Mastering Exception Handling in Functional Programming
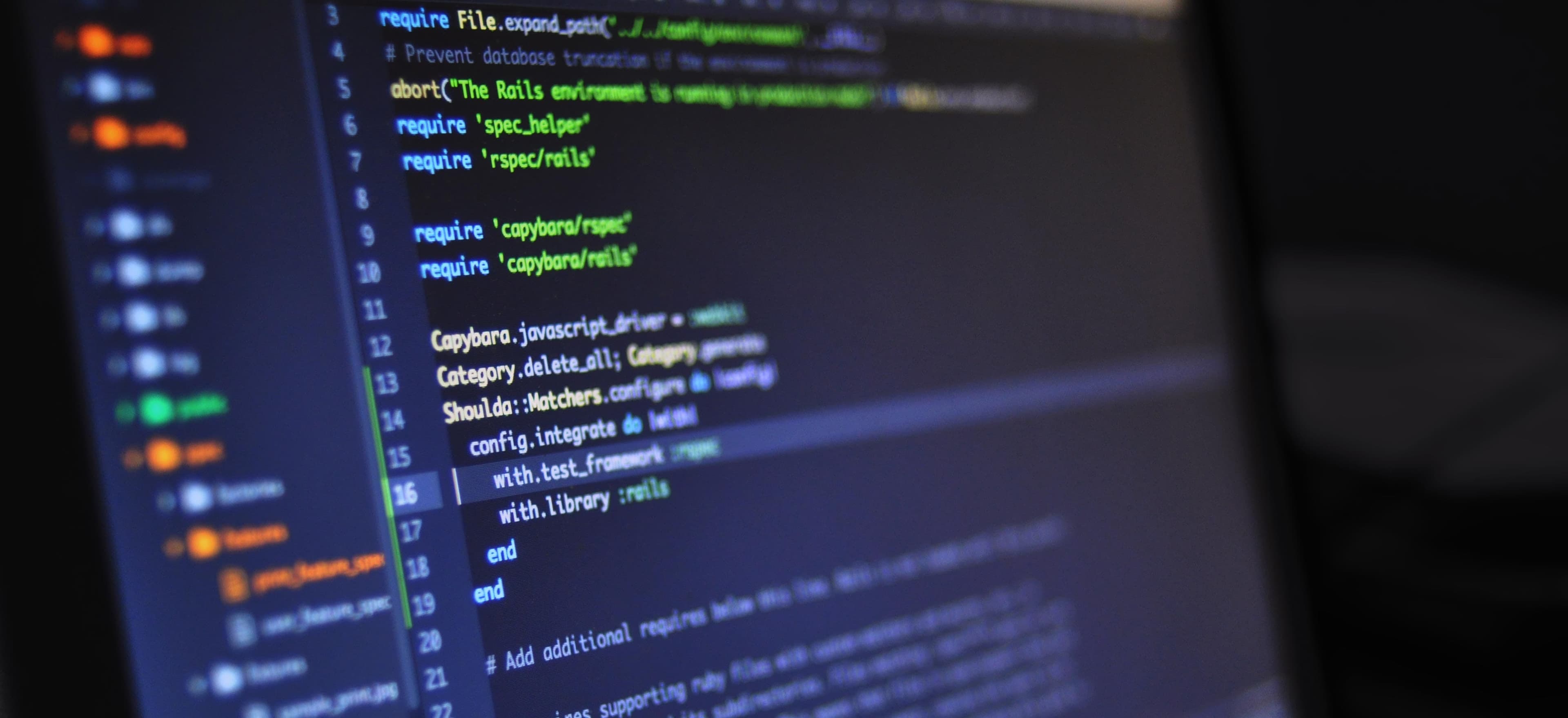
- Published on
Mastering Exception Handling in Functional Programming
Exception handling is a critical aspect of software development, ensuring that your applications can gracefully handle errors and maintain a good user experience. While traditional imperative programming languages like Java often handle exceptions with try-catch blocks, functional programming takes a different approach. In this post, we will explore how to manage exceptions effectively in functional programming, with a particular focus on Java's adoption of functional programming paradigms.
Understanding Functional Programming Concepts
Before diving into exception handling, it's essential to grasp some key concepts of functional programming (FP):
- First-class citizens: In FP, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Immutability: FP emphasizes working with immutable data. Once data is created, it cannot be changed.
- Higher-order functions: These are functions that take other functions as parameters or return them as results.
- Pure functions: A pure function's output is determined solely by its input values, with no side effects.
With these principles in mind, let's explore how to handle exceptions in functional programming.
