Common Pitfalls in Layered Architecture for Spring Boot
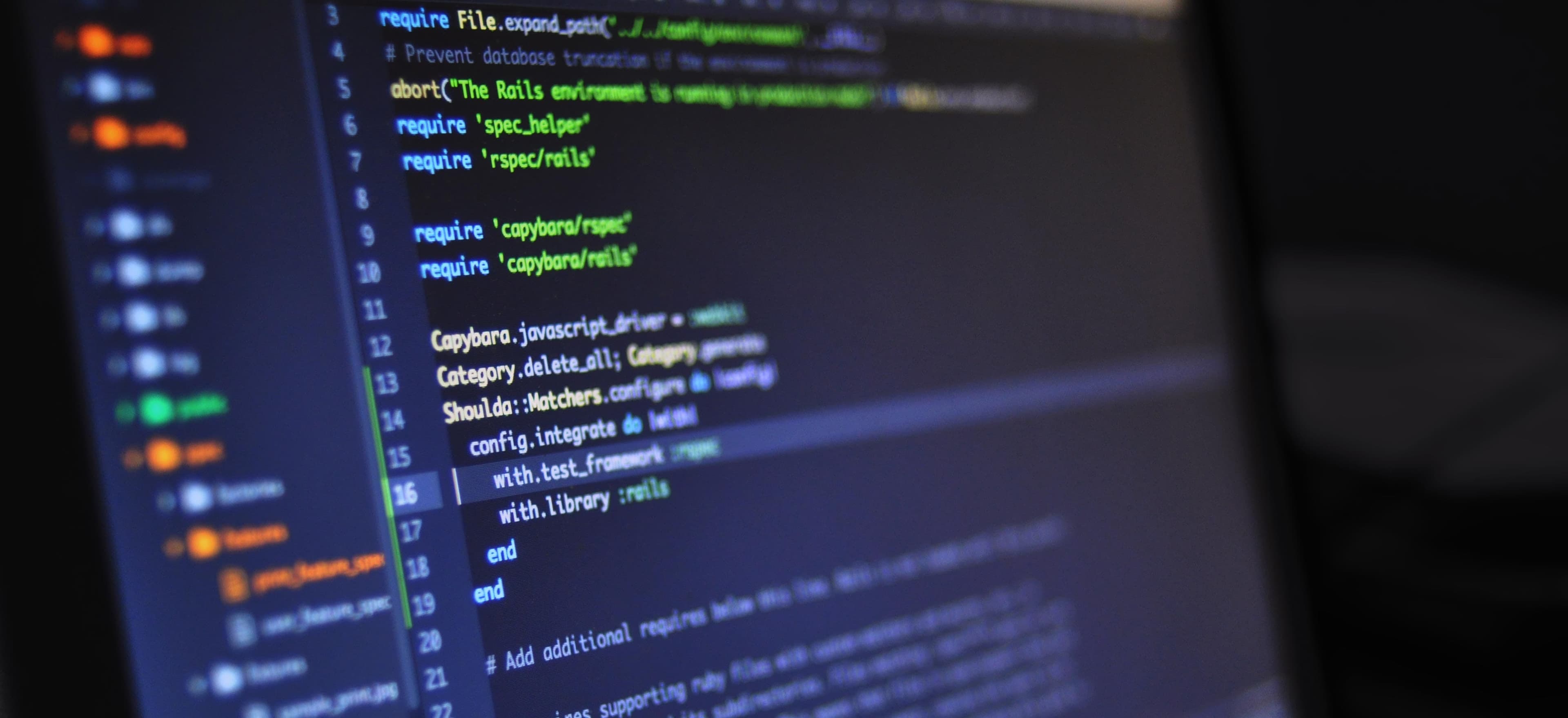
- Published on
Common Pitfalls in Layered Architecture for Spring Boot
Layered architecture is a widely adopted architectural pattern in software development, particularly in frameworks like Spring Boot. It allows developers to separate concerns, promote reusability, and enhance maintainability. However, despite its principles, developers often encounter various pitfalls while implementing this architecture. This blog post will delve into common mistakes made in layered architecture for Spring Boot applications, elucidating how to avoid them for a more robust and efficient codebase.
Understand the Layers
Before we explore these pitfalls, let's clarify the main layers in a typical Spring Boot application:
- Presentation Layer (Controllers)
- Service Layer (Business Logic)
- Data Access Layer (Repositories)
Each layer has its responsibility. The presentation layer handles user inputs and outputs, the service layer processes these inputs, and the data access layer manages database operations.
