Understanding EJB 3.x Lifecycle Pitfalls in Concurrency Handling
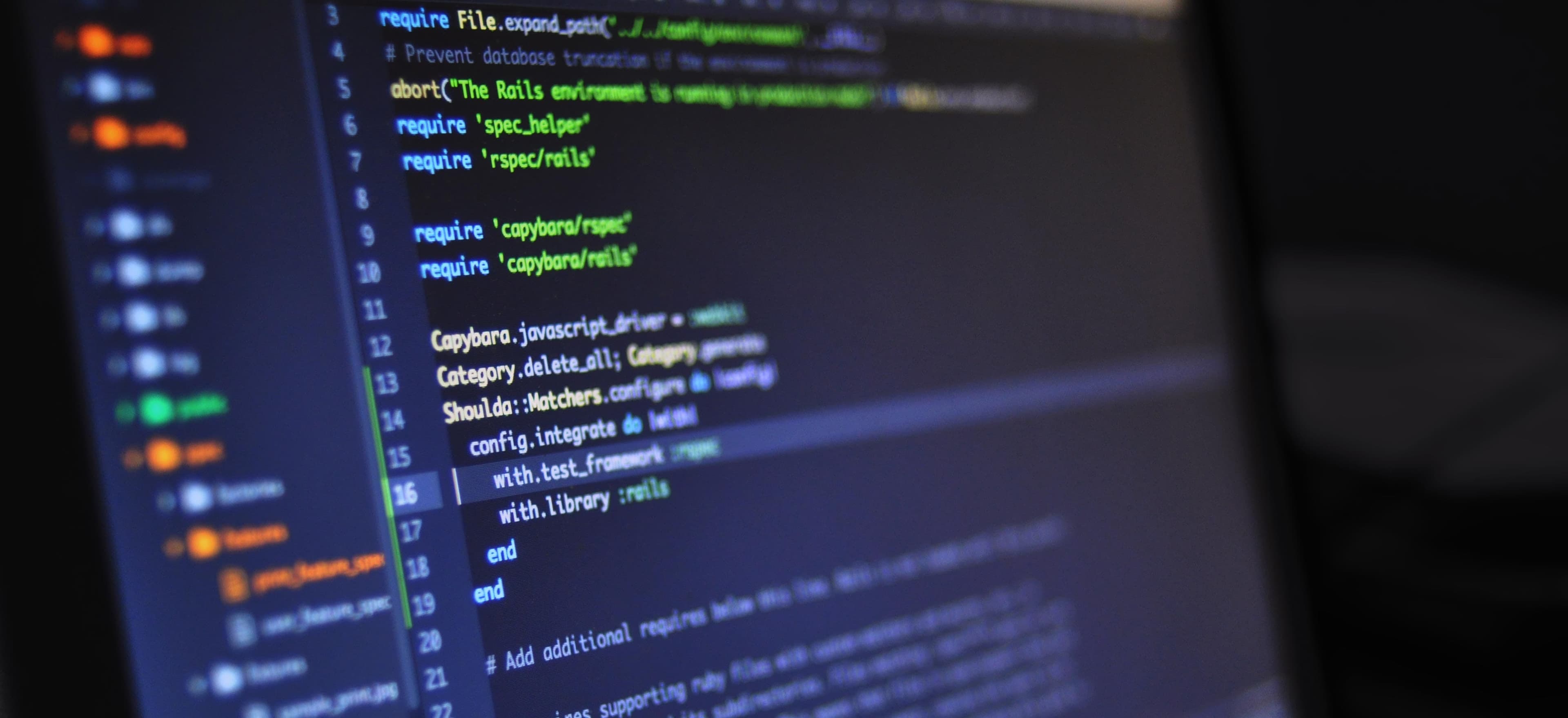
- Published on
Understanding EJB 3.x Lifecycle Pitfalls in Concurrency Handling
Java EE (Enterprise Edition) has provided us with several robust frameworks for enterprise application development. Among these, Enterprise JavaBeans (EJB) 3.x has emerged as a cornerstone for creating scalable, transactional, and multi-threaded applications. However, when dealing with EJBs, one area that frequently raises concerns is concurrency handling.
In this blog post, we'll dive into the lifecycle of EJB 3.x and explore the potential pitfalls you may encounter with concurrency management. Specifically, we will cover:
- EJB Lifecycle Overview
- The Concurrency Problem
- EJB Annotations and Concurrency Control
- Practical Code Examples
- Best Practices for Avoiding Concurrency Issues
- Conclusion
EJB Lifecycle Overview
Before we plunge into concurrency issues, it’s essential to grasp the EJB lifecycle. The EJB container manages the lifecycle of beans, allowing developers to focus on business logic rather than resource management.
The EJB lifecycle can be summarized in the following phases:
- Instantiation: The EJB container creates an instance of the bean class.
- Dependency Injection: Required resources and dependencies are resolved and injected.
- Post-Construct: Any initialization logic defined in the
@PostConstruct
annotated method is executed. - Business Method Invocation: The bean is ready to respond to client requests.
