Overcoming Deployment Downtime in Continuous Integration
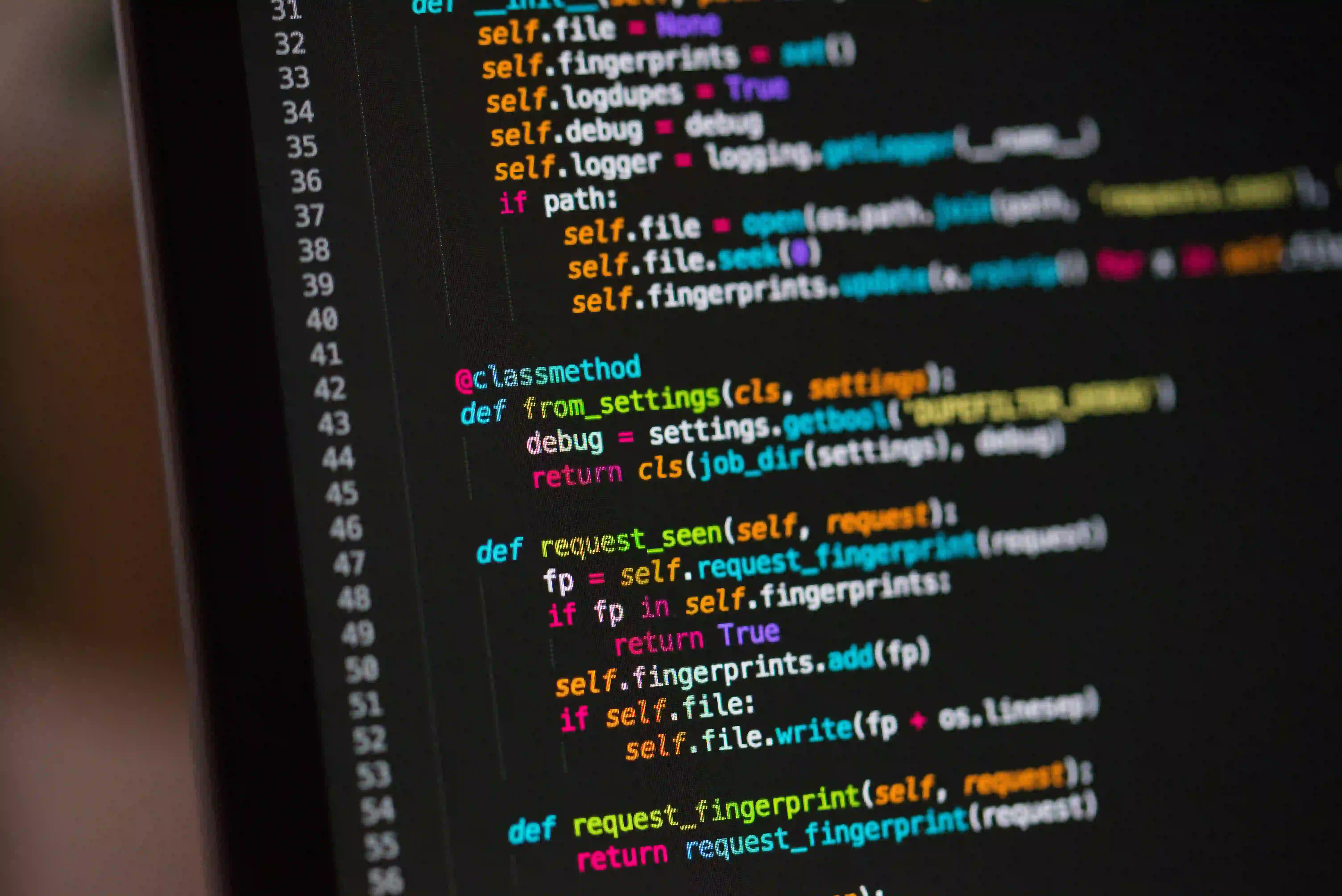
Overcoming Deployment Downtime in Continuous Integration
In the world of software development, the ability to deliver applications with speed and reliability is paramount. Continuous Integration (CI) aims to automate the integration of code changes from multiple contributors. However, the challenge of deployment downtime persists, often hindering the benefits of CI. In this blog post, we will explore effective strategies to minimize or eliminate deployment downtime in CI pipelines. We'll cover key techniques, code examples, and best practices that can enhance your CI/CD workflows.
Understanding Deployment Downtime
Deployment downtime refers to the period when an application is unable to serve users because of updates or changes being applied. Downtime can be frustrating for users and detrimental to businesses. It often occurs during:
- Code deployments: When new features, bug fixes, or performance updates are pushed to production.
- Infrastructure changes: When changes are made to the underlying hardware, servers, or configurations.
The goal of CI/CD is to automate and streamline these processes, ensuring that teams can deliver high-quality software without interrupting user experience.
