Avoiding Common Pitfalls in JSF-PrimeFaces-Hibernate Integration
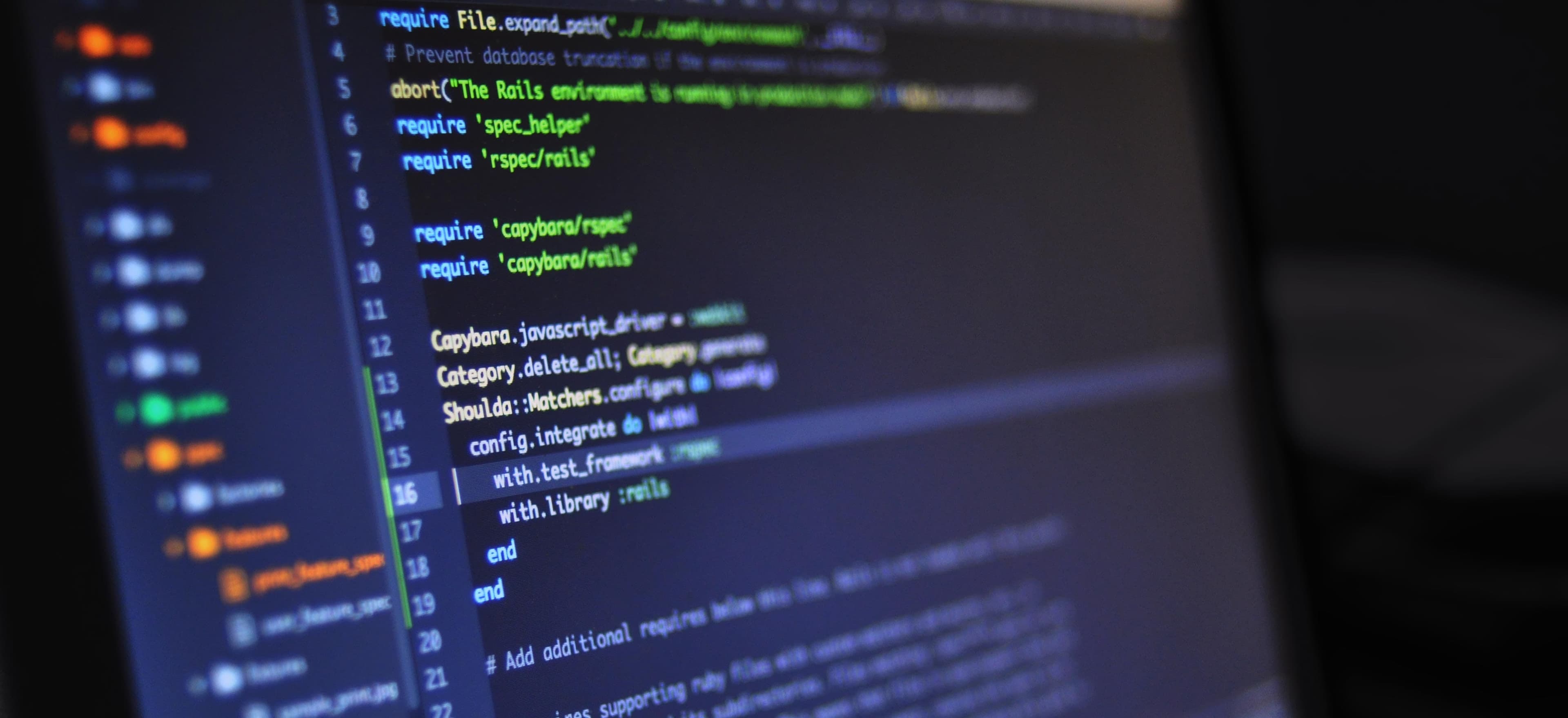
- Published on
Avoiding Common Pitfalls in JSF-PrimeFaces-Hibernate Integration
When developing Java web applications, the trio of JSF (JavaServer Faces), PrimeFaces, and Hibernate offers a robust and scalable solution. However, new developers often encounter challenges that can lead to frustrating setbacks. This blog post explores common pitfalls in integrating these technologies and how to avoid them, providing clear explanations and code snippets to help solidify your understanding.
Understanding the Stack
Before we dive in, let's get a brief overview of each technology in this stack:
-
JSF: A component-based web framework that simplifies the development of user interfaces for Java EE applications.
-
PrimeFaces: A popular JSF library that provides a rich set of UI components, enhancing the developer experience.
