Common Pitfalls When Using JPA for Database Views
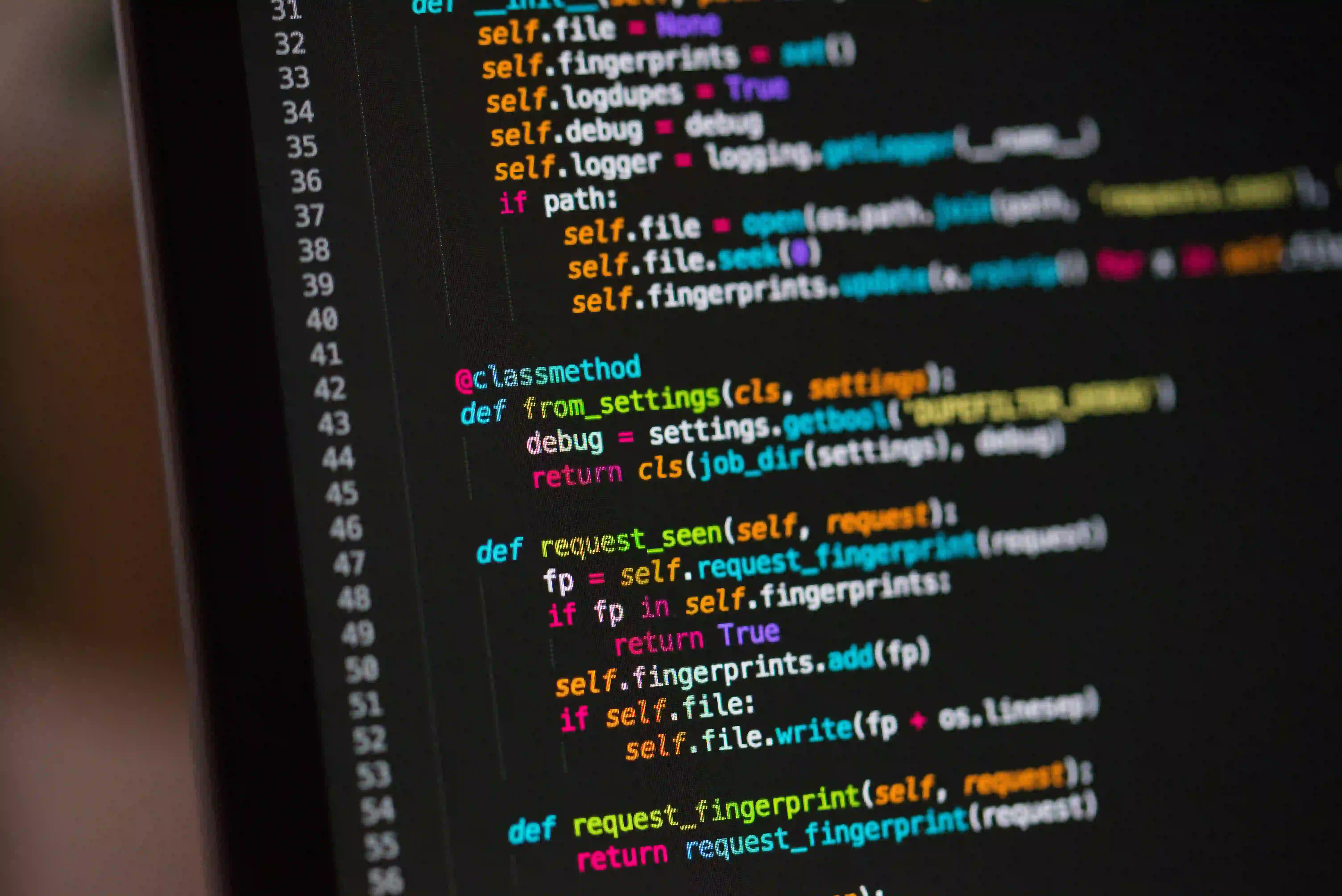
Common Pitfalls When Using JPA for Database Views
Java Persistence API (JPA) simplifies data layer development for Java applications, enabling developers to work with databases using Java objects. While JPA is powerful and flexible, using it with database views can lead to several pitfalls. This blog post discusses these common pitfalls and how to navigate them, ensuring your application runs smoothly and efficiently.
Understanding Database Views and JPA
Before diving into common pitfalls, it's important to clarify what database views are. A database view is a virtual table that provides a way to present data from one or more tables in a specific format without storing it separately. Views can simplify complex queries and allow for modular data access.
