Mastering Final Variables in JavaFX: Common Pitfalls Explained
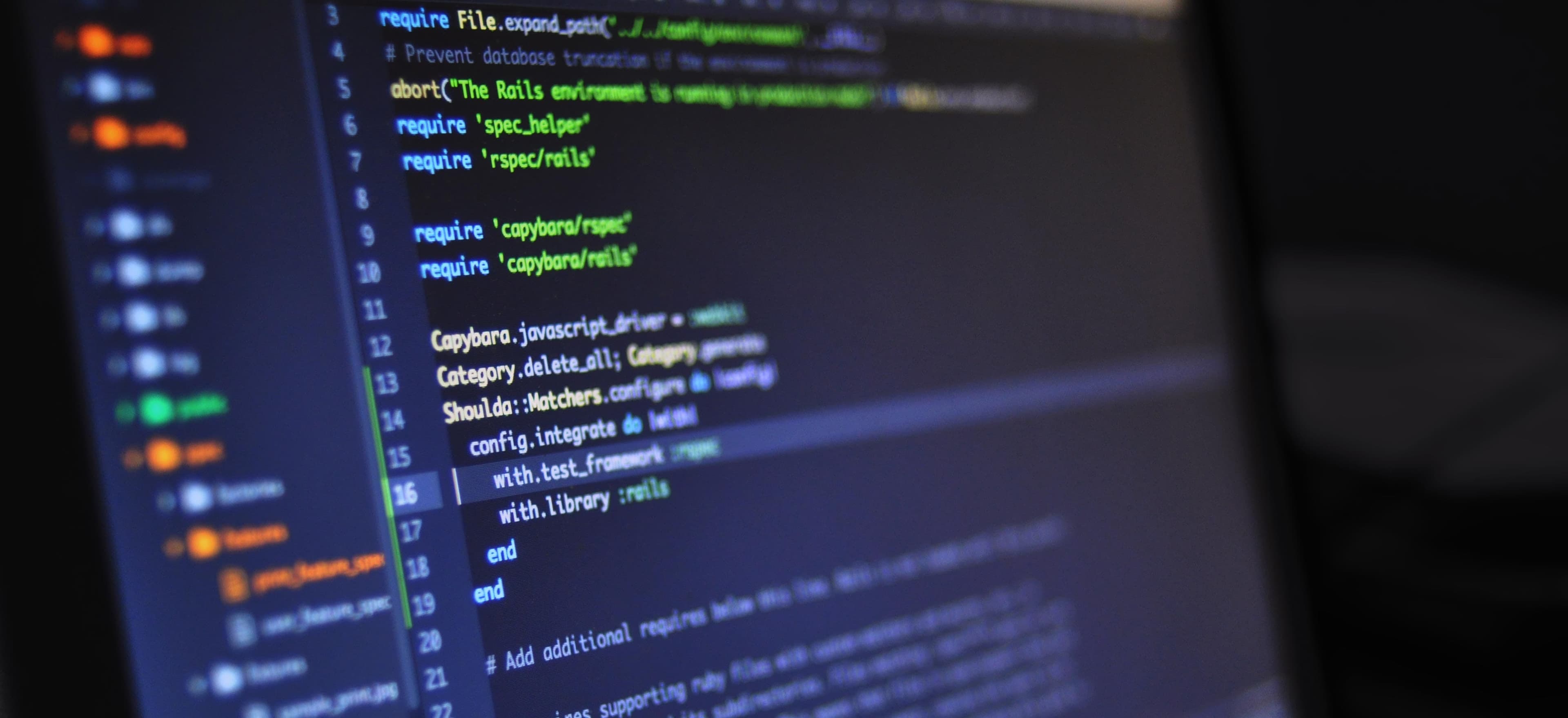
- Published on
Mastering Final Variables in JavaFX: Common Pitfalls Explained
In the world of Java programming, understanding and effectively utilizing the final
keyword can significantly impact your code quality and maintainability. This is especially true in the context of JavaFX development, where UI elements and their interactions often demand clarity and stability. In this blog post, we will delve into the intricacies of final variables, the nuances that come with them, and the common pitfalls that developers face when using them in JavaFX applications.
