Is Your Technical Debt Slowly Sinking Your Project?
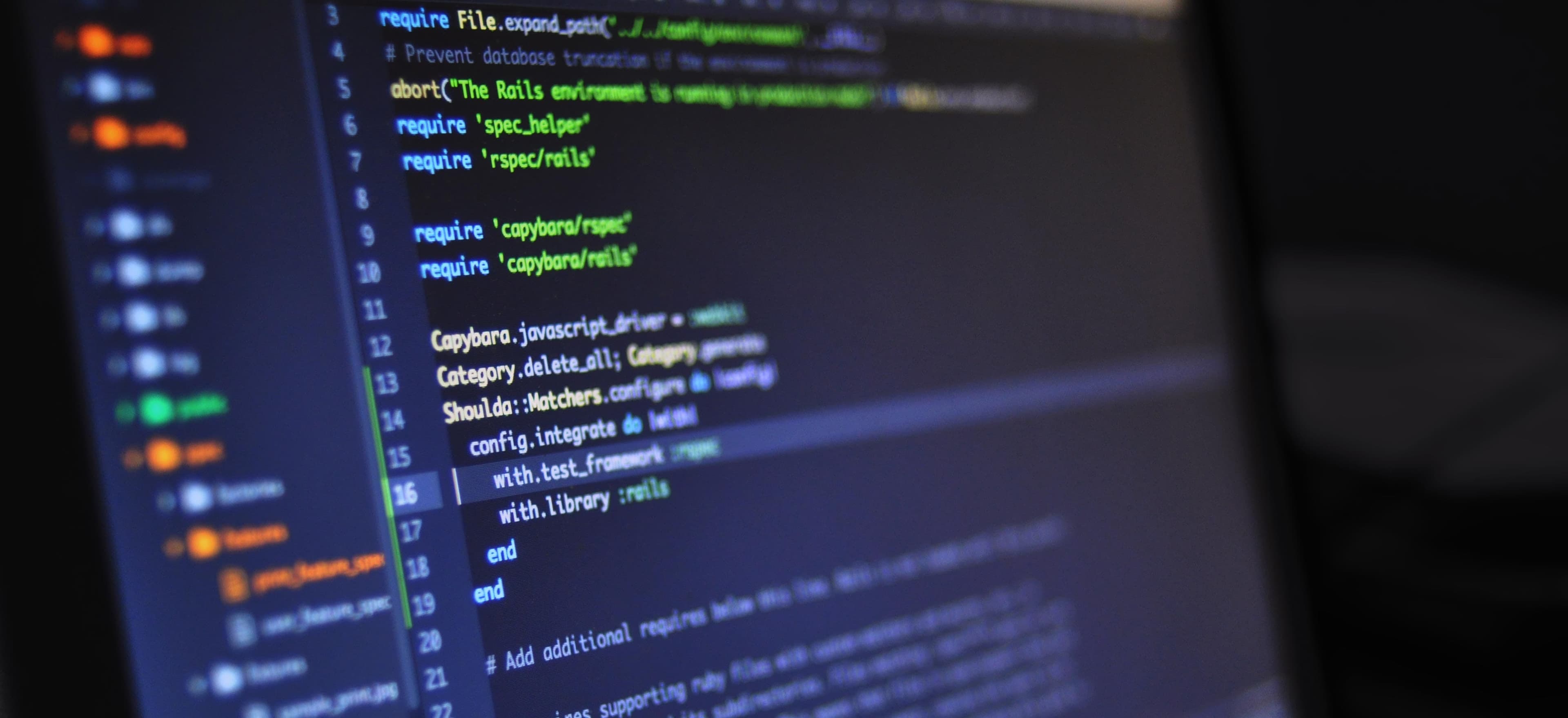
- Published on
Is Your Technical Debt Slowly Sinking Your Project?
In today's fast-paced software development world, meeting deadlines and delivering features quickly is often prioritized over maintaining clean code. As a result, technical debt accumulates, which can jeopardize a project's long-term sustainability. In this blog post, we will explore the concept of technical debt, its implications in Java projects, how to measure and manage it, and best practices to reduce its impact.
What is Technical Debt?
Technical debt is a metaphor introduced by Ward Cunningham to describe the consequences that come from choosing an easy, limited, or temporarily expedient solution rather than using a better approach that would take longer. The term suggests that, just like financial debt, technical debt can accrue "interest" over time, leading to higher costs and complications down the road.
Types of Technical Debt
-
Deliberate Debt: This occurs when teams opt for a quicker solution with the intention of refactoring later. It's often a trade-off between immediate delivery and long-term quality.