- Passivation: The bean is temporarily removed from memory.
- Activation: A passivated bean is reactivated and becomes active again.
- Destruction: Finally, when the bean is no longer needed, the container invokes the
@PreDestroy
annotated method, and the instance is removed.
Understanding this lifecycle is crucial as it impacts how we handle concurrency.
The Concurrency Problem
Concurrency occurs when two or more processes access shared resources in an unpredictable manner. In a multi-user environment, it leads to inconsistent states, data corruption, and various unexpected behavior.
Consider a scenario:
- Scenario: An EJB manages user account balances. If two transactions try to update the balance simultaneously, the final state may not reflect both updates correctly.
Concurrency Issues Overview
- Lost Updates: When two or more transactions read the same data and update it without knowledge of each other, leading to lost changes.
- Dirty Reads: A transaction reads uncommitted changes made by another transaction.
- Non-repeatable Reads: A transaction sees different values for a specific record if read multiple times within the same transaction.
EJB Annotations and Concurrency Control
EJB 3.x provides several annotations for managing concurrency in enterprise applications. The key annotations include:
@Lock
: Defines the concurrency strategy.@TransactionAttribute
: Specifies transaction behavior.
Locking Types
EJB containers can manage their concurrency using different locking strategies defined via the @Lock
annotation. There are two lock types:
- @Lock(LockType.WRITE): Only one client can access the bean at a time, which effectively serializes access to business methods.
- @Lock(LockType.READ): Multiple clients can read concurrently but not write.
Here’s how you could implement it:
import javax.ejb.Lock;
import javax.ejb.LockType;
import javax.ejb.Stateless;
@Stateless
public class AccountBean {
private double balance;
@Lock(LockType.WRITE)
public void deposit(double amount) {
balance += amount;
}
@Lock(LockType.WRITE)
public void withdraw(double amount) throws Exception {
if (amount > balance) {
throw new Exception("Insufficient balance");
}
balance -= amount;
}
@Lock(LockType.READ)
public double getBalance() {
return balance;
}
}
Why This Code?
- Thread Safety: By applying
@Lock(LockType.WRITE)
to thedeposit
andwithdraw
methods, you ensure that only one transaction can change the account balance at a time. The@Lock(LockType.READ)
annotation ongetBalance
allows multiple threads to read the balance without interfering with each other, enhancing performance.
Practical Code Example
Let’s create a simple EJB to demonstrate the issues and solutions to concurrency. The example below implements an Account EJB with both transaction and locking controls.
import javax.ejb.Lock;
import javax.ejb.LockType;
import javax.ejb.Stateless;
@Stateless
public class BankAccount {
private double balance;
@Lock(LockType.WRITE)
public void deposit(double amount) {
balance += amount;
System.out.println("Deposited: " + amount + "; New Balance: " + balance);
}
@Lock(LockType.WRITE)
public void withdraw(double amount) throws Exception {
if(amount > balance) {
throw new Exception("Insufficient funds");
}
balance -= amount;
System.out.println("Withdrew: " + amount + "; New Balance: " + balance);
}
@Lock(LockType.READ)
public double getBalance() {
return balance;
}
}
Why Use This Example?
- Clear Application of Locking: The EJB uses appropriate locking to ensure that simultaneous deposits and withdrawals do not compromise data integrity.
- Comprehensible Output: Console logging illustrates the state of the account after each transaction, making it easier to debug.
By adopting such straightforward practices, we can mitigate concurrency issues effectively.
Best Practices for Avoiding Concurrency Issues
-
Use the Right Locking Strategy: Choose between READ and WRITE locks wisely to enhance performance while ensuring data integrity.
-
Stateless vs Stateful Beans: Avoid using stateful beans when possible. Stateless beans do not maintain state across multiple calls, reducing the risk of concurrency pitfalls.
-
Transactions: Always leverage container-managed transactions. Ensure that related operations occur within a single transactional context.
-
Optimistic vs Pessimistic Locking: Depending on your use case, consider using optimistic locking with versioning for scenarios with high-read and low-write operations. Pessimistic locking can be useful in contexts with frequent updates.
-
JPA and Entity Beans: If you are using JPA, be cautious with entity beans. Ensure that concurrent updates are managed properly to avoid stale data.
Final Thoughts
Concurrency management in EJB 3.x requires a comprehensive understanding of the lifecycle and the available tools for maintaining consistency. By using appropriate locking mechanisms and adhering to best practices, we can minimize risks and create robust, concurrent enterprise applications.
To deepen your understanding of EJB and concurrency, feel free to explore the official Java EE documentation, which offers further insights.
By staying vigilant and proactive about concurrency handling, you'll build applications that not only perform well but also require less debugging and maintenance in the long run. Remember, a good application is not just about features; it’s about reliability and stability, especially in a multi-threaded environment.
We hope this exploration into the pitfalls of EJB 3.x lifecycle concerning concurrency has been enlightening. As you develop your applications, keep these principles close, and you will navigate through concurrency challenges with greater finesse. Happy coding!
Checkout our other articles