Common Pitfalls
1. Ignoring Separation of Concerns
One of the core principles of layered architecture is separation of concerns. However, developers often blend responsibilities, leading to bloated classes and decreased maintainability.
Why This Matters: Mixing responsibilities makes the code harder to understand. Changes in one area may inadvertently affect another, leading to bugs.
Example: Let's consider a controller that contains both business logic and data access.
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
// Fetching users directly in the controller - violating separation of concerns
return userRepository.findAll();
}
}
Solution: The above example should be modified to extract the data access into a service layer.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
Now, the controller is only responsible for handling requests and responses, delegating business logic to the service layer.
2. Overusing Annotations
Spring Boot offers a plethora of annotations to simplify development. However, overusing annotations can lead to obfuscation and confusion.
Why This Matters: Annotations can sometimes hide the actual workings of the code, making it difficult for new developers to grasp the functionality quickly.
Example: Excessively chaining multiple annotations on a single method can reduce readability.
@GetMapping
@CrossOrigin
@PreAuthorize("hasRole('USER')")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
Solution: Keep annotations simple or segment the methods for better clarity.
3. Tight Coupling Between Layers
Creating a tightly coupled relationship between layers can hinder flexibility. A change in the service layer may require changes in the controller layer, which can affect the overall architecture.
Why This Matters: This undermines the advantages of using a layered architecture, particularly reusability and testability.
Example: A controller directly invoking repository methods instead of service methods can lead to tight coupling.
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userRepository.findById(id).orElseThrow(() -> new ResourceNotFoundException("User not found"));
}
Solution: Always use service methods to decouple these layers.
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id); // Using service layer to decouple
}
4. Neglecting Exception Handling
Exception handling is critical in any application. Neglecting this aspect leads to unhandled exceptions, which can expose inner workings or mislead clients.
Why This Matters: Proper exception handling helps in understanding the flow of execution and ensures that users receive meaningful feedback.
Example: Ignoring error handling in controller methods can lead to obscure error messages.
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id); // No error handling
}
Solution: Implement global exception handling using @ControllerAdvice
.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
return new ResponseEntity<>(new ErrorResponse(ex.getMessage()), HttpStatus.NOT_FOUND);
}
}
5. Data Access Logic in Service Layer
While it's vital to access data via the service layer, putting too much data access logic into your service layer violates the data access layer's purpose.
Why This Matters: This leads to services being overly complex and difficult to test.
Example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getActiveUsers() {
// Data query logic belongs in the repository
return userRepository.findAll().stream()
.filter(User::isActive)
.collect(Collectors.toList());
}
}
Solution: Move specific data access logic back into the repository layer.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByActiveTrue();
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getActiveUsers() {
return userRepository.findByActiveTrue();
}
}
6. Not Using DTOs (Data Transfer Objects)
DTOs allow you to control the data that travels between your layers. Not using DTOs can expose the internal structure of your entity models, leading to security risks and unnecessary data transmission.
Why This Matters: This can lead to performance issues and over-exposing data that should remain internal.
Example: Returning an entity directly from a controller.
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id); // Exposing the entity directly
}
Solution: Incorporate DTOs.
public class UserDTO {
private Long id;
private String username;
// Additional fields as required
}
@GetMapping("/{id}")
public UserDTO getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
return convertToDTO(user);
}
// Utility Method
private UserDTO convertToDTO(User user) {
UserDTO dto = new UserDTO();
dto.setId(user.getId());
dto.setUsername(user.getUsername());
return dto;
}
7. Inconsistent Naming Conventions
In large applications, maintaining consistent naming conventions across layers is crucial. Discrepancies can confuse new developers and team members.
Why This Matters: Consistent naming improves readability and maintainability.
Example: A service named UserManager
and a repository called UserPersistence
.
Solution: Standardize naming conventions, such as using the suffix -Service for service classes and -Repository for repository classes.
My Closing Thoughts on the Matter
Adopting a layered architecture in Spring Boot can significantly enhance the quality and maintainability of your applications. However, it's essential to be mindful of the common pitfalls discussed in this article. By ensuring proper separation of concerns, avoiding tight coupling, and adhering to effective naming conventions and exception handling practices, you can create a more robust codebase.
By actively implementing these suggestions and regularly reviewing your architecture, you can build resilient applications that are easier to maintain and scale over time.
For more resources on Spring Boot and layered architecture, consider visiting Spring's Documentation or Baeldung on Spring Boot.
Happy coding!