-
Accidental Debt: This is caused by a lack of knowledge or experience, leading to decisions that result in suboptimal implementations.
-
Bit Rot: Over time, systems can degrade due to changing requirements and the inability to adapt code that wasn't designed for flexibility.
Understanding these types of debt is crucial for effectively managing your project.
Why is Technical Debt a Problem?
While taking on technical debt may seem beneficial in the short term, it introduces several challenges:
-
Increased Complexity: As code accumulates shortcuts and workarounds, overall complexity increases, making it more difficult to understand and manage.
-
Reduced Productivity: Developers spend more time fixing bugs and understanding code than delivering new features.
-
Higher Costs: The longer technical debt remains in the system, the more expensive it becomes to resolve it.
-
Corporate Reputation: Companies known for delivering unstable or low-quality software may suffer long-term reputational damage.
The Cost of Ignoring Technical Debt
Many teams assume they can simply push technical debt aside, but allowing it to accumulate can hinder innovation and lead to project failure. It is vital to recognize that unresolved debt can stifle a team's ability to pivot and adapt to shifting markets or user requirements.
Recognizing Technical Debt in Your Java Project
Understanding how to recognize technical debt in a Java project is the first step to addressing it. Here are some signs to look out for:
-
Code Quality: Over-reliance on code smells, meaning parts of the codebase that indicate deeper problems (e.g., long methods, duplicated code).
-
Test Coverage: Low unit test coverage indicates that the code is fragile and untested, resulting in unanticipated issues during maintenance.
-
Lack of Documentation: Poorly documented code can lead to misunderstandings and miscommunications among team members.
-
Frequent Bugs: If bugs are frequently reported, it's likely that there are underlying issues that need to be addressed.
Measuring Technical Debt
To manage technical debt efficiently, you should measure it. One popular approach is using a scorecard driven by metrics such as:
-
Code Complexity: Tools like SonarQube can help evaluate the complexity of your code.
-
Code Duplication: Look for duplicated code blocks that could be refactored into reusable methods.
-
Unit Test Coverage: Measure how much of your code is tested, with tools such as Jacoco for Java.
public class Calculator {
// Calculate the sum of two numbers
public int add(int a, int b) {
return a + b;
}
}
Commentary on This Code
- The method
add
is simple and clear, but what if we needed to perform 20 different mathematical operations? As we add more methods to the class, we risk creating a chaotic class structure that can be hard to maintain.
Strategies for Managing Technical Debt
To manage and mitigate technical debt in your Java projects, consider the following strategies:
1. Prioritize Refactoring
Make refactoring part of your workflow. Dedicate specific time during development sprints for refactoring efforts. This allows you to address technical debt proactively rather than reactively.
public class Calculator {
// This method is a candidate for refactoring because it only handles add operation
// In the future, more operations may be needed, consider using interfaces.
}
// Improved Implementation
public interface Operation {
int execute(int a, int b);
}
public class Addition implements Operation {
@Override
public int execute(int a, int b) {
return a + b;
}
}
2. Emphasize Code Reviews
Code reviews are an excellent way to identify technical debt. Ensure team members provide constructive feedback during the review process. This not only helps catch issues but fosters a shared responsibility culture for code quality.
3. Maintain Documentation
Encourage developers to keep documentation up to date. If code changes, documentation should reflect those changes and should be easy to read and understand.
4. Automated Testing
Automated testing is essential. Use frameworks like JUnit to write unit tests for your Java methods. Higher test coverage helps ensure that changes do not introduce new bugs—a common issue in debt-laden codebases.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Commentary on Testing
- This JUnit test verifies that the
add
method works as expected. As you add more methods, ensure that each has corresponding unit tests.
5. Create a Debt Management Plan
Treat technical debt like financial debt; have a clear plan for how you'll manage it. Prioritize your debts and focus on paying them off in a timely manner to avoid accruing more interest (i.e., more problems).
Final Considerations: Mitigating Technical Debt for a Healthier Project
Technical debt is an unavoidable aspect of software development, but it doesn't have to be a project-killer. By prioritizing code quality, implementing robust testing practices, and maintaining open lines of communication within your team, you can manage your debt effectively.
It requires commitment and a proactive approach, but the payoff can be significant: reduced costs, increased productivity, and a healthier, more sustainable software project. Embrace technical debt management as an essential part of your agile process.
For further reading on technical debt, consider checking out Martin Fowler’s insights or the resource on code quality provided by SonarSource.
Happy coding!
Checkout our other articles