Exception Handling Basics in Java
In Java, exception handling typically relies on try-catch blocks. Here's a quick overview:
try {
// Code that might throw an exception
} catch (SpecificException e) {
// Handle the specific exception
} catch (AnotherException e) {
// Handle another specific exception
} finally {
// Code that will always execute, regardless of exception occurrence
}
While this classic method works well in an imperative style, it can lead to cluttered code when dealing with multiple potential exceptions. In functional programming, however, we can encapsulate error handling more elegantly.
Using Optional
for Handling Results
In functional programming, it's common to use types like Optional
, which can represent a value that may or may not be present. This pattern allows you to avoid direct exception handling and work with the possibility of absence instead.
Here’s a basic example:
import java.util.Optional;
public class OptionalExample {
public static Optional<String> findValue(String key) {
// Simulate fetching a value.
if ("validKey".equals(key)) {
return Optional.of("Found Value");
} else {
return Optional.empty();
}
}
public static void main(String[] args) {
Optional<String> result = findValue("validKey");
result.ifPresent(value -> System.out.println("Value: " + value)); // Print if present
System.out.println(result.orElse("Value not found")); // Provide a default if absent
}
}
Why Use Optional
?
- Clarity: It explicitly states that the result may not be present.
- Avoiding Null: It minimizes the risks associated with
NullPointerException
. - Functional Style: Encourages a more functional style of programming, using methods like
map
,flatMap
, andfilter
.
The Either Type for Error Handling
While Optional
is great for missing results, there's another useful construct for error signaling: the Either
type. The Either
type can represent two possible outcomes: a success (e.g., a value) or a failure (e.g., an error). While Java doesn't have a built-in Either
type, we can easily create one.
Here’s how you can implement it:
public class Either<L, R> {
private final L left;
private final R right;
private final boolean isLeft;
private Either(L left, R right, boolean isLeft) {
this.left = left;
this.right = right;
this.isLeft = isLeft;
}
public static <L, R> Either<L, R> left(L value) {
return new Either<>(value, null, true);
}
public static <L, R> Either<L, R> right(R value) {
return new Either<>(null, value, false);
}
public boolean isLeft() {
return this.isLeft;
}
public L getLeft() {
if (isLeft) return left;
throw new RuntimeException("No left value present");
}
public R getRight() {
if (!isLeft) return right;
throw new RuntimeException("No right value present");
}
}
Usage of Either Type
Incorporating this Either
type in function signatures leads to clean, functional error handling, as illustrated below:
public class EitherExample {
public static Either<String, Integer> divide(int numerator, int denominator) {
if (denominator == 0) {
return Either.left("Cannot divide by zero!");
}
return Either.right(numerator / denominator);
}
public static void main(String[] args) {
Either<String, Integer> result = divide(5, 0);
if (result.isLeft()) {
System.out.println("Error: " + result.getLeft());
} else {
System.out.println("Result: " + result.getRight());
}
}
}
Why Use Either?
- Expressiveness: Clearly represents success and failure conditions.
- Flexibility: You can define any type of error message.
- Functional Composition: Facilitates chaining operations in a functional style.
Handling Checked Exceptions
Java's checked exceptions can make working within a functional paradigm cumbersome. However, we can encapsulate these exceptions using Either
for better error handling without compromising the functional style:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class CheckedExceptionExample {
public static Either<Exception, String> readFile(String filePath) {
try {
Path path = Paths.get(filePath);
return Either.right(Files.readString(path));
} catch (Exception e) {
return Either.left(e);
}
}
public static void main(String[] args) {
Either<Exception, String> fileContent = readFile("test.txt");
if (fileContent.isLeft()) {
System.out.println("Error: " + fileContent.getLeft().getMessage());
} else {
System.out.println("File Content: " + fileContent.getRight());
}
}
}
Why Embrace Checked Exceptions in FP?
- Structured error management: Provides a clear approach to signal exceptions within a functional paradigm.
- Ease of reasoning: You can reason about both success and error states uniformly.
In Conclusion, Here is What Matters
Mastering exception handling in functional programming can significantly enhance the robustness and clarity of your code. By leveraging constructs like Optional
and Either
, you can approach error management from a functional perspective, reducing boilerplate code, and making error handling an integrated part of your application flow.
As Java continues to evolve, embracing functional programming paradigms will lead to more maintainable, readable, and resilient code. For further reading on functional programming in Java, check out Java's official documentation on functional interfaces and lambdas.
Keep exploring, keep coding, and remember: handling exceptions is not just about managing errors; it's about enhancing the user experience and delivering quality software. Embrace the power of functional programming and turn exceptions into opportunities for better design!
Checkout our other articles