Customizing HTTP Error Messages with JAX-RS and Jersey
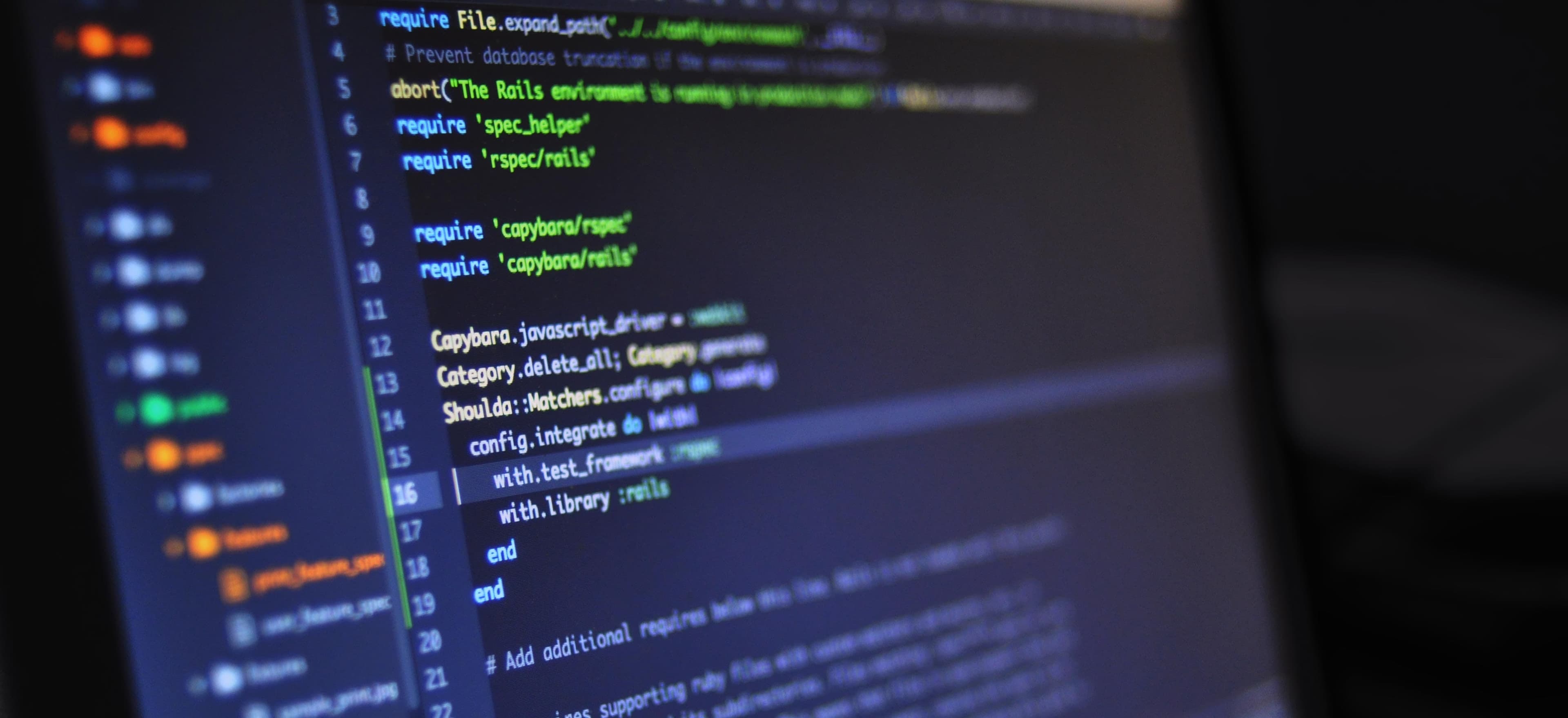
- Published on
Customizing HTTP Error Messages with JAX-RS and Jersey
When building RESTful services with JAX-RS and Jersey, one major aspect of improving user experience is customizing HTTP error messages. This customization allows for clearer communication with users or clients about what went wrong, making your API more user-friendly and more maintainable.
