Taming Legacy Code: Strategies for Modern Developers
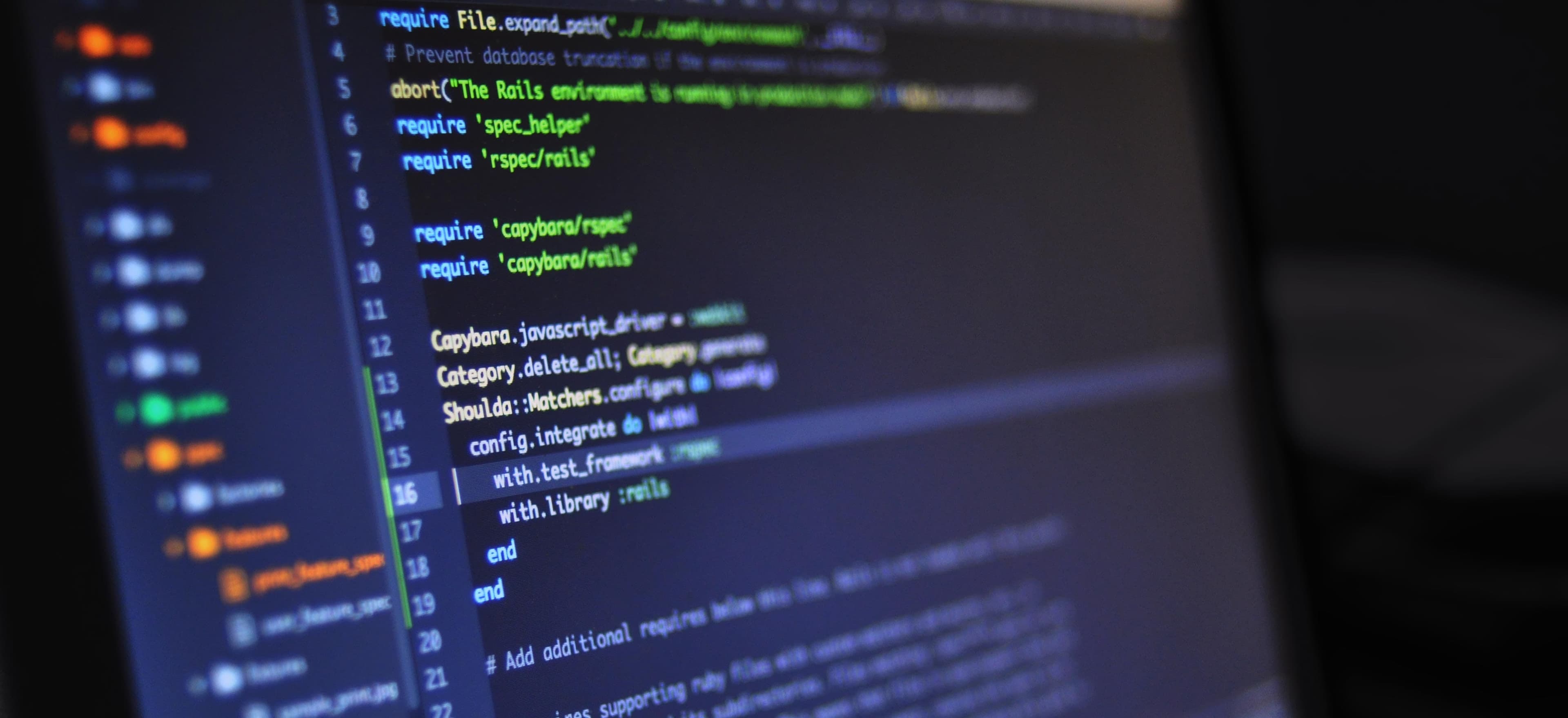
- Published on
Taming Legacy Code: Strategies for Modern Developers
Legacy code can be both daunting and exhilarating. For many developers, dealing with legacy code is a rite of passage—a necessary step in becoming proficient in the craft. While legacy systems often come with a host of challenges, they also represent valuable knowledge and history. In this blog post, we’ll explore effective strategies to handle legacy code, aiding developers in transforming seemingly archaic systems into manageable, modern, and maintainable codebases.
Understanding Legacy Code
In our context, legacy code refers to systems or code that are outdated but still in use, often lacking proper documentation or tests. This code can be written in older programming languages, frameworks, or styles that are not in line with contemporary practices.
