Mastering Java 9's HttpClient: Common Pitfalls to Avoid
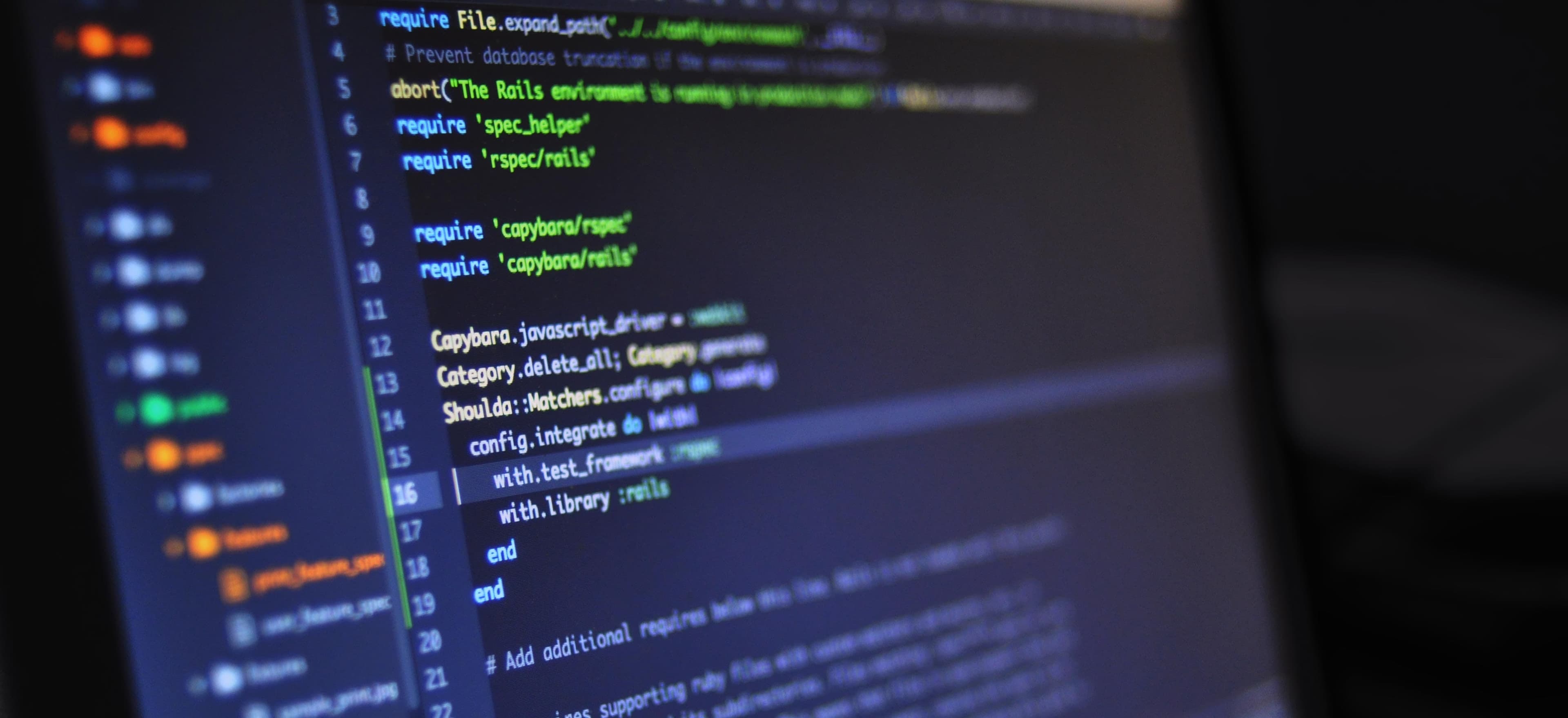
- Published on
Mastering Java 9's HttpClient: Common Pitfalls to Avoid
Java 9 introduced the new HttpClient
API, which set a new standard for sending HTTP requests in a more efficient and flexible manner. The new API supports both synchronous and asynchronous programming models and includes modern HTTP features like WebSocket support. However, as with any new library, users may stumble upon common pitfalls. In this blog post, we'll explore these common issues, showcasing best practices along the way.
Understanding HttpClient
Before diving into pitfalls, it's essential to understand what the HttpClient
offers. Here’s a brief overview:
- Simplicity: It provides a straightforward API for sending and receiving HTTP requests.
- Asynchronous Capabilities: You can perform non-blocking operations.
- HTTP/2 Support: Enhanced performance through multiplexed streams.