The Importance of Zero-Downtime Deployments
Why Zero-Downtime?
Achieving zero-downtime deployments has several advantages:
- Enhanced User Experience: Users can access services without interruptions, boosting satisfaction.
- Business Continuity: Reduces the financial impact of downtime on revenue and operational costs.
- Faster Feedback Loops: Enables teams to integrate and deploy changes quickly, facilitating faster development cycles.
By focusing on strategies that facilitate zero-downtime deployments, you can leverage the full potential of CI processes.
Strategies to Overcome Deployment Downtime
1. Blue-Green Deployments
Blue-green deployments involve maintaining two identical environments, one active and one idle.
How it Works:
- Deploy the new version of your application to the idle environment.
- Run automated tests to validate that everything is functioning as expected.
- Switch traffic from the active environment to the newly deployed version.
Advantages:
- Instant rollback capability: If issues arise, you can revert to the previous version within moments.
- Seamless transitions for users.
Example Code Snippet:
Let's implement a simple blue-green deployment strategy using a configuration file:
# deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-green
spec:
replicas: 3
template:
metadata:
labels:
app: my-app
version: green
spec:
containers:
- name: my-app
image: my-app:latest
In this YAML configuration, we define a deployment for the green environment. To switch to this version, you can update your load balancer rules to route traffic to my-app-green
.
2. Canary Releases
A canary release involves rolling out the new version of your application to only a portion of your user base.
How it Works:
- Release the new version to a small percentage (e.g., 5-10%) of users.
- Monitor performance and user feedback closely.
- Gradually increase exposure if no issues are detected.
Advantages:
- Reduced risk: You can identify potential issues without destabilizing the entire application.
- More granular control over deployment.
Example Code Snippet:
Here is an example of using Kubernetes to implement a canary release:
# canary-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-canary
spec:
replicas: 1 # Start with a single replica
template:
metadata:
labels:
app: my-app
version: canary
spec:
containers:
- name: my-app
image: my-app:canary
Initially, this deployment will only have a single replica running the canary version. You can gradually scale up the replicas should everything go smoothly.
3. Feature Toggles
Feature toggles allow developers to turn features on or off in production without deploying code.
How it Works:
- Wrap new features in toggles enabled through configurations.
- Deploy the feature toggle in a dormant state.
- Activate the toggle to release the feature to users as needed.
Advantages:
- Greater control over feature visibility.
- Quicker fixes since code can be deployed without enabling new features.
Example Code Snippet:
Consider the following Java code snippet implementing a simple feature toggle:
public class FeatureToggle {
private boolean newFeatureEnabled;
public FeatureToggle(boolean newFeatureEnabled) {
this.newFeatureEnabled = newFeatureEnabled;
}
public void executeFeature() {
if (newFeatureEnabled) {
// Execute new feature code
System.out.println("New feature is active!");
} else {
// Execute old feature code
System.out.println("Legacy feature is active.");
}
}
}
In this example, the FeatureToggle
class allows the control of a new feature based on configuration. This ensures the application can be deployed constantly while letting developers safely experiment with features.
4. Database Migrations
Database migrations are an often overlooked but critical aspect of minimizing downtime. Traditional migrations may lead to disruptions, particularly with tables that are heavily in use.
Strategies:
- Backward-Compatible Changes: Always ensure database changes are compatible with the existing production code.
- Shadow Writes: Write to both new and old schemas during migration processes. Once verified, you can remove the old.
5. Infrastructure as Code (IaC)
Implementing IaC allows for the automation and consistency of infrastructure provisioning, ensuring that environments are predictable and easily replicable.
Using tools like Terraform, you can manage your infrastructure effectively, enabling quick rollbacks and recovery if something goes awry.
Example Code Snippet:
Here’s an example of a basic Terraform script to deploy an application:
# main.tf
resource "aws_instance" "app_server" {
ami = "ami-0c55b159cbfafe1fe"
instance_type = "t2.micro"
tags = {
Name = "MyAppInstance"
}
}
In this configuration file, we create an AWS EC2 instance for our application. Utilizing IaC not only simplifies the processes but also allows for versioning of infrastructure giving you more control during deployments.
Final Considerations
Overcoming deployment downtime in Continuous Integration requires a multi-faceted approach. Techniques like blue-green deployments, canary releases, feature toggles, and robust database migration strategies are essential. Moreover, leveraging Infrastructure as Code can streamline and secure your deployment processes.
Implementing these strategies will not only enhance the user experience but also empower your development team to deliver software quickly and effectively. As industry practices evolve, staying equipped with the right tools and methodologies is crucial for success.
For further reading on Continuous Integration best practices, check out articles from Martin Fowler or DevOps.com.
Embrace the power of CI/CD, minimize downtime, and watch your application soar!