-
Hibernate: An Object-Relational Mapping (ORM) tool for Java that simplifies database interactions.
By leveraging these technologies together, you can create dynamic, data-driven web applications. However, improper integration can lead to various issues.
Common Pitfalls and How to Avoid Them
1. Bean Scoping Issues
Problem: One of the most frequent issues in JSF is bean scope, particularly when using @ViewScoped
beans with data modification operations. Incorrect scoping can lead to stale data or unexpected behaviors.
Solution: Choose the right scope for your beans based on your application's needs. For instance, use @RequestScoped
for simple fetch operations and @ViewScoped
when you need to maintain data throughout the user's interaction with a view.
Here's an example of a @ViewScoped
bean:
import javax.faces.view.ViewScoped;
import javax.inject.Named;
import java.io.Serializable;
@Named
@ViewScoped
public class UserBean implements Serializable {
private User user = new User();
public void saveUser() {
// Save user using Hibernate
// This method interacts with the database
}
public User getUser() {
return user;
}
}
Why this is important: Using the wrong scope can lead to data cleanup issues or additional database hits when the user navigates through the application.
2. Validation and Conversion Issues
Problem: JSF components perform validation and conversion automatically. However, mismatches in data types between the UI and your Hibernate entity can cause exceptions during data processing.
Solution: Implement custom converters to handle type conversions explicitly, ensuring that data is transformed correctly between the view and model layers.
Example of a custom converter:
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.convert.Converter;
import javax.faces.convert.FacesConverter;
@FacesConverter(value = "userConverter")
public class UserConverter implements Converter {
@Override
public Object getAsObject(FacesContext context, UIComponent component, String value) {
// Convert string id to User object
}
@Override
public String getAsString(FacesContext context, UIComponent component, Object value) {
// Convert User object to string id
}
}
Why this is critical: Properly handling types prevents runtime exceptions, ensuring a smoother user experience.
3. Lazy Loading Misunderstandings
Problem: Hibernate's lazy loading can lead to LazyInitializationException
errors when entitities are accessed outside of an active session.
Solution: Use eager fetching judiciously or ensure your Hibernate session is open when accessing lazily-loaded properties.
Example of eager fetching:
@Entity
@NamedQuery(name="User.findAll", query="SELECT u FROM User u JOIN FETCH u.orders")
public class User {
@OneToMany(fetch = FetchType.EAGER)
private Set<Order> orders;
}
Why this matters: Understanding how Hibernate manages sessions can prevent unexpected behaviors during data retrieval.
4. Transaction Management
Problem: Managing transactions improperly can lead to database inconsistencies or failures.
Solution: Use the @Transactional
annotation in a service layer to ensure that your database operations are conducted within a transactional context.
Here's a simplified example using Spring:
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUser(User user) {
userRepository.save(user);
}
}
Why this is essential: Proper transaction management ensures your data remains consistent in multi-step processes.
5. Unsynchronized State between UI and Backing Beans
Problem: Changes made in the backing bean may not reflect in the UI, causing developers to believe their data is up-to-date.
Solution: Utilize update
attributes in PrimeFaces components to synchronize the UI when actions are performed.
Example using PrimeFaces:
<p:commandButton value="Save" action="#{userBean.saveUser()}" update="form" />
Why synchronization is key: This ensures that all UI updates reflect the latest state of your managed beans.
6. Over Fetching Data
Problem: Fetching unnecessary data can slow down your application, as fetching large datasets not only increases load times but can also lead to memory issues.
Solution: Define the data you need explicitly, possibly through JPQL or Criteria API.
Example of a simple JPQL query:
public List<User> findActiveUsers() {
return entityManager.createQuery("SELECT u FROM User u WHERE u.active = true", User.class).getResultList();
}
Why is this crucial?: Optimizing data retrieval minimizes performance issues and enhances user experience.
The Last Word
Integrating JSF, PrimeFaces, and Hibernate offers developers a powerful framework for building dynamic web applications. By being mindful of common pitfalls—such as bean scoping issues, validation and conversion missteps, and improper transaction management—you can avoid potential headaches and create a more robust application.
Additional Resources
For further reading, you may find these resources helpful:
- JSF Official Documentation
- PrimeFaces User Guide
- Hibernate ORM User Guide
By following these guidelines and leveraging best practices, you can successfully navigate the integration of JSF, PrimeFaces, and Hibernate in your Java applications. Happy coding!