published on
Struggling with Unit Test Scaffolding? Here’s How to Fix It!
Unit testing is an essential part of software development. It ensures that your code functions correctly and helps to identify bugs early, reducing the cost of fixing them later. However, getting started with unit testing, especially if you're new to it, can feel overwhelming. In this blog post, we will discuss unit test scaffolding, what it is, why it's important, and how to fix any struggles you may face while setting it up in your Java applications.
Understanding Unit Test Scaffolding
Unit test scaffolding is the process of creating the necessary structure to write and run unit tests effectively. It involves setting up a framework, organizing your test cases, and ensuring that you have the right tools to execute these tests.
In Java, there are several options available for unit testing frameworks, including JUnit and TestNG. JUnit is the most widely used framework for unit testing in Java applications.
Why Use JUnit for Unit Testing?
- Community Support: JUnit has a large and vibrant community behind it, offering extensive documentation and resources for developers.
- Simplicity: It has a simple and intuitive API, making it easy to integrate into any project.
- Integration: JUnit can be easily integrated with various build tools such as Maven and Gradle.
If you want to learn more about JUnit, visit JUnit's official site.
Getting Started with Unit Test Scaffolding in Java
1. Setting Up Your Project
Before writing your tests, you need to set your project up. If you're using Maven, you can add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
This dependency allows you to use JUnit 5 for your tests. Make sure the <scope>
is set to test
, as you only want this library available in your test phase.
2. Creating a Test Class
Next, you need to create a new test class. It is a common convention to name your test class after the class you are testing, appending "Test" to it. Here's an example where we test a simple class Calculator
.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
You would create a test class like this:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
Explanation of the Test Code
@Test
Annotation: This annotation marks the method as a test method.assertEquals()
: This is an assertion method that checks whether the expected value (5) matches the actual value returned by theAadd()
method.
3. Running Your Tests
To run your tests, you can either use your IDE’s built-in functionality or your build tool. For Maven, use the following command:
mvn test
This will compile your project and run all tests in the src/test/java
directory. You’ll see output that informs you whether your tests passed or failed.
Common Pitfalls and How to Overcome Them
Pitfall 1: Ignoring Test Coverage
Failing to measure test coverage can lead to untested parts of your code. Use tools like JaCoCo or Cobertura to gauge how much of your code is covered by tests.
To include JaCoCo in your Maven project, add this plugin to your pom.xml
:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
</executions>
</plugin>
Pitfall 2: Not Writing Meaningful Tests
It's easy to write tests that are merely pass/fail checks. However, it's crucial to write meaningful tests that evaluate the expected outcomes based on multiple scenarios.
Take the following simple use case for a Calculator
class:
@Test
public void testAddWithNegativeNumbers() {
Calculator calculator = new Calculator();
assertEquals(1, calculator.add(-2, 3), "-2 + 3 should equal 1");
}
Here, you are testing the behavior of addition when negative numbers are involved.
Pitfall 3: Lack of Setup and Teardown
If your tests require a common setup, use the @BeforeEach
and @AfterEach
annotations:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.AfterEach;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setUp() {
calculator = new Calculator();
}
@AfterEach
public void tearDown() {
// Clean up resources or reset states if necessary.
}
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
These methods are helpful for instantiating objects before each test.
Enhancing Your Unit Testing Strategy
1. Test-Driven Development (TDD)
Incorporating Test-Driven Development (TDD) can help you write better tests. TDD advocates writing tests before you write the code. This approach ensures that your tests are directly aligned with your code's functionality.
2. Continuous Integration (CI)
By integrating your unit tests into a CI pipeline (using tools like Jenkins or GitHub Actions), you can automatically run tests whenever changes are made. This helps catch issues sooner.
Lessons Learned
Unit testing is an invaluable practice in software development that leads to higher quality code. Proper unit test scaffolding sets the foundational structure needed for effective tests. By employing frameworks like JUnit, measuring test coverage, and following best practices, you'll be well on your way to a solid testing strategy.
Struggling with unit test scaffolding doesn’t have to be a bottleneck in your development. Start small and build upon your strategies as you gain familiarity and confidence in writing tests. For the latest updates on unit testing practices in Java, check out the JUnit 5 User Guide.
Happy testing!
Checkout our other articles