Why Legacy Code Matters
- Business Value: Legacy code can hold critical business logic and data that is irreplaceable.
- Learning Opportunity: Working with legacy systems can provide valuable insights into the evolution of coding practices and architectures.
The Challenges of Legacy Code
- Lack of Documentation: Many legacy systems come with little to no documentation, making it hard to understand.
- No Tests or Outdated Tests: Without tests, large refactoring efforts become risky.
- Dependencies on Obsolete Technologies: Legacy systems may rely on outdated libraries or frameworks, increasing maintenance costs.
Strategies for Managing Legacy Code
-
Identify and Prioritize
Before diving headfirst into the code, it’s essential to identify what parts of the codebase need your immediate attention.
- Conduct a Code Review: This involves analyzing the code to find potential pain points that could hinder your project's progress.
- Prioritize by Impact: Focus on areas of the code that have a high impact on your system's functionality or performance.
Example of a Code Review Checklist
// Checklist to review legacy code public class CodeReviewChecklist { public static void main(String[] args) { boolean isWellDocumented = false; // Check for comments and documentation boolean hasTests = false; // Verify if tests exist boolean usesCurrentLibraries = false; // Check for outdated libraries if (!isWellDocumented || !hasTests) { System.out.println("Focus on documenting and adding tests."); } if (usesCurrentLibraries) { System.out.println("Consider refactoring to use modern libraries."); } } }
The above code serves as a simplistic representation of a source of evaluation. Instead of diving into problem areas right away, ensure that you assess the codebase exhaustively.
-
Incremental Refactoring
Refactoring legacy code does not have to happen all at once. Instead, focus on small, manageable changes that can incrementally improve the codebase.
- Apply the Boy Scout Rule: "Leave the camp cleaner than you found it." This means modifying the code to improve its structure whenever you touch it, even in small ways.
Example of Incremental Refactoring
Before:
public void processUser(User user) { if(user.getName() != null){ System.out.println(user.getName()); } }
After refactoring:
public void processUser(User user) { String userName = user.getName(); if(userName != null){ printUserName(userName); } } private void printUserName(String name) { System.out.println(name); }
In the above example, the method has been improved by delegating responsibility to
printUserName
. This enhances readability and maintainability. -
Testing
One of the best defenses against legacy code is a solid suite of automated tests. Build your test coverage step by step.
- Write Tests Before Refactoring: Whenever possible, write tests before making substantial changes. This enables you to detect any issues introduced by your refactoring efforts.
Example of a Simple Unit Test
import static org.junit.jupiter.api.Assertions.*; public class UserTest { @Test public void testUserNameNotNull() { User user = new User(null); assertThrows(IllegalArgumentException.class, () -> { user.processUser(user); }); } }
Not only do unit tests provide you with a safety net for your code, but they also increase developers' confidence in the codebase.
-
Utilizing Dependency Management Tools
Legacy code often depends on outdated libraries. Use modern dependency management tools to identify and resolve these issues.
- Maven/Gradle for Java: These tools can help in managing libraries effectively. They enable you not only to include the latest versions but also to automate dependency updates.
Example of a Simple Maven Dependency
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.10</version> </dependency>
Including updated dependencies can significantly enhance your code quality and security.
-
Implementing Code Reviews and Pair Programming
Subjecting your code to peer review can be an eye-opener. Code reviews ensure that the knowledge is shared among team members while improving code quality.
- Pair Programming: Regularly sessioning with another developer can provide fresh perspectives on a problem. Pair programming often leads to better design and fewer bugs.
Final Thoughts
Legacy code may seem intimidating, but with the right strategies, it can become a well-refined and valued part of your development practice. By identifying priorities, incrementally refactoring, implementing tests, managing dependencies, and fostering collaboration, developers can gradually transform legacy systems into flexible and maintainable code.
While not every legacy system can be fully modernized, understanding its structure and identifying ways to improve it can lead to significant benefits. The journey can be challenging, but those who persevere will find a deeper appreciation for both legacy systems and their evolving craft.
For further reading on techniques for managing legacy code, check out Martin Fowler's Refactoring and Michael Feathers' Working Effectively with Legacy Code.
Embrace the challenge, learn from the legacy, and enjoy the process of becoming a better developer every day.