When using JPA with database views, it is essential to understand both aspects of the technology, as improper use can lead to performance degradation, data inconsistency, or even application crashes. Here are some common pitfalls in leveraging JPA with database views and ways to avoid them.
Pitfall 1: Inadequate Mapping of Views
One of the critical steps in using JPA with views is mapping the view properly. JPA requires annotations to bind Java object fields with database table/view columns.
Solution: Use @Entity and @SqlResultSetMapping
When mapping views, make sure you use the @Entity
annotation correctly. Also, you can create a @SqlResultSetMapping
if the view returns a complex structure.
@Entity
@SqlResultSetMapping(
name = "MyViewMapping",
columns = {
@ColumnResult(name = "column1"),
@ColumnResult(name = "column2")
}
)
public class MyView {
@Id
private Long id;
private String column1;
private String column2;
// Getters and setters
}
Why This Matters: Proper mapping ensures that JPA can interact with the database view correctly. Neglecting this can lead to misalignment in data retrieval, causing runtime exceptions.
Pitfall 2: Read-Only Data Operations
Database views are often used to encapsulate complex queries for read operations. By default, JPA treats entity classes mapped to views as read-write, which can lead to unexpected behavior when updates are attempted.
Solution: Set Views to Read-Only
You can configure your entity to be read-only by using the @Immutable
annotation.
@Entity
@Immutable
public class MyView {
// Fields, constructors, getters, and setters
}
Why This Matters: Setting views to read-only prevents accidental data modifications, especially when working with complex queries, ensuring that your application adheres to the intended purpose of the database view.
Pitfall 3: Ignoring Query Optimization
While JPA offers great facilities for developing queries, it can be inefficient if you do not implement it wisely, especially when querying views.
Solution: Write Efficient JPQL Queries
JPQL
(Java Persistence Query Language) can be used to navigate views, but writing an inefficient query can lead to significant performance degradation. Consider using the SELECT
statement judiciously.
@Query("SELECT v FROM MyView v WHERE v.column1 = :value")
List<MyView> findViewsByColumn1(@Param("value") String value);
Why This Matters: Efficient queries reduce the load on the database and enhance performance. An optimized query ensures that it fetches the necessary data quickly.
Pitfall 4: Confusion between Tables and Views
Developers may forget that views do not behave exactly like tables. When you attempt to perform DML (Data Manipulation Language) operations, you may face unexpected behaviors.
Solution: Focus on Read-Only Operations
Always design your application logic expecting the view's nature as a read-only construct. Using it for operations like MERGE
, DELETE
, or UPDATE
can introduce inconsistency.
public void updateViewData(MyView view) {
// This operation is not applicable for database view
throw new UnsupportedOperationException("Cannot update a view");
}
Why This Matters: Knowing that views should not be treated like tables helps avoid runtime errors and maintain data integrity.
Pitfall 5: Lazy vs Eager Loading
Using JPA’s fetch strategies can lead to complexities when accessing views, especially when dealing with large datasets.
Solution: Use Fetch Type Appropriately
When you define your entity, consider whether to load associated entities eagerly or lazily.
@Entity
public class MyView {
@OneToMany(fetch = FetchType.LAZY)
private List<AnotherEntity> relatedEntities;
// Fields, getters, and setters
}
Why This Matters: Choosing the right fetch strategy helps in balancing memory efficiency and application performance. Lazy loading is often more efficient as it delays loading until absolutely necessary, reducing data transfer and memory usage.
Pitfall 6: Lack of Testing and Validation
When integrating views into your application, many developers skip the crucial stages of testing and validation, leading to undetected issues.
Solution: Implement Unit Tests
Integrate unit tests that validate your JPA queries against views. Utilize testing frameworks like JUnit or TestNG for solid coverage.
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyViewTests {
@Autowired
private MyViewRepository repository;
@Test
public void testFindByColumn1() {
List<MyView> views = repository.findViewsByColumn1("testValue");
assertFalse(views.isEmpty());
}
}
Why This Matters: Testing helps catch issues early in the development cycle, ensuring the application functions as intended with database views.
The Closing Argument
Working with JPA and database views can greatly enhance your application's architecture and performance when done correctly. Marking entities as read-only, optimizing queries, understanding the limitations and behaviors of views, and ensuring thorough testing can prevent many pitfalls.
By keeping these considerations in mind, you can leverage JPA to its fullest potential, efficiently utilizing database views for your application's needs.
For more information on database views, check out Oracle's Database Views Documentation and JPA's Official Documentation.
Happy coding!