What Are Final Variables?
In Java, declaring a variable as final
means that once it has been assigned, its value cannot be altered. This immutability offers several benefits, primarily promoting consistency and reducing the likelihood of bugs arising from unintended modifications.
Consider the following example:
final int MAX_USERS = 100;
Here, MAX_USERS
is a constant that will always retain the value of 100 throughout the program. Attempting to change this value later on will result in a compilation error.
Benefits of Using Final Variables
- Immutability: Once defined, the value can't change. This minimizes risks related to side-effects.
- Maintainability: Future modifications are simplified. For instance, if you want to change the maximum user count, you only have to do it in one place.
- Readability: The use of
final
signals to readers that the variable is intended not to change, thus clarifying the programmer's intention.
Final Variables in JavaFX
When working with JavaFX, final
variables can play a crucial role, particularly in event handlers and asynchronous tasks. Often, JavaFX applications involve the creation of UI components and event listeners where the scope and behavior can be tricky to manage.
Common Pitfall #1: Final Local Variables in Anonymous Inner Classes
One of the most frequent issues that arise in JavaFX development is the scope of local variables when using them inside anonymous inner classes or lambda expressions. In Java, only effectively final variables can be accessed when inside an inner class, meaning they cannot be modified after declaration.
Example of the Issue
Button myButton = new Button("Click me");
int counter = 0; // Not effectively final
myButton.setOnAction(event -> {
counter++; // Compilation error: counter cannot be referenced from a lambda expression
});
In the above code snippet, trying to modify counter
within the lambda expression causes a compilation error. To resolve this, you should declare counter
as final
or use an array or a mutable object to hold the value.
Correct Approach
final int[] counter = new int[1]; // Use an array to simulate mutability
myButton.setOnAction(event -> {
counter[0]++;
System.out.println("Counter: " + counter[0]);
});
In this corrected example, we use a single-element array to achieve the desired mutability while adhering to the final
modifier constraints.
Common Pitfall #2: Using Final in JavaFX Property Bindings
JavaFX provides properties which bind together UI elements and model data, and sometimes developers get confused with the use of final
. Although the hold of final
can assure certain immutability, it can also lead to confusion.
In a typical scenario where you want to bind a property of a node to a model, you might mistakenly declare the property as final
:
final BooleanProperty isVisible = new SimpleBooleanProperty(true);
isVisible.set(!isVisible.get()); // Wrong manipulation might seem fine
While you can still manipulate the property value, you would not be able to reassign isVisible
to a different BooleanProperty
. Instead, it's usually advisable to declare properties without final
if you expect to reassign them.
Working Example Combining Final and JavaFX Properties
Here’s a complete working example illustrating how to use final variables with JavaFX properties effectively.
import javafx.application.Application;
import javafx.beans.property.SimpleBooleanProperty;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class FinalVariablesExample extends Application {
@Override
public void start(Stage primaryStage) {
final Button toggleButton = new Button("Toggle Visibility");
final SimpleBooleanProperty isVisible = new SimpleBooleanProperty(true);
// Bind the visibility of the button to the isVisible property
toggleButton.visibleProperty().bind(isVisible);
// Using final, but ensuring that the property itself can change
toggleButton.setOnAction(event -> {
isVisible.set(!isVisible.get()); // Toggle visibility
System.out.println("Button visible: " + isVisible.get());
});
StackPane root = new StackPane();
root.getChildren().add(toggleButton);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("JavaFX Final Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example:
- We declare the
Button toggleButton
andSimpleBooleanProperty isVisible
asfinal
. - The button's visibility is bound to the
isVisible
property. - Clicking the button toggles its visibility while ensuring clarity in intention with
final
.
Bringing It All Together
Mastering the use of final variables in Java and JavaFX is crucial for writing clean, maintainable code. By learning to navigate common pitfalls such as scoping issues with inner classes and understanding how properties function in binding, you can enhance your JavaFX applications remarkably.
Further Reading
By following the guidelines and examples provided in this post, you'll be well on your way to mastering final variables in JavaFX, making your applications more robust and less prone to errors. Remember, clarity and consistency are key! Happy coding!