Here's a quick snippet to illustrate how to create an instance of HttpClient
:
HttpClient client = HttpClient.newHttpClient();
This is a good starting point, but as we proceed, we will see how to avoid some common issues that developers face.
Common Pitfalls in Using HttpClient
1. Not Handling Exceptions Properly
One of the most critical aspects of working with the HttpClient
is error handling. When you send requests and receive responses, exceptions may occur due to network issues, timeouts, or malformed requests.
Example:
try {
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
// Process the response
} catch (InterruptedException | IOException e) {
e.printStackTrace();
}
Why: It's crucial to handle both InterruptedException
and IOException
. Ignoring these can lead to incomplete operations, leaving your application in an unstable state. Always log or otherwise manage these exceptions gracefully.
2. Ignoring Response Codes
HTTP response codes provide critical information about the success or failure of an HTTP request. Developers sometimes overlook checking them.
Example:
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
if (response.statusCode() != 200) {
System.out.println("Error: " + response.statusCode());
} else {
System.out.println("Success: " + response.body());
}
Why: By ignoring the status code, you may misinterpret the success of your request. For example, a 404 or 500 status can indicate a problem that you need to address.
3. Not Utilizing Asynchronous Capabilities
The HttpClient
API supports asynchronous requests, which can dramatically improve performance, especially in I/O-heavy applications. However, many developers still revert to synchronous calls.
Example:
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println)
.exceptionally(e -> {
e.printStackTrace();
return null;
});
Why: By leveraging asynchronous methods, you can free up resources and keep your application responsive, particularly during high network latency scenarios.
4. Default Connection Timeout
Java's HttpClient
has a default connection timeout period, which can lead to unresponsive behavior if not carefully controlled. Specifically, the client might wait indefinitely for a response.
Example:
HttpClient client = HttpClient.newBuilder()
.connectTimeout(Duration.ofSeconds(10))
.build();
Why: Setting a connection timeout ensures that your application does not hang endlessly on network requests. This is crucial for applications that require reliability.
5. Overlooking SSL Configuration
When dealing with HTTPS requests, it's vital to be aware of default SSL settings. Incorrect SSL configurations can lead to security vulnerabilities.
Example:
To send requests over HTTPS:
HttpClient client = HttpClient.newBuilder()
.version(HttpClient.Version.HTTP_2)
.sslContext(SSLContext.getDefault())
.build();
Why: Ensuring the correct SSL context is critical for the integrity of the data transferred over the network. This protects against potential man-in-the-middle attacks.
6. Starving Threads with Blocking Calls
Since HttpClient
supports both synchronous and asynchronous methods, consider using the asynchronous approach when dealing with multiple requests to avoid blocking resources.
Example:
CompletableFuture<HttpResponse<String>> futureResponse = client.sendAsync(request, HttpResponse.BodyHandlers.ofString());
futureResponse.thenAccept(response -> {
// Process the response
});
Why: Blocking the main thread can lead to an unresponsive application, especially when dealing with high load. Asynchronous processing allows other operations to continue while waiting for a response.
7. Misusing Body Handlers
Choosing the appropriate body handler is crucial for how response data is processed. Developers often default to the ofString
method without considering other options.
Example:
HttpResponse<InputStream> response = client.send(request, HttpResponse.BodyHandlers.ofInputStream());
Why: Using ofInputStream
is more efficient for large content, allowing you to process the response incrementally without loading the entire body into memory.
Best Practices for Using HttpClient
Now that we've covered common pitfalls, let’s encapsulate some best practices to enhance your HttpClient
usage:
- Always Check Response Codes: Assess the status of your response to handle errors appropriately.
- Utilize Asynchronous Features: Enhance application responsiveness by leveraging async calls.
- Implement Proper Exception Handling: Capture and log all exceptions to avoid silent failures.
- Set Timeouts: Always set connection and read timeouts suitable for your application.
- Be Mindful of SSL: Secure your connections with the right SSL configurations.
- Choose Suitable Body Handlers: Optimize your response processing strategy with the right body handlers.
To Wrap Things Up
Mastering the HttpClient
in Java 9 goes beyond simply sending requests and receiving responses. By being aware of common pitfalls and adopting best practices, you can build robust and efficient applications.
For more information on Java's HttpClient
, you can check the official Java Documentation.
Further Reading
By carefully navigating these common pitfalls, you will harness the full potential of Java 9's HttpClient
, creating efficient and reliable applications.
Checkout our other articles