In this article, we will explore how to customize error responses in a JAX-RS application, focusing on Jersey as the implementation. We will cover the following key areas:
- Understanding the role of HttpResponse.
- Implementing a custom exception mapper.
- Configuring global error handling.
- Practical examples and code snippets.
Understanding HTTP Status Codes and Error Responses
HTTP status codes are an essential part of any web service, acting as the initial communication from the server to the client. A well-defined error message can offer context about the server's state and potential remedies. Common status codes include:
- 400 Bad Request - the server cannot process the request due to a client error.
- 404 Not Found - the requested resource could not be found.
- 500 Internal Server Error - the server encountered an unexpected condition.
In a world driven by API first strategies, a clean and informative error response can mean the difference between a positive user experience and frustration.
Implementing a Custom Exception Mapper
One of the most effective ways to customize HTTP error responses in JAX-RS is by implementing a custom ExceptionMapper. This allows you to catch specific exceptions thrown within your application and provide a tailored JSON response to the client.
Step 1: Create Custom Exception
First, let’s create a custom exception that we want to handle. For example:
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
Here, we are defining a simple runtime exception that includes a message indicating what resource was not found.
Step 2: Create an Exception Mapper
Next, we implement the ExceptionMapper interface to customize how the application handles this exception:
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class ResourceNotFoundExceptionMapper implements ExceptionMapper<ResourceNotFoundException> {
@Override
public Response toResponse(ResourceNotFoundException exception) {
return Response.status(Response.Status.NOT_FOUND)
.entity(new ErrorResponse("Resource Not Found", exception.getMessage()))
.build();
}
}
In this implementation, we catch the ResourceNotFoundException
and respond with a 404 status code. The ErrorResponse
class (which we will define next) encapsulates the error details.
Step 3: Define Error Response Object
We will create an ErrorResponse class to structure our error messages:
public class ErrorResponse {
private String error;
private String message;
public ErrorResponse(String error, String message) {
this.error = error;
this.message = message;
}
// Getters
public String getError() {
return error;
}
public String getMessage() {
return message;
}
}
This structure allows us to provide a consistent way to send error messages back to the client, giving them useful context about the nature of the error.
Configuring Global Error Handling
While custom exception mappers are excellent for specific cases, there may be times where you want a fallback for uncaught exceptions. You can create a global exception handler.
Global Exception Mapper
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class GenericExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable throwable) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity(new ErrorResponse("Internal Server Error", "An unexpected error occurred"))
.build();
}
}
This generic exception mapper will catch any uncaught exceptions and return a 500 response with a general error message.
Putting It All Together
Now that we have created our custom exception and exception mappers, it’s time to see them in action.
For example, suppose we have a REST endpoint to fetch a user:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/users")
public class UserResource {
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public User getUser(@PathParam("id") String id) {
User user = userService.findUserById(id);
if (user == null) {
throw new ResourceNotFoundException("User with ID " + id + " not found.");
}
return user;
}
}
In this method, when a user is not found, we throw our ResourceNotFoundException
. Our custom exception mapper will handle this exception and return a friendly error message formatted as JSON.
Testing the Error Responses
To illustrate the behavior of our newly created error handling, we can use tools like Postman or cURL to simulate requests.
-
Simulating a valid request:
curl -X GET http://localhost:8080/api/users/1
-
Simulating a not found request:
curl -X GET http://localhost:8080/api/users/99
For the second command, you should receive a 404 response in JSON format:
{
"error": "Resource Not Found",
"message": "User with ID 99 not found."
}
In Conclusion, Here is What Matters
Customizing HTTP error messages is crucial for building robust, user-friendly RESTful services with JAX-RS and Jersey. By implementing custom exception mappers, we can provide detailed and informative responses tailored to specific scenarios.
The approach outlined in this article can significantly enhance the API's usability and facilitate easier debugging and maintenance.
For further reading and examples regarding JAX-RS and Jersey, you might find the following resources helpful:
By implementing and customizing HTTP error messages, you take a significant step towards building an effective and user-oriented API.
Checkout our other articles